How to implement a tabbed content area with Bootstrap
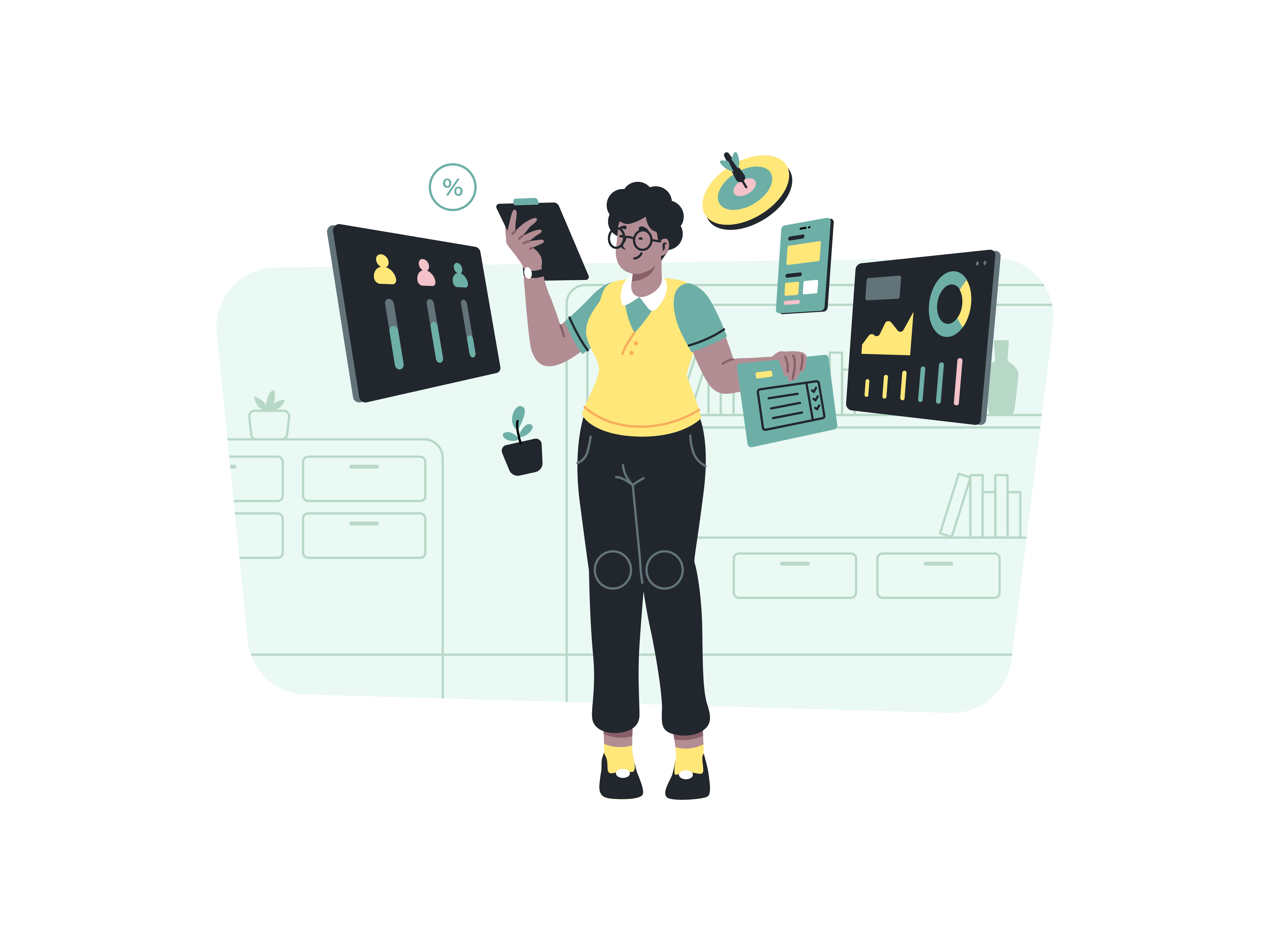
Tabbed content areas are a fantastic way to organize and present information on your website, especially when you have a lot of content to display within limited space. These interactive tabs allow users to switch between different sections of content effortlessly, improving user experience and engagement. In this step-by-step guide, we’ll explore how to implement a tabbed content area using Bootstrap, a popular front-end framework. By the end of this tutorial, you’ll have a dynamic tabbed interface that enhances your website’s usability.
