How to create a responsive timeline with Bootstrap
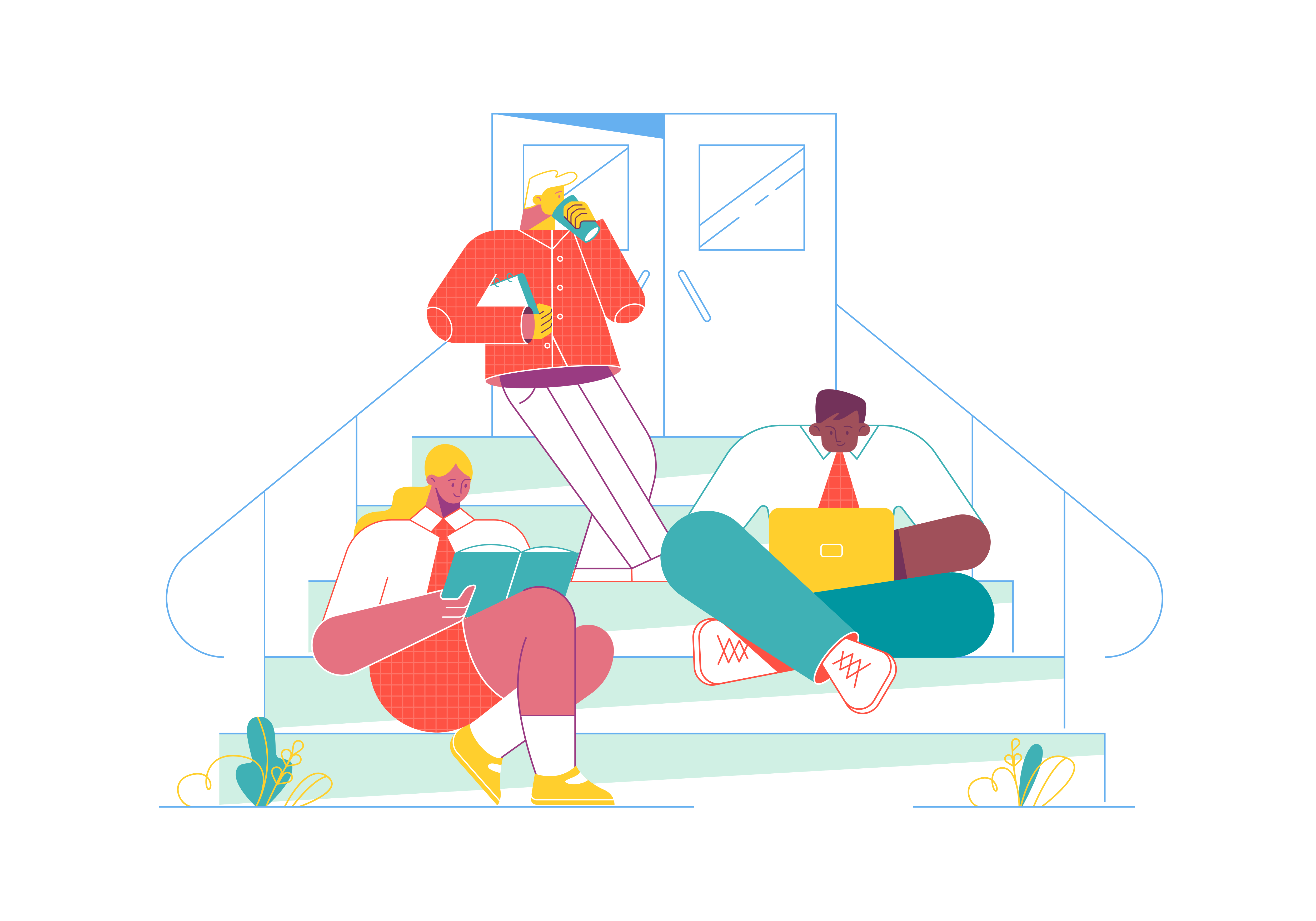
Timelines are an effective way to display chronological information on your website, making it easy for users to follow the sequence of events. Whether you want to showcase historical milestones, project progress, or personal achievements, creating a responsive timeline is essential to ensure your content looks great and functions flawlessly on all devices. In this comprehensive guide, we’ll walk you through the process of building a responsive timeline using Bootstrap, a popular front-end framework.
