How to implement a card flip animation with Bootstrap
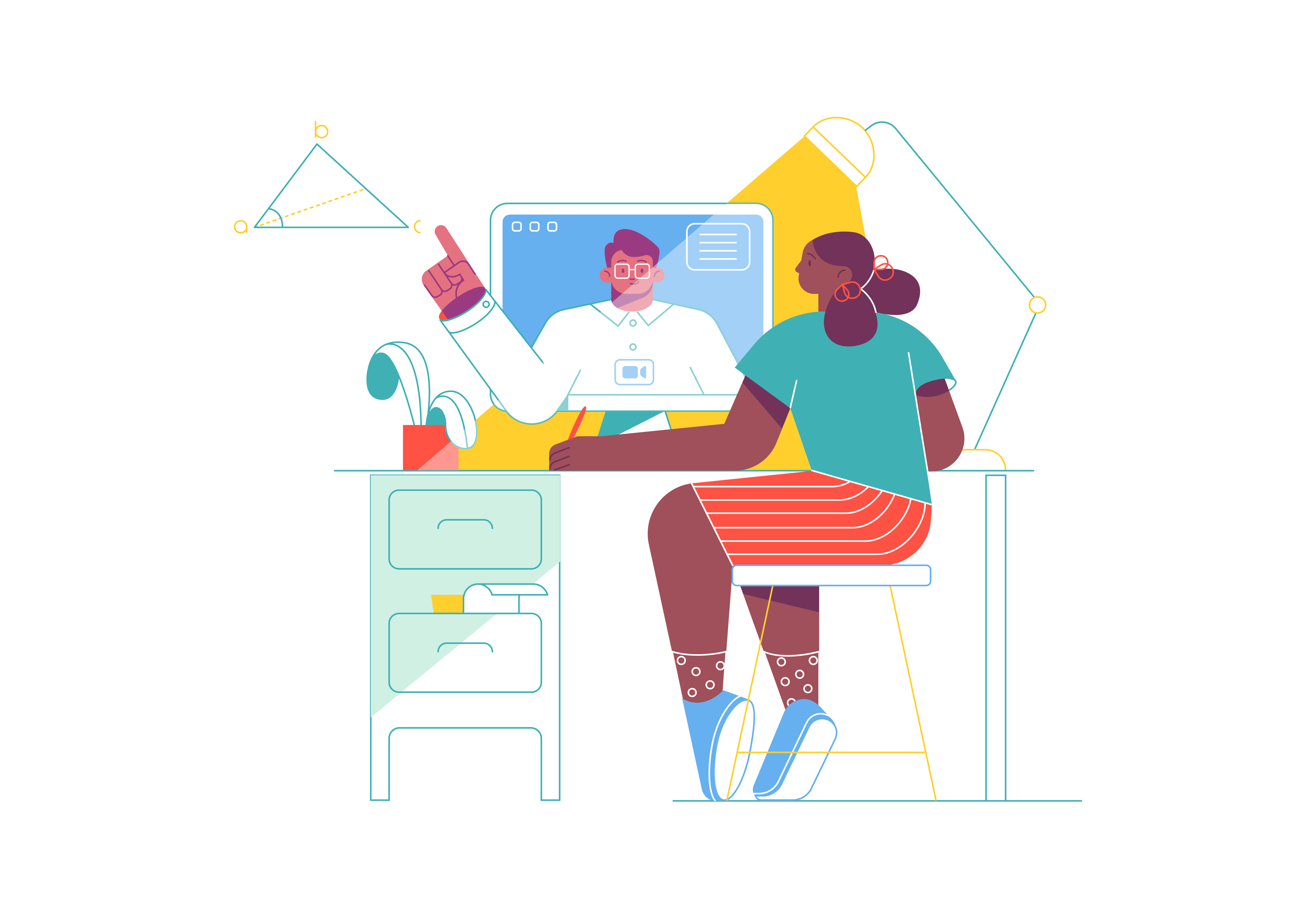
Card flip animations are a visually appealing way to display information on your website. They can be used for various purposes, from showcasing product details to creating engaging user interfaces. Bootstrap, a popular front-end framework, provides tools to implement card flip animations seamlessly. In this comprehensive guide, we’ll walk you through the process of creating card flip animations with Bootstrap, enhancing your website’s interactivity and user experience.
