Integrating Bootstrap with React: A Complete Guide
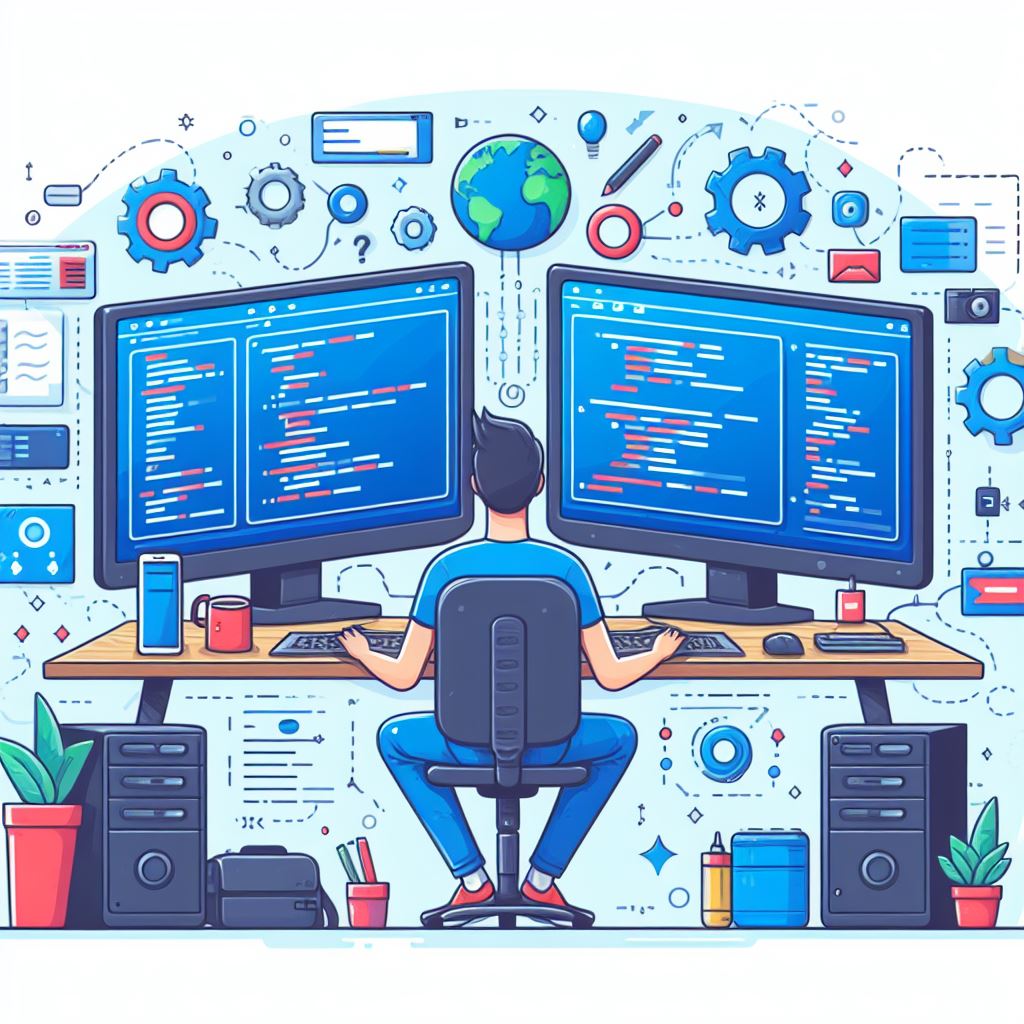
In the world of web development, creating visually appealing and responsive interfaces efficiently is a priority for developers. Bootstrap, one of the most popular CSS frameworks, provides a comprehensive collection of pre-designed components and utilities that streamline the development process. React, on the other hand, has revolutionized frontend development with its component-based architecture and virtual DOM implementation.
Combining these two powerful technologies can significantly enhance your development workflow, allowing you to leverage Bootstrap’s extensive UI components while maintaining the benefits of React’s component model. This guide will walk you through various approaches to integrating Bootstrap with React, from using plain CSS imports to specialized libraries like React Bootstrap and Reactstrap.
By the end of this comprehensive guide, you’ll have a thorough understanding of how to effectively integrate Bootstrap with React, which approach best suits your project needs, and how to implement common patterns and solutions to potential challenges.
Understanding Bootstrap and React
Bootstrap: The CSS Framework
Bootstrap is a popular open-source CSS framework developed by Twitter that provides a collection of CSS and JavaScript components for creating responsive, mobile-first websites. Its key features include:
- Grid System: A flexible 12-column layout system that adapts to different screen sizes
- Pre-styled Components: Buttons, forms, cards, navbars, and more
- Utility Classes: Helper classes for spacing, colors, flexbox, and other common CSS needs
- JavaScript Components: Interactive elements like modals, dropdowns, and carousels
Bootstrap 5, the latest major version, has removed its jQuery dependency, making it more compatible with modern JavaScript frameworks like React.
