How do you use Svelte with Storybook
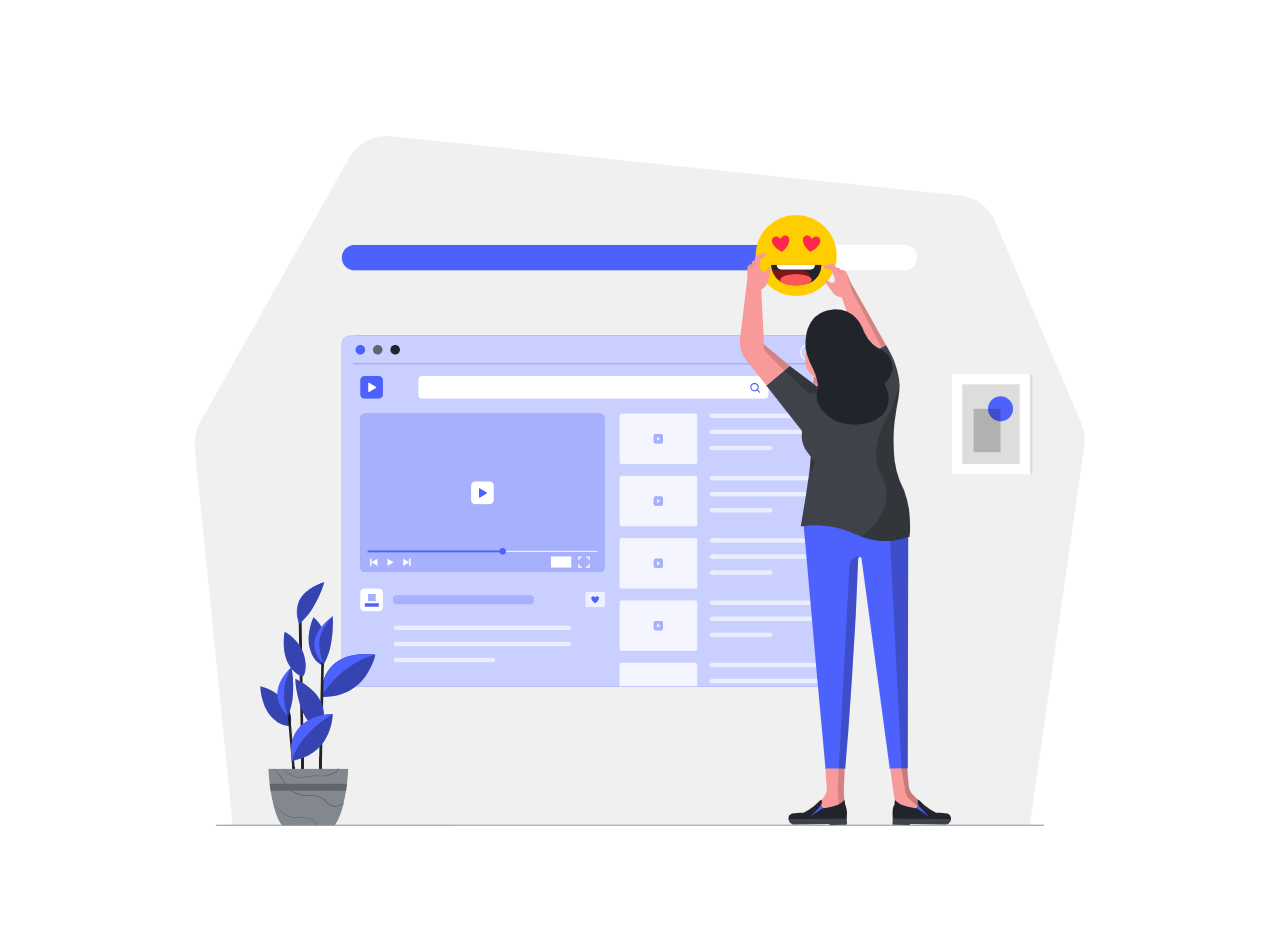
Storybook is a popular tool for developing and testing user interface components in isolation. It provides a sandbox environment where you can build and showcase components independently of your application. Integrating Storybook with Svelte allows you to leverage these benefits within the Svelte ecosystem, enabling you to create, test, and document your Svelte components more effectively. This article will guide you through setting up Storybook with Svelte and using it to develop and showcase your Svelte components.
