Explain the concept of API routes in Next.js
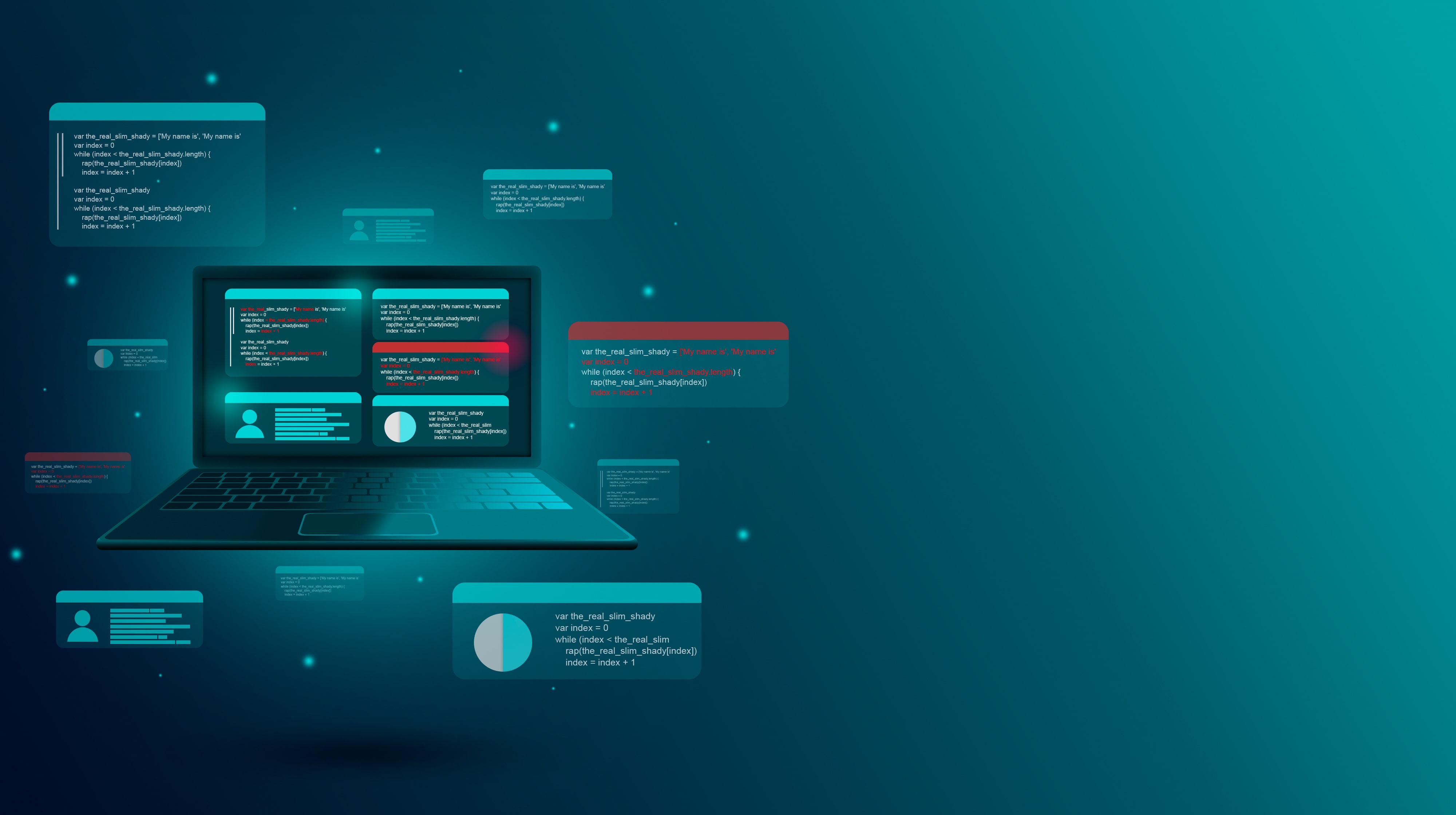
Next.js, a popular React framework, is known for its powerful features that simplify the development of modern web applications. One such feature is API routes, which allow developers to create serverless functions to handle various server-side tasks directly within a Next.js project. In this blog post, we will delve into the concept of API routes in Next.js, exploring their benefits, how to create them, and best practices for using them effectively.
What Are API Routes?
API routes in Next.js provide a way to define server-side endpoints directly within your application. These routes can be used to handle HTTP requests, perform server-side logic, and interact with databases or external APIs. Essentially, API routes enable you to create backend functionality within a Next.js app without the need for an additional server or backend framework.