React: The JavaScript Library
React is a JavaScript library for building user interfaces, particularly single-page applications. It focuses on:
- Component-Based Architecture: UI is broken down into reusable, encapsulated components
- Virtual DOM: Efficiently updates the actual DOM by only rendering changed components
- Declarative Syntax: Describe what your UI should look like, and React handles the updates
- Unidirectional Data Flow: Data flows one way, making applications easier to reason about
Why Integrate Bootstrap with React?
Combining Bootstrap with React brings several advantages:
- Accelerated Development: Bootstrap’s pre-built components save time on styling and responsive design
- Consistent UI: Bootstrap ensures a uniform look across your application
- Responsive Design: Built-in responsive capabilities adapt to different screen sizes
- Component-Based Approach: Both technologies use a component-based methodology
- Large Community Support: Both have extensive communities, documentation, and resources
Integration Approaches
There are several ways to integrate Bootstrap with React, each with its own advantages and considerations.
Using Bootstrap CSS with React
The simplest approach is to import Bootstrap’s CSS files directly into your React application.
Installation
npm install bootstrap
Usage
In your index.js
or App.js
:
import 'bootstrap/dist/css/bootstrap.min.css';
For JavaScript components that require it:
import 'bootstrap/dist/js/bootstrap.bundle.min.js';
Advantages
- Simple setup
- No additional libraries needed
- Full access to all Bootstrap features
Disadvantages
- No React component integration
- Manual DOM manipulation required for interactive components
- Potential conflicts with React’s lifecycle
React Bootstrap
React Bootstrap is a complete rebuild of Bootstrap components using React. It replaces Bootstrap’s JavaScript with React implementations.
Installation
npm install react-bootstrap bootstrap
Usage
Import Bootstrap CSS:
import 'bootstrap/dist/css/bootstrap.min.css';
Import and use components:
import { Button, Card, Container } from 'react-bootstrap';
function MyComponent() {
return (
<Container>
<Card>
<Card.Body>
<Card.Title>Card Title</Card.Title>
<Card.Text>Some text here</Card.Text>
<Button variant="primary">Click Me</Button>
</Card.Body>
</Card>
</Container>
);
}
Advantages
- True React components
- Props-based configuration
- Compatible with React’s component lifecycle
- TypeScript support
Disadvantages
- Slightly different API from vanilla Bootstrap
- Potential learning curve for Bootstrap purists
Reactstrap
Reactstrap is another library that provides React components for Bootstrap 4 and 5.
Installation
npm install reactstrap bootstrap
Usage
Import Bootstrap CSS:
import 'bootstrap/dist/css/bootstrap.min.css';
Import and use components:
import { Button, Card, CardBody, CardTitle, CardText, Container } from 'reactstrap';
function MyComponent() {
return (
<Container>
<Card>
<CardBody>
<CardTitle tag="h5">Card Title</CardTitle>
<CardText>Some text here</CardText>
<Button color="primary">Click Me</Button>
</CardBody>
</Card>
</Container>
);
}
Advantages
- API closely mirroring Bootstrap
- No jQuery dependency
- Extensive component support
Disadvantages
- Different component structure than React Bootstrap
- Less TypeScript support historically
Bootstrap + React Hooks
Using React hooks with Bootstrap can provide more control over Bootstrap’s JavaScript functionality.
Usage Example
import { useEffect, useRef } from 'react';
import 'bootstrap/dist/css/bootstrap.min.css';
import { Modal } from 'bootstrap';
function ModalComponent() {
const modalRef = useRef(null);
let modalInstance = null;
useEffect(() => {
// Initialize the modal
modalInstance = new Modal(modalRef.current);
// Cleanup function
return () => {
if (modalInstance) {
modalInstance.dispose();
}
};
}, []);
const openModal = () => {
modalInstance.show();
};
return (
<>
<button className="btn btn-primary" onClick={openModal}>
Open Modal
</button>
<div className="modal fade" ref={modalRef} tabIndex="-1">
<div className="modal-dialog">
<div className="modal-content">
<div className="modal-header">
<h5 className="modal-title">Modal title</h5>
<button type="button" className="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div className="modal-body">
<p>Modal body text goes here.</p>
</div>
</div>
</div>
</div>
</>
);
}
Advantages
- Fine-grained control over Bootstrap’s JavaScript behavior
- No additional library dependencies
- Pure React implementation
Disadvantages
- More manual work
- Need to handle initialization and cleanup
- More complex implementation
Setting Up Your Development Environment
Let’s set up a new React project with Bootstrap integration.
Creating a New React Project
npx create-react-app my-bootstrap-app
cd my-bootstrap-app
Installing Bootstrap
For a React Bootstrap approach:
npm install react-bootstrap bootstrap
Project Structure
A typical structure for a React project with Bootstrap might look like:
my-bootstrap-app/
├── node_modules/
├── public/
├── src/
│ ├── components/
│ │ ├── Button.js
│ │ ├── Card.js
│ │ ├── Navbar.js
│ │ └── ...
│ ├── pages/
│ │ ├── Home.js
│ │ ├── About.js
│ │ └── ...
