How to use Bootstrap forms and form validation
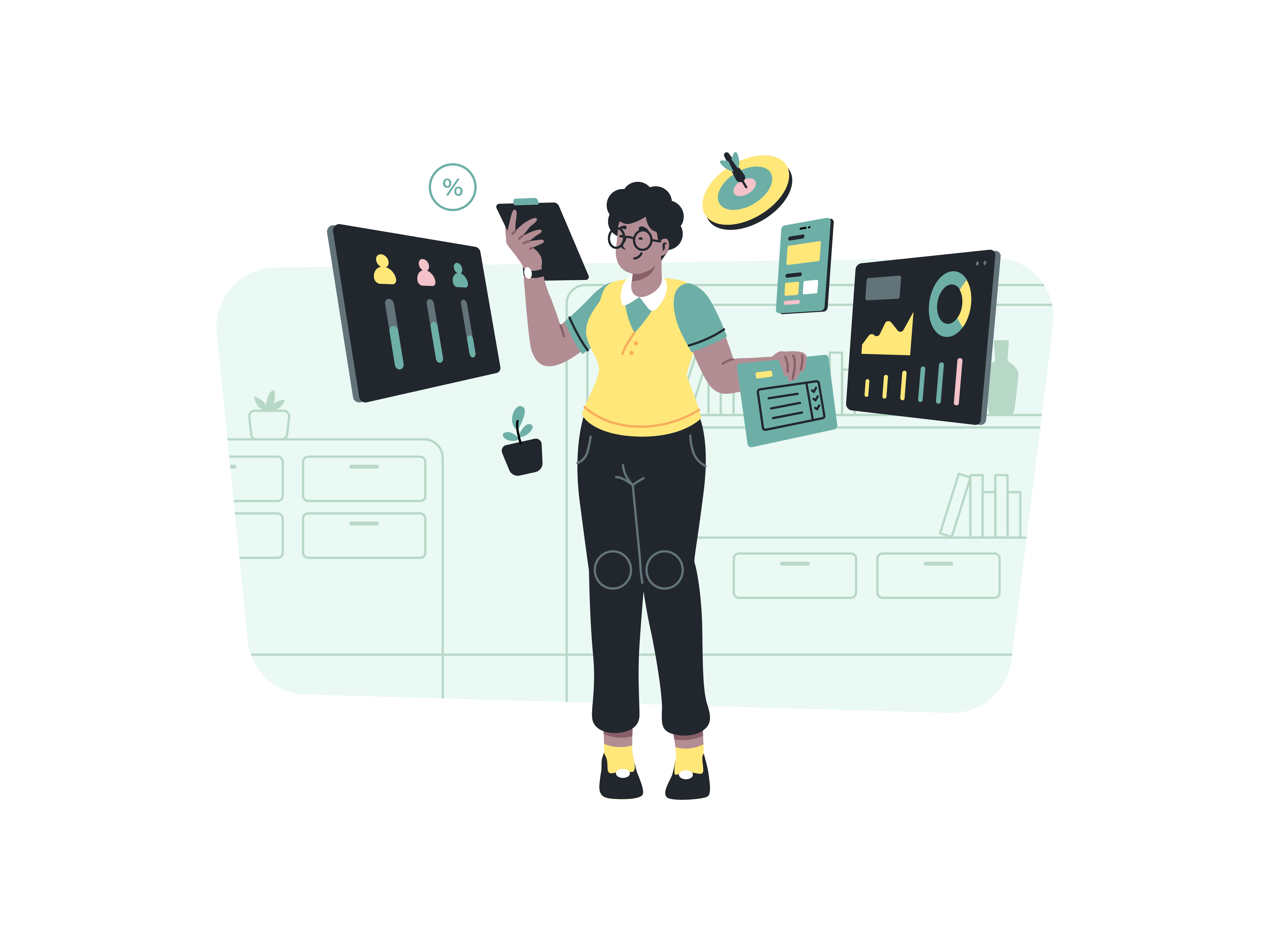
Forms are the backbone of interactive web experiences, enabling users to submit data, provide feedback, and engage with online services. Bootstrap, the popular front-end framework, offers a range of powerful tools for creating stylish and responsive forms, while also providing built-in form validation for enhanced data integrity. In this comprehensive guide, we’ll explore how to effectively utilize Bootstrap’s form components and leverage its form validation features to create user-friendly and error-free forms.
