How does React differ from other JavaScript frameworks
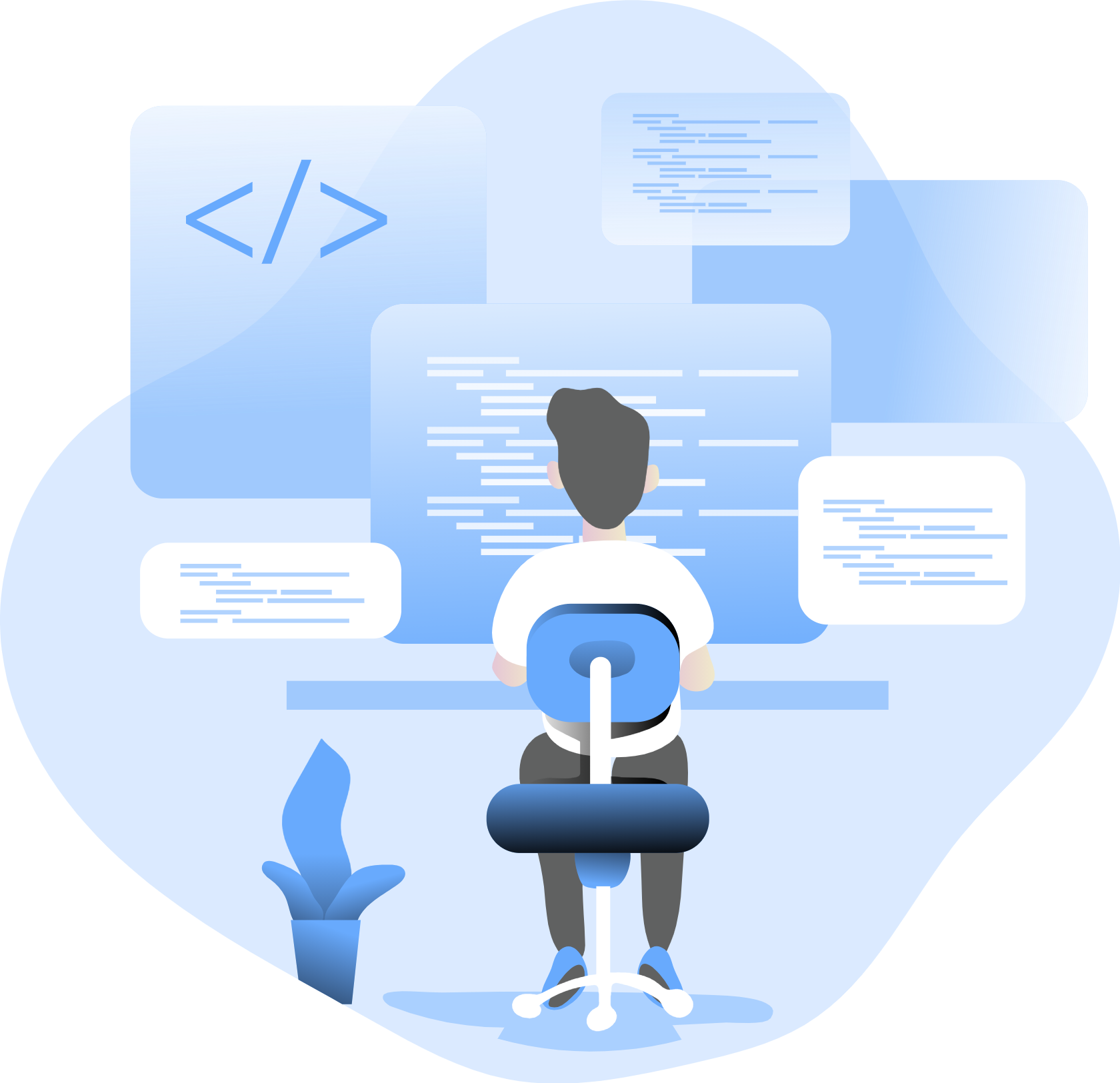
JavaScript frameworks have become integral components of web development, providing developers with tools and structures to build robust, interactive user interfaces. Among these frameworks, React stands out for its unique features and approach to building user interfaces. In this article, we will explore how React differs from other JavaScript frameworks.
