What is the lifecycle of React
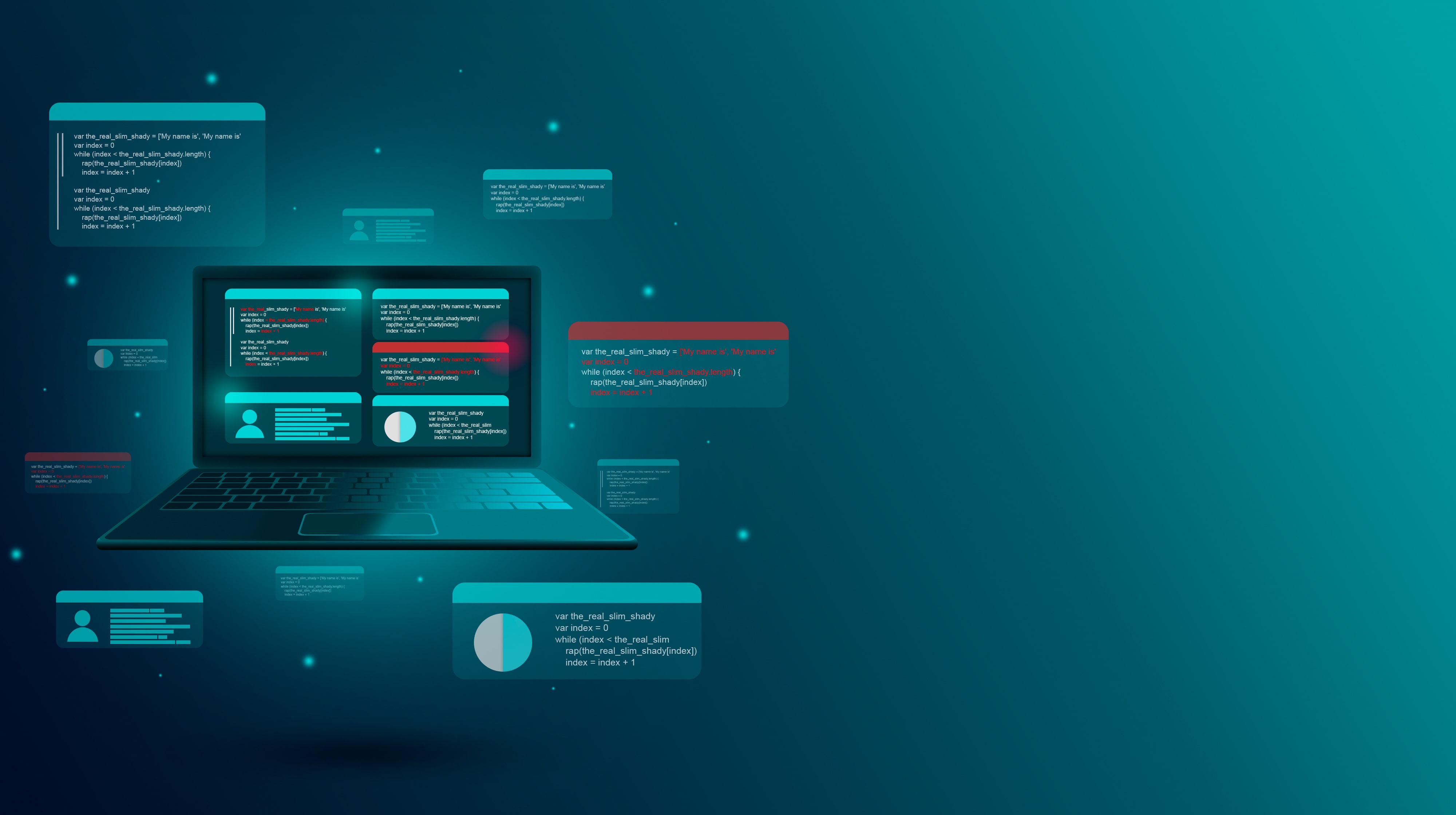
The lifecycle of a React component refers to the series of phases that a component goes through, from its creation to its removal from the DOM (Document Object Model). React components have several lifecycle methods that allow developers to hook into different points in this process. However, it’s important to note that with the introduction of React Hooks, the use of class components and lifecycle methods has been somewhat superseded by functional components and hooks.
