How does Next.js handle SEO for dynamic pages
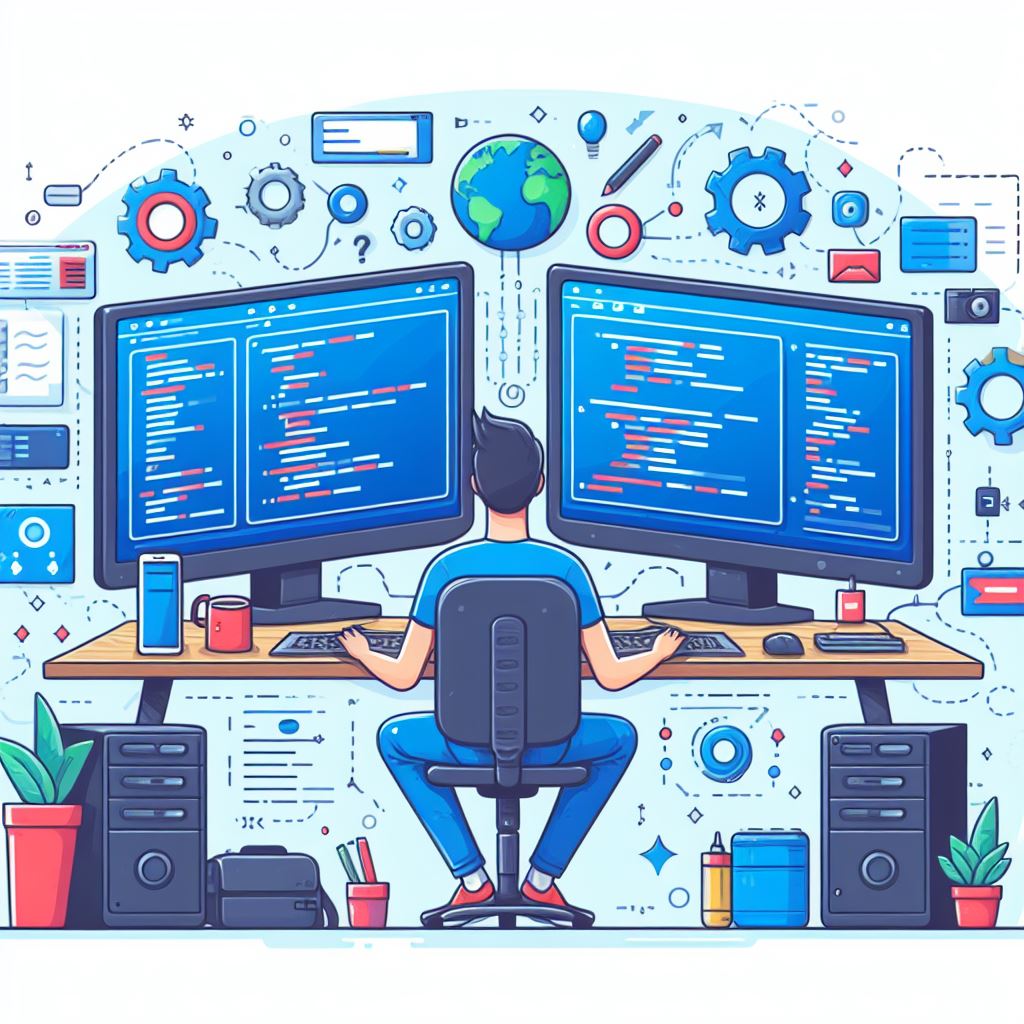
In the ever-evolving landscape of web development, achieving optimal search engine optimization (SEO) is paramount for ensuring the visibility and success of a website. Next.js, a powerful React framework, has garnered attention for its efficient handling of SEO, especially when dealing with dynamic pages. In this article, we will explore how Next.js tackles SEO challenges for dynamic pages, unveiling the mechanisms and best practices that empower developers to strike the right balance between dynamic content and search engine visibility.
The Challenge of SEO for Dynamic Pages
Dynamic pages, fueled by real-time data or user interactions, present a unique challenge for SEO. Traditional search engines favor static content that can be readily indexed, making it challenging for dynamically generated content to achieve optimal visibility. This is where Next.js, with its versatile features, steps in to bridge the gap and ensure that dynamic pages don’t compromise on SEO effectiveness.
Leveraging Server-Side Rendering (SSR)
One of Next.js' key strengths in handling SEO for dynamic pages lies in its support for server-side rendering (SSR). With SSR, Next.js generates the HTML content on the server for each request, providing search engines with fully rendered pages that are easily indexable.
