How to create a dropdown menu in Bootstrap
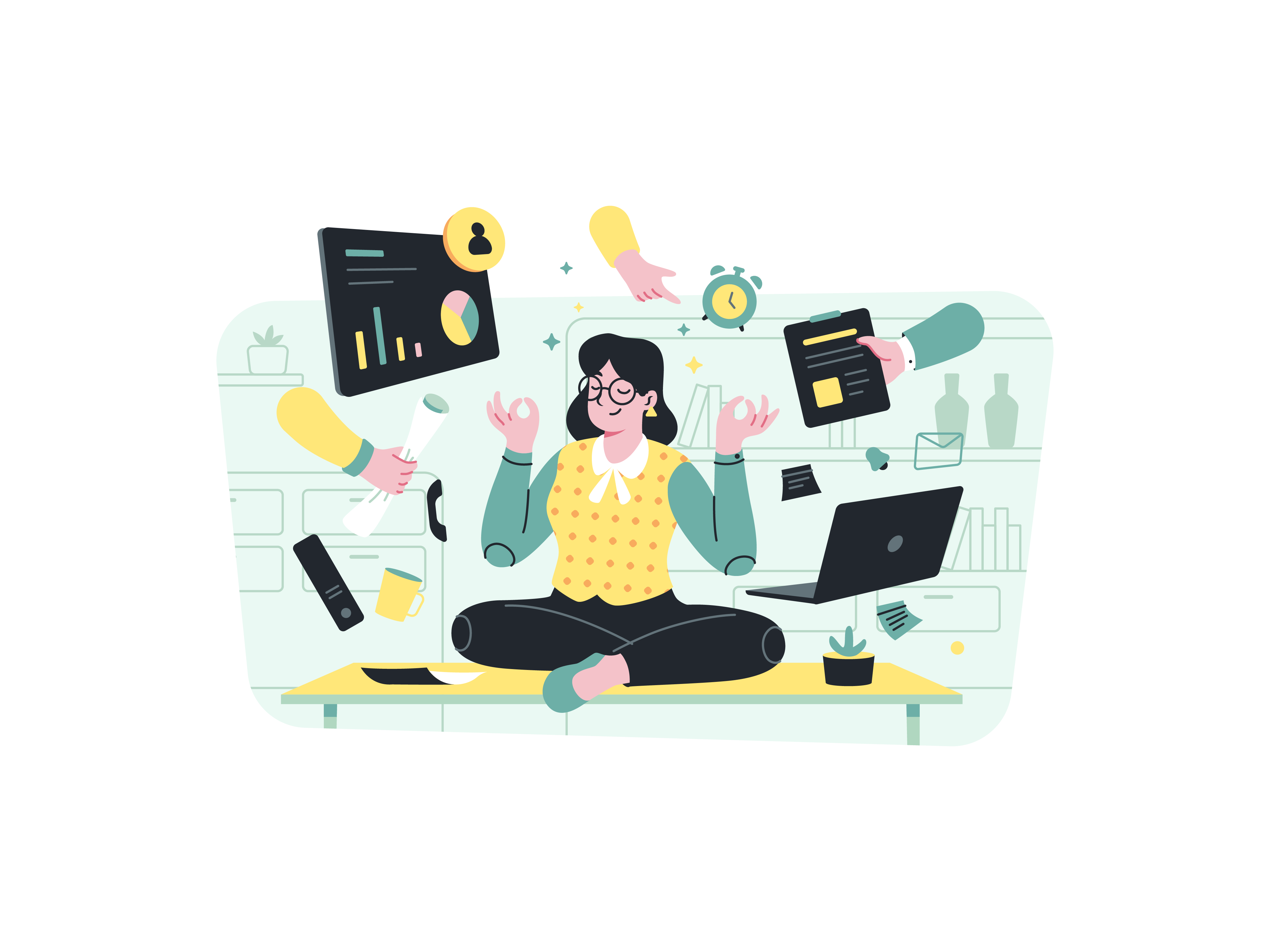
Dropdown menus are a staple in web design, offering a clean and organized way to present a variety of options to users. When it comes to building responsive and stylish dropdown menus, Bootstrap provides an excellent toolkit. In this blog post, we’ll dive deep into the world of dropdown menus in Bootstrap, exploring how to create them from scratch and customize them to suit your website’s needs.
The Power of Bootstrap Dropdowns
Before we delve into the technical aspects, let’s briefly discuss why Bootstrap’s dropdowns are such a game-changer. Bootstrap, a popular front-end framework, simplifies the creation of dynamic web components. Dropdown menus in Bootstrap are no exception. They not only provide a user-friendly way to present a list of options but also come with built-in responsive behavior, making them adaptable to various screen sizes. Bootstrap’s dropdowns blend seamlessly with your website’s design, ensuring a consistent look and feel.
