How to create a responsive form layout with Bootstrap
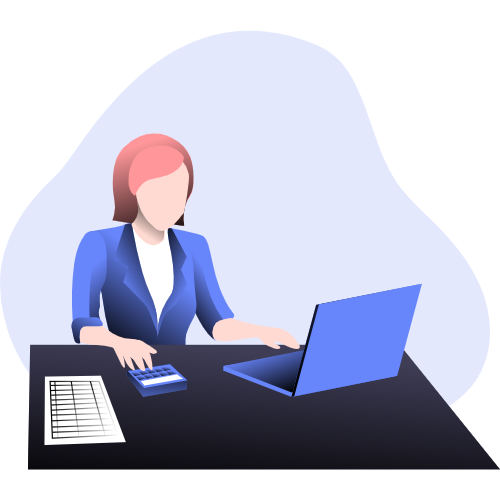
Creating a responsive form is a critical aspect of web development. Forms are used for a variety of purposes, such as collecting user data, processing orders, and enabling communication. Bootstrap, a popular front-end framework, provides a range of tools and components that make it easy to build responsive and visually appealing forms. In this comprehensive guide, we’ll walk you through the process of creating a responsive form layout with Bootstrap, ensuring that your forms adapt seamlessly to different screen sizes and devices.
The Importance of Responsive Forms
Before we dive into the technical details, let’s understand why responsive forms are crucial for web design:
Cross-Device Compatibility: With users accessing websites on various devices, responsive forms ensure that your forms are accessible and usable on smartphones, tablets, and desktops.
Improved User Experience: Responsive design enhances the user experience by providing a consistent and user-friendly interface regardless of the screen size.
Mobile Optimization: As mobile usage continues to grow, responsive forms are essential for capturing user data and facilitating actions on small screens.
Now, let’s explore how to implement a responsive form layout with Bootstrap.
Setting Up Bootstrap
To get started with Bootstrap for your responsive forms, you need to integrate Bootstrap into your project. You can do this by downloading the Bootstrap CSS and JavaScript files and adding them to your project, or you can use the Bootstrap CDN for quicker integration.
Once Bootstrap is set up, you can begin crafting your responsive form.
Building the Form Structure
The HTML structure for a responsive form with Bootstrap typically includes a form
element, form-group
classes for each input field, and responsive columns to control the layout. Here’s a basic example:
<form>
<div class="row">
<div class="col-md-6">
<div class="form-group">
<label for="firstName">First Name</label>
<input type="text" class="form-control" id="firstName" placeholder="Enter your first name">
</div>
</div>
<div class="col-md-6">
<div class="form-group">
<label for="lastName">Last Name</label>
<input type="text" class="form-control" id="lastName" placeholder="Enter your last name">
</div>
</div>
</div>
<div class="form-group">
<label for="email">Email Address</label>
<input type="email" class="form-control" id="email" placeholder="Enter your email">
</div>
<div class="form-group">
<label for="message">Message</label>
<textarea class="form-control" id="message" rows="4" placeholder="Type your message here"></textarea>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
In this structure:
- We use the Bootstrap grid system with
row
andcol-md-6
classes to create a two-column layout for the first and last name fields. Adjust the number of columns and their sizes as needed for your form. - Each input field is enclosed in a
form-group
div, which helps with styling and spacing. Labels are associated with input fields using thefor
attribute. - The form includes text inputs, an email input, and a textarea for the message. Customize these fields based on your form’s requirements.
- A submit button with the class
btn btn-primary
is included to submit the form.
Making the Form Responsive
Bootstrap’s grid system ensures that the form is responsive by default. However, you can further enhance responsiveness by using Bootstrap classes for different screen sizes. Here are some key classes for responsiveness:
col-xs-
: Extra small screens (phones).col-sm-
: Small screens (tablets).col-md-
: Medium screens (laptops).col-lg-
: Large screens (desktops).
For example, if you want a certain input field to span the entire width on small screens but use half the width on medium screens and larger, you can use the following classes:
<div class="col-12 col-md-6">
<!-- This field takes up the entire width on small screens and half on medium screens and larger -->
</div>
Additionally, Bootstrap offers responsive utility classes that allow you to hide or show elements on specific screen sizes. For example, you can hide a form field on small screens like this:
<div class="d-none d-sm-block">
<!-- This field is hidden on small screens but visible on screens of at least small size (tablets and larger) -->
</div>
Styling and Customization
Bootstrap provides extensive CSS classes for styling form elements. You can customize the form’s appearance by applying these classes or by writing your own CSS rules. For instance, you can change the form’s background color, text color, or font size to match your website’s design.
/* Custom styles for the form */
form {
background-color: #f8f9fa;
color: #333;
font-size: 16px;
}
/* Custom styles for
the submit button */
.btn-primary {
background-color: #007bff;
color: #fff;
border: none;
}
/* Add more custom styles as needed */
Customizing the form’s styles allows you to align it with your brand identity and design preferences.
Testing and Optimization
After creating your responsive form, it’s essential to thoroughly test it on various devices and screen sizes to ensure it adapts as expected. Pay attention to input field sizes, button placement, and overall usability.
Optimize your form layout based on user feedback and real-world testing. Consider factors like input validation, error handling, and mobile-friendly interactions when making improvements.
Conclusion
Creating a responsive form layout with Bootstrap is an essential skill for web developers. Bootstrap’s grid system and responsive classes make it relatively straightforward to build forms that work seamlessly across different devices and screen sizes. By following the steps outlined in this guide and customizing your form’s structure and styles to match your project’s requirements, you can create user-friendly and visually appealing forms that enhance the overall user experience on your website.