How to use Bootstrap's tooltip and popover components
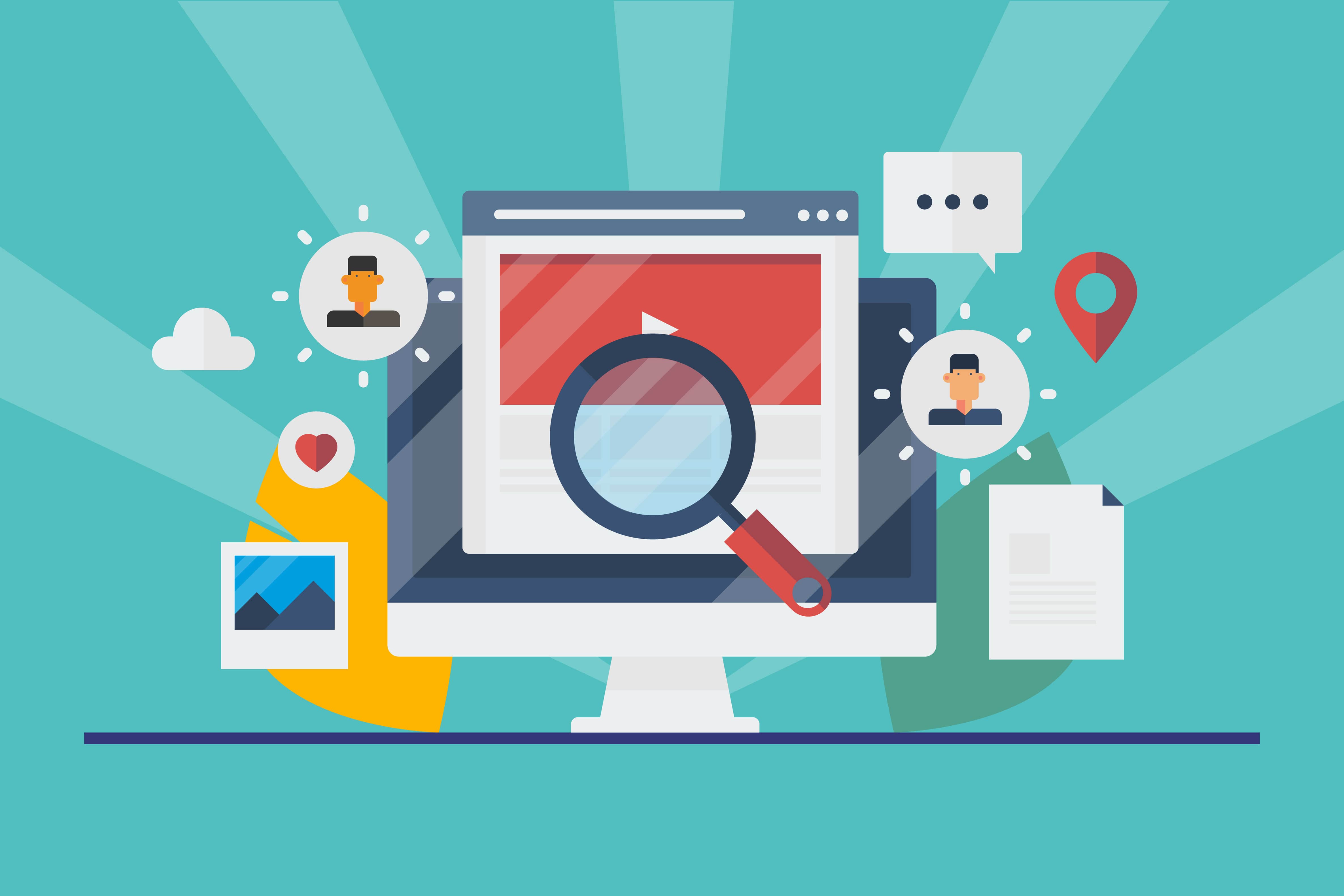
Tooltips and popovers are essential elements in web design, offering a convenient way to display additional information or context to users when they interact with specific elements on a webpage. Bootstrap, a widely-used front-end framework, provides built-in components to create tooltips and popovers seamlessly. In this comprehensive guide, we’ll explore how to effectively employ Bootstrap’s tooltip and popover components, enhancing your website’s user experience and interactivity.
Understanding the Role of Tooltips and Popovers
Before we dive into the technical aspects, let’s clarify the significance of tooltips and popovers in web design;
Tooltips
- Informational Hints: Tooltips are small, contextual messages that provide brief information when users hover over or click on an element like buttons, icons, or links.
