How to implement a responsive navbar with a toggle button for mobile
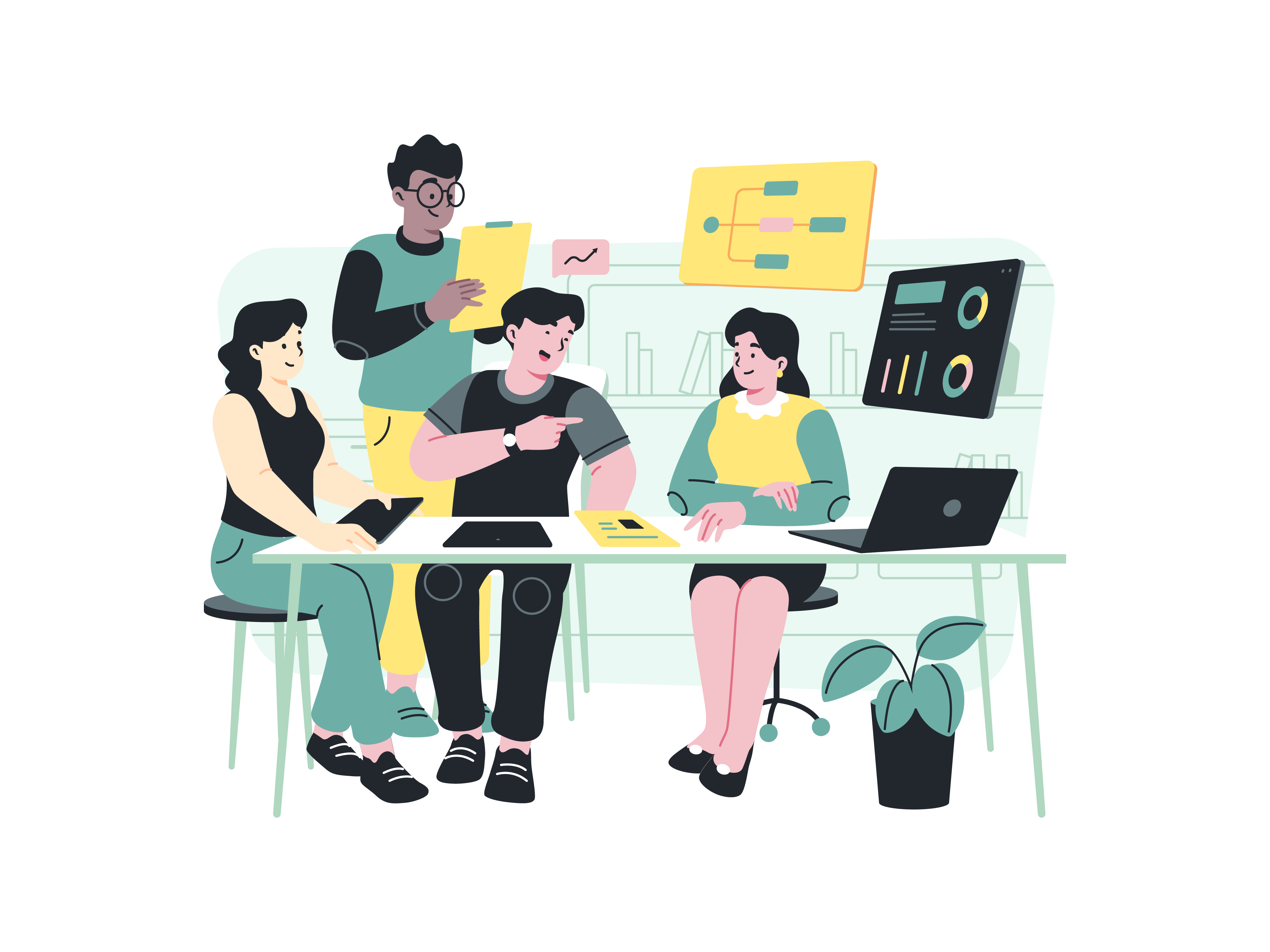
A responsive navigation bar is a fundamental element of modern web design. It ensures that your website is accessible and user-friendly across various devices and screen sizes. To achieve this, you can implement a responsive navbar with a toggle button for mobile devices. In this comprehensive guide, we’ll walk through the steps to create such a navbar using HTML, CSS, and a touch of JavaScript, allowing you to provide an optimal browsing experience for all your users.
