How to create a carousel/slider with Bootstrap
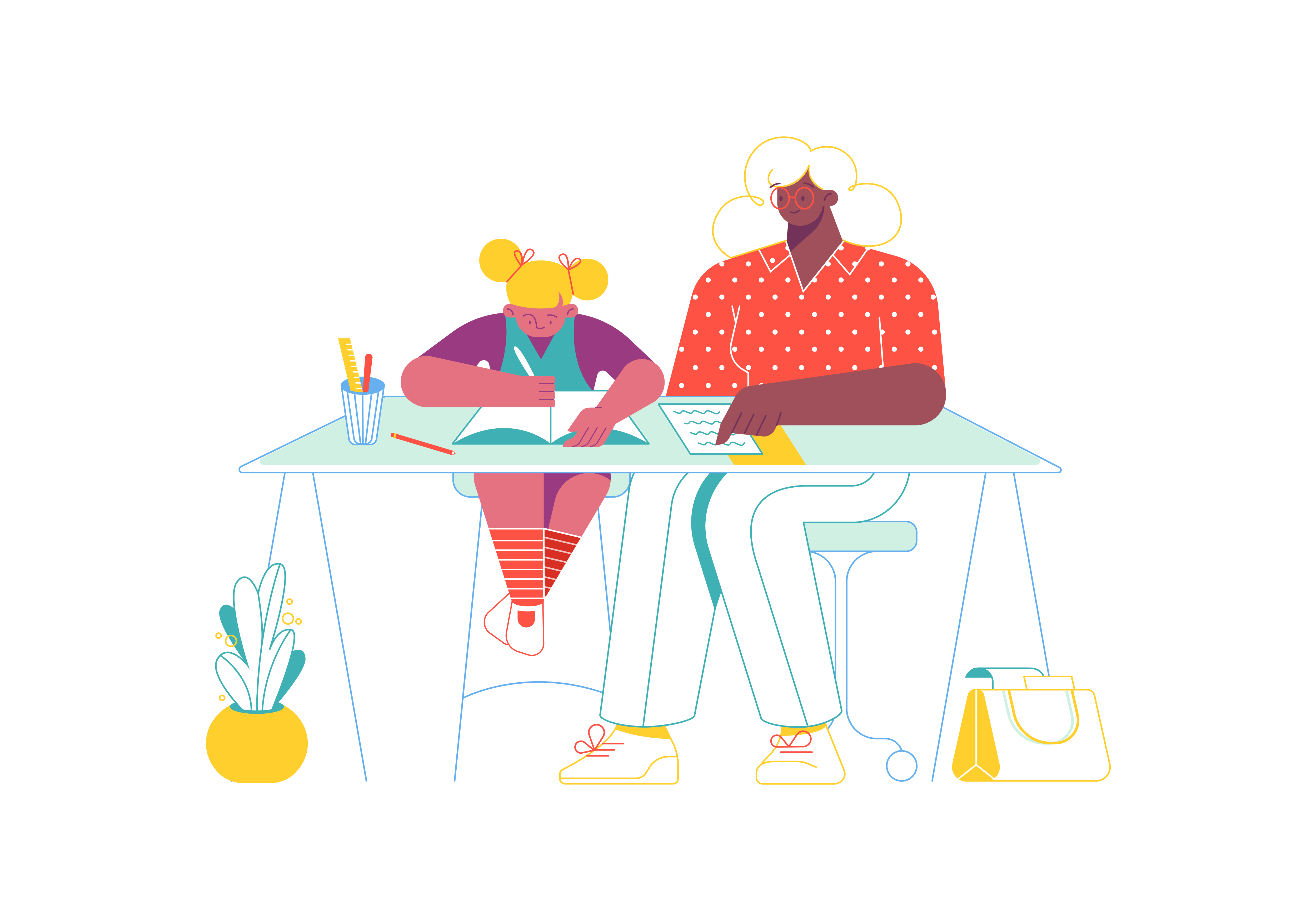
If you’re aiming to showcase multiple images or pieces of content in an interactive and visually appealing manner on your website, a carousel (also known as a slider) can be the perfect solution. A carousel allows you to display a series of items that automatically cycle through, providing an engaging experience for your users. In this blog post, we’ll explore how to create a carousel/slider using the popular front-end framework, Bootstrap.
