How do I create a responsive layout with Tailwind CSS?
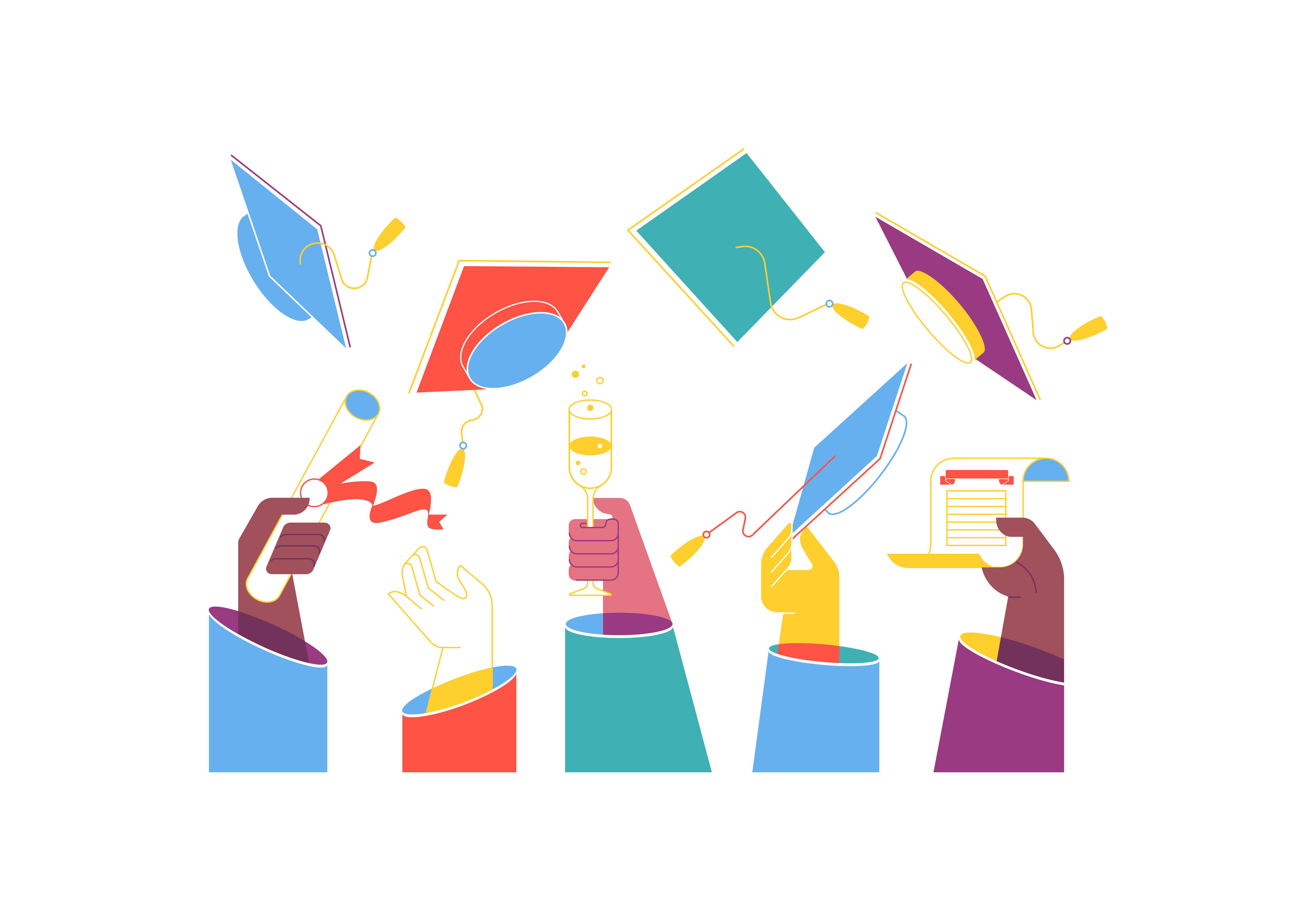
Tailwind CSS is one of the most popular front-end frameworks for designing responsive websites. It provides pre-defined classes to help you create a responsive layout without writing any custom CSS code. In this article, we will explore how to create a responsive layout with Tailwind CSS.
Step 1: Install Tailwind CSS
Before creating a responsive layout with Tailwind CSS, you need to install it in your project. You can do this by running the following command in your terminal:
npm install tailwindcss
Once installed, you can create a configuration file for Tailwind CSS by running the following command:
npx tailwindcss init
This will generate a configuration file named tailwind.config.js
in your project directory.
Step 2: Create a Responsive Layout
Now that you have Tailwind CSS installed, you can start creating your responsive layout. To do this, you need to define the screen sizes for which you want to create a responsive design. You can do this by adding the following code to your tailwind.config.js
file:
module.exports = {
// Define the screen sizes for which you want to create a responsive design
screens: {
'sm': '640px',
'md': '768px',
'lg': '1024px',
'xl': '1280px'
},
// Define the layout for each screen size
layout: {
'sm': {
'container': 'flex',
'justify-content': 'center',
'align-items': 'center'
},
'md': {
'container': 'flex-grow',
'justify-content': 'space-between',
'align-items': 'baseline'
},
'lg': {
'container': 'flex-grow',
'justify-content': 'space-between',
'align-items': 'center'
},
'xl': {
'container': 'flex-grow',
'justify-content': 'space-between',
'align-items': 'baseline'
}
}
}
This code defines four screen sizes (sm
, md
, lg
, and xl
) and assigns a layout to each size. You can adjust the values for each screen size based on your needs.
Step 3: Add Responsive Classes
–
Now that you have defined your responsive layout, you need to add responsive classes to your HTML elements. To do this, you can use the following classes:
| Class Name | Description |
| | |
| sm:*
| Applies a class to an element only on small screens (640px) |
| md:*
| Applies a class to an element only on medium screens (768px) |
| lg:*
| Applies a class to an element only on large screens (1024px) |
| xl:*
| Applies a class to an element only on extra-large screens (1280px) |
For example, if you want an element to have a certain style only on small screens, you can add the following code to your HTML:
<div class="sm:text-xl">This text will only be visible on small screens</div>
This will apply the text-xl
class to the element only on small screens.
Conclusion
In this article, we learned how to create a responsive layout with Tailwind CSS. We defined screen sizes for which we want to create a responsive design and assigned a layout to each size. We also added responsive classes to our HTML elements to apply styles based on the screen size. With these techniques, you can create a responsive layout that works well on all screens, regardless of their size.