│ ├── App.js
│ ├── index.js
│ └── index.css
├── package.json
└── README.md
Basic Configuration
In src/index.js
or src/App.js
, import Bootstrap CSS:
import 'bootstrap/dist/css/bootstrap.min.css';
For custom theming, consider creating a src/custom.scss
file:
// Custom variable overrides
$primary: #007bff;
$secondary: #6c757d;
// Import Bootstrap
@import "~bootstrap/scss/bootstrap";
// Custom styles
.custom-element {
background-color: lighten($primary, 10%);
}
Then import this file instead of the default CSS:
import './custom.scss';
Note: You’ll need to install sass
for this approach:
npm install sass
Basic Integration Tutorial
Let’s create a simple application with Bootstrap components to demonstrate the integration.
Step 1: Set Up the Project
Follow the steps in the previous section to create a new React project and install Bootstrap.
Step 2: Create a Basic Layout
Create a file src/components/Layout.js
:
import React from 'react';
import { Container, Row, Col, Navbar, Nav } from 'react-bootstrap';
function Layout({ children }) {
return (
<>
<Navbar bg="dark" variant="dark" expand="lg">
<Container>
<Navbar.Brand href="#home">React-Bootstrap App</Navbar.Brand>
<Navbar.Toggle aria-controls="basic-navbar-nav" />
<Navbar.Collapse id="basic-navbar-nav">
<Nav className="me-auto">
<Nav.Link href="#home">Home</Nav.Link>
<Nav.Link href="#features">Features</Nav.Link>
<Nav.Link href="#pricing">Pricing</Nav.Link>
</Nav>
</Navbar.Collapse>
</Container>
</Navbar>
<Container className="mt-4">
<Row>
<Col>
{children}
</Col>
</Row>
</Container>
<footer className="bg-dark text-white text-center py-3 mt-5">
<Container>
<p className="mb-0">© 2025 React-Bootstrap Example</p>
</Container>
</footer>
</>
);
}
export default Layout;
Step 3: Create a Home Page Component
Create a file src/pages/Home.js
:
import React from 'react';
import { Card, Button, Alert, Carousel } from 'react-bootstrap';
function Home() {
return (
<>
<h1>Welcome to React Bootstrap</h1>
<Alert variant="info" className="my-4">
This is a simple example of using React Bootstrap components!
</Alert>
<Carousel className="mb-4">
<Carousel.Item>
<img
className="d-block w-100"
src="https://via.placeholder.com/800x400?text=First+slide"
alt="First slide"
/>
<Carousel.Caption>
<h3>First slide</h3>
<p>Some description text here.</p>
</Carousel.Caption>
</Carousel.Item>
<Carousel.Item>
<img
className="d-block w-100"
src="https://via.placeholder.com/800x400?text=Second+slide"
alt="Second slide"
/>
<Carousel.Caption>
<h3>Second slide</h3>
<p>More description text here.</p>
</Carousel.Caption>
</Carousel.Item>
</Carousel>
<div className="d-flex flex-wrap">
{[1, 2, 3].map((item) => (
<Card key={item} style={{ width: '18rem' }} className="m-2">
<Card.Img variant="top" src={`https://via.placeholder.com/300x200?text=Card+${item}`} />
<Card.Body>
<Card.Title>Card {item}</Card.Title>
<Card.Text>
Some quick example text to build on the card title and make up the bulk of
the card's content.
</Card.Text>
<Button variant="primary">Go somewhere</Button>
</Card.Body>
</Card>
))}
</div>
</>
);
}
export default Home;
Step 4: Update App.js
Update your src/App.js
:
import React from 'react';
import 'bootstrap/dist/css/bootstrap.min.css';
import Layout from './components/Layout';
import Home from './pages/Home';
function App() {
return (
<Layout>
<Home />
</Layout>
);
}
export default App;
Step 5: Run Your Application
npm start
You should now have a working React application with Bootstrap components!
Advanced Component Customization
Bootstrap components can be customized to match your application’s design requirements.
Customizing with Props
React Bootstrap and Reactstrap components accept props for customization:
<Button
variant="outline-success"
size="lg"
className="custom-button"
onClick={handleClick}
>
Custom Button
</Button>
Creating Wrapper Components
For consistent customization across your application, create wrapper components:
// src/components/CustomButton.js
import React from 'react';
import { Button } from 'react-bootstrap';
function CustomButton({ children, ...props }) {
return (
<Button
{...props}
className={`rounded-pill shadow-sm ${props.className || ''}`}
>
{children}
</Button>
);
}
export default CustomButton;
Using SASS for Deep Customization
For deeper customization, use SASS variables:
// src/custom.scss
$theme-colors: (
"primary": #0074d9,
"secondary": #6c757d,
"success": #28a745,
"info": #17a2b8,
"warning": #ffc107,
"danger": #dc3545,
"light": #f8f9fa,
"dark": #343a40
);
$border-radius: 0.5rem;
$btn-border-radius: 1.5rem;
@import "~bootstrap/scss/bootstrap";
Extending Bootstrap Classes
Use utilities from Bootstrap alongside custom CSS:
<div className="bg-light p-4 mb-3 border rounded shadow-sm custom-container">
<h3 className="text-primary">Custom Container</h3>
<p className="lead">This container uses Bootstrap utilities with custom styles.</p>
</div>
Responsive Layouts with Bootstrap in React
Bootstrap’s grid system is a powerful tool for creating responsive layouts in React applications.