Here is an overview of the lifecycle phases for class components;
-
Initialization
- constructor(): This is called when a component is created. It’s used for initializing state and binding methods.
-
Mounting
- componentWillMount() (deprecated): Invoked just before rendering (on both the client and server).
- render(): Responsible for rendering the component.
- componentDidMount(): Invoked after the component is mounted to the DOM. It is often used for network requests or interacting with the DOM.
-
Updating
- componentWillReceiveProps() (deprecated): Invoked before a mounted component receives new props.
- shouldComponentUpdate(): Allows the developer to prevent unnecessary re-rendering by returning
true
orfalse
. - componentWillUpdate() (deprecated): Invoked just before rendering when new props or state are being received.
- render(): Re-renders the component.
- componentDidUpdate(): Invoked after the component’s updates are flushed to the DOM. It’s often used for updating the DOM in response to prop or state changes.
-
Unmounting
- componentWillUnmount(): Invoked just before a component is unmounted and destroyed. It’s used for cleanup tasks such as canceling network requests, clearing up subscriptions, etc.
-
Error Handling (introduced in React 16):
- componentDidCatch(): Catches JavaScript errors anywhere in the component tree and logs those errors.
With the introduction of React Hooks, functional components can now use hooks like useEffect
to achieve similar lifecycle behavior. The lifecycle methods described above are part of class components and are considered legacy. Functional components with hooks are now the preferred way of writing components in React.
What is render () in React
In React, the render()
method is a mandatory method for class components. It is responsible for determining what will be displayed on the UI. The render()
method returns a React element, which is a lightweight description of what to render.
Here’s a simple example of a React class component with a render()
method:
import React, { Component } from 'react';
class MyComponent extends Component {
render() {
return (
<div>
<h1>Hello, React!</h1>
<p>This is a simple React component.</p>
</div>
);
}
}
export default MyComponent;
In this example, the render()
method returns a JSX element, which describes the structure of the component. The JSX syntax looks similar to HTML but is transpiled by tools like Babel into JavaScript.
When a component is initially mounted or when its state/props change, the render()
method is called to update the UI. It describes what the UI should look like based on the current state and props of the component.
It’s important to note that while render()
is a crucial method in class components, functional components in React Hooks don’t have a render()
method. Instead, they return the JSX directly from the function body. Here’s an example of a functional component:
import React from 'react';
function MyFunctionalComponent() {
return (
<div>
<h1>Hello, React Hooks!</h1>
<p>This is a functional React component.</p>
</div>
);
}
export default MyFunctionalComponent;
In functional components, the return value serves the same purpose as the render()
method in class components.
What is JSX in React
JSX (JavaScript XML) is a syntax extension for JavaScript that looks similar to XML or HTML. It is a syntax sugar provided by React to describe what the UI should look like. JSX allows you to write HTML elements and components in a syntax that closely resembles XML or HTML but is still valid JavaScript.
Here’s a simple example of JSX:
const element = <h1>Hello, JSX!</h1>;
In the example above, the <h1>
tag is not a string; it’s JSX syntax. JSX gets transformed into regular JavaScript function calls during the build process. In the case of React, it gets translated into calls to React.createElement
:
const element = React.createElement('h1', null, 'Hello, JSX!');
This React.createElement
function call creates a virtual DOM element, which React uses to efficiently update the actual DOM when the state or props of a component change.
JSX allows you to embed JavaScript expressions within curly braces {}
. This means you can dynamically insert values into your JSX code. Here’s an example:
const name = 'John';
const element = <p>Hello, {name}!</p>;
In the above example, the value of the name
variable is inserted into the JSX code.