Introduction to Storybook
What is Storybook?
Storybook is an open-source tool for developing UI components in isolation. It enables you to build, test, and document components separately from your application, providing a focused environment for component development. Key features of Storybook include:
- Component Isolation: Develop and test components without the need for a full application context.
- Interactive UI: View and interact with your components in a live environment.
- Documentation: Automatically generate and maintain documentation for your components.
- Add-ons: Extend Storybook’s functionality with a rich ecosystem of add-ons for testing, accessibility, and more.
Setting Up Storybook with Svelte
Prerequisites
Before setting up Storybook with Svelte, ensure you have the following prerequisites:
- Node.js and npm (or yarn) installed
- A Svelte project set up
If you don’t have a Svelte project yet, you can create one using the following command:
npx degit sveltejs/template svelte-storybook
cd svelte-storybook
npm install
Installing Storybook
To add Storybook to your Svelte project, follow these steps:
-
Install Storybook CLI
First, install the Storybook CLI globally if you haven’t already:
npm install -g @storybook/cli
-
Initialize Storybook
Navigate to your Svelte project directory and initialize Storybook:
npx sb init
This command will set up Storybook and create a
.storybook
directory in your project. -
Install Svelte Add-ons
You’ll need the
@storybook/svelte
package to integrate Storybook with Svelte:npm install --save-dev @storybook/svelte
Additionally, you may want to install the
svelte
andsvelte-loader
packages:npm install --save-dev svelte svelte-loader
-
Configure Storybook
Update your Storybook configuration to work with Svelte. In the
.storybook
directory, modifymain.js
to include the Svelte configuration:// .storybook/main.js module.exports = { stories: ['../src/**/*.stories.svelte'], addons: ['@storybook/addon-links', '@storybook/addon-essentials'], framework: '@storybook/svelte', };
Create a
preview.js
file in the.storybook
directory to configure Storybook’s preview settings:// .storybook/preview.js import '../src/styles/global.css'; // Import global styles if any export const parameters = { actions: { argTypesRegex: "^on[A-Z].*" }, controls: { expanded: true }, };
Creating Your First Story
With Storybook set up, you can now create your first story. A story represents a single state of a component. Here’s how to create a basic story for a Svelte component:
-
Create a Svelte Component
Let’s create a simple button component. Create a file
Button.svelte
in thesrc
directory:<!-- src/Button.svelte --> <script> export let label = 'Click me'; </script> <button>{label}</button>
-
Create a Story for the Component
Next, create a story file for the Button component. Create a file
Button.stories.svelte
in thesrc
directory:<!-- src/Button.stories.svelte --> <script> import Button from './Button.svelte'; </script> <h2>Button</h2> <Button label="Default Button" /> <Button label="Primary Button" style="background-color: blue; color: white;" />
In this story, we import the
Button
component and render it with different props to showcase its different states. -
Run Storybook
Start the Storybook development server to view your stories:
npm run storybook
Open your browser and navigate to
http://localhost:6006
to see your Svelte component in Storybook.
