How do I integrate third-party libraries or CSS components into a Tailwind CSS project?
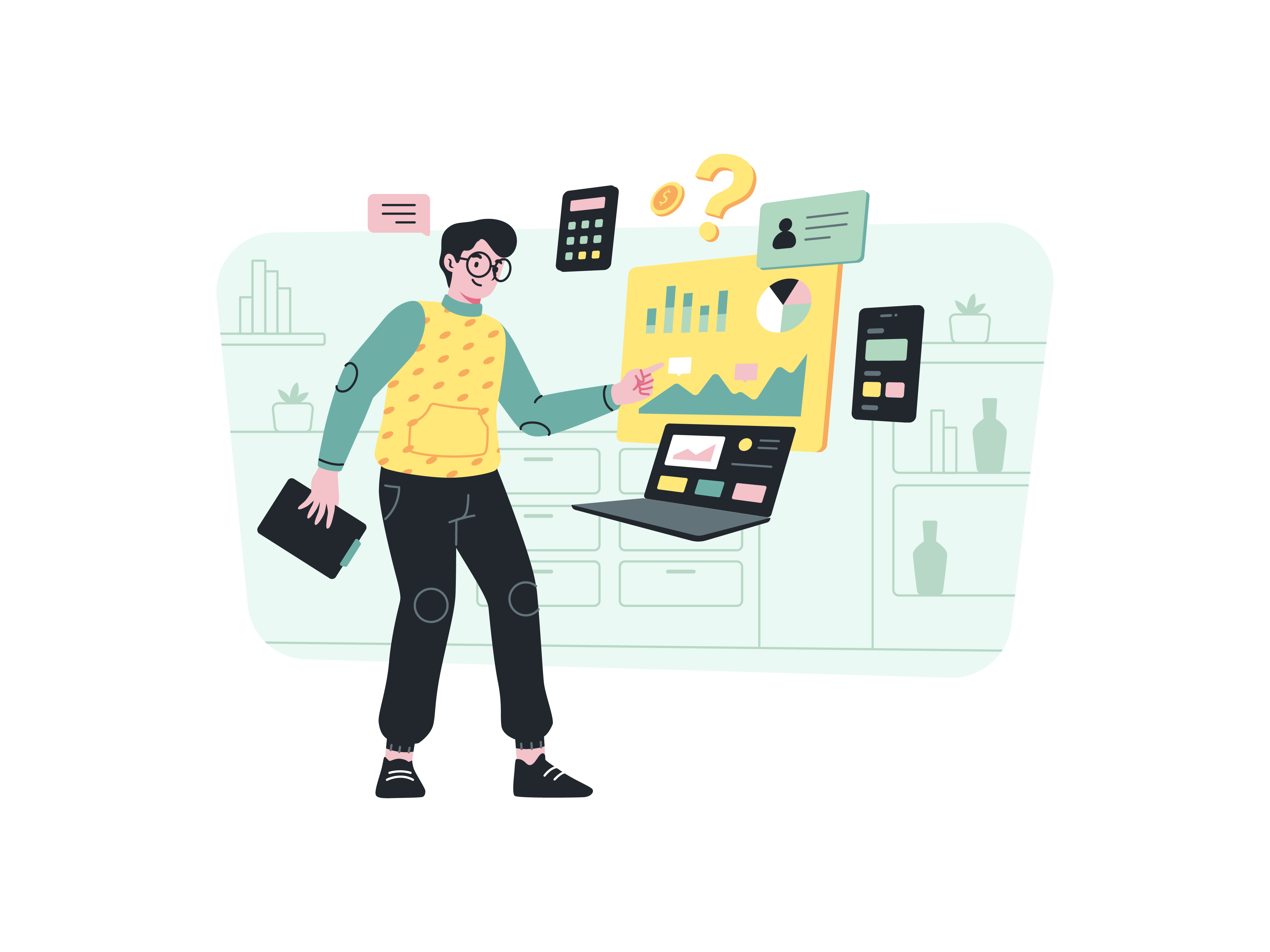
Integrating Third-Party Libraries and CSS Components into a Tailwind CSS Project
Tailwind CSS is an extremely powerful and versatile framework for building responsive designs quickly and efficiently. However, sometimes you may need to integrate third-party libraries or CSS components into your project to achieve a specific design or functionality. In this article, we will explore how to do just that while maintaining the integrity of your Tailwind CSS project.
Understanding Tailwind CSS’s Flexible Nature
Before diving into the process of integrating third-party libraries and components, it’s essential to understand the flexible nature of Tailwind CSS. Unlike other CSS frameworks that enforce a rigid structure, Tailwind CSS is designed to be highly adaptable and customizable. This means you can easily incorporate third-party libraries and components into your project without disrupting the existing framework.
Approaches to Integrating Third-Party Libraries and Components
There are two primary approaches to integrating third-party libraries and components into a Tailwind CSS project:
- Inline Inclusion: In this approach, you include the third-party library or component directly within your Tailwind CSS configuration file using the
@import
rule. This method is straightforward and works well when the third-party resource is not extensive. However, it can lead to longer configuration files and may cause conflicts with other styles if not done carefully. - External Inclusion: In this approach, you keep the third-party library or component separate from your Tailwind CSS configuration file. This method allows for better organization and maintenance of your codebase while preventing conflicts between different styles. To use an external resource, you need to create a new file with the appropriate import statements and then include it in your Tailwind CSS configuration file using the
extend
rule.
Inline Inclusion Example
Let’s say you want to integrate a third-party library like Font Awesome into your Tailwind CSS project. Here’s an example of how to do it using inline inclusion:
// tailwind.config.js
module.exports = {
theme: {
// ...
fontFamily: ['Inter', 'Roboto', 'Arial', 'sans-serif'],
// Include Font Awesome
@import 'https://use.typekit.com/aq0bmyi';
},
// ...
};
In this example, we’ve added the @import
rule to include the Font Awesome stylesheet in our Tailwind CSS configuration file. This will allow us to use Font Awesome icons throughout our project.
External Inclusion Example
Now let’s consider integrating a third-party CSS component like a date picker library. Here’s an example of how to do it using external inclusion:
// tailwind.config.js
module.exports = {
theme: {
// ...
},
extend: {
// Include the date picker library
'date-picker': require('@fortawesome/fontawesome-free/css/all.min.css'),
},
// ...
};
In this example, we’ve created a new file named date-picker.css
that contains the import statements for the date picker library:
// date-picker.css
@import 'https://use.typekit.com/aq0bmyi';
@import 'https://cdnjs.cloudflare.com/ajax/libs/bootstrap-datepicker/1.9.0/css/bootstrap-datepicker3.min.css';
We’ve then included this file in our Tailwind CSS configuration file using the extend
rule, which allows us to use the date picker component throughout our project while maintaining a clean and organized codebase.
Conclusion
Incorporating third-party libraries and components into a Tailwind CSS project is a straightforward process that can enhance your design and functionality without disrupting the existing framework. By understanding the flexible nature of Tailwind CSS and the two primary approaches to integration, you’ll be well on your way to building robust and responsive web applications with ease. Happy coding!