How can you optimize CSS delivery in a Next.js project
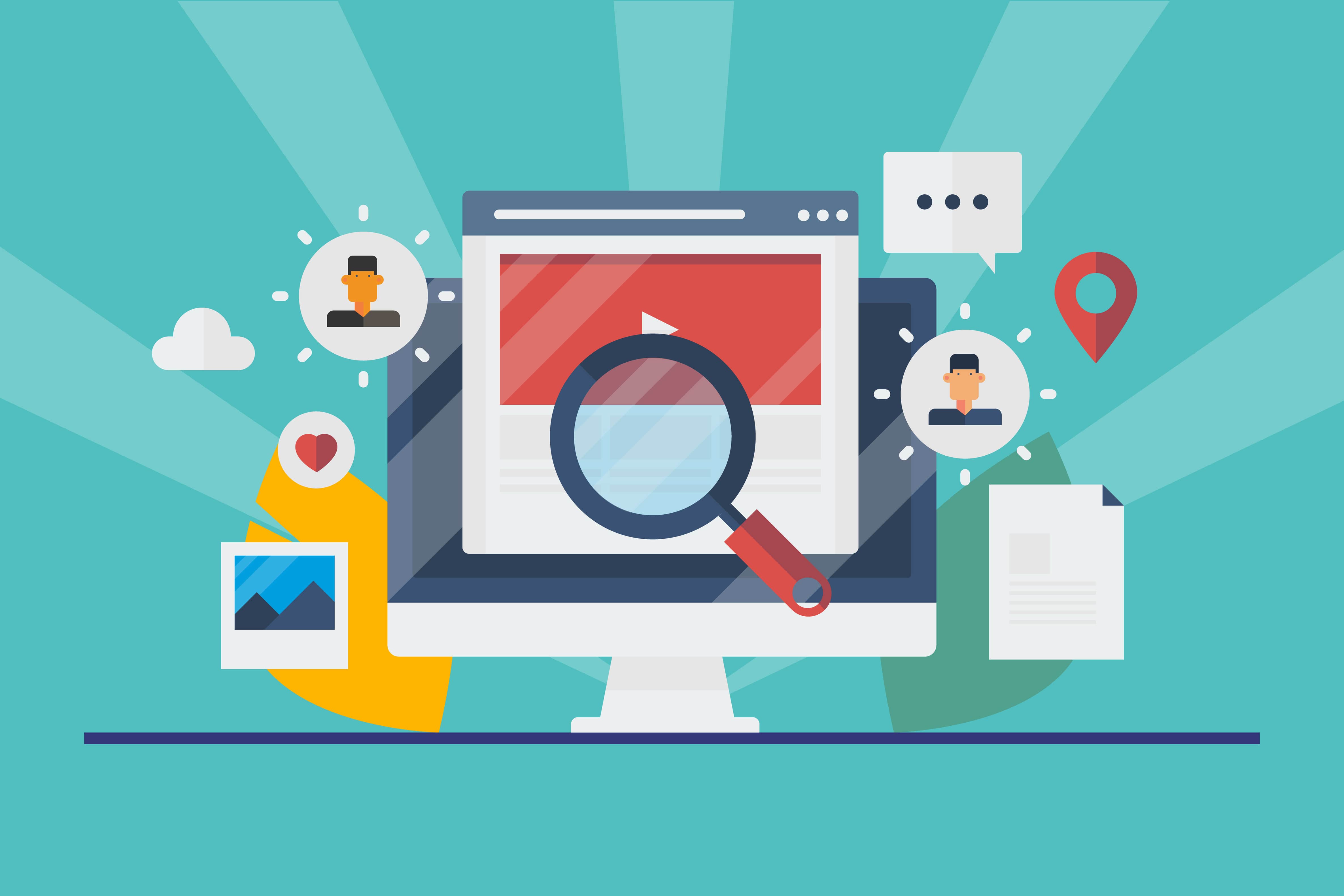
Optimizing CSS delivery in a Next.js project is crucial for improving the performance and user experience of your web application. Efficient CSS delivery reduces load times, minimizes render-blocking resources, and ensures that your site is visually rendered as quickly as possible. In this article, we will explore various techniques and strategies to optimize CSS delivery in a Next.js project.