Advanced Usage
Using Add-ons
Storybook has a rich ecosystem of add-ons that can enhance your development experience. Here are some popular add-ons and how to use them with Svelte:
-
@storybook/addon-actions
The
actions
add-on helps you monitor the actions your components are performing. Install it with:npm install --save-dev @storybook/addon-actions
Update your
.storybook/main.js
to include theactions
add-on:// .storybook/main.js module.exports = { stories: ['../src/**/*.stories.svelte'], addons: ['@storybook/addon-links', '@storybook/addon-essentials', '@storybook/addon-actions'], framework: '@storybook/svelte', };
Use the
action
function in your stories to log actions:<!-- src/Button.stories.svelte --> <script> import { action } from '@storybook/addon-actions'; import Button from './Button.svelte'; </script> <h2>Button</h2> <Button on:click={action('button-click')} label="Click me" />
-
@storybook/addon-knobs
The
knobs
add-on allows you to dynamically edit props of your components in the Storybook UI. Install it with:npm install --save-dev @storybook/addon-knobs
Update your
.storybook/main.js
to include theknobs
add-on:// .storybook/main.js module.exports = { stories: ['../src/**/*.stories.svelte'], addons: ['@storybook/addon-links', '@storybook/addon-essentials', '@storybook/addon-knobs'], framework: '@storybook/svelte', };
Use the
withKnobs
decorator in your stories to add interactive knobs:<!-- src/Button.stories.svelte --> <script> import { withKnobs, text } from '@storybook/addon-knobs'; import Button from './Button.svelte'; </script> export default { title: 'Button', decorators: [withKnobs], }; export const Default = () => ({ Component: Button, props: { label: text('Label', 'Click me'), }, });
Writing Stories in Different Formats
Storybook supports various formats for writing stories, including:
-
Component Story Format (CSF)
CSF is a popular format for writing stories, where each story is exported as a named export from a
.stories.js
or.stories.svelte
file.// src/Button.stories.js import Button from './Button.svelte'; export default { title: 'Button', component: Button, }; export const Default = () => ({ Component: Button, props: { label: 'Click me', }, }); export const Primary = () => ({ Component: Button, props: { label: 'Primary Button', }, styles: { backgroundColor: 'blue', color: 'white', }, });
-
Storybook Docs
Storybook Docs provides a way to write documentation for your components alongside your stories. Install the
@storybook/addon-docs
package:npm install --save-dev @storybook/addon-docs
Update your
.storybook/main.js
to include thedocs
add-on:// .storybook/main.js module.exports = { stories: ['../src/**/*.stories.svelte'], addons: ['@storybook/addon-links', '@storybook/addon-essentials', '@storybook/addon-docs'], framework: '@storybook/svelte', };
Write documentation in your story files using MDX (Markdown for JSX):
<!-- src/Button.stories.mdx --> import { Meta, Story, Preview } from '@storybook/addon-docs'; import Button from './Button.svelte'; <Meta title="Button" component={Button} /> # Button Component The `Button` component is used to render a button. <Preview> <Story name="Default"> <Button label="Click me" /> </Story> <Story name="Primary"> <Button label="Primary Button" style="background-color: blue; color: white;" /> </Story> </Preview>
Best Practices
-
Keep Stories Focused
Write stories that focus on a single aspect or state of a component. This makes it easier to test and understand each component’s behavior.
-
**Use Knobs for Dynamic
Props**
Use the knobs
add-on to allow dynamic editing of component props. This can help you test different scenarios without modifying your story files.
-
Document Your Components
Take advantage of Storybook Docs to provide detailed documentation for your components. This helps other developers understand how to use and integrate them.
-
Organize Your Stories
Organize your stories in a logical structure, such as grouping related components together. This makes it easier to navigate and maintain your Storybook.
-
Test Component Interactions
Use add-ons like
actions
to test and monitor interactions between components. This helps ensure your components work as expected in different scenarios.
Conclusion
Integrating Storybook with Svelte provides a powerful environment for developing, testing, and documenting your components. By setting up Storybook and using its features, you can create high-quality Svelte components, test their behavior in isolation, and generate comprehensive documentation.
With the ability to render components in isolation, test interactions, and document usage, Storybook enhances your development workflow and improves the overall quality of your UI components. By following the steps outlined in this article, you can effectively use Storybook with Svelte to build and showcase your components with confidence.
Where is state stored in React