How do I optimize the CSS output for production in Tailwind CSS?
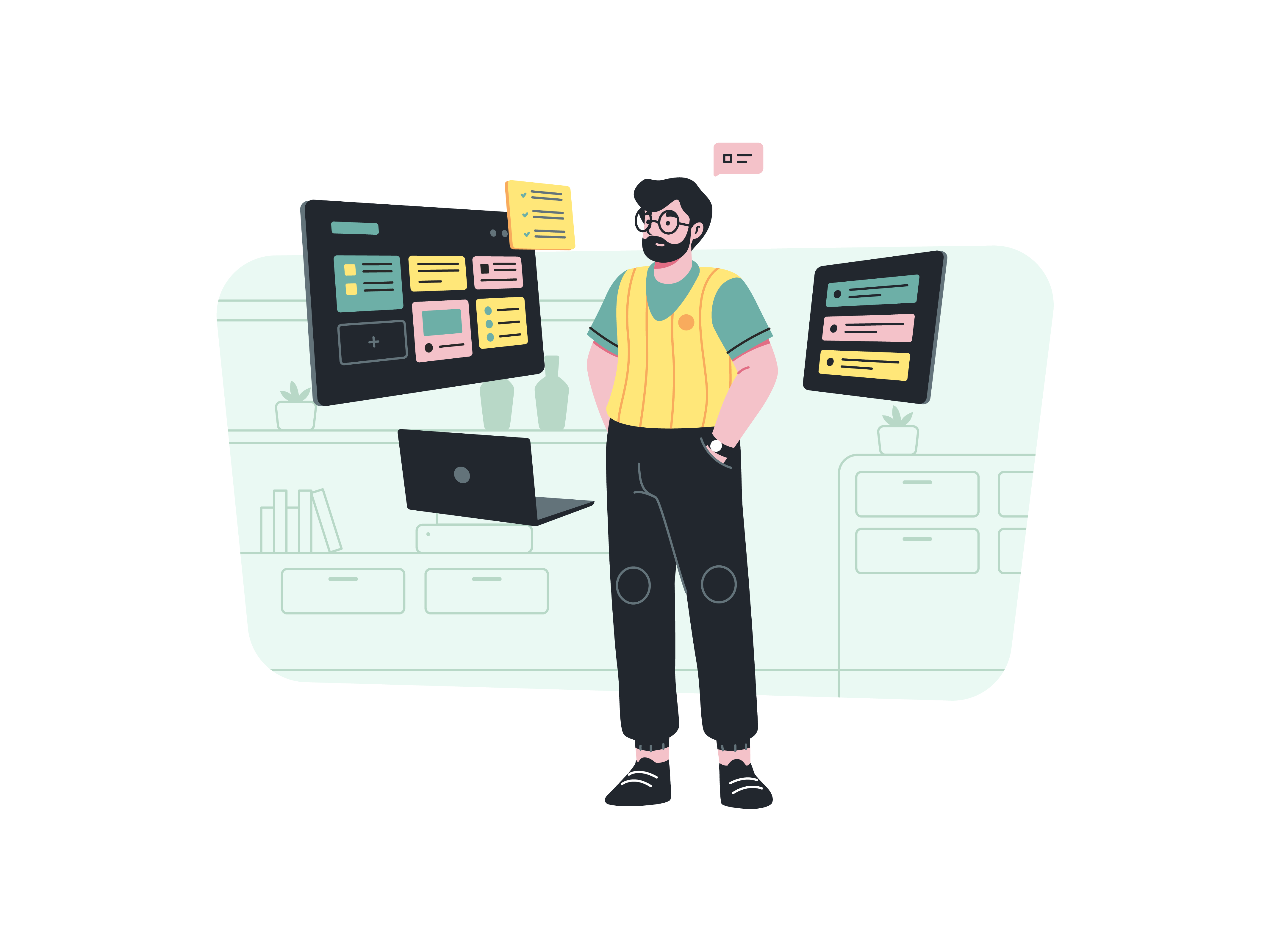
When it comes to deploying a web application, there are several factors to consider, such as performance and load times. One of the most critical aspects is optimizing the CSS output for production. Tailwind CSS provides various features that enable you to achieve this goal efficiently. In this article, we will explore how to optimize the CSS output for production in Tailwind CSS.
1. Purge Unused CSS
One of the primary reasons for optimizing CSS is to remove unused styles from your final bundle. Tailwind CSS provides a built-in purge feature that helps you achieve this goal. To use the purge feature, you need to run tailwindcss --purge
before building your application. This command will remove all unused CSS classes, leaving only the essential styles in your output.
2. Use @apply
Another way to optimize CSS output is by using the @apply
directive. This feature allows you to apply multiple classes at once, reducing the number of selectors in your CSS. Instead of writing flex-direction: column; align-items: center;
, you can use @apply flex-direction-column align-items-center
. This approach not only simplifies your code but also reduces the amount of output.
3. Use Variants
Tailwind CSS provides a powerful feature called variants, which enables you to create custom themes and variations of your design. By using variants, you can reduce the number of CSS classes in your output. For example, instead of writing text-xl font-bold
, you can use text-xl-variant font-bold-variant
. This approach helps you keep your code organized and maintainable while reducing the overall size of your CSS bundle.
4. Preprocessors
Preprocessors like Sass or Less provide more advanced features for optimizing CSS output. Tailwind CSS supports both Sass and Less, allowing you to write more efficient and modular code. By using preprocessors, you can create reusable functions and variables, reducing the amount of repetitive code in your output.
5. Use a CDN
Finally, consider using a Content Delivery Network (CDN) to serve your CSS files. A CDN will cache your CSS files across different locations, reducing the load time for your users. Tailwind CSS provides seamless integration with popular CDNs like Cloudflare and MaxCDN. By using a CDN, you can significantly improve your website’s performance without sacrificing the functionality of your application.
In conclusion, optimizing the CSS output for production in Tailwind CSS is crucial for delivering a seamless user experience. By using the purge feature, @apply directive, variants, preprocessors, and CDN, you can reduce the size of your CSS bundle and improve the load times of your application. Remember, every byte counts when it comes to web performance, so make sure to take advantage of these features to optimize your Tailwind CSS output.