How do I integrate Tailwind CSS with a JavaScript framework like React or Vue.js?
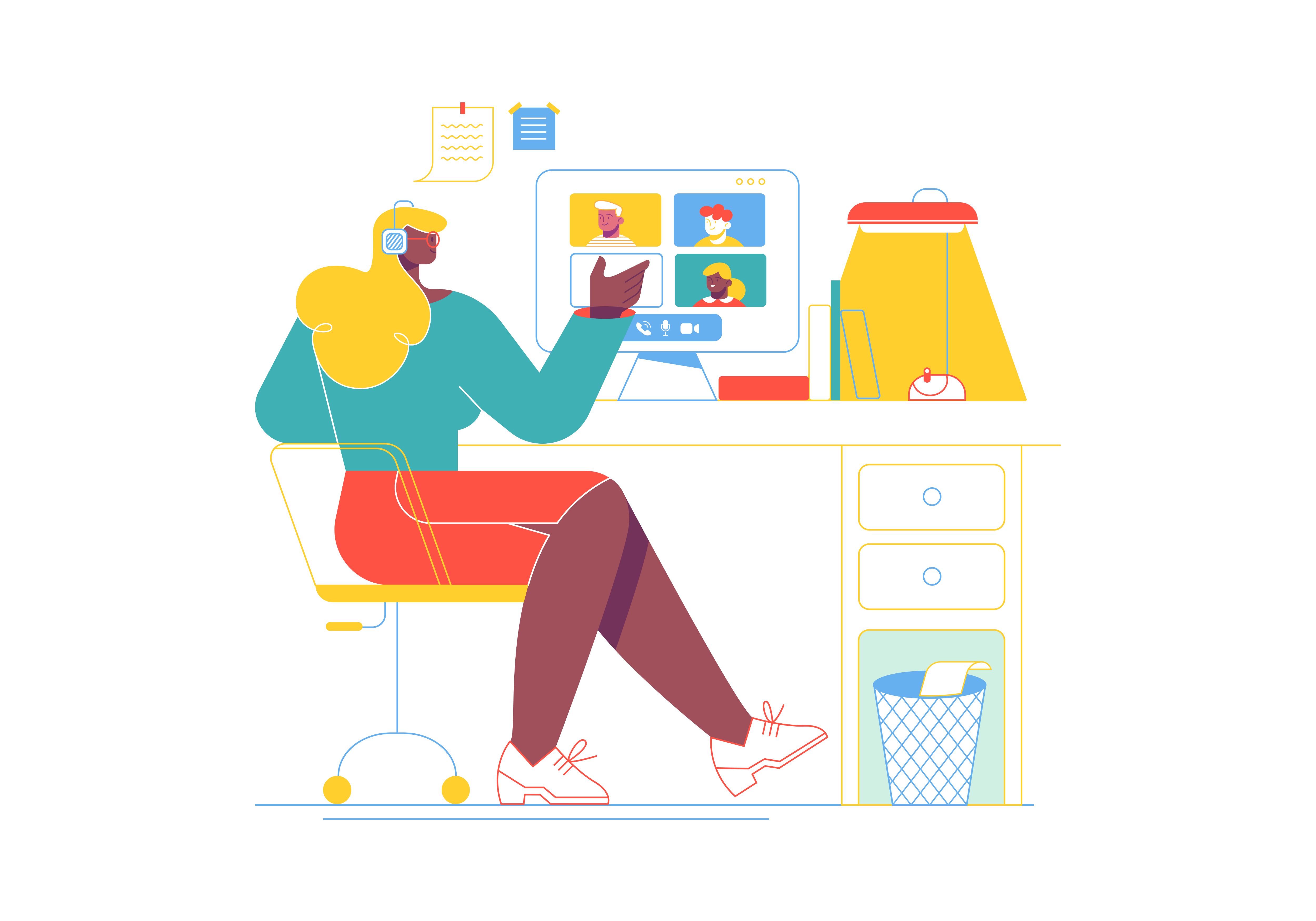
Tailwind CSS is a utility-first CSS framework that allows you to write more concise and maintainable stylesheets. If you’re building a web application using a JavaScript framework like React or Vue.js, you may be wondering how to integrate Tailwind CSS into your project. In this article, we’ll go over the steps to integrate Tailwind CSS with both React and Vue.js.
React Integration
To integrate Tailwind CSS with React, you can use the tailwindcss-react
package. This package provides a set of components that wrap your React components and apply the appropriate styles based on the classes defined in your Tailwind CSS configuration file.
First, install the tailwindcss-react
package by running the following command:
npm install tailwindcss-react
Next, create a new file called tailwind.config.js
in the root of your project and define your Tailwind CSS configuration. For example:
module.exports = {
mode: 'jit',
purge: [
'./src/**/*.{js,jsx,ts,tsx}',
'./public/index.html',
],
theme: {
extend: {},
},
variants: {},
plugins: [],
};
This configuration file tells Tailwind CSS to use the “jit” mode, which compiles your styles only when they’re needed, and to purge any unused styles from your application. It also defines a custom theme with no variants or plugins.
Finally, in your React component, you can import the tailwindcss-react
component like this:
import tailwindcss from 'tailwindcss-react';
And then use it to wrap your React components like this:
function MyComponent() {
return (
<tailwindcss>
<div className="container mx-auto p-4">
<h1 className="text-3xl font-bold mb-4">Welcome to my app!</h1>
<p className="text-xl text-gray-700">This is a paragraph of text.</p>
</div>
</tailwindcss>
);
}
In this example, the tailwindcss
component wraps the div
element with the classes container
, mx-auto
, p-4
, and text-3xl
. The tailwindcss
component also applies any other styles defined in your Tailwind CSS configuration file.
Vue.js Integration
To integrate Tailwind CSS with Vue.js, you can use the tailwindcss-vue
package. This package provides a set of directives that allow you to apply Tailwind CSS classes to your Vue components.
First, install the tailwindcss-vue
package by running the following command:
npm install tailwindcss-vue
Next, create a new file called tailwind.config.js
in the root of your project and define your Tailwind CSS configuration. For example:
module.exports = {
mode: 'jit',
purge: [
'./src/**/*.{vue}'],
},
theme: {
extend: {},
},
variants: {},
plugins: [],
};
This configuration file tells Tailwind CSS to use the “jit” mode, which compiles your styles only when they’re needed, and to purge any unused styles from your application. It also defines a custom theme with no variants or plugins.
Finally, in your Vue component, you can use the tailwind
directive to apply Tailwind CSS classes to your components like this:
<template>
<div :class="{ 'container': true, 'mx-auto': true, 'p-4': true }">
<h1 class="text-3xl font-bold mb-4">Welcome to my app!</h1>
<p class="text-xl text-gray-700">This is a paragraph of text.</p>
</div>
</template>
<script>
import { tailwind } from 'tailwindcss-vue';
export default {
setup() {
return {
tailwind,
};
},
};
</script>
In this example, the tailwind
directive applies the classes container
, mx-auto
, and p-4
to the div
element. The tailwind
directive also allows you to use any other classes defined in your Tailwind CSS configuration file.
Conclusion
Integrating Tailwind CSS with a JavaScript framework like React or Vue.js can be a great way to simplify your styling and improve the maintainability of your application. By using the tailwindcss-react
package for React and the tailwindcss-vue
package for Vue.js, you can easily apply Tailwind CSS styles to your components without having to write custom CSS. Just remember to define your Tailwind CSS configuration file and import the appropriate component into your application.