How to Handle Memory Leaks in a JavaScript Application
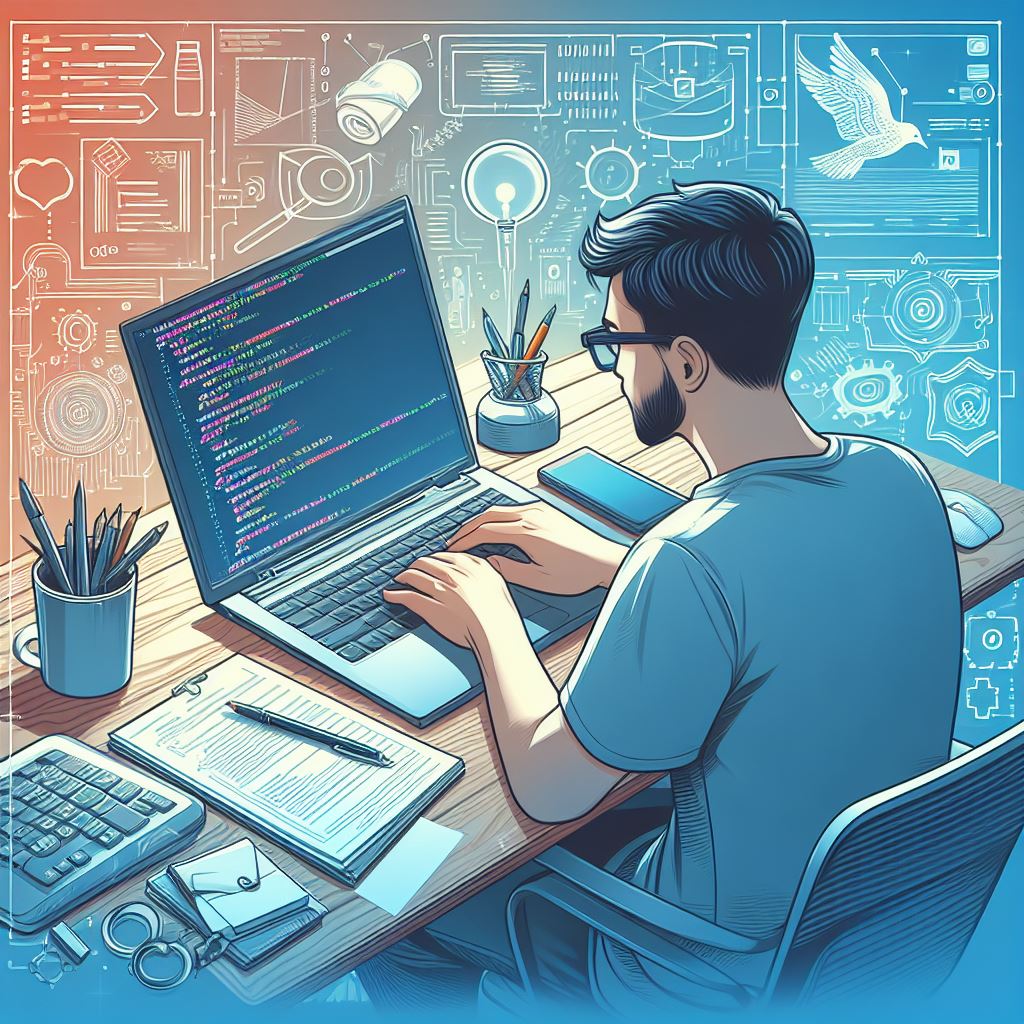
Memory management is crucial for every application, especially those running in resource-constrained environments like the web. JavaScript, being a high-level, garbage-collected language, abstracts away much of the complexity of memory management. However, memory leaks can still occur and seriously affect your application’s performance. In this article, we will explain memory leaks in detail, how they occur in JavaScript, and most importantly, how to prevent and handle them.
What is a Memory Leak?
In simple terms, a memory leak occurs when a program retains memory that is no longer needed, leading to inefficient use of memory and causing performance degradation over time. Memory leaks happen when objects or variables in an application are not properly released after they’re no longer in use, which causes them to consume memory indefinitely.
In JavaScript, memory is managed automatically by a garbage collector, which periodically reclaims unused memory. However, due to certain code patterns or design issues, some objects can remain “alive” even though they are no longer necessary. This leads to a memory leak because the garbage collector cannot free up this memory.
How JavaScript Memory Management Works JavaScript uses a garbage collection process to manage memory. It primarily relies on the concept of reachability—an object is considered reachable if it is accessible from the root, typically the global object (window in browsers).
When a piece of memory becomes unreachable (i.e., there are no references to it), the garbage collector identifies it as unused and reclaims it. However, there are cases where certain objects are mistakenly kept alive even though they are no longer needed.
