How do I set up a build process for my Tailwind CSS project?
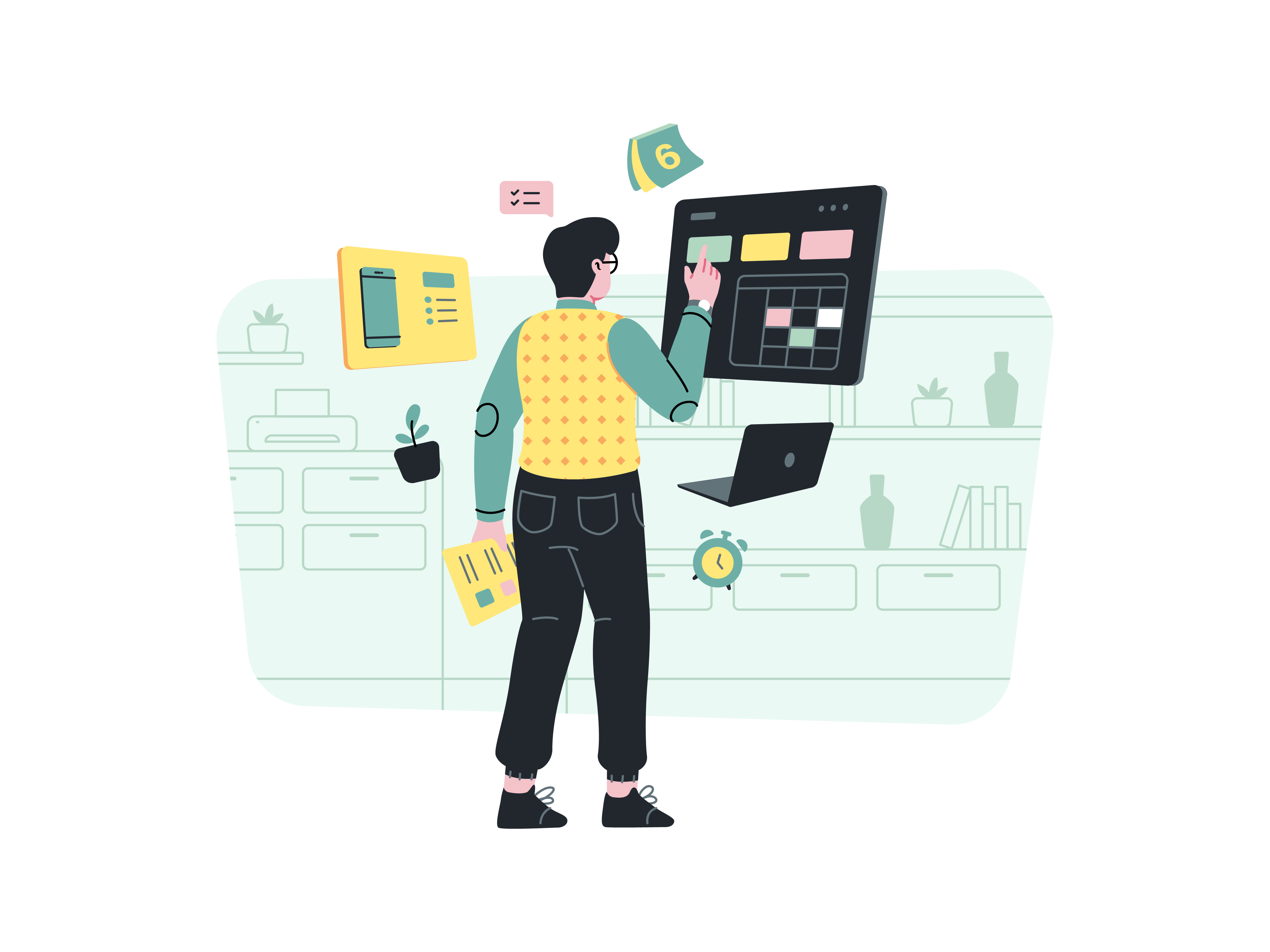
As a frontend developer, you know the importance of having a well-organized and efficient build process for your projects. This is especially true when working with a powerful CSS framework like Tailwind CSS. In this article, we’ll explore how to set up a build process for your Tailwind CSS project using popular tools like Webpack and Gulp.
Why do I need a build process?
Before we dive into the specifics of setting up a build process, let’s talk about why you might need one in the first place. A build process helps you automate the process of compiling your code into a production-ready format, which can save you time and reduce errors. This is especially important when working with a complex framework like Tailwind CSS, which requires a lot of configuration to get up and running properly.
Using Webpack
One popular tool for setting up a build process is Webpack. Webpack is a module bundler that can help you manage your code and dependencies, as well as optimize your code for production. To set up a build process with Webpack, follow these steps:
-
Install Webpack: You can install Webpack using npm by running the following command in your terminal:
npm install webpack
. -
Create a configuration file: Next, you’ll need to create a configuration file for Webpack. This file will tell Webpack how to compile and bundle your code. You can create a file called
webpack.config.js
in the root of your project. -
Add your code: In this file, you’ll need to add your code and dependencies. Here’s an example configuration for a Tailwind CSS project:
module.exports = {
entry: './src/app.js',
output: {
path: './dist',
filename: 'app.js'
},
resolve: {
extensions: ['.js', '.css']
},
module: {
rules: [
{
test: /\.js$/,
exclude: /node_modules/,
use: {
loader: 'babel-loader'
}
},
{
test: /\.css$/,
use: [
{
loader: 'style-loader'
},
{
loader: 'css-loader'
},
{
loader: 'postcss-loader'
}
]
}
]
},
plugins: [
new webpack.optimize.UglifyJsPlugin({
sourceMap: true
})
]
};
This configuration tells Webpack to look for your entry point in the src/app.js
file, compile your JavaScript code using Babel, and bundle your CSS code using PostCSS. It also includes an optimization plugin to minify your code for production.
Using Gulp
Another popular tool for setting up a build process is Gulp. Gulp is a task runner that can help you automate a wide range of tasks, including building and deploying your project. To set up a build process with Gulp, follow these steps:
-
Install Gulp: You can install Gulp using npm by running the following command in your terminal:
npm install gulp
. -
Create a configuration file: Next, you’ll need to create a configuration file for Gulp. This file will tell Gulp how to build and deploy your project. You can create a file called
gulpfile.js
in the root of your project. -
Add your tasks: In this file, you’ll need to add your tasks. Here’s an example configuration for a Tailwind CSS project:
var gulp = require('gulp');
var concat = require('gulp-concat');
var uglify = require('gulp-uglify');
var postcss = require('gulp-postcss');
gulp.task('default', ['build']);
gulp.task('build', [
'styles',
'scripts'
]);
function styles() {
return gulp.src(['src/app.css'])
.pipe(concat('app.css'))
.pipe(postcss())
.pipe(gulp.dest('dist'));
}
function scripts() {
return gulp.src(['src/app.js'])
.pipe(concat('app.js'))
.pipe(uglify({
sourceMap: true
}))
.pipe(gulp.dest('dist'));
}
This configuration tells Gulp to run two tasks by default: build
and default
. The build
task compiles your CSS and JavaScript code, while the default
task simply runs the build
task. The styles
and scripts
functions define how Gulp should compile your code.
Conclusion
Setting up a build process for your Tailwind CSS project can help you automate the compilation of your code and reduce errors. There are many tools available to help you set up this process, including Webpack and Gulp. By following the steps outlined in this article, you can create a robust build process that will help you manage your project as it grows and evolves over time..