Basic Grid Layout
import { Container, Row, Col } from 'react-bootstrap';
function ResponsiveLayout() {
return (
<Container>
<Row>
<Col xs={12} md={6} lg={4}>
<div className="p-3 border">Column 1</div>
</Col>
<Col xs={12} md={6} lg={4}>
<div className="p-3 border">Column 2</div>
</Col>
<Col xs={12} md={12} lg={4}>
<div className="p-3 border">Column 3</div>
</Col>
</Row>
</Container>
);
}
Auto-layout Columns
<Row>
<Col>Equal width</Col>
<Col>Equal width</Col>
<Col>Equal width</Col>
</Row>
Column Ordering
<Row>
<Col xs={{ order: 3 }}>First, but last on small screens</Col>
<Col xs={{ order: 1 }}>Third, but first on small screens</Col>
<Col xs={{ order: 2 }}>Second on all screen sizes</Col>
</Row>
Nesting Rows and Columns
<Row>
<Col md={8}>
<div className="p-3 border">Main Content</div>
</Col>
<Col md={4}>
<div className="p-3 border">Sidebar</div>
<Row className="mt-3">
<Col>
<div className="p-3 border">Nested 1</div>
</Col>
<Col>
<div className="p-3 border">Nested 2</div>
</Col>
</Row>
</Col>
</Row>
Responsive Utilities
Bootstrap provides utility classes for responsive visibility:
<div className="d-none d-md-block">
Visible only on medium and larger screens
</div>
<div className="d-block d-md-none">
Visible only on small screens
</div>
Theming and Styling
Customize the appearance of your Bootstrap-powered React application.
Creating a Custom Theme
- Create a custom SCSS file:
// src/theme.scss
// Variable overrides
$primary: #6200ea;
$secondary: #03dac6;
$success: #00c853;
$info: #2196f3;
$warning: #ffab00;
$danger: #d50000;
// Customize other variables
$body-bg: #f5f5f5;
$body-color: #333;
$border-radius: 0.5rem;
$font-family-base: 'Roboto', sans-serif;
// Import Bootstrap
@import "~bootstrap/scss/bootstrap";
// Additional custom styles
.card {
transition: transform 0.2s ease;
&:hover {
transform: translateY(-5px);
}
}
- Import the custom theme in your entry file:
import './theme.scss';
Using CSS Modules with Bootstrap
You can use CSS Modules alongside Bootstrap for component-specific styling:
// Button.module.css
.customButton {
text-transform: uppercase;
letter-spacing: 1px;
font-weight: bold;
}
// Button.js
import React from 'react';
import { Button as BootstrapButton } from 'react-bootstrap';
import styles from './Button.module.css';
function Button(props) {
return (
<BootstrapButton {...props} className={`${styles.customButton} ${props.className || ''}`}>
{props.children}
</BootstrapButton>
);
}
export default Button;
Theme Switching
Implement theme switching with React’s context API:
// ThemeContext.js
import React, { createContext, useState, useContext, useEffect } from 'react';
const ThemeContext = createContext();
export const ThemeProvider = ({ children }) => {
const [darkMode, setDarkMode] = useState(false);
useEffect(() => {
document.body.setAttribute('data-bs-theme', darkMode ? 'dark' : 'light');
}, [darkMode]);
return (
<ThemeContext.Provider value={{ darkMode, setDarkMode }}>
{children}
</ThemeContext.Provider>
);
};
export const useTheme = () => useContext(ThemeContext);
Then use it in your components:
import { useTheme } from './ThemeContext';
function ThemeSwitcher() {
const { darkMode, setDarkMode } = useTheme();
return (
<Button
variant={darkMode ? 'light' : 'dark'}
onClick={() => setDarkMode(!darkMode)}
>
Switch to {darkMode ? 'Light' : 'Dark'} Mode
</Button>
);
}
Performance Considerations
When integrating Bootstrap with React, consider these performance optimizations:
Code Splitting
Import only the components you need:
import Button from 'react-bootstrap/Button';
import Card from 'react-bootstrap/Card';
// Instead of: import { Button, Card } from 'react-bootstrap';
Using Bootstrap’s Utility API
For Bootstrap 5, you can use the utility API to include only necessary utilities:
@import "~bootstrap/scss/functions";
@import "~bootstrap/scss/variables";
@import "~bootstrap/scss/mixins";
// Include only the utilities you need
@import "~bootstrap/scss/utilities/api";
Lazy Loading Components
Use React’s lazy loading for routes or large components:
import React, { lazy, Suspense } from 'react';
const Dashboard = lazy(() => import('./pages/Dashboard'));
function App() {
return (
<Suspense fallback={<div className="spinner-border text-primary" role="status"></div>}>
<Dashboard />
</Suspense>
);
}
Avoiding Unnecessary Re-renders
Use React’s memo
or shouldComponentUpdate
to prevent unnecessary re-renders:
import React, { memo } from 'react';
import { Card } from 'react-bootstrap';
const ProductCard = memo(({ product }) => {
return (
<Card>
<Card.Img variant="top" src={product.image} />
<Card.Body>
<Card.Title>{product.name}</Card.Title>