JSX makes it more concise and readable to describe the structure of your UI in React components. While it’s not mandatory to use JSX with React, it’s a widely adopted and recommended practice as it makes the code more expressive and closer to the way UIs are typically written in HTML.
How many states are there in React
In React, the term “state” typically refers to the state
object within a component. Each React component can have its own local state, which is an object that represents properties that can be updated and influence the rendering of the component.
The number of states in a React application is not fixed and depends on the design and structure of the application. Each component can manage its own state independently. A React application can have numerous components, each with its own state.
Additionally, in React applications, you may encounter other types of state management solutions beyond local component state. For example;
- Redux State: If you use Redux for state management, the entire application state is often centralized and managed through a single Redux store.
- Context API State: React provides a Context API that allows you to share state across components without having to pass it explicitly through props. This can be considered a form of shared state.
- Router State: In applications with client-side routing (using React Router or a similar library), the router state may also be a part of the overall state.
Therefore, the number of states in a React application can vary depending on the size and complexity of the application, as well as the chosen state management strategies. Each component, and potentially other state management solutions, may contribute to the overall state of the application.
Is Redux still relevant 2023
As of my last knowledge update in January 2023, Redux continued to be a relevant and widely used state management library in the React ecosystem. However, the relevance of Redux in 2023 would depend on the evolving landscape of web development, changes in technology, and the preferences of the developer community.
Here are some factors to consider;
- React Context API and Hooks: The React Context API and the introduction of Hooks (like
useReducer
) provide alternative ways to manage state in React components. For simpler applications or smaller state management needs, developers might choose to use these built-in React features without incorporating Redux. - State Management Trends: The landscape of state management in React is continually evolving. New libraries and patterns may emerge, and the community’s preferences may shift over time. It’s essential to stay updated on the latest trends and best practices in state management.
- Redux Toolkit: The Redux team has introduced the Redux Toolkit, which simplifies the process of working with Redux and helps address some of the boilerplate concerns associated with Redux. It includes utilities like
createSlice
to reduce the amount of code needed. - Specific Use Cases: Redux is well-suited for managing complex global state in larger applications with many interconnected components. If your application has a need for a centralized, predictable state container with features like time-travel debugging and middleware support, Redux might still be a relevant choice.
- Community Adoption: The strength of Redux lies not only in its features but also in its large and active community. If many projects and developers continue to use and contribute to Redux, it will likely remain relevant.
To get the most up-to-date information on the relevance of Redux in 2023, consider checking recent blog posts, community forums, and the official documentation for Redux and React. Additionally, evaluate your specific project requirements and choose the state management solution that best fits your needs.
Where is state stored in React
In React, the state
of a component is a JavaScript object that represents the data specific to that component. The state
is mutable and can be updated over time. Each React component can have its own local state, which is managed internally by the component itself.
When a component’s state changes, React automatically re-renders the component, updating the UI to reflect the new state. The state is an integral part of a component’s internal logic and is used to determine what the rendered output should be.
Here’s an example of how state is defined and used in a React class component:
import React, { Component } from 'react';
class Counter extends Component {
constructor(props) {
super(props);
// Initializing state
this.state = {
count: 0
};
}
// Updating state
incrementCount = () => {
this.setState({ count: this.state.count + 1 });
};
render() {
return (
<div>
<p>Count: {this.state.count}</p>
<button onClick={this.incrementCount}>Increment</button>
</div>
);
}
}
export default Counter;
In the example above
- The
constructor
method is used to initialize the component’s state with an initial value ofcount: 0
. - The
incrementCount
method is defined to update thecount
state when a button is clicked. ThesetState
method is used to modify the state. - The
render
method uses the current state (this.state.count
) to display the count in the rendered output.
It’s important to note that the component’s state is local to that specific component. If you need to share state between components, you may consider lifting the state up to a common ancestor or using other state management solutions like Redux or the React Context API.
