How do you create a real-time chat application with Svelte
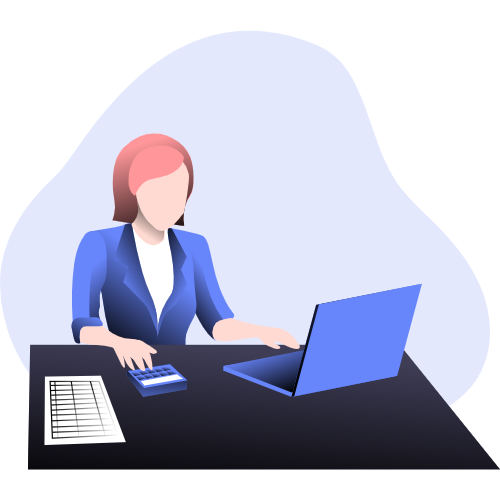
Real-time chat applications are essential for modern web experiences, enabling instant communication between users. In this tutorial, we’ll leverage the simplicity and efficiency of Svelte on the frontend and integrate it with a WebSocket-based backend to create a real-time chat application from scratch.
Prerequisites
Before we begin, make sure you have the following set up:
- Basic understanding of Svelte and JavaScript
- Node.js and npm installed on your machine
- A code editor of your choice (e.g., VS Code)
Backend Setup with WebSocket
Step 1: Setting Up the Backend Server
We’ll use Node.js with ws
(WebSocket) library to create a simple WebSocket server.
-
Initialize a new Node.js project:
mkdir chat-app-backend cd chat-app-backend npm init -y
-
Install
ws
library:npm install ws
-
Create
server.js
file for WebSocket server:// server.js const WebSocket = require('ws'); const wss = new WebSocket.Server({ port: 3030 }); wss.on('connection', function connection(ws) { ws.on('message', function incoming(message) { wss.clients.forEach(function each(client) { if (client !== ws && client.readyState === WebSocket.OPEN) { client.send(message); } }); }); }); console.log('WebSocket server running at ws://localhost:3030');
-
Start the WebSocket server:
node server.js
The WebSocket server is now running and listening for connections on ws://localhost:3030
.
Frontend Development with Svelte
Step 2: Creating the Svelte Chat Application
Now, let’s create the frontend part using Svelte to interact with our WebSocket server.
-
Initialize a new Svelte project:
npx degit sveltejs/template chat-app-frontend cd chat-app-frontend npm install
-
Update
src/App.svelte
with chat interface:<!-- src/App.svelte --> <script> import { onMount } from 'svelte'; import { writable } from 'svelte/store'; let message = ''; let messages = writable([]); let ws; onMount(() => { ws = new WebSocket('ws://localhost:3030'); ws.onmessage = function(event) { messages.update(prev => [...prev, event.data]); }; }); function sendMessage() { if (message.trim() !== '') { ws.send(message); message = ''; } } </script> <h1>Real-Time Chat</h1> <div> {#each $messages as msg (msg)} <p>{msg}</p> {/each} </div> <input type="text" bind:value={message} placeholder="Type your message..." /> <button on:click={sendMessage}>Send</button>
-
Start the Svelte development server:
npm run dev
-
Open the application in your browser:
Navigate to
http://localhost:5000
to see the chat interface.
Enhancing the Chat Application
Adding User Authentication
Implement user authentication using JWT tokens or session cookies to secure chat interactions.
Storing Chat History
Integrate a database (e.g., MongoDB, PostgreSQL) on the backend to store and retrieve chat history.
Implementing Private Messaging
Extend the application to support private messaging between users.
Conclusion
Congratulations! You’ve successfully built a real-time chat application with Svelte and WebSocket. This tutorial covered setting up a WebSocket server with Node.js, creating a basic Svelte frontend for real-time messaging, and potential enhancements to expand functionality. Experiment further by adding features like authentication, message encryption, or integrating with other services. Real-time applications like chat are foundational in web development, showcasing the power and versatility of Svelte and WebSocket technology.
How can you implement custom meta tags for SEO in a Next.js application
Explain the concept of API routes in Next.js
How does React handle code splitting with React.lazy and Suspense
How can you optimize CSS delivery in a Next.js project
How can you implement custom error handling in Next.js
How do you use TypeScript with Svelte