Can a Next.js application use both server-side rendering and client-side rendering
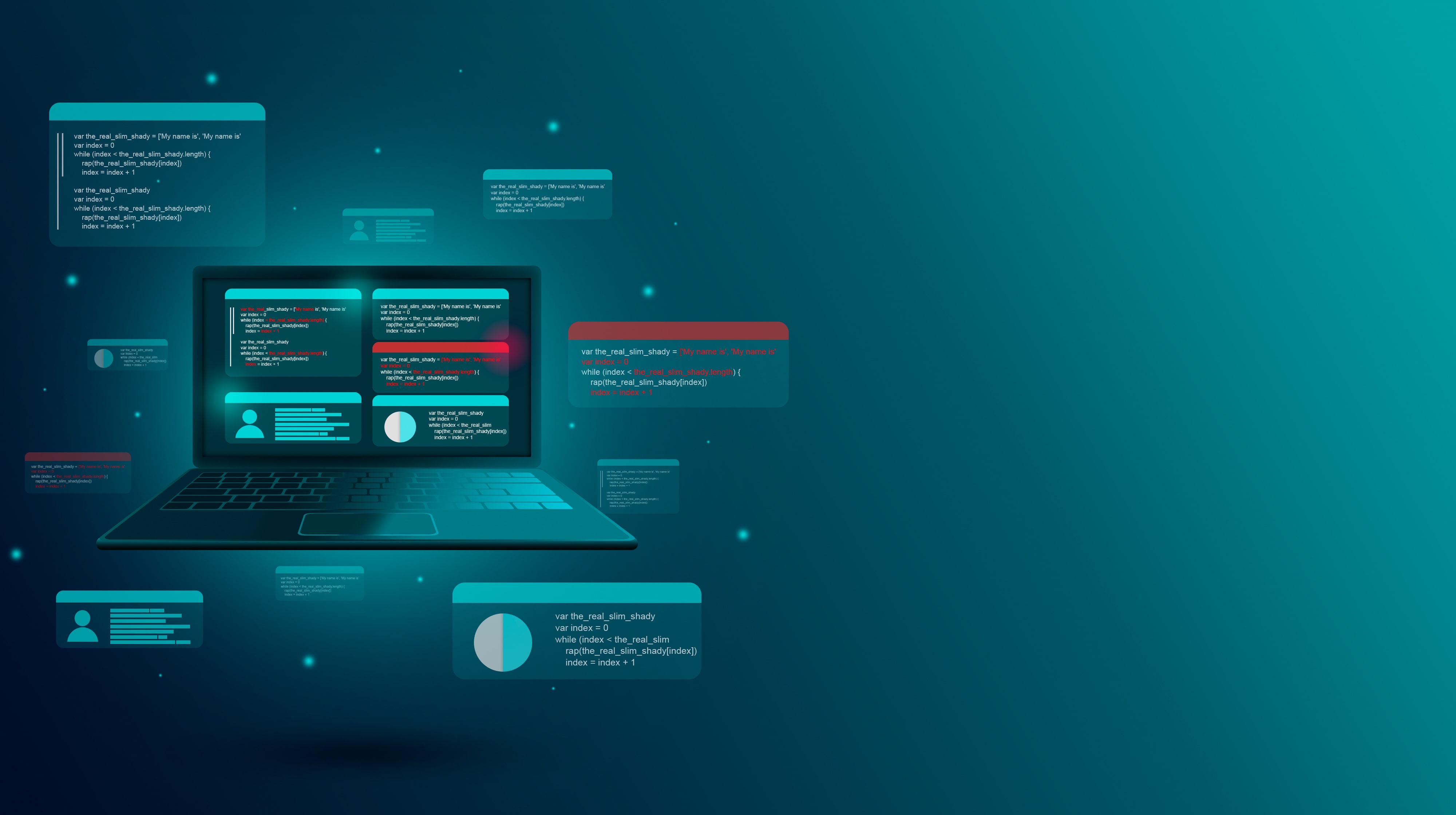
In the dynamic landscape of web development, the ability to deliver fast, interactive, and search engine-friendly applications is a constant pursuit. Next.js, a powerful React framework, has emerged as a versatile tool that provides developers with the flexibility to choose between server-side rendering (SSR) and client-side rendering (CSR). But what if the optimal solution lies in combining both approaches? In this article, we’ll explore the possibilities and intricacies of using both server-side rendering and client-side rendering in a Next.js application.
Understanding Server-Side Rendering (SSR) and Client-Side Rendering (CSR)
Before delving into the synergy of SSR and CSR in Next.js, it’s essential to understand the core concepts of each rendering approach.
