How can I enable or disable certain features/modules in Tailwind CSS
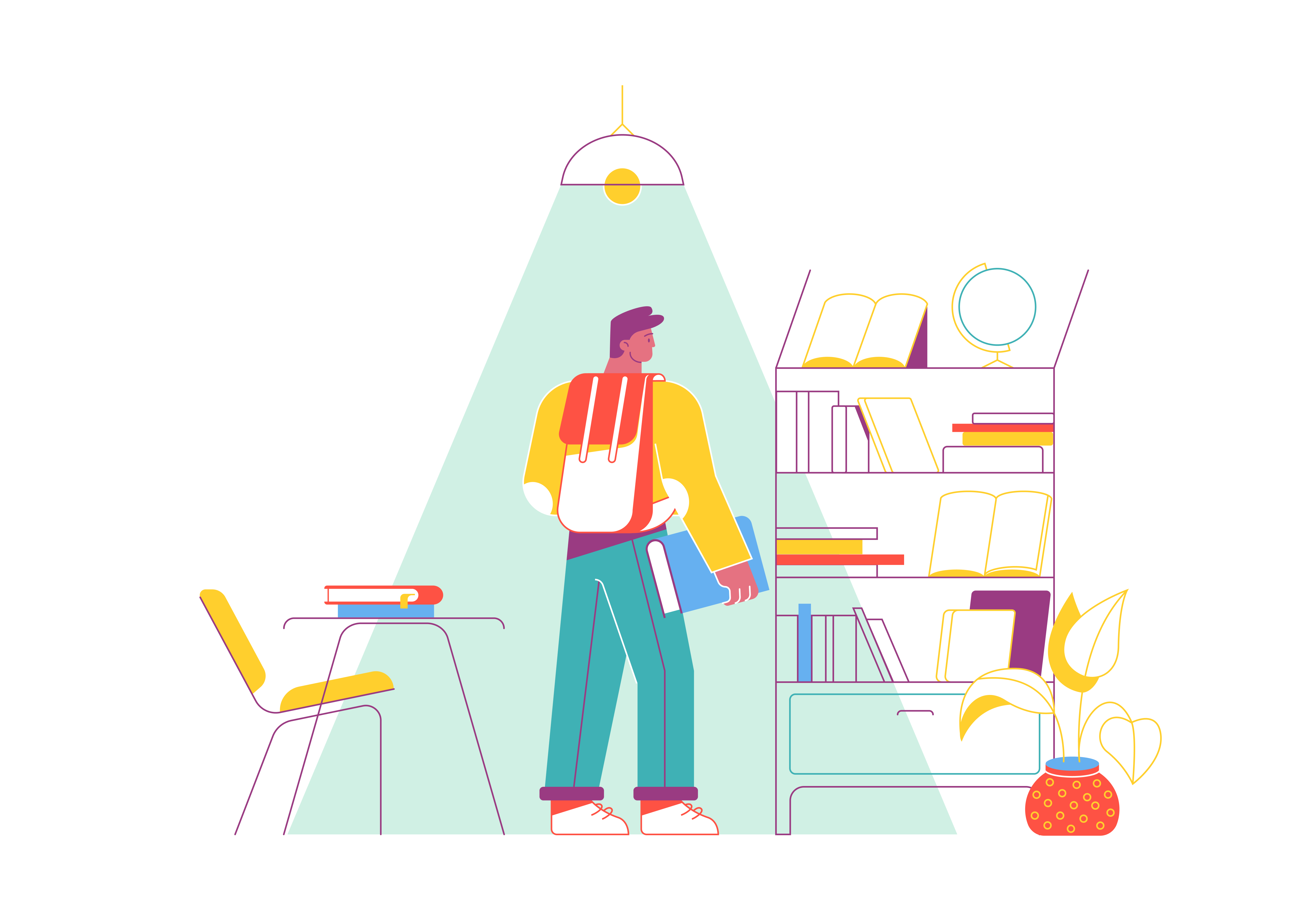
Tailwind CSS has revolutionized the way developers approach styling in web development, offering a utility-first approach that provides unprecedented flexibility. One of the most powerful aspects of Tailwind is its ability to customize and optimize your CSS by enabling or disabling specific features and modules. This comprehensive guide will walk you through various strategies to fine-tune your Tailwind CSS configuration, helping you create leaner, more efficient stylesheets.