<Card.Text>${product.price}</Card.Text>
</Card.Body>
</Card>
);
});
export default ProductCard;
Common Patterns and Best Practices
Composing Components
Create composite components by combining smaller ones:
import React from 'react';
import { Card, Button, Badge } from 'react-bootstrap';
function ProductCard({ product, onAddToCart }) {
return (
<Card className="h-100">
<Card.Img variant="top" src={product.image} />
<Card.Body className="d-flex flex-column">
<div className="d-flex justify-content-between align-items-center mb-2">
<Card.Title>{product.name}</Card.Title>
<Badge bg={product.inStock ? 'success' : 'danger'}>
{product.inStock ? 'In Stock' : 'Out of Stock'}
</Badge>
</div>
<Card.Text>{product.description}</Card.Text>
<div className="mt-auto">
<div className="d-flex justify-content-between align-items-center">
<span className="h5 mb-0">${product.price.toFixed(2)}</span>
<Button
variant="primary"
disabled={!product.inStock}
onClick={() => onAddToCart(product)}
>
Add to Cart
</Button>
</div>
</div>
</Card.Body>
</Card>
);
}
export default ProductCard;
Form Handling with Bootstrap
Implement form validation with React and Bootstrap:
import React, { useState } from 'react';
import { Form, Button, Alert } from 'react-bootstrap';
function ContactForm() {
const [validated, setValidated] = useState(false);
const [formData, setFormData] = useState({
name: '',
email: '',
message: ''
});
const [error, setError] = useState('');
const [success, setSuccess] = useState(false);
const handleChange = (e) => {
const { name, value } = e.target;
setFormData({
...formData,
[name]: value
});
};
const handleSubmit = (e) => {
e.preventDefault();
const form = e.currentTarget;
if (form.checkValidity() === false) {
e.stopPropagation();
setError('Please fill out all required fields correctly.');
} else {
// Form submission logic
console.log('Form submitted:', formData);
setSuccess(true);
setError('');
setFormData({ name: '', email: '', message: '' });
}
setValidated(true);
};
return (
<>
{error && <Alert variant="danger">{error}</Alert>}
{success && <Alert variant="success">Message sent successfully!</Alert>}
<Form noValidate validated={validated} onSubmit={handleSubmit}>
<Form.Group className="mb-3" controlId="formName">
<Form.Label>Name</Form.Label>
<Form.Control
required
type="text"
name="name"
value={formData.name}
onChange={handleChange}
/>
<Form.Control.Feedback type="invalid">
Please provide your name.
</Form.Control.Feedback>
</Form.Group>
<Form.Group className="mb-3" controlId="formEmail">
<Form.Label>Email</Form.Label>
<Form.Control
required
type="email"
name="email"
value={formData.email}
onChange={handleChange}
/>
<Form.Control.Feedback type="invalid">
Please provide a valid email.
</Form.Control.Feedback>
</Form.Group>
<Form.Group className="mb-3" controlId="formMessage">
<Form.Label>Message</Form.Label>
<Form.Control
required
as="textarea"
rows={3}
name="message"
value={formData.message}
onChange={handleChange}
/>
<Form.Control.Feedback type="invalid">
Please enter your message.
</Form.Control.Feedback>
</Form.Group>
<Button variant="primary" type="submit">
Submit
</Button>
</Form>
</>
);
}
export default ContactForm;
Creating a Modal Pattern
Implement a reusable modal component:
import React, { useState } from 'react';
import { Modal, Button } from 'react-bootstrap';
function ConfirmationModal({ show, onHide, onConfirm, title, children }) {
return (
<Modal show={show} onHide={onHide}>
<Modal.Header closeButton>
<Modal.Title>{title}</Modal.Title>
</Modal.Header>
<Modal.Body>{children}</Modal.Body>
<Modal.Footer>
<Button variant="secondary" onClick={onHide}>
Cancel
</Button>
<Button variant="primary" onClick={onConfirm}>
Confirm
</Button>
</Modal.Footer>
</Modal>
);
}
// Usage
function DeleteButton({ itemId, onDelete }) {
const [showModal, setShowModal] = useState(false);
const handleDelete = () => {
onDelete(itemId);
setShowModal(false);
};
return (
<>
<Button variant="danger" onClick={() => setShowModal(true)}>
Delete
</Button>
<ConfirmationModal
show={showModal}
onHide={() => setShowModal(false)}
onConfirm={handleDelete}
title="Confirm Deletion"
>
Are you sure you want to delete this item? This action cannot be undone.
</ConfirmationModal>
</>
);
}
Troubleshooting Common Issues
JavaScript Components Not Working
Problem: Bootstrap JavaScript components like dropdowns or modals aren’t functioning.
Solution: Ensure you’ve imported the Bootstrap JavaScript file:
import 'bootstrap/dist/js/bootstrap.bundle.min.js';
Or better, use React Bootstrap:
import { Dropdown } from 'react-bootstrap';
Style Conflicts
Problem: Bootstrap styles conflict with other CSS in your application.