Understanding the Importance of Responsive Timelines
Before we dive into the practical implementation, let’s take a moment to understand why responsive timelines are essential in web design.
The Challenge of Timelines on Small Screens
Traditional timelines, designed for desktop screens, often struggle to adapt to the limited space available on mobile devices. Without responsiveness, users on smartphones and tablets may have a frustrating experience trying to view and navigate the timeline.
Enhancing User Experience
Responsive design ensures that your timeline adjusts gracefully to different screen sizes and orientations. This not only makes your content accessible to a broader audience but also enhances the user experience by providing a seamless and visually appealing presentation.
Getting Started with Bootstrap
To create a responsive timeline with Bootstrap, you need to have Bootstrap integrated into your project. You can include Bootstrap by downloading the necessary CSS and JavaScript files and adding them to your project, or you can use the Bootstrap CDN for a quicker setup.
Once Bootstrap is set up, you can start building your responsive timeline.
Building the HTML Structure
The HTML structure of your timeline is the foundation of its responsiveness. Here’s a basic example:
<div class="container">
<div class="row">
<div class="col-md-6">
<div class="timeline">
<div class="timeline-item">
<div class="timeline-icon">
<i class="fas fa-calendar-alt"></i>
</div>
<div class="timeline-content">
<h3>Event Title 1</h3>
<p>Event description goes here...</p>
</div>
</div>
<!-- Add more timeline items as needed -->
</div>
</div>
</div>
</div>
In this structure:
- The outer container and grid system classes (
container
,row
,col-md-6
) ensure that your timeline adapts to different screen sizes. - The
timeline
class is a custom class for the timeline container. - Each
timeline-item
represents a single event on the timeline. - The
timeline-icon
andtimeline-content
classes are used to structure each event, with an icon and event details.
Styling Your Timeline
Bootstrap provides a solid foundation for styling your timeline. However, you can further customize its appearance to match your website’s design. Here are some common styling options:
Icons
You can use Bootstrap’s built-in icons or include a third-party icon library like Font Awesome for more icon options. Here’s an example of adding a calendar icon:
<div class="timeline-icon">
<i class="fas fa-calendar-alt"></i>
</div>
Colors and Borders
You can customize the colors and borders of your timeline to match your brand’s style. Use custom CSS to adjust background colors, borders, and fonts as needed.
.timeline-item {
background-color: #f8f8f8;
border: 2px solid #e0e0e0;
/* Add more styling as needed */
}
Animation (Optional)
To add a subtle animation effect when users scroll to view timeline items, you can use CSS transitions. For example, you can gradually increase opacity as items come into view.
.timeline-item {
opacity: 0;
transform: translateY(20px);
transition: opacity 0.5s ease, transform 0.5s ease;
}
.timeline-item.in-view {
opacity: 1;
transform: translateY(0);
}
Making Your Timeline Responsive
Bootstrap’s responsive grid system ensures that your timeline adapts to various screen sizes. However, you can further enhance responsiveness by adjusting the number of columns or stacking events on smaller screens.
<div class="container">
<div class="row">
<div class="col-md-6">
<div class="timeline">
<!-- Timeline items go here -->
</div>
</div>
<div class="col-md-6">
<!-- Additional content, if needed -->
</div>
</div>
</div>
In this example, we’re using a two-column layout on medium-sized screens (col-md-6
). You can modify the number of columns based on your content and design preferences.
Testing and Optimization
After implementing your responsive timeline, thoroughly test it on various devices and screen sizes to ensure it functions as expected. Pay attention to details like text legibility, icon placement, and overall user experience.
Optimize your timeline’s responsiveness based on user feedback and real-world testing. Consider the amount of content, the importance of each event, and user interaction patterns when making adjustments.
Conclusion
Creating a responsive timeline with Bootstrap is a valuable skill for web developers. It ensures that your chronological content remains accessible and user-friendly on devices of all sizes. By following the steps outlined in this guide and customizing your responsive timeline as needed, you can provide an optimal viewing experience for your website’s visitors, regardless of the device they use.