How do I fix "undefined" or "null" errors in JavaScript
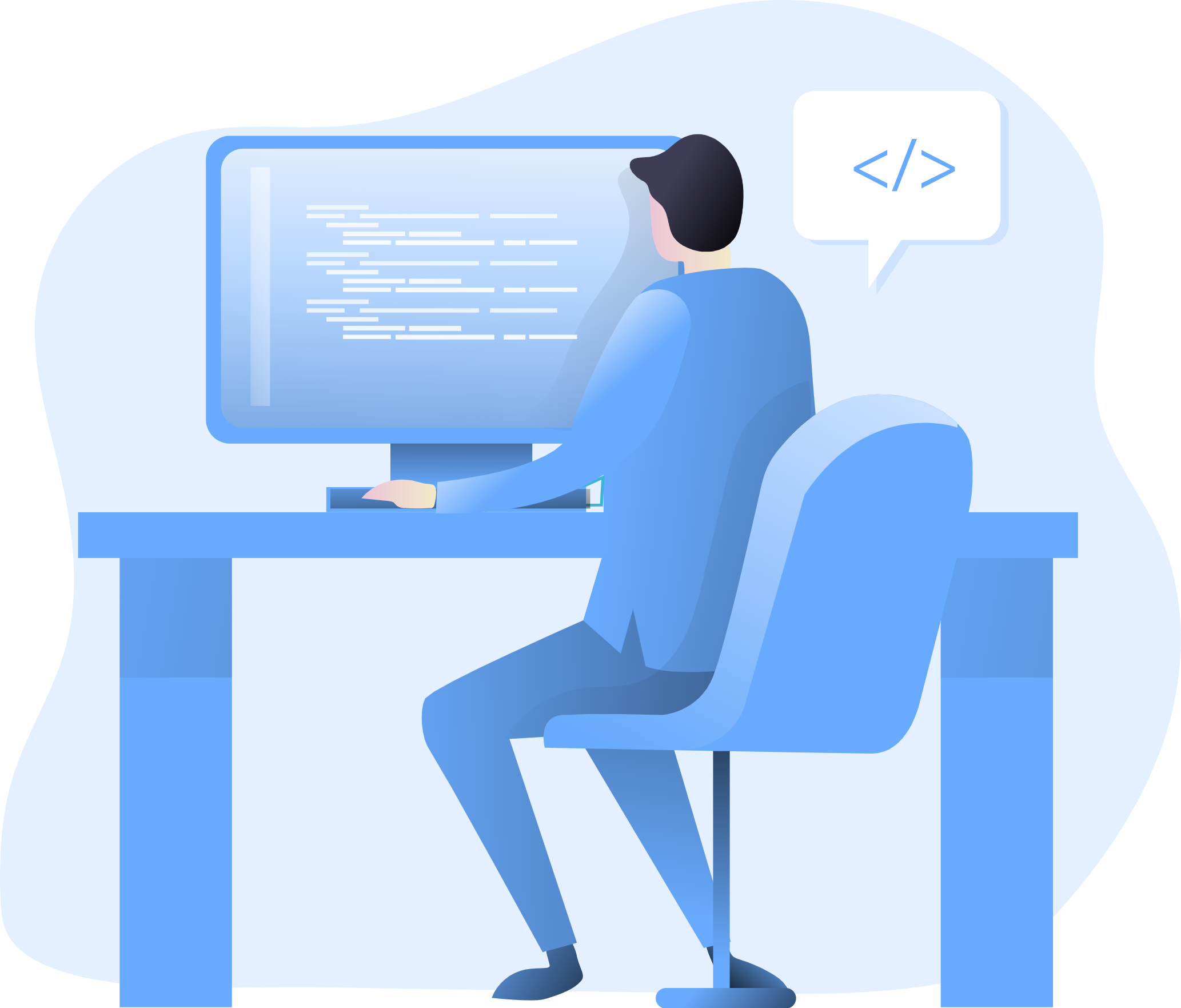
JavaScript developers often encounter errors related to undefined
or null
values. These errors can lead to unexpected behavior, especially when working with dynamic or loosely typed data. Understanding what undefined
and null
mean, why they occur, and how to fix them is crucial to writing robust and error-free JavaScript code.
In this detailed guide, we’ll cover the concepts of undefined
and null
, explore common causes for these errors, and offer strategies for fixing them.
What are undefined
and null
in JavaScript?
In JavaScript, undefined
and null
are two special values that represent “nothing” or the absence of a value. However, they are not the same and behave differently:
-
undefined
: This value is automatically assigned to a variable when it is declared but not yet initialized. It also appears when a function doesn’t return a value, or when accessing an object property that doesn’t exist.let a; console.log(a); // undefined
-
null
: This is an intentional assignment to indicate the absence of any object value. Developers usenull
to manually clear a variable or signal that an object property is empty.let b = null; console.log(b); // null
In general, undefined
is more common in JavaScript than null
, and many errors happen when variables are mistakenly left uninitialized.
Common Causes of undefined
and null
Errors
1. Uninitialized Variables
One of the most common reasons for undefined
errors is using a variable that hasn’t been given a value. When a variable is declared but not initialized, JavaScript automatically assigns it the value undefined
.
