How do I install Tailwind CSS in my project?
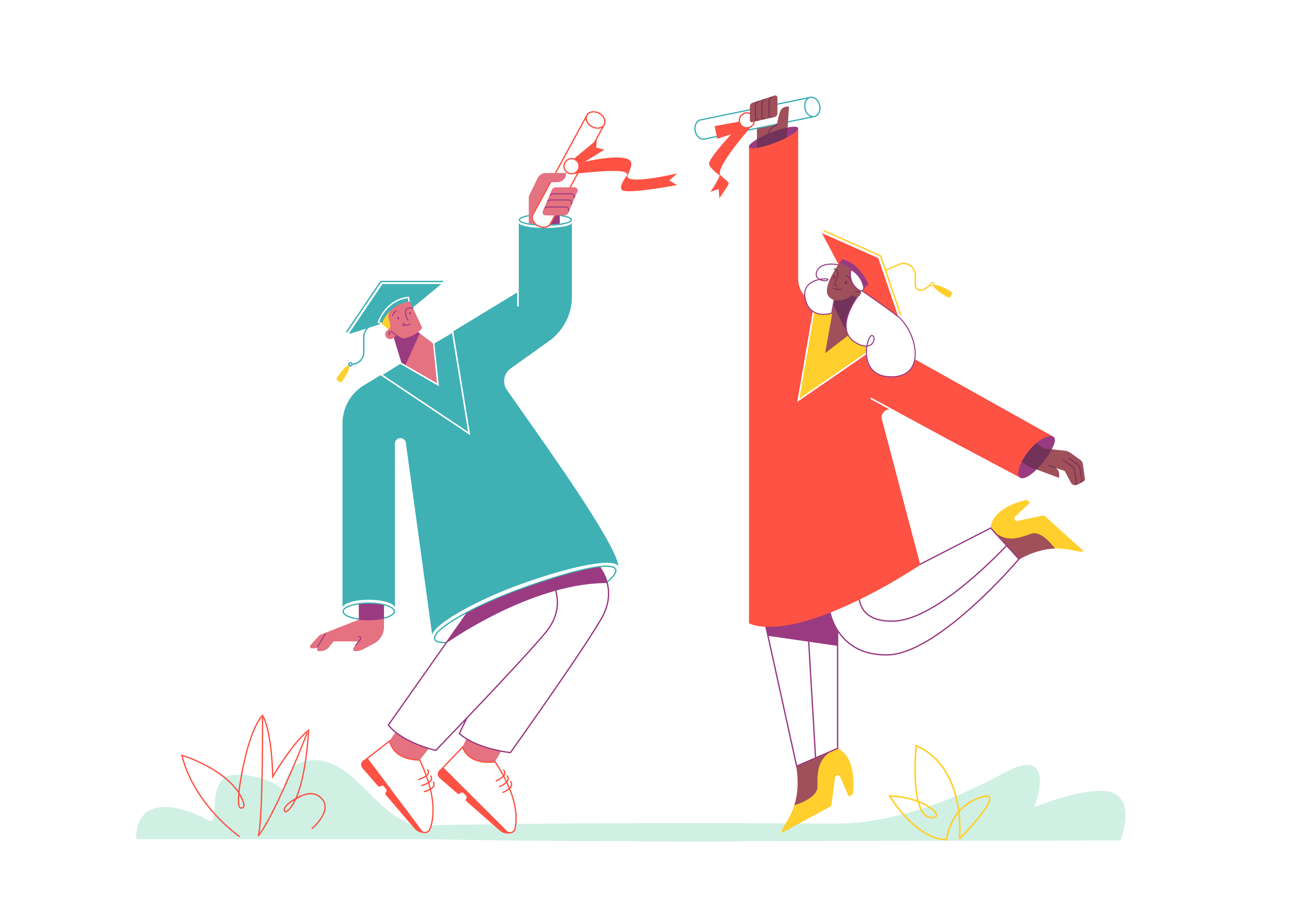
The article should contain the following points:
Step 1: Install Node.js
- Go to official Node.js website and download the appropriate version for your operating system.
- Follow the installation instructions for your operating system.
Step 2: Install npm (Node Package Manager)
- Open your terminal or command prompt and type
npm --version
. - If npm is not installed, you will see an error message. Otherwise, you can proceed to the next step.
Step 3: Install Tailwind CSS using npm
- Open your terminal or command prompt and type
npm install tailwindcss
. - Wait for the installation to complete.
Step 4: Create a configuration file
- Create a new file called
tailwind.config.js
in the root directory of your project. - Add the following code to the file:
module.exports = {
mode: 'jit',
purge: [
'./vendor/laravel/framework/src/Illuminate/Foundation/resources/views/**/*.blade.php',
'./vendor/tailwindcss/base-components/**/*.vue',
'./vendor/tailwindcss/utilities/**/*.vue'
],
theme: {
extend: {},
},
variants: {
extend: {},
},
plugins: [],
}
Step 5: Update your HTML file to use Tailwind CSS classes
- Open your HTML file and replace any previous CSS classes with Tailwind CSS classes.
- For example, instead of using
style="background-color: #f2f2f2;"
you can usebg-gray-100
.
Step 6: Run your application
- Open your command prompt or terminal and type
npm run watch
to start the development server. - Your application should now be using Tailwind CSS classes.
Note: This is just a basic example, you can customize the configuration file to fit your needs. Also, this guide assumes that you are using Laravel as your web framework..