Understanding the Concept
Before we dive into the implementation, let’s grasp the concept of a responsive navbar with a toggle button.
Responsive Navigation
Responsive navigation refers to the design practice of adapting your website’s navigation menu to different screen sizes. On larger screens, you might have a traditional horizontal menu, while on smaller screens, like those of mobile devices, you’ll typically switch to a vertical or collapsible menu to conserve space and improve usability.
Toggle Button
A toggle button, often represented as a hamburger icon (three horizontal lines), is used to expand or collapse the navigation menu on mobile devices. When clicked or tapped, it reveals or hides the menu items, offering a clean and space-efficient way to access navigation links.
Building the Responsive Navbar
Now, let’s create a responsive navbar with a toggle button step by step.
1. HTML Structure
Start with the HTML structure of your navbar. Here’s a basic example:
<nav class="navbar">
<div class="navbar-brand">Your Logo</div>
<div class="navbar-toggle">
<div class="bar"></div>
<div class="bar"></div>
<div class="bar"></div>
</div>
<ul class="navbar-menu">
<li><a href="#">Home</a></li>
<li><a href="#">About</a></li>
<li><a href="#">Services</a></li>
<li><a href="#">Contact</a></li>
</ul>
</nav>
In this structure:
.navbar
represents the main container for the navbar..navbar-brand
is where you can place your website logo or brand name..navbar-toggle
holds the toggle button (hamburger icon)..navbar-menu
contains the navigation menu items as an unordered list.
2. CSS Styling
Add CSS styles to create the visual appearance of your navbar. You can customize colors, fonts, and dimensions to match your website’s design. Here’s a simple example to get you started:
/* Basic styles */
.navbar {
background-color: #333;
color: white;
display: flex;
justify-content: space-between;
align-items: center;
padding: 10px 20px;
}
.navbar-brand {
font-size: 24px;
}
.navbar-menu {
list-style: none;
display: flex;
gap: 20px;
}
.navbar-menu li {
font-size: 18px;
}
.navbar-menu a {
text-decoration: none;
color: white;
}
/* Toggle button styles */
.navbar-toggle {
display: none;
flex-direction: column;
cursor: pointer;
}
.bar {
width: 25px;
height: 3px;
background-color: white;
margin: 3px 0;
}
This CSS code styles the navbar, toggle button, and menu items. It also hides the toggle button by default.
3. Implementing the Toggle Functionality
To make the navbar responsive, you’ll need to add JavaScript to toggle the visibility of the menu when the toggle button is clicked. Here’s a simple JavaScript example:
document.addEventListener("DOMContentLoaded", function () {
const toggleButton = document.querySelector(".navbar-toggle");
const menu = document.querySelector(".navbar-menu");
toggleButton.addEventListener("click", function () {
menu.classList.toggle("active");
});
});
This JavaScript code listens for the DOMContentLoaded
event, which ensures that the page is fully loaded before executing the code. It then toggles the active
class on the .navbar-menu
element when the toggle button is clicked.
4. CSS for Mobile
Now, you’ll want to add some CSS to style the menu for mobile devices. The active
class applied by JavaScript will help you control the visibility of the menu items.
@media (max-width: 768px) {
.navbar-menu.active {
display: flex;
flex-direction: column;
position: absolute;
top: 60px; /* Adjust this value as needed */
left: 0;
width: 100%;
background-color: #333;
padding: 20px;
}
.navbar-menu.active li {
margin-bottom: 10px;
}
.navbar-menu.active a {
font-size: 24px;
}
}
This CSS code applies styles to the menu when the screen width is 768 pixels or less, which is a common breakpoint for mobile devices. You can adjust the @media
query and styles as needed for your design.
5. Making the Toggle Button Visible
Finally, you’ll want to make the toggle button visible on smaller screens. Add the following CSS to achieve this:
@media (max-width: 768px) {
.navbar-toggle {
display: flex;
}
}
Now, the toggle button will appear on screens with a width of 768 pixels or less.
Testing and Refining
After implementing your responsive navbar, be sure to thoroughly test it on various devices and screen sizes to ensure it functions as expected. Make adjustments as needed to refine the appearance and behavior.
Conclusion
Creating a responsive navbar with a toggle button for mobile devices is a crucial step in ensuring a positive user experience on your website. By following the steps outlined in this guide and customizing the design to match your branding, you can provide an accessible and user-friendly navigation solution that adapts seamlessly to different screens, ultimately enhancing the usability of your website.