How do I create a custom plugin in Tailwind CSS?
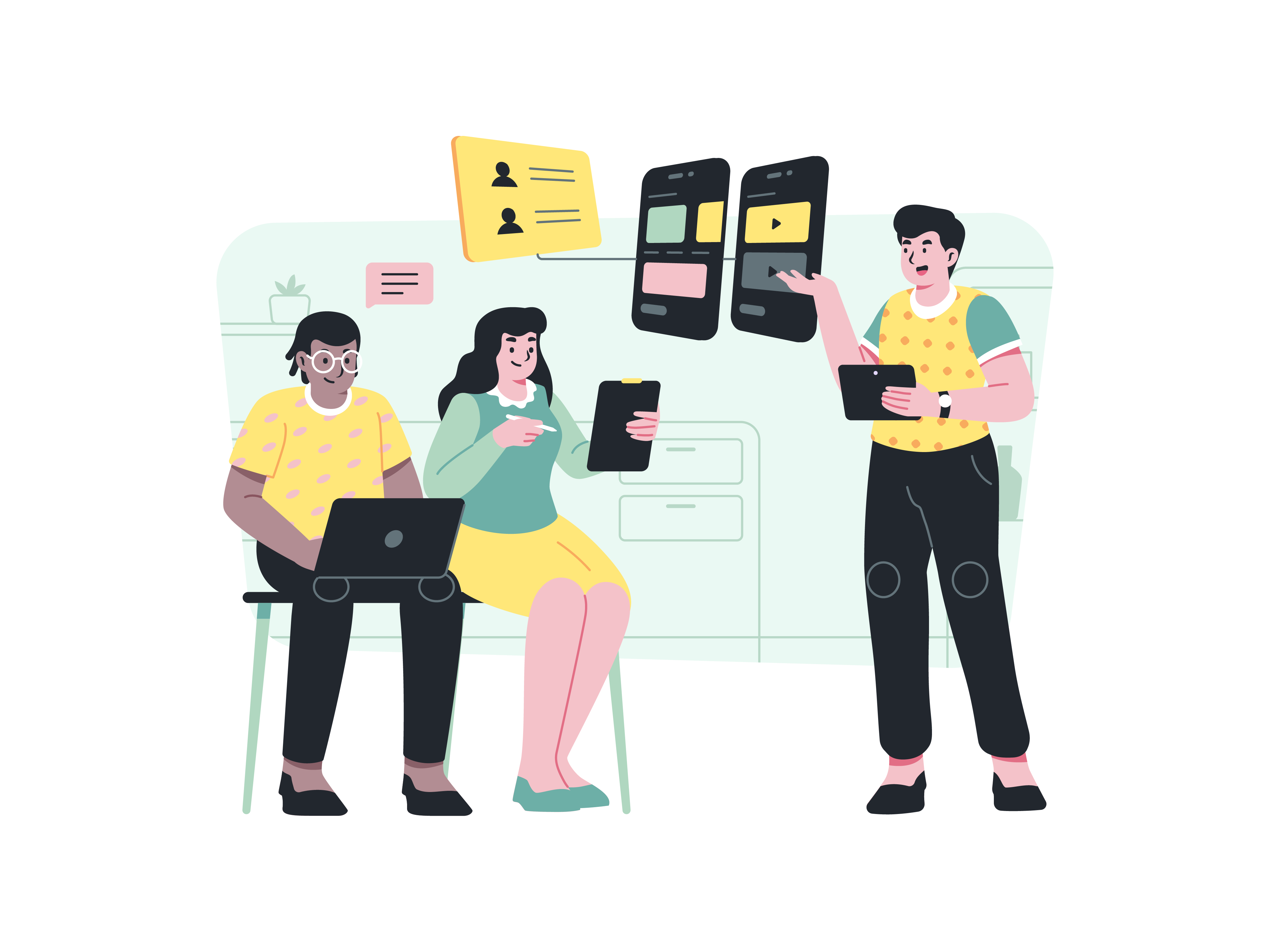
Here is a sample of what you should follow:
Creating Custom Plugins in Tailwind CSS
Are you tired of using the same old plugins in your Tailwind CSS projects? Do you want to create your own custom plugins to make your development workflow more efficient? Look no further! In this article, we will go over how to create a custom plugin in Tailwind CSS.
Step 1: Create a new folder for your plugin
First things first, we need to create a new folder for our plugin. This folder will contain all the files and code for our plugin. To create a new folder, open your terminal or command prompt and run the following command:
mkdir my-tailwind-plugin
This will create a new folder called my-tailwind-plugin
.
Step 2: Create a new JavaScript file for your plugin
Next, we need to create a new JavaScript file for our plugin. This file will contain the code for our plugin. To create a new JavaScript file, open your text editor and navigate to the my-tailwind-plugin
folder. Then, run the following command:
touch my-tailwind-plugin.js
This will create a new JavaScript file called my-tailwind-plugin.js
.
Step 3: Write the code for your plugin
Now it’s time to write the code for our plugin! Open up the my-tailwind-plugin.js
file and add the following code:
// my-tailwind-plugin.js
export class MyPlugin {
constructor(tailwindcss) {
this.tailwindcss = tailwindcss;
}
configure(configuration) {
this.configuration = configuration;
}
init() {
this.addRule('my-plugin-rule', 'your custom CSS rule here');
}
}
This code defines a new class called MyPlugin
that will be used to create our custom plugin. The configure
method is where we will define the configuration for our plugin, and the init
method is where we will add our custom CSS rule.
Step 4: Add your plugin to Tailwind CSS
Finally, we need to add our plugin to Tailwind CSS. To do this, open up your tailwindcss.config.js
file and add the following code at the end:
// tailwindcss.config.js
module.exports = {
plugins: [
require('my-tailwind-plugin'),
],
}
This code tells Tailwind CSS to load our custom plugin when it is initialized.
Using your custom plugin in your project
Now that we have created our custom plugin, we can use it in our Tailwind CSS project! Simply include the my-tailwind-plugin
folder in your project’s styles
directory, and you should be able to use the custom rule we defined in our plugin. For example:
// styles/main.css
@apply my-plugin-rule;
This will apply the custom CSS rule we defined in our plugin to our project.
Conclusion
Creating a custom plugin in Tailwind CSS is a great way to extend the functionality of this powerful CSS framework. By following the steps outlined in this article, you can create your own custom plugins to make your development workflow more efficient and effective. Happy coding!