Understanding CSS in Next.js
Next.js is a popular React framework that provides a robust set of features for building modern web applications, including server-side rendering, static site generation, and API routes. It also supports CSS out of the box, allowing you to use global CSS files, CSS Modules, and even CSS-in-JS libraries. However, simply adding CSS to your project is not enough for optimal performance. Let’s dive into the methods for optimizing CSS delivery.
1. Minimizing CSS
a. CSS Minification
Minifying CSS involves removing unnecessary whitespace, comments, and characters, reducing the file size. This can be easily achieved using tools like cssnano
or built-in support in Next.js via the next.config.js
file.
// next.config.js
module.exports = {
webpack(config, { isServer }) {
if (!isServer) {
const OptimizeCSSAssetsPlugin = require('optimize-css-assets-webpack-plugin');
config.optimization.minimizer.push(new OptimizeCSSAssetsPlugin({}));
}
return config;
},
};
b. Removing Unused CSS
Unused CSS, or “dead CSS,” adds unnecessary weight to your stylesheets. Tools like PurgeCSS can help you remove unused CSS by analyzing your codebase and eliminating styles that are not used in your HTML files.
Next.js can integrate with PurgeCSS easily:
- Install PurgeCSS and the necessary plugins:
npm install @fullhuman/postcss-purgecss
- Configure PurgeCSS in your
postcss.config.js
:
// postcss.config.js
const purgecss = require('@fullhuman/postcss-purgecss')({
content: [
'./pages/**/*.{js,jsx,ts,tsx}',
'./components/**/*.{js,jsx,ts,tsx}',
],
defaultExtractor: content => content.match(/[\w-/:]+(?<!:)/g) || [],
});
module.exports = {
plugins: [
'postcss-flexbugs-fixes',
'postcss-preset-env',
...(process.env.NODE_ENV === 'production' ? [purgecss] : []),
],
};
2. Using CSS Modules
CSS Modules scope CSS by automatically generating unique class names, avoiding conflicts, and minimizing the CSS payload sent to the client. Next.js has built-in support for CSS Modules:
- Create a CSS Module file with the
.module.css
extension:
/* styles.module.css */
.button {
background-color: blue;
color: white;
}
- Import and use it in your components:
import styles from './styles.module.css';
export default function MyComponent() {
return <button className={styles.button}>Click me</button>;
}
3. Critical CSS and Lazy Loading
a. Critical CSS
Critical CSS involves extracting and inlining the CSS required for above-the-fold content, ensuring that the initial render is as fast as possible. Next.js can leverage the next-critters
package to automate this process.
- Install the
next-critters
package:
npm install next-critters
- Update your
next.config.js
to usenext-critters
:
// next.config.js
const withCritters = require('next-critters');
module.exports = withCritters({
/* other next.js config options */
});
b. CSS Lazy Loading
Lazy loading CSS means loading non-critical CSS files asynchronously to avoid blocking the rendering of the page. This can be achieved using the next/dynamic
component for loading CSS files conditionally.
import dynamic from 'next/dynamic';
const MyComponent = dynamic(() => import('./MyComponent'), {
ssr: false,
});
export default function HomePage() {
return (
<div>
<MyComponent />
</div>
);
}
In this example, MyComponent
and its CSS will only be loaded on the client side, preventing it from blocking the initial server-side render.
4. Code Splitting and Bundle Optimization
Next.js automatically splits your code into smaller bundles to improve load times. However, you can optimize it further by ensuring that only necessary CSS is included in each page’s bundle.
a. Dynamic Imports
Using dynamic imports, you can load CSS files or components with CSS only when they are needed.
import dynamic from 'next/dynamic';
const DynamicComponent = dynamic(() => import('./DynamicComponent'));
export default function HomePage() {
return (
<div>
<DynamicComponent />
</div>
);
}
b. Analyzing Bundles
Next.js provides a way to analyze your bundles using webpack-bundle-analyzer
. This helps identify large or duplicate CSS files.