Benefits of API Routes
1. Serverless Architecture
API routes in Next.js run in a serverless environment, meaning they are executed on-demand in response to HTTP requests. This eliminates the need to manage server infrastructure, reduces operational overhead, and scales automatically with traffic.
2. Simplified Development
With API routes, you can keep both frontend and backend code within the same project, simplifying development and maintenance. This unified approach streamlines the process of building full-stack applications.
3. Built-in Routing
Next.js handles routing for API routes automatically based on the file structure within the pages/api
directory. This eliminates the need to configure routing manually, making it easier to define endpoints.
4. Integration with Next.js Features
API routes seamlessly integrate with other Next.js features, such as server-side rendering (SSR) and static site generation (SSG), allowing you to build dynamic and performant applications.
Creating API Routes
1. Basic Setup
To create an API route in Next.js, you need to add a JavaScript or TypeScript file to the pages/api
directory. The filename determines the endpoint’s URL path. For example, creating a file pages/api/hello.js
will expose an endpoint at /api/hello
.
// pages/api/hello.js
export default function handler(req, res) {
res.status(200).json({ message: 'Hello, World!' });
}
In this example, the handler
function takes two arguments, req
(the request object) and res
(the response object), and sends a JSON response with a status code of 200.
2. Handling HTTP Methods
API routes can handle different HTTP methods such as GET, POST, PUT, DELETE, etc. You can use conditional statements to handle different methods within a single route.
// pages/api/user.js
export default function handler(req, res) {
const { method } = req;
switch (method) {
case 'GET':
// Handle GET request
res.status(200).json({ name: 'John Doe' });
break;
case 'POST':
// Handle POST request
const { name } = req.body;
res.status(201).json({ name });
break;
default:
res.setHeader('Allow', ['GET', 'POST']);
res.status(405).end(`Method ${method} Not Allowed`);
}
}
In this example, the route handles both GET and POST requests, responding with different data based on the request method.
3. Accessing Query Parameters
You can access query parameters from the request object in API routes. These parameters are useful for filtering, searching, or fetching specific data.
// pages/api/user/[id].js
export default function handler(req, res) {
const { id } = req.query;
res.status(200).json({ userId: id });
}
In this example, the route extracts the id
parameter from the URL and includes it in the JSON response.
4. Interacting with Databases and External APIs
API routes are commonly used to interact with databases and external APIs. You can use any database client or HTTP request library within your API route functions.
// pages/api/users.js
import { MongoClient } from 'mongodb';
const uri = process.env.MONGODB_URI;
const client = new MongoClient(uri);
export default async function handler(req, res) {
await client.connect();
const db = client.db('mydatabase');
const users = await db.collection('users').find().toArray();
res.status(200).json(users);
}
In this example, the route connects to a MongoDB database, retrieves a list of users, and returns the data as a JSON response.
Best Practices for API Routes
1. Secure Sensitive Information
Avoid exposing sensitive information such as API keys or database credentials directly in your code. Use environment variables to securely manage these values.
2. Error Handling
Implement robust error handling within your API routes to gracefully handle unexpected issues. This includes returning appropriate HTTP status codes and error messages.
// pages/api/user.js
export default async function handler(req, res) {
try {
// Perform database operations
const user = await getUserFromDatabase();
res.status(200).json(user);
} catch (error) {
res.status(500).json({ error: 'Internal Server Error' });
}
}
3. Input Validation
Validate incoming data to ensure it meets expected formats and requirements. This helps prevent issues such as invalid data or security vulnerabilities.
// pages/api/user.js
import Joi from 'joi';
const schema = Joi.object({
name: Joi.string().min(3).required(),
});
export default function handler(req, res) {
const { error } = schema.validate(req.body);
if (error) {
return res.status(400).json({ error: error.details[0].message });
}
// Handle valid input
res.status(201).json({ name: req.body.name });
}
4. Optimize Performance
Minimize response times by optimizing database queries and reducing unnecessary computations. Caching can also be used to improve performance for frequently accessed data.
Conclusion
API routes in Next.js offer a powerful way to create serverless functions and manage backend functionality directly within your application. By understanding how to create and use API routes effectively, you can build dynamic, secure, and scalable applications with ease. Implementing best practices such as securing sensitive information, handling errors, validating input, and optimizing performance will help ensure that your API routes are robust and reliable. With these tools and techniques, you can take full advantage of Next.js to create feature-rich web applications.
What are the best practices for structuring a Next.js project
What is the significance of the api folder in a Next.js project
What is the purpose of the basePath and assetPrefix options in next.config.js
What is the significance of the _document.js file in a Next.js project
How does Next.js handle data fetching for server-side rendering (SSR)
Explain how to use environment variables in a Next.js application