Example:
let x;
console.log(x); // undefined
Fix:
Always initialize variables when declaring them:
let x = 10;
console.log(x); // 10
2. Missing Function Return Values
If a function doesn’t explicitly return a value, JavaScript returns undefined
by default. Using this returned value without checking can cause issues.
Example:
function doSomething() {
// no return statement
}
let result = doSomething();
console.log(result); // undefined
Fix:
Ensure that your functions return values if you plan to use their output.
function doSomething() {
return "done";
}
let result = doSomething();
console.log(result); // "done"
3. Accessing Nonexistent Object Properties
Another common source of undefined
errors occurs when trying to access an object property that doesn’t exist.
Example:
let person = { name: "Alice" };
console.log(person.age); // undefined
Fix:
Check if the property exists before accessing it, or use default values:
let age = person.age || 18;
console.log(age); // 18
4. Nonexistent DOM Elements
In web development, JavaScript often interacts with the Document Object Model (DOM). If you try to access a DOM element that doesn’t exist, it returns null
.
Example:
let button = document.getElementById("submit-btn");
console.log(button); // null (if element with id "submit-btn" doesn't exist)
Fix:
Always check if the element exists before using it:
let button = document.getElementById("submit-btn");
if (button) {
button.addEventListener("click", () => alert("Clicked!"));
}
5. Incorrect JSON Parsing
When dealing with JSON data, null
values can often appear when a key doesn’t exist or is explicitly set to null
. This can cause unexpected behavior if not handled properly.
Example:
let jsonString = '{"name": "Alice", "age": null}';
let person = JSON.parse(jsonString);
console.log(person.age); // null
Fix:
Check if the value exists or use a fallback:
let age = person.age ?? 18; // Use nullish coalescing to set a default value
console.log(age); // 18
6. Asynchronous Code (Promises, Callbacks)
When working with asynchronous code, like fetch()
or promises, it’s common to deal with data that hasn’t arrived yet. Improper handling of promises can lead to undefined
or null
errors.
Example:
fetch("/api/data").then(response => {
let data = response.data; // If response.data doesn't exist, this will be undefined
console.log(data);
});
Fix:
Always handle promises properly and check for null or undefined data:
fetch("/api/data")
.then(response => response.json())
.then(data => {
if (data) {
console.log(data);
} else {
console.error("Data is undefined or null.");
}
});
How to Handle undefined
and null
Safely
1. Default Parameters
When defining a function, you can set default parameter values to avoid undefined
issues when arguments are missing.
Example:
function greet(name = "Guest") {
console.log("Hello, " + name);
}
greet(); // Hello, Guest
2. Using Conditional (Ternary) Operators
You can use the ternary operator to check if a value is undefined
or null
and provide a default.
Example:
let user = null;
let username = user ? user.name : "Anonymous";
console.log(username); // Anonymous
3. Optional Chaining (?.
)
The optional chaining operator (?.
) allows you to safely access nested object properties without causing an error if any part of the chain is undefined
or null
.
Example:
let user = { name: "Alice" };
console.log(user?.address?.street); // undefined (no error)
4. The Nullish Coalescing Operator (??
)
The nullish coalescing operator (??
) is used to provide a fallback value when dealing with null
or undefined
. Unlike ||
, it doesn’t consider falsy values like 0
or false
.
Example:
let userScore = 0;
let score = userScore ?? 100; // 0 is a valid value, so it's used instead of 100
console.log(score); // 0
Practical Solutions
1. Debugging with typeof
The typeof
operator helps you determine if a variable is undefined
, null
, or another type.
Example:
let a;
console.log(typeof a); // "undefined"
let b = null;
console.log(typeof b); // "object"
This is especially useful when debugging to ensure variables have the correct type before performing operations on them.
2. Avoiding Mistakes with Strict Equality (===
and !==
)
JavaScript uses both loose equality (==
) and strict equality (===
). To avoid unexpected behavior when comparing undefined
and null
, use strict equality (===
), which checks both value and type.
Example:
console.log(null == undefined); // true (loose equality)
console.log(null === undefined); // false (strict equality)
Using strict equality avoids issues that arise from implicit type conversions in JavaScript.
3. Checking Object Properties Safely
Before accessing object properties, always verify if they exist to avoid undefined
errors.
Example:
let user = { name: "Alice" };
if (user && user.name) {
console.log(user.name);
} else {
console.log("Name is undefined");
}
Summary and Conclusion
Dealing with undefined
and null
values in JavaScript is a common source of errors, but with careful coding practices, these issues can be mitigated. Key strategies include initializing variables, checking for missing function return values, using default parameters, and handling asynchronous code carefully.
Key Takeaways:
undefined
is automatically assigned when variables are declared but not initialized or when accessing nonexistent object properties.null
is an intentional absence of a value, often used by developers to indicate “no value.”- Safeguard your code by using default values, optional chaining, the nullish coalescing operator, and strict equality to avoid unexpected errors.
- Always validate data when dealing with external sources like APIs or the DOM.
By applying these techniques, you’ll reduce the number of undefined
or null
errors in your code, leading to more reliable and maintainable applications.
How to set up and use Terraform for infrastructure as code
What is AI and how can I integrate it into my applications
How to resolve CORS errors in a web application
How to choose between AWS, Azure, and Google Cloud for my application