- Install
webpack-bundle-analyzer
:
npm install @next/bundle-analyzer
- Configure it in
next.config.js
:
// next.config.js
const withBundleAnalyzer = require('@next/bundle-analyzer')({
enabled: process.env.ANALYZE === 'true',
});
module.exports = withBundleAnalyzer({
/* other next.js config options */
});
- Run the build with analysis:
ANALYZE=true npm run build
5. Leveraging CSS-in-JS
CSS-in-JS libraries like styled-components or emotion allow you to write CSS directly within your JavaScript files, scoped to the component. This approach can improve performance by only injecting the CSS that is necessary for the components on the current page.
a. Styled-Components
- Install styled-components:
npm install styled-components
npm install --save-dev babel-plugin-styled-components
- Configure Babel to use the styled-components plugin:
// .babelrc
{
"presets": ["next/babel"],
"plugins": ["babel-plugin-styled-components"]
}
- Use styled-components in your components:
import styled from 'styled-components';
const Button = styled.button`
background-color: blue;
color: white;
`;
export default function HomePage() {
return <Button>Click me</Button>;
}
b. Emotion
- Install emotion:
npm install @emotion/react @emotion/styled
- Use emotion in your components:
/** @jsxImportSource @emotion/react */
import { css } from '@emotion/react';
const buttonStyle = css`
background-color: blue;
color: white;
`;
export default function HomePage() {
return <button css={buttonStyle}>Click me</button>;
}
6. Caching and Content Delivery Network (CDN)
Caching and CDNs can significantly improve the delivery of your CSS files. By caching CSS files, you reduce the number of requests made to the server. Using a CDN ensures that your CSS files are served from a location closer to the user.
a. Caching
Leverage caching headers to instruct browsers to cache CSS files for a longer duration. Next.js provides a way to set headers in next.config.js
:
// next.config.js
module.exports = {
async headers() {
return [
{
source: '/(.*).css',
headers: [
{
key: 'Cache-Control',
value: 'public, max-age=31536000, immutable',
},
],
},
];
},
};
b. Content Delivery Network (CDN)
Using a CDN to serve your CSS files can drastically reduce the load time for users globally. Next.js allows you to configure the assetPrefix
to serve static assets from a CDN:
// next.config.js
module.exports = {
assetPrefix: 'https://your-cdn-url.com',
};
7. Preloading and Prefetching
a. Preloading CSS
Preloading CSS files ensures that critical CSS is loaded as soon as possible, reducing the render-blocking time. You can use the next/head
component to add preload links.
import Head from 'next/head';
export default function HomePage() {
return (
<>
<Head>
<link rel="preload" href="/styles.css" as="style" />
</Head>
<div>
{/* Page content */}
</div>
</>
);
}
b. Prefetching CSS
Prefetching CSS files allows the browser to fetch non-critical CSS in the background, improving subsequent page load times. Next.js automatically prefetches linked pages' assets, but you can manually add prefetch links if needed.
import Link from 'next/link';
export default function HomePage() {
return (
<div>
<Link href="/about">
<a>About</a>
</Link>
<link rel="prefetch" href="/styles/about.css" />
</div>
);
}
Conclusion
Optimizing CSS delivery in a Next.js project involves a combination of techniques aimed at reducing the size of CSS files, ensuring critical CSS is delivered promptly, and leveraging modern web performance practices like code splitting, caching, and using CDNs. By implementing these strategies, you can significantly enhance the performance and user experience of your Next.js application.
To summarize, here are the key points:
- Minimize CSS: Use minification and remove unused CSS with tools like PurgeCSS.
- CSS Modules: Scope CSS locally to components to avoid conflicts and reduce global CSS.
- Critical CSS and Lazy Loading: Inline critical CSS and load non-critical CSS asynchronously.
- Code Splitting and Bundle Optimization: Use dynamic imports and bundle analyzers to optimize CSS bundles.
- CSS-in-JS: Utilize libraries like styled-components or emotion for component-scoped styling.
- Caching and CDN: Cache CSS files and serve them via a CDN for faster delivery.
- Preloading and Prefetching: Preload critical CSS and prefetch non-critical CSS for improved load times.
What is the significance of the api folder in a Next.js project
What is the purpose of the basePath and assetPrefix options in next.config.js
What is the significance of the _document.js file in a Next.js project
How does Next.js handle data fetching for server-side rendering (SSR)
Explain how to use environment variables in a Next.js application
How can you implement custom meta tags for SEO in a Next.js application