Why Babel is used in React
Babel is a JavaScript compiler that allows developers to use the latest ECMAScript features and syntax in their code, even if the environments where the code will run do not support those features natively. In the context of React, Babel is commonly used for a couple of key reasons;
-
JSX Transformation
- React uses JSX (JavaScript XML) syntax to describe the structure of UI components. JSX is a syntax extension for JavaScript that looks similar to XML or HTML. However, browsers don’t understand JSX directly. Babel is used to transpile JSX into plain JavaScript that browsers can interpret.
// JSX code const element = <h1>Hello, React!</h1>; // Babel-transpiled JavaScript code const element = React.createElement('h1', null, 'Hello, React!');
The transformed code allows developers to write more readable and expressive code using JSX while ensuring compatibility with browsers.
-
ES6/ESNext Features
- Babel allows developers to use the latest ECMAScript features and syntax (such as arrow functions, destructuring, async/await, etc.) without worrying about compatibility issues with older browsers. Babel transpiles modern JavaScript code into an older version of JavaScript that is widely supported.
// ES6 code const add = (a, b) => a + b; // Babel-transpiled JavaScript code var add = function add(a, b) { return a + b; };
This enables developers to write more maintainable and concise code while still supporting a broad range of browsers.
In a React project, Babel is often configured along with tools like Webpack to create a build process. This build process involves transpiling JSX and modern JavaScript code, as well as bundling and optimizing the code for production. By using Babel, React developers can take advantage of the latest language features and write code in a more modern and efficient way, ensuring broad compatibility across different browsers and environments.
Why React is better than JSX
It seems there might be a misunderstanding in the question. React and JSX are not alternatives to each other; in fact, they are closely related concepts, and one is not considered “better” than the other. Let me clarify their relationship:
-
React
- React is a JavaScript library for building user interfaces. It was developed by Facebook and is widely used for creating dynamic and efficient UIs.
- React provides a component-based architecture, allowing developers to build reusable and modular UI elements.
- React also includes a virtual DOM (Document Object Model) for optimizing the performance of UI updates.
-
JSX (JavaScript XML)
- JSX is a syntax extension for JavaScript often used with React. It allows developers to write UI elements in a syntax that looks similar to XML or HTML.
- JSX makes the code more readable and expressive. It’s a convenient way to describe what the UI should look like.
- JSX gets transpiled by tools like Babel into regular JavaScript code that React understands. The transpiled code is what actually gets executed in the browser.
Here’s an example of how React and JSX are used together;
import React from 'react';
class MyComponent extends React.Component {
render() {
return (
<div>
<h1>Hello, React with JSX!</h1>
<p>This is a JSX syntax in action.</p>
</div>
);
}
}
In the example above, the <div>
and <p>
elements are written using JSX syntax. React processes and transforms this JSX code into JavaScript code that creates and updates the corresponding DOM elements.
In summary, JSX is a beneficial and integral part of React, making the code more readable and resembling the final UI structure. React and JSX work together to provide a powerful and declarative way to build user interfaces. It’s not a matter of React being better than JSX; rather, JSX is a key feature that enhances the development experience when working with React.
Why use React without JSX
While JSX is a popular and commonly used syntax with React, it’s not mandatory to use JSX when working with React. You can write React applications using plain JavaScript, but JSX offers several advantages that make the development process more efficient and expressive. However, there might be scenarios where developers choose not to use JSX. Here are some reasons why someone might consider using React without JSX;
-
Preference for JavaScript
- Some developers may prefer writing plain JavaScript and find JSX syntax less familiar or more verbose. They might choose to avoid JSX and use
React.createElement
directly to create React elements.
const element = React.createElement('h1', null, 'Hello, React without JSX!');
- Some developers may prefer writing plain JavaScript and find JSX syntax less familiar or more verbose. They might choose to avoid JSX and use
-
Build System Limitations
- In certain build environments or tooling setups, using JSX might not be configured or supported. While this is less common, it could be a factor in scenarios where the build process is constrained.