Key Concepts Heap: Where all objects, arrays, and functions are stored in JavaScript. Stack: Stores primitive values and execution contexts. Garbage Collection: An automatic process that finds and reclaims memory that is no longer used. Common Causes of Memory Leaks in JavaScript Global Variables
Issue: Variables declared globally in JavaScript remain in memory throughout the life of the application. Unintended global variables, often caused by missing var, let, or const declarations, can accumulate and cause memory leaks. Solution: Always declare variables with proper scope (let, const) and avoid polluting the global namespace. Forgotten Timers/Intervals
Issue: If timers (such as setInterval or setTimeout) are set but never cleared, they can keep references to variables or functions, preventing them from being garbage collected. Solution: Always clear timers with clearInterval or clearTimeout once they are no longer needed. Event Listeners
Issue: When event listeners are attached to DOM elements but not removed after the element is no longer needed, memory leaks can occur. The event listener holds a reference to the element, keeping it alive in memory. Solution: Make sure to remove event listeners with removeEventListener when they are no longer needed or before removing the associated DOM element. Detached DOM Nodes
Issue: If DOM elements are removed from the document but still referenced elsewhere in the code (e.g., in JavaScript variables), they won’t be garbage collected, leading to memory leaks. Solution: Ensure that once an element is removed from the DOM, any reference to it is also deleted. Closures
Issue: Closures, a common feature in JavaScript, can inadvertently cause memory leaks when they capture variables that are no longer needed but keep them alive by retaining references. Solution: Be mindful of which variables are captured in closures, and avoid retaining references to large objects unnecessarily. Caching
Issue: Overzealous caching can lead to memory leaks if cached objects or data are no longer required but are never removed. Solution: Implement strategies like cache eviction (e.g., Least Recently Used (LRU) caching) to remove old data from memory. How to Detect Memory Leaks Detecting memory leaks can be challenging but is a necessary step for efficient memory management. Here are several techniques and tools to help you identify memory leaks:
- Chrome DevTools Chrome DevTools provides a built-in memory profiler that allows you to take memory snapshots and analyze memory usage over time. Here’s how you can use it:
Open DevTools: Right-click on your application, select Inspect, and then navigate to the Memory tab. Take Heap Snapshots: Take multiple heap snapshots at different times during your application’s runtime. Compare Snapshots: Analyze and compare the snapshots to see if any objects are growing in memory when they shouldn’t be.
- Performance Monitoring You can use browser APIs such as Performance.memory to monitor the memory consumption of your JavaScript application.
Example
javascript
Copy code
if (performance.memory) {
console.log(JS Heap Size: ${performance.memory.usedJSHeapSize}
);
}
- Profiling with Tools Node.js Memory Profiling: Tools like clinic.js and v8-profiler can be used to track memory leaks in Node.js applications. Third-Party Tools: Tools like Dynatrace and New Relic can help monitor memory consumption in production environments. Strategies to Prevent Memory Leaks Use the Right Scoping:
Use let and const instead of var to avoid accidental global variable declarations. Properly manage the scope of variables to minimize their lifetime and avoid keeping them around longer than needed. Clean Up Event Listeners
Ensure that event listeners are removed using removeEventListener when they are no longer needed, especially when removing DOM elements. Properly Manage Timers
Clear timers using clearTimeout and clearInterval once they’ve served their purpose. Avoid long-running or recursive timers unless absolutely necessary. Detach DOM Elements Correctly
When removing a DOM element, ensure that all references to it (e.g., in JavaScript variables or event listeners) are also cleared. Avoid Over-Caching
If your application uses caching, ensure that old or unused cache entries are evicted over time to avoid unnecessary memory consumption. Mind Your Closures
Avoid capturing unnecessary variables in closures. If closures are required, be aware of the variables they retain and the potential for leaks. Example of Memory Leak and Solution Let’s consider an example of a memory leak caused by an event listener:
javascript Copy code // Memory leak example const button = document.getElementById(‘myButton’); button.addEventListener(‘click’, () => { console.log(‘Button clicked!'); });
// Assume this element gets removed later in the code document.body.removeChild(button); In this case, the event listener still references the button element even after it has been removed from the DOM, causing a memory leak.
Solution: Before removing the DOM element, make sure to remove the event listener:
javascript Copy code // Prevent memory leak button.removeEventListener(‘click’, () => { console.log(‘Button clicked!'); });
document.body.removeChild(button); By removing the event listener before detaching the element, we ensure that no lingering references keep the element alive in memory.
Advanced Techniques for Memory Leak Prevention Weak References:
JavaScript provides a WeakMap and WeakSet to hold weak references to objects. Weak references allow objects to be garbage collected if they are no longer used elsewhere in the program. MutationObserver:
When manipulating the DOM, use a MutationObserver to detect when elements are removed and automatically clean up associated resources. Weak Event Listeners (Proposed):
New proposals are emerging in JavaScript to support weak references in event listeners, which would automatically clean up event listeners when the element is removed. Conclusion Memory leaks in JavaScript can be challenging to identify and fix, but understanding how they occur and the patterns that lead to them can significantly improve your ability to manage memory in your applications. By using tools like Chrome DevTools, implementing best practices such as removing event listeners and clearing timers, and monitoring memory usage, you can mitigate the impact of memory leaks on your application’s performance.
Proper memory management ensures that your application remains responsive and efficient over time. Always strive to write clean, maintainable code that minimizes the potential for memory leaks and other performance bottlenecks.
What is AI and how can I integrate it into my applications
How to resolve CORS errors in a web application
How to choose between AWS, Azure, and Google Cloud for my application
What Are the Top Programming Languages to Learn in 2024
How to Prepare for Technical Interviews at FAANG Companies