Why Use Tabbed Content
Before we dive into the practical aspects, let’s understand why tabbed content is beneficial:
-
Efficient Use of Space: Tabbed content makes the most of your webpage’s real estate by hiding non-relevant sections until users choose to view them.
-
Organized Information: It keeps related information neatly organized, reducing clutter and helping users find what they need quickly.
-
Enhanced User Experience: Tabs offer an intuitive way to navigate through content, creating a smoother and more engaging experience for your audience.
-
Mobile-Friendly: Tabbed interfaces are responsive by design, making them suitable for both desktop and mobile users.
Now, let’s get started with Bootstrap and create a tabbed content area.
Bootstrap Setup
To create a tabbed content area with Bootstrap, you need to have Bootstrap integrated into your project. You can either download Bootstrap and include the necessary CSS and JavaScript files or use the Bootstrap CDN for faster access.
Once Bootstrap is set up, we can proceed with creating the tabbed interface.
Building the HTML Structure
The HTML structure for tabbed content typically consists of a container with tabs and corresponding content panes. Here’s a basic example:
<div class="container">
<ul class="nav nav-tabs" id="myTabs" role="tablist">
<li class="nav-item" role="presentation">
<a class="nav-link active" id="tab1-tab" data-toggle="tab" href="#tab1" role="tab" aria-controls="tab1" aria-selected="true">Tab 1</a>
</li>
<li class="nav-item" role="presentation">
<a class="nav-link" id="tab2-tab" data-toggle="tab" href="#tab2" role="tab" aria-controls="tab2" aria-selected="false">Tab 2</a>
</li>
<!-- Add more tabs as needed -->
</ul>
<div class="tab-content" id="myTabContent">
<div class="tab-pane fade show active" id="tab1" role="tabpanel" aria-labelledby="tab1-tab">
<!-- Tab 1 content goes here -->
</div>
<div class="tab-pane fade" id="tab2" role="tabpanel" aria-labelledby="tab2-tab">
<!-- Tab 2 content goes here -->
</div>
<!-- Add more content panes as needed -->
</div>
</div>
In this structure:
- We have a container to hold our tabbed content.
- The
<ul>
element with the classnav-tabs
creates the tab navigation. - Each tab is an
<li>
element with a corresponding<a>
link that references a tab content pane. - The tab content is wrapped in a
<div>
with the classtab-content
.
Adding Content
You can add your content within the respective tab content panes. For example:
<div class="tab-pane fade show active" id="tab1" role="tabpanel" aria-labelledby="tab1-tab">
<h2>Tab 1 Content</h2>
<p>This is the content for Tab 1.</p>
</div>
<div class="tab-pane fade" id="tab2" role="tabpanel" aria-labelledby="tab2-tab">
<h2>Tab 2 Content</h2>
<p>This is the content for Tab 2.</p>
</div>
You can customize the content to include text, images, videos, or any other HTML elements to suit your needs.
JavaScript Activation
Bootstrap requires JavaScript to enable the tabbed functionality. You can activate the tabs by adding the following script:
<script>
$(document).ready(function() {
$('#myTabs a').click(function (e) {
e.preventDefault();
$(this).tab('show');
});
});
</script>
Make sure you include the Bootstrap JavaScript library in your project for this script to work.
Styling and Customization
Bootstrap provides a default style for tabs, but you can further customize the appearance using CSS. For example, you can change the background color, text color, and add borders to tabs to match your website’s design.
/* Custom tab styles */
.nav-tabs {
background-color: #f8f8f8;
border-bottom: 2px solid #e0e0e0;
}
.nav-tabs .nav-item .nav-link {
color: #333;
}
.nav-tabs .nav-item .nav-link.active {
background-color: #fff;
border: 1px solid #e0e0e0;
border-bottom-color: transparent;
}
Feel free to customize these styles according to your preferences.
Testing and Optimization
After implementing your tabbed content area, test it thoroughly on various devices and screen sizes to ensure it works as expected. Check for any alignment issues, responsiveness, or usability concerns.
Optimize your tabbed interface based on user feedback and real-world testing. Consider the amount of content, the importance of each tab, and user interaction patterns when making adjustments.
Conclusion
Creating a tabbed content area with Bootstrap is a valuable skill for web developers. It provides an elegant and user-friendly way to organize and present information on your website. By following the steps outlined in this guide and customizing your tabs as needed, you can enhance user engagement and deliver a seamless browsing experience for your audience.