How to use Git and GitHub for version control
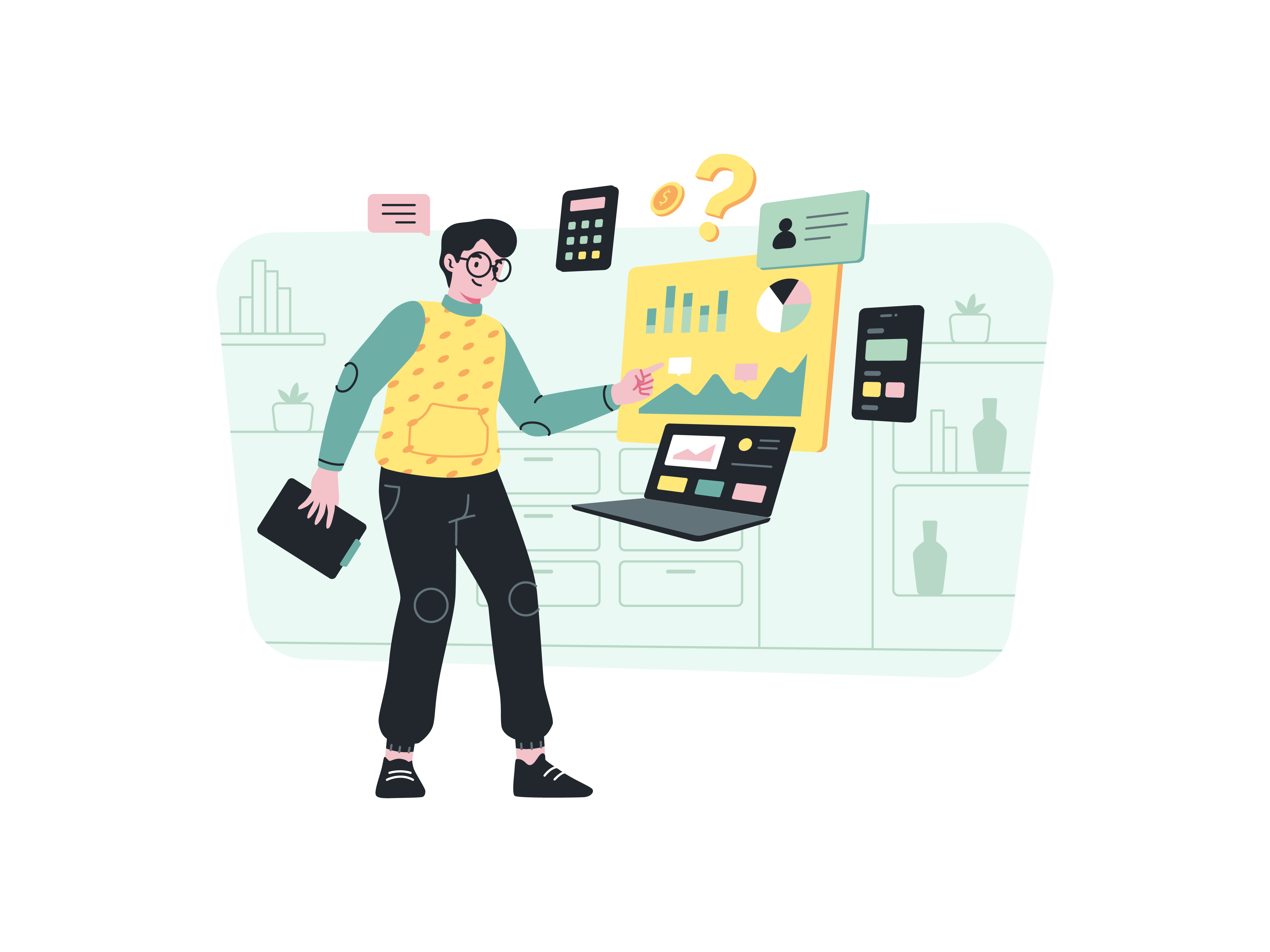
In the world of software development, version control is a critical practice for managing and tracking changes to code. Git, a distributed version control system, and GitHub, a platform built on top of Git for hosting and collaboration, have become the standard tools for both individual developers and large teams. This guide will explore how to use Git and GitHub effectively, from basic workflows to advanced techniques.
Introduction to Version Control
Version control systems (VCS) help developers manage changes to source code over time. They allow for collaboration, prevent conflicts, and provide a history of changes that can be referred to or reverted back to at any point. By tracking every modification, version control makes sure that developers can work simultaneously on the same project without interfering with each other’s work.
Git, unlike other version control systems, is distributed, which means every user gets a full copy of the repository and its history. This feature not only allows for more autonomy but also makes it possible to work offline. GitHub, an online hosting service for Git repositories, enhances collaboration by offering features such as pull requests, issues, and team management tools.
What is Git?
Git is a distributed version control system that tracks changes to files in a repository. It was developed by Linus Torvalds in 2005 to support the development of the Linux kernel. Git is popular due to its flexibility, speed, and powerful branching capabilities.
Key Features of Git
- Distributed Architecture: Unlike centralized systems, each developer has a complete local copy of the repository.
- Efficient Branching: Branches are lightweight, making it easy to experiment and isolate changes.
- Commit Tracking: Git stores each change as a snapshot of the repository, offering a detailed history of all modifications.
- Data Integrity: Git uses SHA-1 hashes to secure the integrity of the data.
- Speed: Git performs most operations locally, which results in faster execution of commands.
Why Choose Git Over Other Systems?
- SVN (Subversion): It is centralized, meaning there is only one master copy of the repository, and it lacks some features like branching, which Git handles more efficiently.