What is Bootstrap
Before we dive into creating a carousel/slider, let’s briefly revisit Bootstrap for those who might be new to it. Bootstrap is an open-source front-end framework developed by Twitter. It offers a collection of pre-designed HTML, CSS, and JavaScript components that make web development faster and easier. Bootstrap is renowned for its responsive design, cross-browser compatibility, and extensive set of tools.
Getting Started with Bootstrap
To create a carousel/slider with Bootstrap, you need to have Bootstrap integrated into your project. You can choose to either download Bootstrap and host it locally or use a Content Delivery Network (CDN). Here’s a simple example of including Bootstrap via CDN:
<!DOCTYPE html>
<html>
<head>
<!-- Add Bootstrap CSS via CDN -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
<title>Bootstrap Carousel Example</title>
</head>
<body>
<!-- Your content goes here -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
With Bootstrap included, you’re now ready to start building your carousel/slider.
Creating a Basic Bootstrap Carousel
Creating a basic carousel with Bootstrap is straightforward. The core component you’ll use is the carousel
class, along with a few additional elements for each carousel item.
Here’s a step-by-step guide on creating a basic Bootstrap carousel:
- Set Up the Structure: Start by creating a
<div>
element with thecarousel
class. Inside this div, add another<div>
element with thecarousel-inner
class. This inner div will hold the individual carousel items.
<div id="myCarousel" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<!-- Carousel items will go here -->
</div>
</div>
- Add Carousel Items: Within the
carousel-inner
div, add individual carousel items. Each item should have thecarousel-item
class. You’ll typically want to include an<img>
element for images or a container for content.
<div id="myCarousel" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img src="image1.jpg" alt="Image 1">
</div>
<div class="carousel-item">
<img src="image2.jpg" alt="Image 2">
</div>
<div class="carousel-item">
<img src="image3.jpg" alt="Image 3">
</div>
<!-- Add more items as needed -->
</div>
</div>
- Add Navigation Controls: To allow users to navigate through the carousel manually, you can add navigation controls, often in the form of left and right arrows. These controls use the
carousel-control-prev
andcarousel-control-next
classes.
<div id="myCarousel" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<!-- Carousel items go here -->
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#myCarousel" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#myCarousel" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
- Add Indicators (Optional): Bootstrap also provides indicators that show which carousel item is currently active. These indicators are created using the
carousel-indicators
class.
<div id="myCarousel" class="carousel slide" data-bs-ride="carousel">
<ol class="carousel-indicators">
<li data-bs-target="#myCarousel" data-bs-slide-to="0" class="active"></li>
<li data-bs-target="#myCarousel" data-bs-slide-to="1"></li>
<li data-bs-target="#myCarousel" data-bs-slide-to="2"></li>
<!-- Add more indicators as needed -->
</ol>
<div class="carousel-inner">
<!-- Carousel items go here -->
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#myCarousel" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
<span class="visually-hidden">Previous</span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#myCarousel" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
<span class="visually-hidden">Next</span>
</button>
</div>
Customizing Your Carousel
The example above gives you a basic carousel structure, but you can further customize it to match your website’s design and functionality.
Here are a few additional customization options:
- Auto-play: You can make the carousel auto-play by adding the
data-bs-interval
attribute to the main carousel div:
<div id="myCarousel" class="carousel slide" data-bs-ride="carousel" data-bs-interval="3000">
<!-- Carousel content -->
</div>
- Adding Captions: If you want to include captions for each carousel item, you can add a
<div>
with thecarousel-caption
class inside eachcarousel-item
.
<div class="carousel-inner">
<div class="carousel-item active">
<img src="image1.jpg" alt="Image 1">
<div class="carousel-caption">
<h3>Image 1 Caption</h3>
<p>This is the caption for image 1.</p>
</div>
</div>
<!-- Add more items with captions as needed -->
</div>
- Responsive Images: To ensure your images are responsive and adjust to different screen sizes, use the
img-fluid
class on your<img>
elements.
<div class="carousel-inner">
<div class="carousel-item active">
<img src="image1.jpg" alt="Image 1" class="img-fluid">
</div>
<!-- Add more items with responsive images as needed -->
</div>
Conclusion
A carousel/slider is an invaluable asset for captivatingly presenting images or content on your website. By harnessing the power of Bootstrap, you can effortlessly craft a responsive and visually appealing showcase, elevating your website’s user experience.