1. getServerSideProps Function
- Next.js utilizes the
getServerSideProps
function to fetch data at runtime before rendering the page. This function plays a crucial role in dynamically generating content for SEO purposes.
// Example using getServerSideProps for SEO on dynamic pages
export async function getServerSideProps(context) {
// Fetch data based on the request context (e.g., dynamic parameters)
const dynamicData = /* fetch dynamic data from API or other source */;
return {
props: {
dynamicData,
},
};
}
2. Dynamic Routes and SEO
- Next.js supports dynamic routes, enabling developers to create pages with dynamic parameters. The combination of dynamic routes and SSR ensures that search engines can crawl and index unique URLs, even for dynamic content.
// Example of a dynamic route in Next.js
// [id].js
export async function getServerSideProps(context) {
// Fetch data based on the dynamic parameter (e.g., id)
const dynamicData = /* fetch dynamic data using context.params.id */;
return {
props: {
dynamicData,
},
};
}
Implementing Static Site Generation (SSG) with Incremental Static Regeneration (ISR)
While SSR is a robust solution for SEO on dynamic pages, Next.js goes a step further by introducing static site generation (SSG) with Incremental Static Regeneration (ISR). SSG allows for the pre-rendering of pages at build time, while ISR ensures that certain pages can be regenerated with fresh data at specified intervals.
1. getStaticPaths and getStaticProps for Dynamic SSG
- The combination of
getStaticPaths
andgetStaticProps
functions facilitates dynamic SSG for pages with dynamic parameters, offering a perfect blend of performance and SEO benefits.
// Example using getStaticPaths and getStaticProps for dynamic SSG
export async function getStaticPaths() {
// Fetch the list of dynamic paths
const dynamicPaths = /* fetch dynamic paths from API or other source */;
return {
paths: dynamicPaths,
fallback: true, // Enable fallback for pages not yet generated
};
}
export async function getStaticProps(context) {
// Fetch data based on the dynamic parameter
const dynamicData = /* fetch dynamic data using context.params.id */;
return {
props: {
dynamicData,
},
revalidate: 60, // Revalidate the page every 60 seconds
};
}
2. ISR for Periodic Updates
- ISR introduces the concept of revalidation intervals, ensuring that pages are periodically regenerated with fresh data. This feature is particularly beneficial for pages with dynamic content that changes over time.
// Example using ISR with revalidate for periodic updates
export async function getStaticProps() {
// Fetch data at build time
const dynamicData = /* fetch dynamic data from API or other source */;
return {
props: {
dynamicData,
},
revalidate: 60, // Revalidate the page every 60 seconds
};
}
Best Practices for SEO on Dynamic Pages in Next.js
To maximize SEO effectiveness on dynamic pages in Next.js, consider the following best practices:
Strategic Use of SSR and SSG
- Strategically leverage SSR and SSG based on the nature of the dynamic content. Use SSR for pages with frequently changing or real-time data, and SSG for pages with content that can be pre-rendered at build time.
Optimize Dynamic Routes
- Make use of dynamic routes to create unique URLs for dynamic content. This ensures that search engines can index and rank individual pages, enhancing visibility.
Fine-Tune Revalidation Intervals
- When implementing ISR, fine-tune revalidation intervals using the
revalidate
key ingetStaticProps
. Consider the frequency at which the dynamic content changes to determine an appropriate revalidation interval.
Handle Fallback Gracefully
- When enabling fallback in dynamic SSG, handle it gracefully by providing a loading state or placeholder content. This ensures a smooth user experience while pages are being generated.
Ensure Accessibility of Dynamic Content
- Ensure that dynamically generated content is accessible to search engine crawlers. Avoid relying solely on client-side rendering for critical content that needs to be indexed.
Conclusion
Next.js stands out as a powerful framework that adeptly addresses the SEO challenges posed by dynamic pages. By seamlessly integrating server-side rendering, dynamic routes, and static site generation with incremental static regeneration, Next.js empowers developers to achieve the delicate balance between dynamic content and search engine visibility.
As websites continue to evolve, incorporating real-time data and dynamic user experiences, the SEO capabilities of a framework become increasingly crucial. Next.js, with its arsenal of features, emerges as a reliable ally in the quest for SEO excellence on dynamic pages, ensuring that developers can deliver both performance and visibility in the ever-expanding digital landscape.
How does React handle dynamic imports
How do you handle code splitting with React Router
Explain the concept of lazy loading in React
What is the significance of the forwardRef function in React
What is the significance of the react-router-dom library
How do you implement server-side rendering with React and Node.js
How do you implement server-side rendering with React and Express