Understanding the Importance of Card Flip Animations
Before we dive into the technical details, let’s explore why card flip animations are essential for web design:
Engagement: Card flip animations capture users' attention, making your content more engaging and memorable.
Information Presentation: They allow you to present two sides of content, ideal for displaying product images and descriptions, or revealing additional information.
User Interaction: Card flips create an interactive element, encouraging users to explore and interact with your website.
Now, let’s delve into the implementation of card flip animations with Bootstrap.
Setting Up Bootstrap
To create card flip animations with Bootstrap, you’ll need to integrate Bootstrap into your project. This can be achieved by downloading the necessary CSS and JavaScript files and adding them to your project, or by using the Bootstrap CDN for a quicker setup.
Once Bootstrap is set up, you can start building your card flip animations.
Building the HTML Structure
Creating card flip animations with Bootstrap requires a specific HTML structure. You’ll need two elements: a container and the card itself. Here’s a basic example:
<div class="card-container">
<div class="card">
<div class="card-front">
<!-- Front content goes here -->
</div>
<div class="card-back">
<!-- Back content goes here -->
</div>
</div>
</div>
In this structure:
- The
.card-container
acts as a wrapper for the card to control its dimensions and positioning. - The
.card
element represents the card that will flip. - Inside the
.card
, you have a.card-front
and.card-back
div for the front and back content, respectively.
You can customize this structure to fit your specific design and content requirements.
Styling the Card
Bootstrap provides a set of CSS classes to style cards. You can use these classes as a starting point and customize them further to achieve the desired look for your card flip animation.
<div class="card-container">
<div class="card">
<div class="card-front">
<img src="front-image.jpg" alt="Front Image">
<h3>Front Title</h3>
<p>Front content goes here.</p>
</div>
<div class="card-back">
<img src="back-image.jpg" alt="Back Image">
<h3>Back Title</h3>
<p>Back content goes here.</p>
</div>
</div>
</div>
/* Custom styles for the card and its front/back faces */
.card {
width: 300px;
height: 400px;
perspective: 1000px; /* Depth of the 3D effect */
}
.card-front,
.card-back {
width: 100%;
height: 100%;
position: absolute;
backface-visibility: hidden; /* Hides the backface when not flipped */
transition: transform 0.5s; /* Animation duration and type */
}
.card-front {
transform: rotateY(0deg); /* Front face starts flat */
}
.card-back {
transform: rotateY(180deg); /* Back face is initially rotated 180 degrees */
}
In this example, we’ve applied some custom styles to the card container, front, and back faces. You can adjust the dimensions, perspective, and transition properties to achieve the desired visual effect.
Implementing the Flip Animation
To create the card flip animation, you’ll need to use CSS transitions and JavaScript to toggle the card’s state. Bootstrap provides the rotateY
class for this purpose.
Here’s an example of how you can implement the flip animation using Bootstrap classes and a bit of JavaScript:
<div class="card-container">
<div class="card">
<div class="card-front">
<img src="front-image.jpg" alt="Front Image">
<h3>Front Title</h3>
<p>Front content goes here.</p>
</div>
<div class="card-back">
<img src="back-image.jpg" alt="Back Image">
<h3>Back Title</h3>
<p>Back content goes here.</p>
</div>
</div>
</div>
<button id="flipButton" class="btn btn-primary">Flip Card</button>
// JavaScript to toggle the card flip
document.getElementById('flipButton').addEventListener('click', function () {
const card = document.querySelector('.card');
card.classList.toggle('rotateY');
});
/* CSS to apply the flip animation */
.rotateY {
transform: rotateY(180deg);
}
In this example:
- We’ve added a button (
#flipButton
) that users can click to trigger the flip animation. - The JavaScript event listener toggles the
rotateY
class on the card, which triggers the flip animation.
Testing and Optimization
After implementing the card flip animation, it’s essential to thoroughly test it on various devices and screen sizes. Ensure that the animation is smooth, and the content remains readable and accessible.
Optimize your card flip animation based on user feedback and real-world testing. Consider factors like animation speed, visual aesthetics, and overall user experience when making adjustments.
Conclusion
Bootstrap provides a straightforward way to implement captivating card flip animations on your website. By following the steps outlined in this guide and customizing the card’s structure, styles, and animations, you can create engaging user interfaces and display information in an interactive and visually appealing manner. Card flip animations not only enhance user engagement but also contribute to a more memorable and enjoyable browsing experience.