Getting Started with Bootstrap Dropdowns
To start using Bootstrap’s dropdowns, make sure you have Bootstrap integrated into your project. You can choose to download Bootstrap and host it locally or use a Content Delivery Network (CDN) for quick inclusion. Here’s an example of including Bootstrap via CDN:
<!DOCTYPE html>
<html>
<head>
<!-- Include Bootstrap CSS via CDN -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
<title>Bootstrap Dropdown Menu</title>
</head>
<body>
<!-- Your content goes here -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
With Bootstrap in place, you’re ready to start creating dynamic dropdown menus.
Basic Bootstrap Dropdown Menu
Creating a basic dropdown menu in Bootstrap involves a few key components:
- Dropdown Trigger: This is the element (usually a button or a link) that, when clicked, reveals the dropdown menu.
- Dropdown Menu: This is the container that holds the list of options or links.
- Dropdown Items: These are the individual items within the dropdown menu.
Here’s an example of a basic Bootstrap dropdown menu:
<div class="dropdown">
<button class="btn btn-secondary dropdown-toggle" type="button" id="dropdownMenuButton" data-bs-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Dropdown Menu
</button>
<div class="dropdown-menu" aria-labelledby="dropdownMenuButton">
<a class="dropdown-item" href="#">Option 1</a>
<a class="dropdown-item" href="#">Option 2</a>
<a class="dropdown-item" href="#">Option 3</a>
</div>
</div>
In this example, we’ve used the dropdown
class to define the dropdown container. The trigger button has the btn
and dropdown-toggle
classes, indicating it’s a button that toggles the dropdown. The data-bs-toggle
attribute specifies that it’s a dropdown, and the data-bs-target
attribute points to the ID of the dropdown menu. The dropdown-menu
class defines the dropdown menu container, and the individual items within the menu have the dropdown-item
class.
Dropdown Variations and Customization
While the basic dropdown we just created is functional, Bootstrap offers a plethora of customization options and variations to make your dropdowns more engaging and visually appealing.
Dropdown Position
By default, Bootstrap’s dropdowns appear below the trigger element. However, you can control the placement by using the data-bs-placement
attribute. Common placement values are top
, bottom
, start
(left-aligned), and end
(right-aligned).
<div class="dropdown">
<button class="btn btn-secondary dropdown-toggle" type="button" id="dropdownMenuButton" data-bs-toggle="dropdown" data-bs-placement="top" aria-haspopup="true" aria-expanded="false">
Top Dropdown
</button>
<!-- Dropdown menu items -->
</div>
Split Dropdown Buttons
Bootstrap allows you to create split dropdown buttons, where the trigger button is separate from the dropdown toggle.
<div class="btn-group">
<button type="button" class="btn btn-primary">Action</button>
<button type="button" class="btn btn-primary dropdown-toggle dropdown-toggle-split" data-bs-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
<span class="visually-hidden">Toggle Dropdown</span>
</button>
<div class="dropdown-menu">
<!-- Dropdown menu items -->
</div>
</div>
Dropdown Headers and Dividers
You can add headers or dividers to your dropdown menu for better organization.
<div class="dropdown">
<button class="btn btn-secondary dropdown-toggle" type="button" id="dropdownMenuButton" data-bs-toggle="dropdown" aria-haspopup="true" aria-expanded="false">
Dropdown Menu
</button>
<div class="dropdown-menu" aria-labelledby="dropdownMenuButton">
<h6 class="dropdown-header">Header</h6>
<a class="dropdown-item" href="#">Option 1</a>
<a class="dropdown-item" href="#">Option 2</a>
<div class="dropdown-divider"></div>
<a class="dropdown-item" href="#">Option 3</a>
</div>
</div>
Customizing Dropdowns
Bootstrap allows you to fully customize the appearance of dropdowns using your CSS. You can change the colors, fonts, and other styles to match your website’s design.
Handling Dropdowns with JavaScript
In some cases, you might want to control the behavior of your dropdowns programmatically using JavaScript. Bootstrap provides a JavaScript API to handle this.
For example, you can use JavaScript to show or hide a dropdown programmatically:
var myDropdown = new bootstrap.Dropdown(document.querySelector('.dropdown-toggle'));
// To show the dropdown
myDropdown.show();
// To hide the dropdown
myDropdown.hide();
Conclusion
Dropdown menus are essential for creating intuitive and organized user interfaces, and Bootstrap’s built-in support makes them a breeze to implement. By following the basic structure and exploring the various customization options, you can create dynamic and visually pleasing dropdown menus that enhance user interaction on your website. Whether you need a simple list of options or a sophisticated dropdown with headers and dividers, Bootstrap has you covered. Elevate your website’s navigation with Bootstrap dropdowns and provide a seamless user experience for your visitors.