How to create a responsive login form with Bootstrap
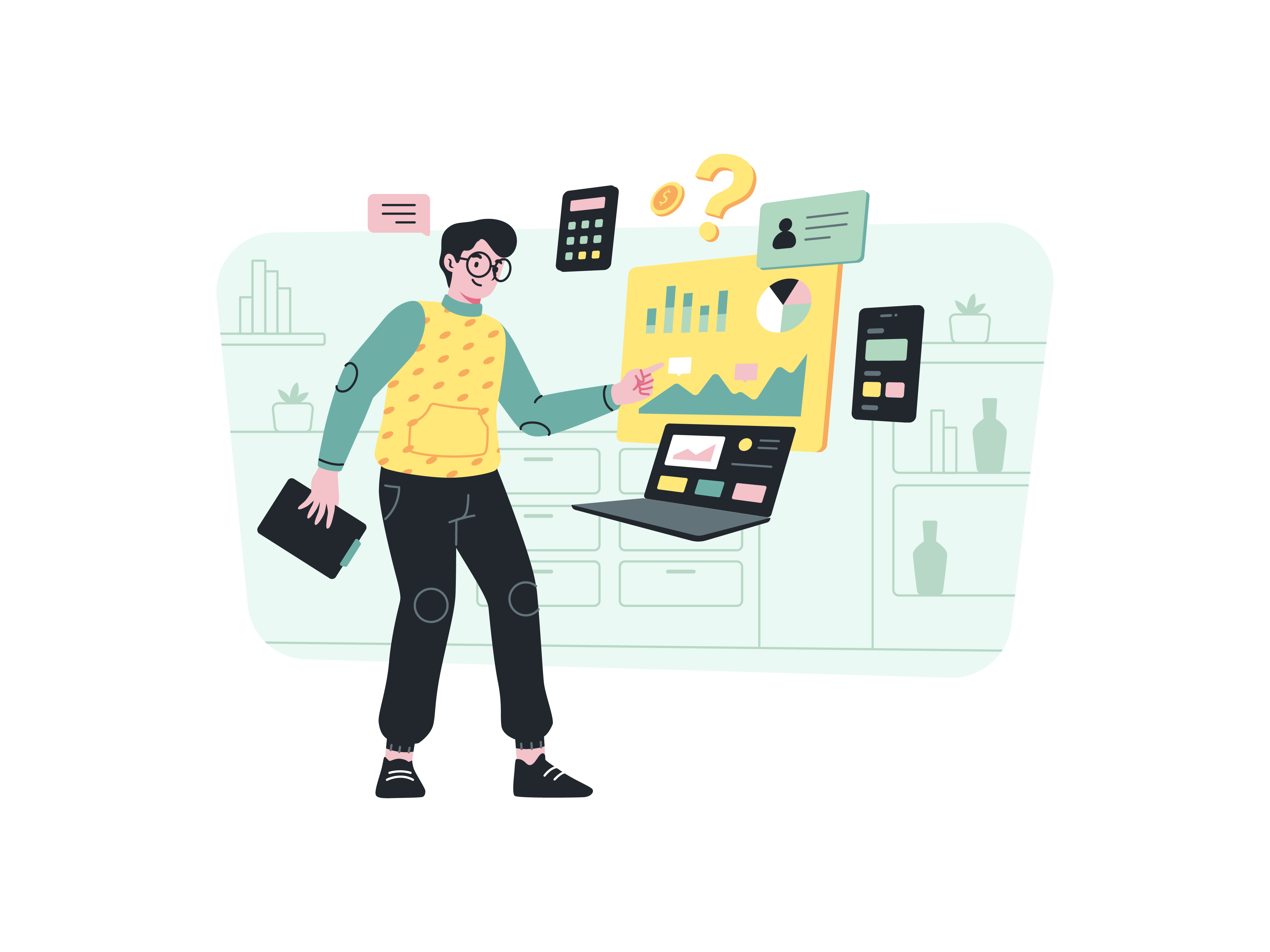
A well-designed login form is a crucial element of any web application or website that requires user authentication. It should not only be functional but also visually appealing and responsive to various screen sizes and devices. Bootstrap, a popular front-end framework, provides a set of tools and components that make it easy to create a responsive login form that looks great and works seamlessly on both desktop and mobile devices. In this comprehensive guide, we’ll walk you through the step-by-step process of creating a responsive login form using Bootstrap.