Understanding Tailwind CSS Modules
Before diving into enabling and disabling features, it’s crucial to understand what Tailwind CSS modules are. Tailwind is composed of multiple core modules that provide different types of utility classes:
- Layout Utilities: Controlling display, positioning, and box sizing
- Flexbox and Grid: Utilities for flexible and grid-based layouts
- Spacing Utilities: Margin and padding classes
- Sizing Utilities: Width, height, and max/min dimension controls
- Typography Utilities: Font size, weight, alignment, and text styling
- Background Utilities: Background color, images, and other background properties
- Border Utilities: Border width, color, radius, and styling
- Effect Utilities: Opacity, box shadow, and other visual effects
- Filter Utilities: Color manipulation and visual transformations
- Transition Utilities: Animation and transition properties
Why Disable or Enable Modules?
There are several compelling reasons to customize Tailwind’s module inclusion:
- Performance Optimization: Reducing bundle size by removing unused utilities
- Customization: Tailoring the framework to your specific project needs
- Consistency: Enforcing design system constraints
- Preventing Unnecessary Overrides: Limiting utility classes that might conflict with your design
Configuration Methods for Enabling and Disabling Features
1. Tailwind Configuration File (tailwind.config.js)
The primary method for controlling Tailwind modules is through the tailwind.config.js
file. This JavaScript configuration allows granular control over your project’s Tailwind setup.
Core Approach: Disabling Entire Modules
module.exports = {
corePlugins: {
// Completely disable specific modules
flexbox: false,
grid: false,
backgroundOpacity: false
}
}
Selective Module Enabling
module.exports = {
corePlugins: {
// Only enable specific modules you need
display: true,
position: true,
margin: true
}
}
2. Variants Configuration
Variants allow you to control which responsive, hover, and other state variants are generated for your utilities:
module.exports = {
variants: {
// Control which variants are generated
extend: {
opacity: ['hover', 'focus'],
backgroundColor: ['active', 'odd']
},
// Completely remove specific variants
display: []
}
}
3. Purge (Removal) Configuration
Tailwind’s purge feature allows you to dramatically reduce your CSS file size by removing unused utilities:
module.exports = {
purge: {
// Specify exactly which files to scan for used classes
content: [
'./src/**/*.html',
'./src/**/*.js',
'./src/**/*.jsx',
'./src/**/*.vue'
],
// Specific classes you always want to keep
safelist: [
'bg-red-500',
'text-3xl',
'lg:text-4xl'
]
}
}
4. Using @layer Directive
CSS layers provide another method to control utility generation:
@layer utilities {
/* Custom utilities you want to add */
.content-auto {
content-visibility: auto;
}
}
@layer base {
/* Modify or remove base styles */
h1 {
@apply text-2xl;
}
}
5. Just-in-Time (JIT) Mode Considerations
Tailwind’s JIT mode offers more dynamic control:
module.exports = {
mode: 'jit',
purge: [
// Your template files
],
theme: {
// Customize or remove entire sections
fontSize: {
// Only keep specific font sizes
'sm': '0.875rem',
'base': '1rem',
'xl': '1.25rem'
}
}
}
Advanced Customization Techniques
Programmatic Module Control
For more complex scenarios, you can programmatically control module inclusion:
module.exports = {
plugins: [
function({ addUtilities, theme }) {
const newUtilities = {
// Dynamically add or modify utilities
}
addUtilities(newUtilities)
}
],
// Conditional module inclusion
corePlugins: {
float: process.env.NODE_ENV === 'production' ? false : true
}
}
Performance Considerations
When disabling modules, consider these performance implications:
- Bundle Size: Removing unused modules can significantly reduce CSS file size
- Specificity: Be cautious about removing core utilities that might break layout
- Consistency: Ensure design system remains consistent across components
Common Pitfalls and Best Practices
Best Practices
- Minimal Disabling: Only disable modules you’re absolutely certain you won’t use
- Regular Auditing: Periodically review your configuration
- Use Purge Effectively: Leverage Tailwind’s built-in purge mechanism
- Test Thoroughly: Verify site-wide styling after configuration changes
Potential Issues to Watch
- Unintended Layout Breaks: Disabling core layout utilities can cause unexpected rendering
- Over-optimization: Removing too many utilities can limit design flexibility
- Variant Complexity: Complex variant configurations can increase build complexity
Debugging and Validation
Checking Generated CSS
Use tools like:
- Browser developer tools
npx tailwindcss debug
- Webpack bundle analyzers
Validation Strategies
- Cross-browser testing
- Responsive design checks
- Performance monitoring
Conclusion
Mastering the art of enabling and disabling Tailwind CSS modules empowers developers to create more efficient, performant, and tailored stylesheets. By understanding the various configuration methods, you can optimize your CSS workflow, reduce unnecessary bloat, and maintain a clean, consistent design system.
Remember, the key is balance – customize enough to be efficient, but not so much that you lose the framework’s core benefits.
Additional Resources
Pro Tip: Always keep a backup of your original configuration and test changes incrementally to ensure smooth implementation.
Where is state stored in React