How to create a responsive navbar with Bootstrap
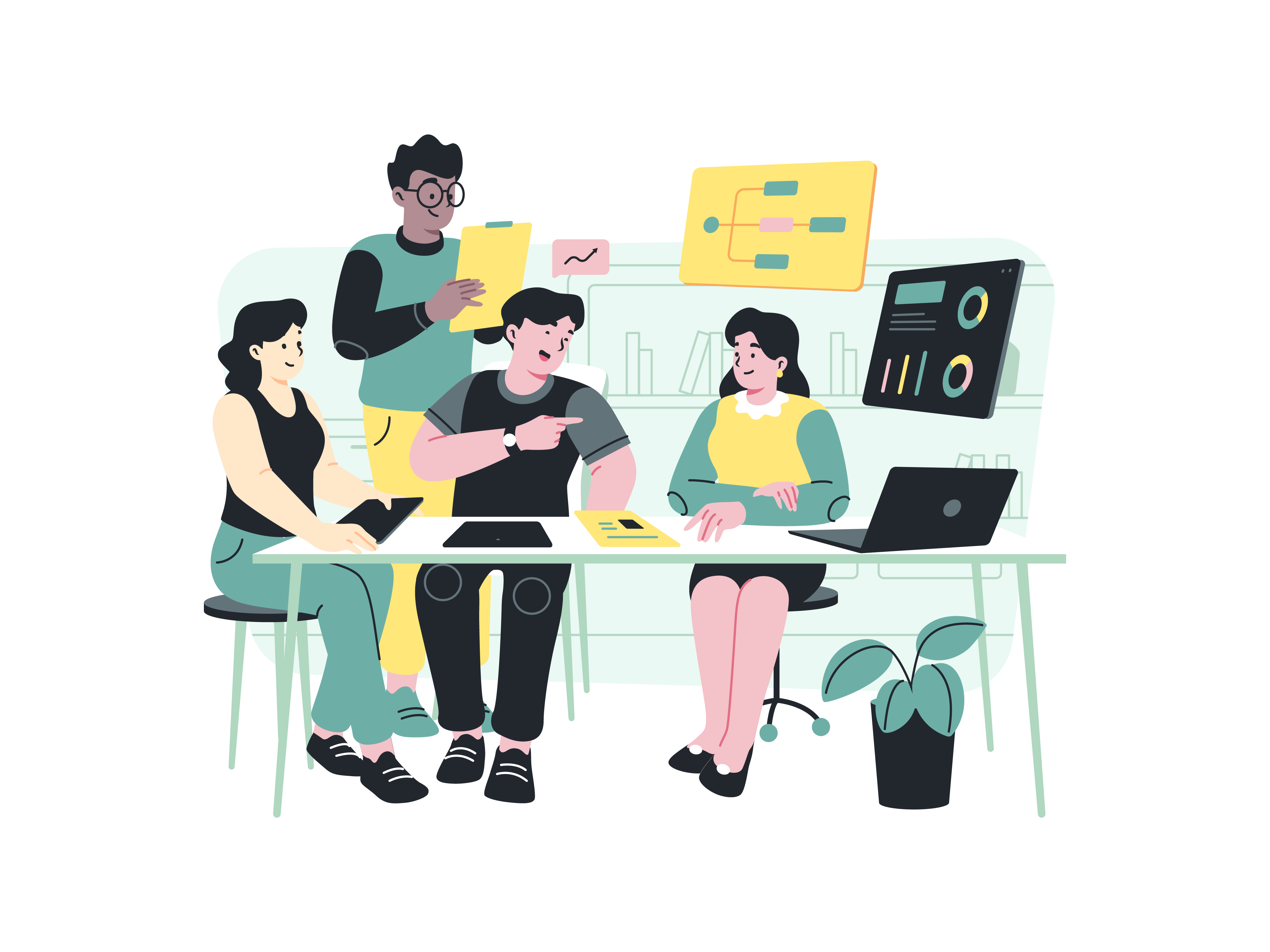
The navigation bar, often referred to as the navbar, is a fundamental component of any website’s user interface. It serves as a guiding beacon, helping users navigate through the content seamlessly. With the surge of mobile devices and varying screen sizes, creating a responsive navbar is crucial to ensure an optimal user experience. In this comprehensive guide, we’ll explore how to craft a responsive navbar using Bootstrap, the acclaimed front-end framework.
Embracing the Significance of a Responsive Navbar
Before we dive into the technicalities, let’s understand why a responsive navbar matters. A responsive navbar adapts its layout and appearance to different screen sizes, ensuring that users can easily access navigation links, menus, and other essential content regardless of the device they’re using. Bootstrap’s responsive design principles empower developers to create a navbar that remains effective, visually appealing, and user-friendly across various devices.
Getting Started with Bootstrap’s Navbar
To begin crafting a responsive navbar with Bootstrap, you need to have Bootstrap integrated into your project. You can opt to download Bootstrap and host it locally or employ a Content Delivery Network (CDN). Below is an example of including Bootstrap via CDN:
<!DOCTYPE html>
<html>
<head>
<!-- Include Bootstrap CSS via CDN -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
<title>Responsive Navbar with Bootstrap</title>
</head>
<body>
<!-- Your content goes here -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
With Bootstrap integrated, you’re prepared to embark on creating a responsive navbar.
Constructing a Basic Navbar Structure
Bootstrap’s navbar component offers a wealth of options for customization. Let’s start by building a basic navbar structure that is responsive.
Basic Navbar Markup
Here’s a simple example of a responsive navbar using Bootstrap’s .navbar
class:
<nav class="navbar navbar-expand-lg navbar-light bg-light">
<a class="navbar-brand" href="#">Logo</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav ml-auto">
<li class="nav-item active">
<a class="nav-link" href="#">Home</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">About</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Services</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#">Contact</a>
</li>
</ul>
</div>
</nav>
In this example, the .navbar-expand-lg
class ensures that the navbar collapses into a hamburger menu on smaller screens. The navbar-toggler
button triggers the collapse and expansion of the navigation links.
Adding Responsive Behaviors
Bootstrap’s responsive navbar adapts dynamically to different screen sizes. Let’s explore some common responsive behaviors:
Hamburger Menu for Small Screens
To create a hamburger menu for small screens, add the .navbar-toggler
button along with the data-bs-toggle
and data-bs-target
attributes:
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav" aria-controls="navbarNav" aria-expanded="false" aria-label="Toggle navigation">
<span class="navbar-toggler-icon"></span>
</button>
Centering Navbar Items
For center-aligned navigation items, use the .navbar-nav justify-content-center
classes:
<div class="collapse navbar-collapse justify-content-center" id="navbarNav">
<!-- Navigation items -->
</div>
Right-Aligned Navbar Items
To right-align navigation items, use the .navbar-nav ml-auto
classes:
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav ml-auto">
<!-- Navigation items -->
</ul>
</div>
Customization and Styling
Bootstrap’s navbar is highly customizable. You can add your logo, adjust colors, typography, and spacing to match your website’s design.
Conclusion
A responsive navbar is a cornerstone of user-friendly web design in today’s multi-device landscape. By leveraging Bootstrap’s powerful navbar component, you can create an intuitive and visually appealing navigation system that adapts seamlessly to various screen sizes. From constructing the basic structure to implementing responsive behaviors and customizing the style, this guide has provided you with the tools to create a responsive navbar that enhances user experience and engagement. Start integrating these techniques into your projects and guide your users effortlessly through your website’s content, regardless of the device they’re using.