Solution: Use CSS modules or more specific selectors:
// Apply Bootstrap only to a specific container
<div className="bootstrap-container">
{/* Bootstrap components here */}
</div>
// In your CSS
.bootstrap-container {
/* Reset any conflicting styles */
}
Responsive Issues
Problem: Layout doesn’t adapt correctly on different screen sizes.
Solution: Make sure you’re using the grid system correctly:
<Row>
<Col xs={12} sm={6} md={4}>
{/* This content will take different widths at different breakpoints */}
</Col>
</Row>
React Bootstrap Modal Focus Issues
Problem: Modal doesn’t properly manage focus.
Solution: Use the autoFocus
prop:
<Modal.Dialog>
<Modal.Header closeButton>
<Modal.Title>Modal title</Modal.Title>
</Modal.Header>
<Modal.Body>
<Form.Control autoFocus type="text" placeholder="Focus here first" />
</Modal.Body>
</Modal.Dialog>
Server-Side Rendering Issues
Problem: Bootstrap causes issues with server-side rendering.
Solution: Import Bootstrap only on the client side:
import React, { useEffect } from 'react';
function App() {
useEffect(() => {
import('bootstrap/dist/css/bootstrap.min.css');
}, []);
return (
// Your app content
);
}
Case Study: Building a Dashboard
Let’s create a comprehensive example of a dashboard using React and Bootstrap.
Dashboard Layout Component
import React from 'react';
import { Container, Row, Col, Nav } from 'react-bootstrap';
import { BellFill, GearFill, PersonFill } from 'react-bootstrap-icons';
function DashboardLayout({ children }) {
return (
<div className="dashboard">
{/* Top Navbar */}
<header className="navbar navbar-dark sticky-top bg-dark flex-md-nowrap p-0 shadow">
<a className="navbar-brand col-md-3 col-lg-2 me-0 px-3" href="#home">
Company Name
</a>
<button
className="navbar-toggler d-md-none collapsed"
type="button"
data-bs-toggle="collapse"
data-bs-target="#sidebarMenu"
>
<span className="navbar-toggler-icon"></span>
</button>
<input
className="form-control form-control-dark w-100"
type="text"
placeholder="Search"
aria-label="Search"
/>
<div className="navbar-nav">
<div className="nav-item text-nowrap">
<a className="nav-link px-3" href="#signout">
Sign out
</a>
</div>
</div>
</header>
<Container fluid>
<Row>
{/* Sidebar */}
<Col md={3} lg={2} className="d-md-block bg-light sidebar collapse" id="sidebarMenu">
<div className="position-sticky pt-3">
<Nav className="flex-column">
<Nav.Item>
<Nav.Link href="#dashboard" className="active">
Dashboard
</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#orders">Orders</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#products">Products</Nav.Link>
</Nav.Item>
<Nav.Item>
I’ll finish the article by continuing from where it was cut off, completing the dashboard example and providing the conclusion sections.
Case Study: Building a Dashboard (continued)
<Nav.Item>
<Nav.Link href="#customers">Customers</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#reports">Reports</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#integrations">Integrations</Nav.Link>
</Nav.Item>
</Nav>
<h6 className="sidebar-heading d-flex justify-content-between align-items-center px-3 mt-4 mb-1 text-muted">
<span>Saved reports</span>
<a className="link-secondary" href="#add-report" aria-label="Add a new report">
<span data-feather="plus-circle"></span>
</a>
</h6>
<Nav className="flex-column mb-2">
<Nav.Item>
<Nav.Link href="#current-month">Current month</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#last-quarter">Last quarter</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#social-engagement">Social engagement</Nav.Link>
</Nav.Item>
<Nav.Item>
<Nav.Link href="#year-end-sale">Year-end sale</Nav.Link>
</Nav.Item>
</Nav>
</div>
</Col>
{/* Main Content */}
<Col md={9} lg={10} className="ms-sm-auto px-md-4">
<div className="d-flex justify-content-between flex-wrap flex-md-nowrap align-items-center pt-3 pb-2 mb-3 border-bottom">
<h1 className="h2">Dashboard</h1>
<div className="btn-toolbar mb-2 mb-md-0">
<div className="btn-group me-2">
<button type="button" className="btn btn-sm btn-outline-secondary">
Share
</button>
<button type="button" className="btn btn-sm btn-outline-secondary">
Export
</button>
</div>
<button type="button" className="btn btn-sm btn-outline-secondary dropdown-toggle">
<span data-feather="calendar"></span>
This week
</button>
</div>
</div>
{/* Dashboard Content */}
{children}
</Col>
</Row>
</Container>
</div>
);
}
export default DashboardLayout;
Dashboard Stats Component
import React from 'react';
import { Row, Col, Card } from 'react-bootstrap';
import { ArrowUp, ArrowDown, GraphUp } from 'react-bootstrap-icons';
function DashboardStats() {
return (
<Row className="g-4 mb-4">
<Col md={6} xl={3}>
<Card className="h-100">
<Card.Body>
<div className="d-flex justify-content-between align-items-start">
<div>
<h6 className="text-muted">Total Revenue</h6>
<h3 className="mb-0">$84,686</h3>
</div>
<div className="p-2 bg-light-success rounded">
<GraphUp size={24} className="text-success" />
</div>
</div>
<div className="mt-3">
<span className="text-success">