Understanding Bootstrap Forms and Their Importance
Before we delve into the technical details, let’s understand the significance of Bootstrap forms. A well-designed form ensures a smooth user experience by collecting accurate data efficiently. Bootstrap’s form components provide a consistent and visually appealing structure, ensuring forms look great on various devices and screen sizes. Additionally, Bootstrap’s form validation empowers developers to minimize errors and guide users through the data submission process seamlessly.
Getting Started with Bootstrap Forms
To begin harnessing Bootstrap’s form capabilities, ensure that you have Bootstrap integrated into your project. You can opt to download Bootstrap and host it locally or use a Content Delivery Network (CDN). Below is an example of including Bootstrap via CDN:
<!DOCTYPE html>
<html>
<head>
<!-- Include Bootstrap CSS via CDN -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
<title>Bootstrap Forms and Validation</title>
</head>
<body>
<!-- Your content goes here -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
With Bootstrap integrated, you’re ready to dive into creating effective and visually appealing forms.
Building a Basic Bootstrap Form
Bootstrap offers a wide range of form controls, from text inputs to checkboxes, radio buttons, and more. Let’s create a basic form structure using some of these components.
Basic Form Markup
Here’s an example of a simple Bootstrap form with text inputs, radio buttons, checkboxes, and a submit button:
<form>
<div class="mb-3">
<label for="name" class="form-label">Name</label>
<input type="text" class="form-control" id="name" placeholder="Enter your name">
</div>
<div class="mb-3">
<label class="form-label">Gender</label>
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="male" value="male">
<label class="form-check-label" for="male">Male</label>
</div>
<div class="form-check">
<input class="form-check-input" type="radio" name="gender" id="female" value="female">
<label class="form-check-label" for="female">Female</label>
</div>
</div>
<div class="mb-3">
<div class="form-check">
<input class="form-check-input" type="checkbox" id="subscribe" value="subscribe">
<label class="form-check-label" for="subscribe">Subscribe to newsletter</label>
</div>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
In this example, we’ve used Bootstrap’s .form-control
class for text inputs, .form-check
for checkboxes and radio buttons, and a .btn
class for the submit button.
Implementing Form Validation
Bootstrap provides built-in form validation classes and JavaScript functionality to improve data accuracy and user experience.
Basic Validation Markup
To implement basic form validation, you can use Bootstrap’s validation classes along with HTML5’s built-in validation attributes:
<form class="needs-validation" novalidate>
<div class="mb-3">
<label for="email" class="form-label">Email</label>
<input type="email" class="form-control" id="email" placeholder="Enter your email" required>
<div class="invalid-feedback">
Please enter a valid email address.
</div>
</div>
<div class="mb-3">
<!-- Additional form controls with validation -->
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
In this example, the .needs-validation
class on the form triggers the built-in form validation. The required
attribute ensures that the field must be filled out before submission, and the .invalid-feedback
class provides error messages.
Custom Validation Styles
Bootstrap offers additional validation styles for different states, such as valid and invalid inputs:
<form class="needs-validation" novalidate>
<div class="mb-3">
<label for="password" class="form-label">Password</label>
<input type="password" class="form-control is-invalid" id="password" required>
<div class="invalid-feedback">
Please provide a password.
</div>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
In this example, the .is-invalid
class on the input field triggers the display of the invalid feedback message.
Advanced Validation with JavaScript
For more complex validation scenarios, Bootstrap’s JavaScript functionality allows you to provide custom validation logic.
<script>
// Example custom validation using JavaScript
(function () {
'use strict';
document.addEventListener('DOMContentLoaded', function () {
var forms = document.querySelectorAll('.needs-validation');
Array.from(forms).forEach(function (form) {
form.addEventListener('submit', function (event) {
if (!form.checkValidity()) {
event.preventDefault();
event.stopPropagation();
}
form.classList.add('was-validated');
}, false);
});
}, false);
})();
</script>
In this example, the JavaScript code ensures that the form’s custom validation logic is triggered when the form is submitted.
Conclusion
Bootstrap’s form components and form validation features are invaluable assets in creating interactive and error-free user experiences. By understanding the basics of form structure, implementing validation classes, and utilizing custom validation logic, you can create forms that are not only visually appealing but also efficient in collecting accurate data. From text inputs to checkboxes and radio buttons, Bootstrap provides a comprehensive toolkit to cater to your form-related needs. Start integrating these techniques into your projects and elevate your web forms to a new level of functionality and user satisfaction.