1. Component-Based Architecture
One of the key differentiators of React is its emphasis on a component-based architecture. React allows developers to break down the user interface into modular components, each responsible for a specific piece of the UI. This approach promotes reusability, making it easier to manage and maintain complex UIs. Other frameworks may follow a different structure, such as the MVC (Model-View-Controller) architecture, which can lead to a steeper learning curve.
2. Virtual DOM
React introduced the concept of a Virtual DOM, which significantly improves performance. Instead of updating the entire DOM when changes occur, React compares the Virtual DOM with the actual DOM and updates only the necessary parts. This minimizes the amount of manipulation required, resulting in faster rendering times. Other frameworks may not leverage a Virtual DOM, leading to potential performance bottlenecks, especially in large applications.
3. JSX Syntax
React uses JSX (JavaScript XML), a syntax extension that allows developers to write HTML elements and components in a way that resembles XML or HTML. This makes the code more readable and expressive. Some frameworks, on the other hand, use templating languages or rely on pure JavaScript for rendering, which can be less intuitive and more verbose.
// React JSX example
const element = <h1>Hello, React!</h1>;
4. One-Way Data Binding
React follows a one-way data binding paradigm, meaning the flow of data is unidirectional. Changes in the parent components trigger updates in child components, but the reverse is not true. This simplicity makes it easier to understand the flow of data in an application. Other frameworks may implement two-way data binding, which can lead to unexpected behavior and make it harder to trace the source of changes.
5. React Native
React extends its influence beyond the web with React Native, a framework for building mobile applications using JavaScript and React. This allows developers to use their React skills to create cross-platform mobile apps. While some frameworks have attempted to offer similar capabilities, React Native’s popularity and community support make it a compelling choice for mobile development.
6. Unidirectional Data Flow
React enforces a unidirectional data flow, which contributes to the predictability of state changes. Data flows in a single direction, making it easier to understand and debug how changes propagate through the application. Other frameworks might adopt different data flow patterns, such as bidirectional or circular, which can lead to challenges in understanding and maintaining code.
7. React Hooks
Introduced in React 16.8, hooks revolutionized state management and side effects in functional components. Hooks, such as useState
and useEffect
, allow developers to use state and lifecycle features in functional components, eliminating the need for class components in many cases. This functional approach provides cleaner and more concise code. While some other frameworks have adopted similar concepts, React Hooks remain a powerful and widely adopted feature.
// Example of React Hooks
import React, { useState, useEffect } from 'react';
function ExampleComponent() {
const [count, setCount] = useState(0);
useEffect(() => {
document.title = `Count: ${count}`;
}, [count]);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
}
8. Large and Active Community
React boasts a large and active community of developers, contributing to its ecosystem with a wealth of libraries, tools, and resources. This community support is crucial for problem-solving, knowledge sharing, and the overall growth of the framework. While other frameworks may have substantial communities, React’s popularity and widespread adoption make it a standout choice for developers seeking a well-supported ecosystem.
9. Declarative Approach
React takes a declarative approach to building user interfaces, where developers describe how the UI should look based on the current state. This is in contrast to an imperative approach, where developers would explicitly specify each step to achieve a desired state. The declarative nature of React makes the code more expressive and less error-prone.
10. Integration with Redux
While not exclusive to React, Redux has become synonymous with React state management. React’s architecture allows seamless integration with Redux, a predictable state container for JavaScript applications. This integration provides a centralized and predictable state management solution, making it easier to handle the state in larger applications. Other frameworks may have their own state management solutions, but Redux’s popularity and compatibility with React give it a unique edge.
In conclusion, React distinguishes itself from other JavaScript frameworks through its component-based architecture, virtual DOM, JSX syntax, one-way data binding, React Native for mobile development, unidirectional data flow, powerful hooks, a vibrant community, a declarative approach, and seamless integration with Redux. Understanding these unique qualities empowers developers to make informed decisions when choosing a framework that aligns with their project requirements and coding preferences.