How do you use Svelte with Firebase
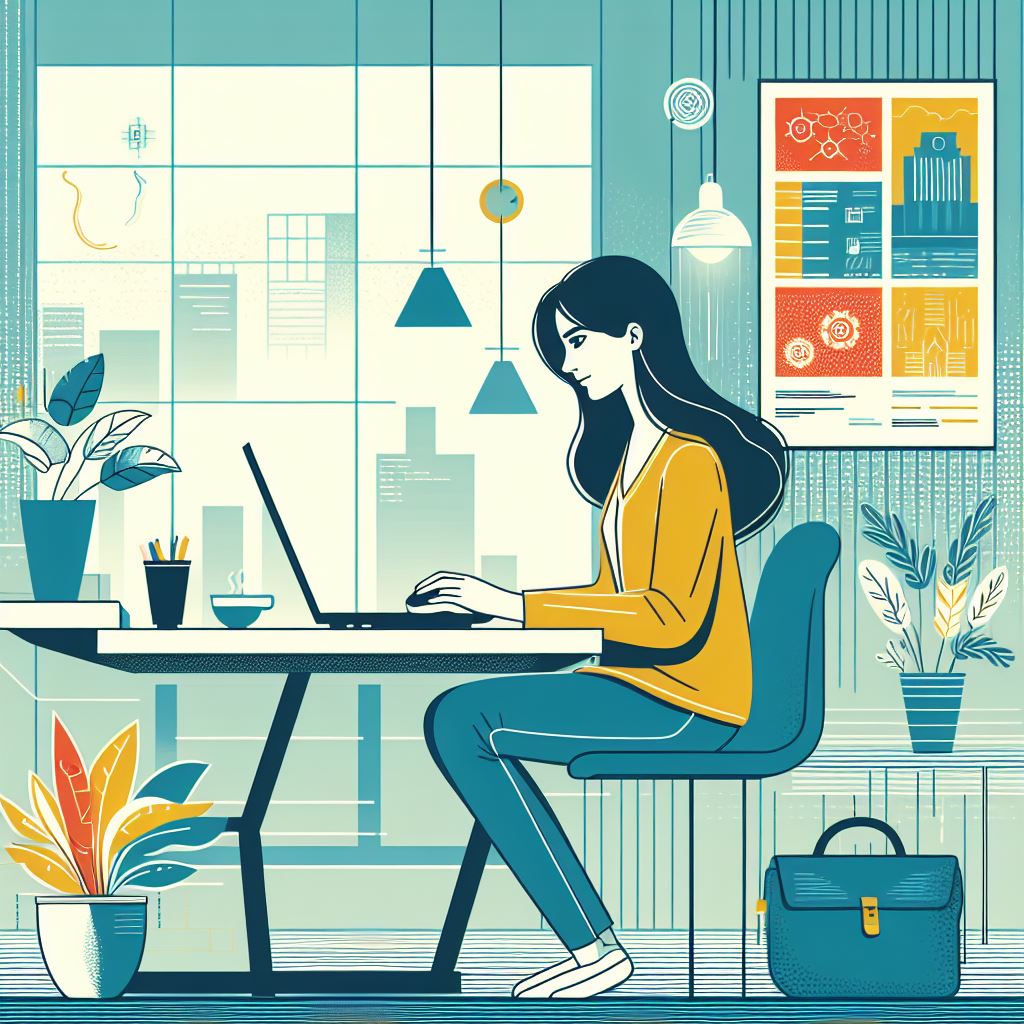
Integrating Svelte with Firebase allows you to create powerful, real-time web applications with ease. Firebase provides a comprehensive suite of backend services such as real-time databases, authentication, cloud functions, and more. In this article, we’ll explore how to use Firebase with Svelte, covering topics like setting up Firebase, integrating authentication, using Firestore for real-time data, and deploying your Svelte app to Firebase Hosting.
1. Setting Up Firebase
Step 1: Create a Firebase Project
- Go to the Firebase Console.
- Click on “Add Project” and follow the setup wizard to create a new project.
- Once the project is created, navigate to the project dashboard.
Step 2: Register Your App
- In the Firebase Console, click on the “Web” icon to register a new web app.
- Follow the instructions to register your app and obtain the Firebase configuration object.
Step 3: Install Firebase SDK
-
In your Svelte project, install the Firebase SDK:
npm install firebase
2. Creating a Svelte Project
Step 1: Set Up a New Svelte Project
-
Create a new Svelte project using the template:
npx degit sveltejs/template svelte-firebase cd svelte-firebase npm install
Step 2: Start the Development Server
-
Start the development server:
npm run dev
-
Your Svelte project is now set up and running at
http://localhost:5000
.
3. Integrating Firebase with Svelte
Step 1: Initialize Firebase
-
Create a new file
src/firebase.js
and initialize Firebase with your configuration:// src/firebase.js import firebase from 'firebase/app'; import 'firebase/auth'; import 'firebase/firestore'; const firebaseConfig = { apiKey: "YOUR_API_KEY", authDomain: "YOUR_AUTH_DOMAIN", projectId: "YOUR_PROJECT_ID", storageBucket: "YOUR_STORAGE_BUCKET", messagingSenderId: "YOUR_MESSAGING_SENDER_ID", appId: "YOUR_APP_ID" }; // Initialize Firebase firebase.initializeApp(firebaseConfig); export const auth = firebase.auth(); export const firestore = firebase.firestore();
-
Replace the placeholders with your Firebase project configuration values.