<ArrowUp size={14} className="me-1" />
8.2%
</span>
<span className="text-muted ms-2">Since last month</span>
</div>
</Card.Body>
</Card>
</Col>
<Col md={6} xl={3}>
<Card className="h-100">
<Card.Body>
<div className="d-flex justify-content-between align-items-start">
<div>
<h6 className="text-muted">Total Orders</h6>
<h3 className="mb-0">1,463</h3>
</div>
<div className="p-2 bg-light-primary rounded">
<GraphUp size={24} className="text-primary" />
</div>
</div>
<div className="mt-3">
<span className="text-success">
<ArrowUp size={14} className="me-1" />
5.7%
</span>
<span className="text-muted ms-2">Since last month</span>
</div>
</Card.Body>
</Card>
</Col>
<Col md={6} xl={3}>
<Card className="h-100">
<Card.Body>
<div className="d-flex justify-content-between align-items-start">
<div>
<h6 className="text-muted">Customers</h6>
<h3 className="mb-0">8,549</h3>
</div>
<div className="p-2 bg-light-warning rounded">
<GraphUp size={24} className="text-warning" />
</div>
</div>
<div className="mt-3">
<span className="text-success">
<ArrowUp size={14} className="me-1" />
12.1%
</span>
<span className="text-muted ms-2">Since last month</span>
</div>
</Card.Body>
</Card>
</Col>
<Col md={6} xl={3}>
<Card className="h-100">
<Card.Body>
<div className="d-flex justify-content-between align-items-start">
<div>
<h6 className="text-muted">Refunds</h6>
<h3 className="mb-0">$4,624</h3>
</div>
<div className="p-2 bg-light-danger rounded">
<GraphUp size={24} className="text-danger" />
</div>
</div>
<div className="mt-3">
<span className="text-danger">
<ArrowDown size={14} className="me-1" />
2.3%
</span>
<span className="text-muted ms-2">Since last month</span>
</div>
</Card.Body>
</Card>
</Col>
</Row>
);
}
export default DashboardStats;
Dashboard Chart Component
import React from 'react';
import { Card } from 'react-bootstrap';
function SalesChart() {
// In a real application, you would use a chart library like Chart.js or Recharts
// This is a placeholder for demonstration purposes
return (
<Card className="mb-4">
<Card.Header className="d-flex justify-content-between align-items-center">
<h5 className="mb-0">Sales Overview</h5>
<div>
<select className="form-select form-select-sm">
<option>Last 7 Days</option>
<option>Last 30 Days</option>
<option>Last 90 Days</option>
</select>
</div>
</Card.Header>
<Card.Body>
{/* Placeholder for chart */}
<div
className="bg-light p-3 text-center rounded"
style={{ height: '300px', display: 'flex', alignItems: 'center', justifyContent: 'center' }}
>
<p className="mb-0 text-muted">
Chart showing sales data over time would appear here.<br />
(Using Chart.js, Recharts, or other charting libraries)
</p>
</div>
</Card.Body>
</Card>
);
}
export default SalesChart;
Recent Orders Table Component
import React from 'react';
import { Card, Table, Badge, Button } from 'react-bootstrap';
function RecentOrders() {
const orders = [
{ id: 'ORD-1234', customer: 'John Smith', date: '2023-03-15', amount: 256.80, status: 'Completed' },
{ id: 'ORD-1235', customer: 'Alice Johnson', date: '2023-03-15', amount: 125.50, status: 'Processing' },
{ id: 'ORD-1236', customer: 'Robert Brown', date: '2023-03-14', amount: 458.25, status: 'Completed' },
{ id: 'ORD-1237', customer: 'Emily Davis', date: '2023-03-14', amount: 95.40, status: 'Pending' },
{ id: 'ORD-1238', customer: 'Michael Wilson', date: '2023-03-13', amount: 368.00, status: 'Cancelled' },
];
const getStatusBadge = (status) => {
switch(status) {
case 'Completed':
return <Badge bg="success">Completed</Badge>;
case 'Processing':
return <Badge bg="primary">Processing</Badge>;
case 'Pending':
return <Badge bg="warning">Pending</Badge>;
case 'Cancelled':
return <Badge bg="danger">Cancelled</Badge>;
default:
return <Badge bg="secondary">{status}</Badge>;
}
};
return (
<Card>
<Card.Header className="d-flex justify-content-between align-items-center">
<h5 className="mb-0">Recent Orders</h5>
<Button variant="outline-primary" size="sm">View All</Button>
</Card.Header>
<Table responsive className="mb-0">
<thead>
<tr>
<th>Order ID</th>
<th>Customer</th>
<th>Date</th>
<th>Amount</th>
<th>Status</th>
<th>Action</th>
</tr>
</thead>
<tbody>
{orders.map(order => (
<tr key={order.id}>
<td>{order.id}</td>
<td>{order.customer}</td>
<td>{order.date}</td>
<td>${order.amount.toFixed(2)}</td>
<td>{getStatusBadge(order.status)}</td>
<td>
<Button variant="outline-secondary" size="sm">Details</Button>
</td>
</tr>
))}
</tbody>
</Table>
</Card>
);
}
export default RecentOrders;
Putting It All Together
import React from 'react';
import 'bootstrap/dist/css/bootstrap.min.css';
import DashboardLayout from './components/DashboardLayout';
import DashboardStats from './components/DashboardStats';
import SalesChart from './components/SalesChart';
import RecentOrders from './components/RecentOrders';
import { Row, Col, Card } from 'react-bootstrap';
function App() {
return (
<DashboardLayout>
<DashboardStats />
<Row className="mb-4">
<Col lg={8}>
<SalesChart />
</Col>
<Col lg={4}>
<Card className="h-100">
<Card.Header>
<h5 className="mb-0">Top Products</h5>