-
Learning Purposes
- In educational contexts or when introducing React to beginners, some tutorials might initially teach React without JSX to provide a clearer understanding of the underlying JavaScript concepts.
const element = React.createElement('div', null, React.createElement('h1', null, 'Hello, React without JSX!'), React.createElement('p', null, 'This is a React element created without JSX.') );
-
Integration with Other Libraries
- If you’re integrating React into an existing project or working with other libraries that prefer plain JavaScript, you might choose to avoid JSX to maintain consistency.
const element = React.createElement('button', { onClick: handleClick }, 'Click me');
While using React without JSX is possible, JSX is widely adopted and recommended by the React community due to its readability, conciseness, and similarity to HTML. It’s essential to understand that JSX gets transpiled into JavaScript during the build process, and the resulting code is essentially the same whether you write JSX or use React.createElement
directly. Ultimately, the choice between JSX and plain JavaScript is a matter of personal preference and project requirements.
What is frontend state
Frontend state refers to the data that is managed and maintained on the client side of a web application within the frontend or user interface. In a web application, the frontend is responsible for presenting the user interface and handling user interactions. The frontend state encompasses various aspects of the application’s data and behavior that are directly related to the presentation layer.
Here are some key components of frontend state;
-
Component State
- Many frontend frameworks, such as React, Angular, and Vue.js, allow developers to manage the state of individual UI components. This includes data specific to a particular component, such as input values, visibility flags, and other UI-related information.
-
UI State
- UI state encompasses information related to the visual presentation of the user interface. This includes things like the current state of modal dialogs, the visibility of certain elements, the selected tab in a tabbed interface, etc.
-
Form State
- When users interact with forms on a website, the state of the form elements, such as input values, checkboxes, radio buttons, and dropdown selections, is considered part of the frontend state.
-
Client-Side Routing State
- In single-page applications (SPAs), the state of the client-side router, including the current route or URL parameters, is part of the frontend state. This is especially relevant when using frontend libraries or frameworks that handle client-side routing.
-
Session State
- Information related to the user’s session, such as authentication status, user roles, and user-specific preferences, is considered frontend state. This information is often stored in local storage or session storage.
-
Global State Management
- In larger applications, there might be a need for global state management to share data between components that are not directly connected. This can be achieved through state management libraries or patterns like Redux in React, Vuex in Vue.js, or Angular services.
Frontend state is dynamic and can change based on user interactions, form submissions, or other events. Effective management of frontend state is crucial for creating responsive and interactive user interfaces. Different frontend libraries and frameworks provide tools and patterns for managing state in a structured and scalable way.
What is difference between variable and state in React
In React, both variables and state are used to store and manage data, but they serve different purposes and are used in different contexts.
1. Variables
-
Variables in React, like in JavaScript in general, are used to store values that can be changed or manipulated during the execution of the program.
-
Variables declared within a component using
const
,let
, orvar
are local to that component’s scope. -
Variables are typically used for holding temporary values, computations, or constants within the scope of a function or a component.
Example:
function MyComponent() {
const message = "Hello, React!";
// ...
}
2. State
-
State in React is a special type of variable that is used to manage and track changes to data that affect the rendering of the component.
-
State is managed using the
useState
hook (for functional components) or thethis.state
object (for class components). -
When state is updated, React will automatically re-render the component to reflect the changes in the UI.
Example using
useState
:
import React, { useState } from 'react';
function MyComponent() {
const [count, setCount] = useState(0);
const increment = () => {
setCount(count + 1);
};
// ...
}
In summary, while variables are used for general data storage and manipulation within a local scope, state in React is specifically designed for managing dynamic data that affects the rendering of a component. State is essential for creating interactive and dynamic user interfaces in React, as it triggers updates to the UI when the data it manages changes.
