Explain the concept of lifting state up in React
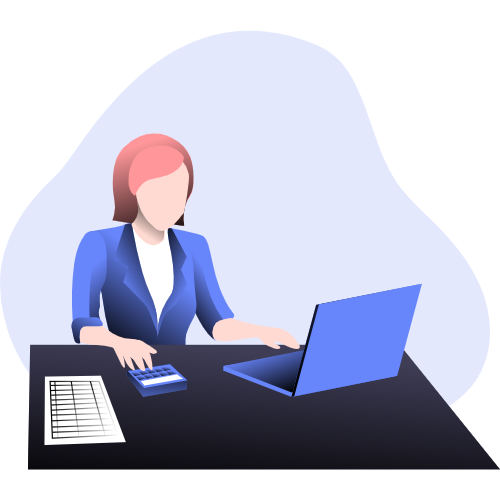
React, a popular JavaScript library for building user interfaces, emphasizes the concept of unidirectional data flow. In larger applications, managing state becomes crucial, and the “lifting state up” pattern emerges as a powerful solution. This pattern involves moving the state from child components to their common ancestor, facilitating better control and synchronization of shared data.
The Need for Lifting State Up
React applications are often structured as component hierarchies, with parent and child components. Each component can have its local state, but as the complexity grows, managing shared state across multiple components becomes challenging. Consider a scenario where two sibling components need to share some data or where a child component needs to update the state of its parent. In such cases, lifting state up becomes an elegant solution.
Basics of State in React
In React, state is a JavaScript object that stores component-specific data, influencing the component’s rendering. Local state is confined to a particular component, and changes in this state trigger a re-render of that component.
However, there are cases where multiple components need access to the same piece of data, and maintaining it in a shared state at a higher level in the component tree becomes advantageous. This is where lifting state up comes into play.
Lifting State Up: How It Works
Lifting state up involves promoting the state from a child component to a common ancestor in the component hierarchy. By doing this, the shared state becomes accessible to both the parent and its children, enabling seamless communication and updates.
Example Scenario
Consider a simple temperature conversion application with two components: TemperatureInput
and Calculator
. Each TemperatureInput
component handles the input for a specific scale (Celsius or Fahrenheit). The Calculator
component manages the shared state, converting the temperature between the two scales.
Implementation Steps
-
Create TemperatureInput Component
TemperatureInput
maintains its local state for the input value.
class TemperatureInput extends React.Component {
constructor(props) {
super(props);
this.state = { temperature: '' };
}
render() {
return (
<fieldset>
<legend>Enter temperature in {this.props.scale}:</legend>
<input
value={this.state.temperature}
onChange={(e) => this.setState({ temperature: e.target.value })}
/>
</fieldset>
);
}
}
-
Create Calculator Component
Calculator
component lifts the state up and manages the shared temperature value.
class Calculator extends React.Component {
constructor(props) {
super(props);
this.state = { temperature: '', scale: 'celsius' };
}
render() {
const celsius =
this.state.scale === 'celsius'
? this.state.temperature
: toCelsius(this.state.temperature);
const fahrenheit =
this.state.scale === 'fahrenheit'
? this.state.temperature
: toFahrenheit(this.state.temperature);
return (
<div>
<TemperatureInput scale="celsius" />
<TemperatureInput scale="fahrenheit" />
</div>
);
}
}
In this example, the Calculator
component holds the shared state (temperature
) and passes it down to each TemperatureInput
as a prop. The TemperatureInput
components then update the shared state through callbacks.
Benefits of Lifting State Up
1. Single Source of Truth
- Lifting state up promotes a single source of truth for shared data. The shared state resides in a common ancestor, simplifying data management and reducing inconsistencies.
2. Easier Debugging and Testing
- With state lifted to a higher level, debugging becomes more straightforward. Developers can inspect and modify the shared state at the common ancestor, enhancing code maintainability and testability.
3. Facilitates Propagation of Changes
- Changes to the shared state trigger re-renders in the common ancestor, ensuring that all components receiving the state as props update accordingly. This facilitates a predictable flow of data changes throughout the component tree.
4. Encourages Reusability
- Lifting state up encourages the creation of more modular and reusable components. Child components become stateless and focused on presentation, while the parent components manage the shared state and business logic.
Potential Challenges and Considerations
1. Prop Drilling
- Lifting state up may lead to “prop drilling,” where intermediate components receive the shared state as props without actually using it. This can be addressed by using context or state management libraries like Redux.
2. Complex Component Trees
- In large applications, component hierarchies can become complex. Lifting state up might result in passing props through multiple levels. Context or other state management solutions might be more appropriate in such scenarios.
3. Maintaining Immutability
- When lifting state up, care must be taken to maintain immutability. Directly modifying state can lead to unexpected behavior and bugs. Immutability ensures that changes are tracked correctly by React.
Conclusion
In React, lifting state up is a powerful pattern that addresses the challenges of managing shared state in component hierarchies. By promoting the state to a common ancestor, developers can create more maintainable, predictable, and scalable applications. Understanding when and how to lift state up is a crucial skill for React developers, enabling them to design robust and efficient component architectures.
- What is render () in React
- What is JSX in React
- How many states are there in React
- Is Redux still relevant 2023
- Where is state stored in React
- Why Babel is used in React
- Why React is better than JSX
- Why use React without JSX
- What is frontend state
- What is difference between variable and state in React
- What is useEffect in React
- Why we should never update React state directly