Server-Side Rendering (SSR)
- In SSR, the server generates the HTML for each request, delivering fully rendered pages to the client. This approach improves initial page load times, facilitates better SEO, and ensures that users receive content faster.
Client-Side Rendering (CSR)
- In CSR, the initial HTML is minimal, and the browser takes on the responsibility of rendering the page and fetching data asynchronously. While CSR provides a more dynamic user experience, it can lead to slower initial page loads.
Next.js and the Hybrid Rendering Approach
Next.js allows developers to choose between SSR, CSR, or even adopt a hybrid approach that combines both. This hybrid rendering approach leverages the strengths of SSR for initial page loads and SEO while incorporating the dynamism of CSR for subsequent interactions.
1. File-Based Routing for SSR
- Next.js employs file-based routing, where pages are automatically generated based on the structure of the “pages” directory. For pages requiring SSR, developers can utilize the
getServerSideProps
function to fetch data on each request and provide a fully rendered HTML page.
// Example using getServerSideProps for SSR
export async function getServerSideProps(context) {
// Fetch data based on the request context
const data = /* fetch data from API or other source */;
return {
props: {
data,
},
};
}
2. Automatic Code Splitting for CSR
- For pages where dynamic updates and interactions are crucial, developers can rely on automatic code splitting to implement CSR. Next.js generates separate bundles for each page, ensuring that only the necessary JavaScript code is loaded when a specific page is accessed.
// Example using useEffect for CSR
import { useEffect, useState } from 'react';
function DynamicPage() {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data asynchronously on the client side
const fetchData = async () => {
const result = /* fetch data from API or other source */;
setData(result);
};
fetchData();
}, []);
return (
// Render the component using the fetched data
// ...
);
}
3. getStaticProps for Static Site Generation (SSG)
- Next.js also supports static site generation (SSG) using the
getStaticProps
function. This approach generates HTML at build time, providing the benefits of both SSR and CSR by ensuring fast initial page loads while allowing for dynamic updates.
// Example using getStaticProps for SSG
export async function getStaticProps() {
// Fetch data at build time
const data = /* fetch data from API or other source */;
return {
props: {
data,
},
revalidate: 60, // Revalidate the page every 60 seconds
};
}
Best Practices for Combining SSR and CSR in Next.js
To strike the perfect balance and harness the benefits of both SSR and CSR in Next.js, consider the following best practices:
Identify Critical Pages
- Identify pages where SSR is crucial for initial load performance and SEO. Use
getServerSideProps
for these pages.
Leverage Automatic Code Splitting
- Let Next.js handle automatic code splitting to ensure that only the necessary JavaScript is loaded for each page, optimizing performance.
Optimize Dynamic Interactions with CSR
- Use CSR for pages that require dynamic interactions, real-time updates, and asynchronous data fetching.
Consider Static Site Generation (SSG)
- Explore the benefits of SSG using
getStaticProps
for pages with content that can be pre-rendered at build time.
Fine-Tune Revalidation Intervals
- If employing SSG with ISR (Incremental Static Regeneration), fine-tune revalidation intervals using the
revalidate
key ingetStaticProps
.
// Example with ISR using revalidate
export async function getStaticProps() {
const data = /* fetch data from API or other source */;
return {
props: {
data,
},
revalidate: 60, // Revalidate the page every 60 seconds
};
}
Pros and Cons of Hybrid Rendering in Next.js
Pros
- Optimized Performance: Combining SSR and CSR allows for optimized performance with fast initial page loads and dynamic client-side interactions.
- Improved SEO: SSR contributes to better SEO as search engines can crawl fully rendered pages.
- Enhanced User Experience: CSR provides a smoother user experience with dynamic updates and real-time interactions.
Cons
- Complexity: Combining SSR and CSR introduces complexity to the project, requiring careful consideration of data fetching strategies and balancing between server-side and client-side rendering.
- Increased Build Time: The use of both SSR and CSR may lead to increased build times, especially in larger projects.
Conclusion
In conclusion, Next.js empowers developers to choose the most suitable rendering approach for their applications, be it server-side rendering, client-side rendering, or a seamless combination of both. By leveraging the strengths of SSR for initial page loads and SEO, while embracing CSR for dynamic interactions, developers can strike a harmonious balance that optimizes performance and user experience.
As the web development landscape continues to evolve, the flexibility offered by Next.js in rendering strategies becomes increasingly valuable. Whether aiming for faster page loads, improved SEO, or dynamic user interactions, the ability to combine SSR and CSR in a Next.js application opens up a world of possibilities, ensuring that developers can tailor their approach to meet the unique requirements of their projects.
How many states are there in React
Where is state stored in React