What is useEffect in React
In React, useEffect
is a Hook that allows you to perform side effects in your functional components. Side effects refer to tasks that are typically not directly related to rendering UI but are essential for certain functionalities, such as data fetching, subscriptions, manual DOM manipulations, etc.
The useEffect
Hook is used to manage side effects in a functional component, and it is executed after the component has been rendered to the screen. It takes two arguments: a function containing the code to run for the side effect, and an optional dependency array.
Here’s a basic example of using useEffect
:
import React, { useEffect, useState } from 'react';
function MyComponent() {
const [data, setData] = useState(null);
useEffect(() => {
// Code inside this block will run after every render
// Perform side effects here
// Example: Fetching data from an API
const fetchData = async () => {
const response = await fetch('https://api.example.com/data');
const result = await response.json();
setData(result);
};
fetchData();
// Cleanup function (optional)
return () => {
// Code to run on component unmount or before the next render
// (similar to componentWillUnmount in class components)
};
}, []); // Dependency array
// Render UI based on the data
return (
<div>
{data ? (
<p>Data: {data}</p>
) : (
<p>Loading...</p>
)}
</div>
);
}
export default MyComponent;
In this example:
- The
useEffect
Hook is used to fetch data from an API after the component has been rendered. - The empty dependency array (
[]
) indicates that the effect has no dependencies, and it should only run once after the initial render. If you have dependencies, you can include them in the array to make the effect run whenever those dependencies change. - The
return
statement inside theuseEffect
function is used for cleanup. It’s optional but can be used to perform cleanup when the component unmounts or before the next render.
useEffect
is a powerful and flexible tool for managing side effects in functional components and is a key feature of React Hooks.
Why we should never update React state directly
In React, it is strongly advised not to update the state directly by modifying its value directly, and instead, the setState
method should be used. Here are the reasons why you should avoid updating React state directly:
-
Asynchronous State Updates
- The
setState
method in React is asynchronous. When you callsetState
, React schedules an update to the component’s state, and the new state may not be immediately available. If you update the state directly, you might not get the expected behavior.
- The
-
Batching of State Updates
- React batches state updates for performance reasons. If you directly mutate the state multiple times, React may batch those changes into a single update. If you bypass
setState
, React may not be aware of these changes and could miss updates or apply them out of order.
- React batches state updates for performance reasons. If you directly mutate the state multiple times, React may batch those changes into a single update. If you bypass
-
Reconciliation Process
- React uses a process called reconciliation to determine the most efficient way to update the DOM based on changes in the component state. Directly modifying state can lead to inconsistencies in the internal representation of the component and result in unexpected behavior during reconciliation.
-
Component Re-rendering
- React relies on the
setState
method to trigger a re-render of the component when the state changes. If you modify the state directly, React might not recognize the change, and the component may not update as expected.
- React relies on the
-
Immutability for Predictable Behavior
- React encourages the use of immutable data structures to ensure a predictable state and behavior. Directly updating the state violates the principle of immutability, making it harder to reason about the application’s state and causing unexpected bugs.
Here is an example illustrating the correct usage of setState;
// Incorrect
this.state.counter = this.state.counter + 1; // Direct mutation
// Correct
this.setState((prevState) => ({
counter: prevState.counter + 1,
}));
In the correct example, the setState
function is used with a callback that receives the previous state (prevState
). This ensures that the state is updated based on the previous state, avoiding issues related to asynchronous updates and ensuring the correctness of the state changes.
By following the recommended practice of using setState
, you can help maintain the integrity of your React application and prevent hard-to-debug issues associated with direct state mutations.
How does React handle component-based architecture
What is the significance of state in React
What are states in React functional components
What is the purpose of the “purge” option in the Tailwind CSS configuration
What are the utility classes for responsive design in Tailwind CSS
How do I create a fixed navbar using Tailwind CSS
How to use Bootstrap’s utilities for hiding and showing elements
How to implement a sticky footer with a content area that scrolls