- Enhanced Usability: They improve the user experience by offering explanations or clarifications for elements, helping users understand their functionality.
- Reduced Cognitive Load: Tooltips eliminate the need for users to guess or explore elements' purposes, reducing cognitive load and frustration.
Popovers
- Interactive Context: Popovers are similar to tooltips but often contain more detailed or interactive content, such as forms, buttons, or images.
- User Engagement: They engage users by presenting dynamic information or actions, making them ideal for forms, clickable elements, or image galleries.
- Enhanced User Assistance: Popovers provide comprehensive information or actions in a visually appealing way, aiding users in their tasks.
Now, let’s explore how to implement Bootstrap’s tooltip and popover components effectively.
Setting Up Bootstrap
To utilize Bootstrap’s tooltip and popover components, you’ll need to integrate Bootstrap into your project. You can either download the necessary CSS and JavaScript files and include them in your project, or you can utilize the Bootstrap CDN for faster integration.
Once Bootstrap is set up, you can begin incorporating tooltips and popovers into your website.
Implementing Tooltips
Basic Tooltip
You can add a simple tooltip to an element by adding the data-bs-toggle
and data-bs-tooltip
attributes.
<button type="button" class="btn btn-primary" data-bs-toggle="tooltip" data-bs-placement="top" title="Click me!">Hover Me</button>
In this example, we’ve created a button with a tooltip that appears when the user hovers over it. The data-bs-placement
attribute specifies the tooltip’s position (top, right, bottom, or left).
Customizing Tooltips
You can further customize tooltips by using Bootstrap classes or adding your own CSS.
<button type="button" class="btn btn-primary" data-bs-toggle="tooltip" data-bs-placement="right" title="Custom Tooltip">
Hover Me
</button>
/* Custom tooltip style */
.tooltip {
background-color: #007bff;
color: #fff;
}
Enabling Tooltips via JavaScript
If you prefer JavaScript to activate tooltips, you can use the following code:
var tooltipTriggerList = [].slice.call(document.querySelectorAll('[data-bs-toggle="tooltip"]'))
var tooltipList = tooltipTriggerList.map(function (tooltipTriggerEl) {
return new bootstrap.Tooltip(tooltipTriggerEl)
})
This JavaScript code initializes tooltips for elements with the data-bs-toggle="tooltip"
attribute.
Implementing Popovers
Basic Popover
Creating a basic popover is similar to tooltips. Use the data-bs-toggle
and data-bs-content
attributes.
<button type="button" class="btn btn-primary" data-bs-toggle="popover" title="Popover Title" data-bs-content="Popover Content">Click Me</button>
In this example, a button triggers a popover with a title and content when clicked.
Customizing Popovers
Customize popovers by specifying the placement, trigger, and adding your own CSS.
<button type="button" class="btn btn-primary" data-bs-toggle="popover" data-bs-placement="bottom" data-bs-trigger="focus" title="Custom Popover" data-bs-content="Custom Content">Click Me</button>
/* Custom popover style */
.popover {
background-color: #ffc107;
color: #333;
}
Enabling Popovers via JavaScript
If you prefer JavaScript for popover activation, use this code:
var popoverTriggerList = [].slice.call(document.querySelectorAll('[data-bs-toggle="popover"]'))
var popoverList = popoverTriggerList.map(function (popoverTriggerEl) {
return new bootstrap.Popover(popoverTriggerEl)
})
This JavaScript code initializes popovers for elements with the data-bs-toggle="popover"
attribute.
Advanced Techniques
Dynamically Generated Content
You can dynamically generate popover content using JavaScript. This is useful when you need to load content from an API or database.
var myPopover = new bootstrap.Popover(document.querySelector('#myButton'), {
content: function () {
return fetch('https://example.com/api/data')
.then(response => response.text())
}
})
Event Handling
Use Bootstrap’s event handling to trigger actions when a tooltip or popover is shown or hidden.
var myTooltip = new bootstrap.Tooltip(document.querySelector('#myTooltip'))
myTooltip.show()
myTooltip.hide()
Testing and Optimization
After implementing tooltips and popovers, thoroughly test them on various devices and screen sizes to ensure they work as expected. Verify that the content appears correctly and that the user experience is smooth.
Optimize your tooltips and popovers based on user feedback and real-world testing. Consider factors like responsiveness, accessibility, and the clarity of content when making adjustments.
Conclusion
Bootstrap’s tooltip and popover components are powerful tools for enhancing user experience and interactivity on your website. By following the steps outlined in this guide and customizing tooltips and popovers to meet your specific needs, you can provide users with informative and engaging elements that improve usability and engagement.