Step 2: Use Firebase in Svelte Components
-
In your Svelte components, import the Firebase services:
<!-- src/App.svelte --> <script> import { auth, firestore } from './firebase'; </script> <main> <h1>Welcome to Svelte with Firebase!</h1> </main>
4. Firebase Authentication
Step 1: Implement Authentication
-
Create a new component
src/Auth.svelte
to handle user authentication:<!-- src/Auth.svelte --> <script> import { auth } from './firebase'; import { writable } from 'svelte/store'; let email = ''; let password = ''; let user = writable(null); let error = writable(null); const signUp = async () => { try { const userCredential = await auth.createUserWithEmailAndPassword(email, password); user.set(userCredential.user); } catch (err) { error.set(err.message); } }; const signIn = async () => { try { const userCredential = await auth.signInWithEmailAndPassword(email, password); user.set(userCredential.user); } catch (err) { error.set(err.message); } }; const signOut = async () => { await auth.signOut(); user.set(null); }; </script> <div> <h2>Authentication</h2> <input type="email" bind:value={email} placeholder="Email" /> <input type="password" bind:value={password} placeholder="Password" /> <button on:click={signUp}>Sign Up</button> <button on:click={signIn}>Sign In</button> <button on:click={signOut}>Sign Out</button> {#if $error} <p style="color: red">{$error}</p> {/if} {#if $user} <p>Welcome, {$user.email}</p> {/if} </div>
-
Update
App.svelte
to include theAuth
component:<!-- src/App.svelte --> <script> import Auth from './Auth.svelte'; </script> <main> <h1>Welcome to Svelte with Firebase!</h1> <Auth /> </main>
Step 2: Handling Auth State
-
Update
src/Auth.svelte
to listen for authentication state changes:<!-- src/Auth.svelte --> <script> import { auth } from './firebase'; import { writable } from 'svelte/store'; let email = ''; let password = ''; let user = writable(null); let error = writable(null); auth.onAuthStateChanged(currentUser => { user.set(currentUser); }); const signUp = async () => { try { const userCredential = await auth.createUserWithEmailAndPassword(email, password); user.set(userCredential.user); } catch (err) { error.set(err.message); } }; const signIn = async () => { try { const userCredential = await auth.signInWithEmailAndPassword(email, password); user.set(userCredential.user); } catch (err) { error.set(err.message); } }; const signOut = async () => { await auth.signOut(); user.set(null); }; </script> <div> <h2>Authentication</h2> <input type="email" bind:value={email} placeholder="Email" /> <input type="password" bind:value={password} placeholder="Password" /> <button on:click={signUp}>Sign Up</button> <button on:click={signIn}>Sign In</button> <button on:click={signOut}>Sign Out</button> {#if $error} <p style="color: red">{$error}</p> {/if} {#if $user} <p>Welcome, {$user.email}</p> {/if} </div>
5. Using Firestore with Svelte
Step 1: Setting Up Firestore
-
Create a new component
src/Firestore.svelte
to handle Firestore interactions:<!-- src/Firestore.svelte --> <script> import { firestore } from './firebase'; import { writable } from 'svelte/store'; let message = ''; let messages = writable([]); const addMessage = async () => { try { await firestore.collection('messages').add({ text: message, timestamp: firebase.firestore.FieldValue.serverTimestamp() }); message = ''; } catch (err) { console.error('Error adding message:', err); } }; firestore.collection('messages').orderBy('timestamp') .onSnapshot(snapshot => { const data = snapshot.docs.map(doc => ({ id: doc.id, ...doc.data() })); messages.set(data); }); </script> <div> <h2>Firestore Messages</h2> <input type="text" bind:value={message} placeholder="Type a message..." /> <button on:click={addMessage}>Send</button> <ul> {#each $messages as msg (msg.id)} <li>{msg.text}</li> {/each} </ul> </div>
-
Update
App.svelte
to include theFirestore
component:<!-- src/App.svelte --> <script> import Auth from './Auth.svelte'; import Firestore from './Firestore.svelte'; </script> <main> <h1>Welcome to Svelte with Firebase!</h1> <Auth /> <Firestore /> </main>
6. Deploying Svelte App to Firebase Hosting
Step 1: Install Firebase Tools
-
Install the Firebase CLI:
npm install -g firebase-tools
Step 2: Initialize Firebase Hosting
-
Initialize Firebase in your project directory:
firebase init
-
Follow the prompts to set up Firebase Hosting and select your Firebase project. Choose
dist
as the public directory (this is where the Svelte app will be built).
Step 3: Build and Deploy
-
Build your Svelte app:
npm run build
-
Deploy to Firebase Hosting:
firebase deploy
Your Svelte app is now deployed to Firebase Hosting!
Conclusion
By combining Svelte with Firebase, you can build powerful, real-time web applications with ease. In this article, we’ve covered how to set up Firebase, integrate authentication, use Firestore for real-time data, and deploy your Svelte app to Firebase Hosting. With these tools at your disposal, you can create dynamic and responsive applications that provide a seamless user experience.
How can you implement custom error handling in Next.js
How do you use TypeScript with Svelte
How do you handle authentication in Svelte
How do you test Svelte components
How do you use Svelte with static site generation