</Card.Header>
<Card.Body>
<div className="mb-3">
<div className="d-flex justify-content-between mb-1">
<span>Laptop Pro X</span>
<span>65%</span>
</div>
<div className="progress" style={{ height: '6px' }}>
<div className="progress-bar bg-primary" style={{ width: '65%' }}></div>
</div>
</div>
<div className="mb-3">
<div className="d-flex justify-content-between mb-1">
<span>Smartphone Ultra</span>
<span>52%</span>
</div>
<div className="progress" style={{ height: '6px' }}>
<div className="progress-bar bg-info" style={{ width: '52%' }}></div>
</div>
</div>
<div className="mb-3">
<div className="d-flex justify-content-between mb-1">
<span>Wireless Earbuds</span>
<span>48%</span>
</div>
<div className="progress" style={{ height: '6px' }}>
<div className="progress-bar bg-warning" style={{ width: '48%' }}></div>
</div>
</div>
<div className="mb-3">
<div className="d-flex justify-content-between mb-1">
<span>Smart Watch</span>
<span>35%</span>
</div>
<div className="progress" style={{ height: '6px' }}>
<div className="progress-bar bg-danger" style={{ width: '35%' }}></div>
</div>
</div>
<div>
<div className="d-flex justify-content-between mb-1">
<span>Wireless Charger</span>
<span>24%</span>
</div>
<div className="progress" style={{ height: '6px' }}>
<div className="progress-bar bg-success" style={{ width: '24%' }}></div>
</div>
</div>
</Card.Body>
</Card>
</Col>
</Row>
<RecentOrders />
</DashboardLayout>
);
}
export default App;
This dashboard example demonstrates how to create a comprehensive interface using React and Bootstrap components. It includes:
- A responsive layout with sidebar navigation
- Statistics cards with status indicators
- Placeholder for data visualization charts
- A recent orders table with status badges
- A product performance section with progress bars
Conclusion
Integrating Bootstrap with React provides a powerful combination for creating responsive, visually appealing web applications efficiently. Throughout this guide, we’ve explored various approaches to this integration, from using plain Bootstrap CSS to specialized libraries like React Bootstrap and Reactstrap.
Summary of Key Points
- Multiple Integration Options: Choose the approach that best fits your project needs—direct CSS import for simplicity, React Bootstrap for true React components, Reactstrap for a familiar API, or React hooks for fine-grained control.
- Development Setup: Setting up your project properly from the start will save time and prevent integration issues later. Consider using SASS for deep customization.
- Component Customization: Extend Bootstrap components to match your design requirements using props, wrapper components, or SASS variables.
- Responsive Design: Leverage Bootstrap’s grid system and utility classes to create layouts that work across all device sizes.
- Theming: Customize the appearance of your application with Bootstrap’s theming capabilities and CSS variables.
- Performance Optimization: Implement code splitting, lazy loading, and selective imports to keep your application fast and lightweight.
- Common Patterns: Follow established patterns for component composition, form handling, and modal implementation to maintain a consistent user experience.
- Troubleshooting: Be aware of common issues and their solutions to quickly resolve any problems that arise.
When to Choose Each Approach
- Bootstrap CSS Import: Best for simple projects or when migrating existing Bootstrap sites to React.
- React Bootstrap: Ideal for new projects seeking true React components with Bootstrap styling.
- Reactstrap: Good choice for developers familiar with Bootstrap’s API who want React integration.
- Bootstrap + React Hooks: Suitable for complex use cases requiring fine-grained control over Bootstrap’s JavaScript behavior.
Future Considerations
As both Bootstrap and React continue to evolve, keep an eye on:
- Bootstrap’s increasing focus on utility classes and customization
- React’s new features and patterns
- Emerging integration libraries and approaches
- Performance improvements in both technologies
By mastering the integration of Bootstrap with React, you’ll be well-equipped to create modern, responsive web applications that combine the best of both worlds—Bootstrap’s comprehensive component library and styling system with React’s efficient rendering and component-based architecture.
Whether you’re building a simple landing page or a complex dashboard application, the techniques and patterns covered in this guide will help you achieve your goals with less effort and more consistent results.
What is AI and how can I integrate it into my applications
How to resolve CORS errors in a web application
How to choose between AWS, Azure, and Google Cloud for my application
What Are the Top Programming Languages to Learn in 2024
How to Prepare for Technical Interviews at FAANG Companies
How do I fix “undefined” or “null” errors in JavaScript
How to get started with Machine Learning using Python
How do I implement authentication and authorization in a Node.js application