- Mercurial: While similar to Git, Mercurial has fewer users and less widespread adoption.
- Perforce: Mainly used for large binary files, Perforce can be cumbersome to set up and maintain.
Git is the go-to tool for most software developers because of its flexibility, speed, and robust branching and merging capabilities.
What is GitHub?
GitHub is a cloud-based platform that hosts Git repositories. It allows you to store your code online, collaborate with others, and manage your project’s development process. GitHub adds additional functionality to Git, such as an interface for pull requests, issue tracking, and project management tools.
Key Features of GitHub
- Remote Hosting: GitHub hosts your repositories in the cloud, making them accessible from anywhere.
- Collaboration Tools: It facilitates teamwork through features like pull requests, issues, and project boards.
- CI/CD Integration: Automate workflows and deployments with GitHub Actions.
- GitHub Pages: Host static websites directly from repositories.
- Community Engagement: GitHub is widely used for open-source projects, allowing developers to contribute to a variety of public projects.
Setting Up Git
Before you can start using Git, you need to install it and configure your personal settings. Here’s how you can do that.
Installing Git
On Windows
-
Download the Git installer from git-scm.com.
-
Follow the installation wizard’s default settings.
-
After installation, confirm by running the following in your command prompt:
git --version
On macOS
-
You can install Git using Homebrew by running:
brew install git
-
Verify the installation by running:
git --version
On Linux
-
On most Linux distributions, Git can be installed using the system’s package manager. For Ubuntu, use:
sudo apt update sudo apt install git
-
Check if Git is installed:
git --version
Configuring Git
Once Git is installed, you need to configure it with your user details, which will be associated with your commits.
git config --global user.name "Your Name"
git config --global user.email "[email protected]"
Core Git Concepts
Understanding basic Git concepts is essential for working with it effectively. Here are the primary concepts you’ll encounter:
- Repository: The main directory containing all project files and their version history.
- Commit: A snapshot of changes made to the repository, which includes a message describing the change.
- Branch: A parallel version of your repository. Branches allow you to work on different features or bug fixes without affecting the main project.
- Remote: A version of your repository hosted online, typically on a service like GitHub.
- Merge: The process of combining changes from one branch into another.
- HEAD: A pointer to the most recent commit on the current branch.
Basic Git Workflows
Now that you know the core concepts, let’s walk through the basic Git workflow:
Step 1: Initialize a Repository
To start using Git in your project, initialize a Git repository:
git init
Step 2: Add Files to Staging
Before committing, you need to stage the changes. Use the following command to stage all modified files:
git add .
Step 3: Commit Changes
Once changes are staged, commit them to the repository with a message describing the change:
git commit -m "Initial commit"
Step 4: Check Repository Status
To see which files have been modified or staged for commit:
git status
Step 5: View Commit History
To view the history of commits in the repository:
git log
Branching and Merging
Branches in Git allow you to develop features, fix bugs, and experiment without affecting the main branch. Git makes branching easy and efficient.
Creating a Branch
You can create and switch to a new branch with the following commands:
git branch feature-branch
git checkout feature-branch
Merging a Branch
To merge changes from one branch (e.g., a feature branch) into another (e.g., the main branch), first check out the target branch:
git checkout main
git merge feature-branch
Using GitHub
GitHub adds additional collaboration tools on top of Git. Here’s how to use GitHub effectively.
Creating a Repository
- Go to GitHub and click on New Repository.
- Provide a repository name, and choose whether it should be public or private.
- Initialize with a README file (optional).
Cloning a Repository
If you want to work on a project that’s already on GitHub, you can clone it to your local machine:
git clone <repository-url>
Pushing Changes
Once you’ve made changes to your repository locally, push them to GitHub:
git remote add origin <repository-url>
git push -u origin main
Advanced Git Techniques
As you become more comfortable with Git, you can start using some of its advanced features.
Rebasing
Rebasing is an alternative to merging and offers a cleaner commit history:
git rebase main
Stashing
Sometimes you might want to save changes without committing them. Git’s stash feature allows you to temporarily store changes:
git stash
git stash apply
Submodules
Submodules allow you to include one Git repository inside another. This is useful for managing dependencies:
git submodule add <repository-url>
Troubleshooting Common Issues
While working with Git, you may run into a few issues. Here are some common ones:
-
Detached HEAD
This happens when you’re not on a branch. To resolve, check out a branch:git checkout main
-
Merge Conflicts
When merging branches, Git may encounter conflicts. Manually resolve the conflicts, then commit the changes.
Best Practices
To work effectively with Git, follow these best practices:
- Write Clear Commit Messages: Every commit should include a concise, clear message explaining what was changed.
- Keep Branches Short-Lived: Avoid long-running branches. Merge frequently to keep your repository clean.
- Use
.gitignore
: This file tells Git which files should not be tracked (e.g., IDE settings or build artifacts).
Integrating Git and GitHub with Other Tools
Git and GitHub integrate well with a wide range of development tools.
- GitHub Desktop: A GUI for Git that simplifies version control for beginners.
- Visual Studio Code: A code editor with built-in Git support.
- CI/CD Tools: Automate testing and deployment pipelines with services like Jenkins or GitHub Actions.
Conclusion
Git and GitHub are indispensable tools for developers today. Understanding Git’s core functionality, best practices, and advanced features, along with GitHub’s collaborative tools, will allow you to manage your projects efficiently and work collaboratively with teams. Whether you are working alone or as part of a team, mastering Git and GitHub is a crucial skill in modern software development.
Where is state stored in React