Understanding the Importance of Responsive Design
Before we start building our responsive login form with Bootstrap, let’s briefly discuss why responsive design is essential.
The Significance of Responsive Design
In today’s digital landscape, users access websites and web applications from a wide range of devices, including desktop computers, laptops, tablets, and smartphones. To provide a consistent and user-friendly experience across all these devices, responsive design is crucial.
A responsive design ensures that your login form (and your entire website) adapts to different screen sizes and orientations. This means that users can easily interact with your login form whether they’re on a large desktop monitor or a small mobile phone screen.
Now that we understand the importance of responsiveness, let’s start building our responsive login form.
Getting Started with Bootstrap
To create a responsive login form with Bootstrap, you need to have Bootstrap integrated into your project. You can include Bootstrap by downloading it and adding the necessary CSS and JavaScript files to your project, or you can use the Bootstrap CDN for a quicker setup.
Once you have Bootstrap in place, you’re ready to start building your responsive login form.
Building the HTML Structure
The HTML structure of your login form is crucial for creating a responsive design. Here’s a basic example:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<link rel="stylesheet" href="bootstrap.min.css">
<title>Responsive Login Form</title>
</head>
<body>
<div class="container">
<div class="row justify-content-center">
<div class="col-md-6">
<h2 class="text-center">Login</h2>
<form>
<div class="mb-3">
<label for="email" class="form-label">Email address</label>
<input type="email" class="form-control" id="email" placeholder="Enter your email">
</div>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control" id="password" placeholder="Enter your password">
</div>
<div class="text-center">
<button type="submit" class="btn btn-primary">Login</button>
</div>
</form>
</div>
</div>
</div>
<script src="bootstrap.min.js"></script>
</body>
</html>
In this structure:
- We include the necessary Bootstrap CSS and JavaScript files.
- The
container
androw
classes help create a responsive layout. - The
col-md-6
class ensures that the login form takes up half of the available width on medium-sized screens and above. - Form elements like
input
fields and the submitbutton
are styled using Bootstrap classes.
Adding Responsiveness with Bootstrap Classes
Bootstrap provides responsive classes that you can apply to your HTML elements to make them adapt to different screen sizes. In our login form, we’ve already used some of these classes like col-md-6
to control column width on medium-sized screens.
You can also use classes like form-control
to make input fields responsive. Bootstrap takes care of the styling and layout adjustments for you.
Customizing Your Responsive Login Form
While Bootstrap provides a solid foundation for creating a responsive login form, you can customize it to match your branding and design preferences. You can do this by:
- Custom CSS: Create custom CSS rules to modify the appearance of your form elements. You can change colors, fonts, and more to match your website’s style.
- Adding Icons: Use icon libraries like Font Awesome to add icons to your form fields for a modern look.
- Validation: Implement client-side and server-side validation to ensure that user input is accurate and secure.
- Error Handling: Create a user-friendly error-handling system to provide clear feedback to users in case of login errors.
- Accessibility: Ensure your login form is accessible to all users, including those with disabilities, by following accessibility best practices.
Testing and Optimization
Once your responsive login form is ready, thoroughly test it on various devices and screen sizes to ensure it works as expected. Make adjustments as needed to optimize the user experience.
Conclusion
Creating a responsive login form with Bootstrap is a practical and efficient way to ensure that users can log in from any device without encountering issues. By following the steps outlined in this guide and customizing your form to match your website’s design, you can provide a seamless and user-friendly login experience for your audience. Remember that responsive design is not just a trend; it’s a necessity in today’s multi-device world.