How can I integrate Tailwind CSS with a CSS-in-JS solution like Emotion or Styled Components?
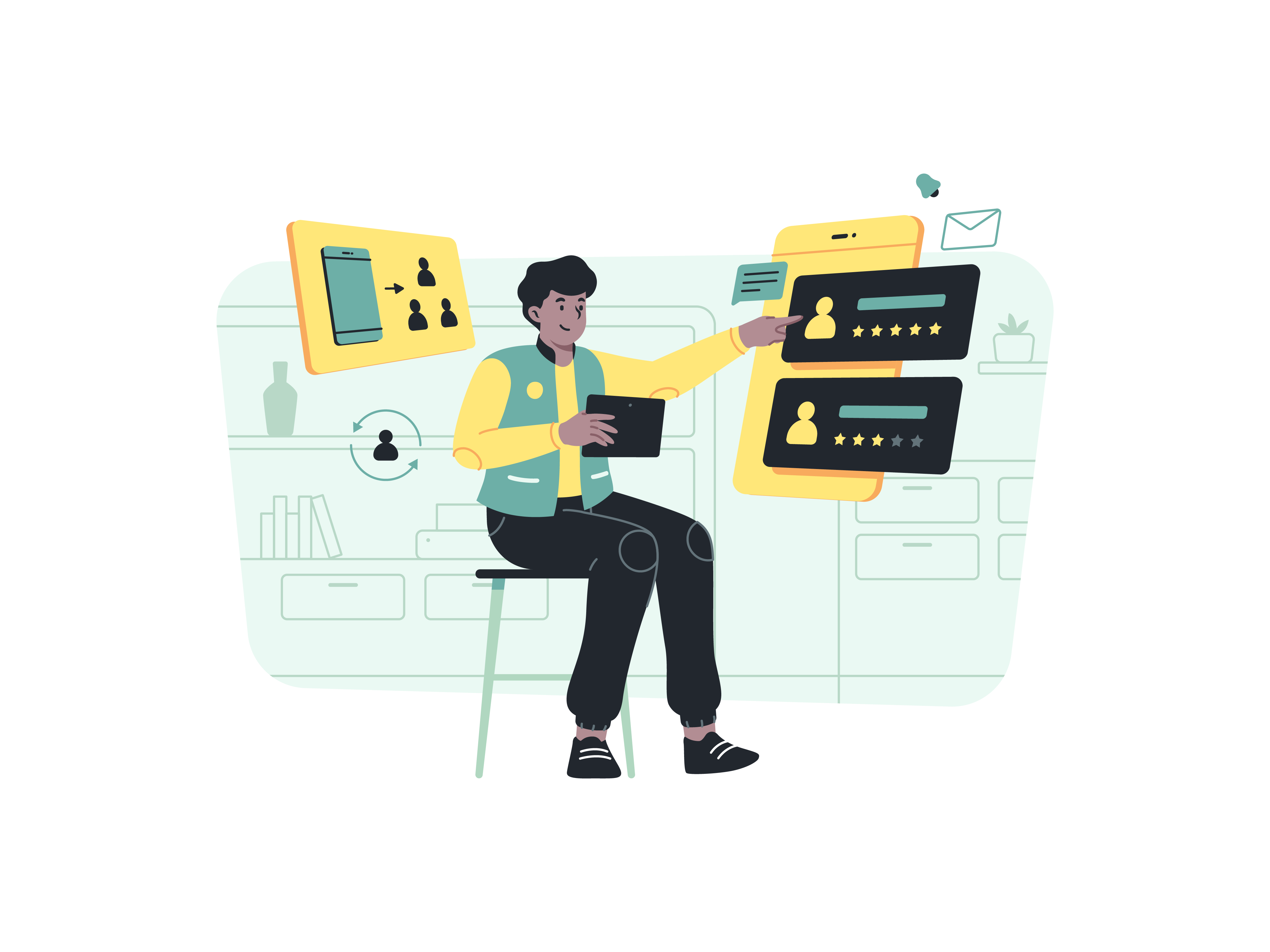
Introduction
Tailwind CSS is a popular CSS framework that simplifies the process of styling web applications by providing pre-defined classes for common design patterns. However, when working on complex projects, it can be challenging to manage stylesheets and maintain consistency across different components. This is where CSS-in-JS solutions like Emotion or Styled Components come into play. In this article, we will explore how to integrate Tailwind CSS with Emotion or Styled Components, providing a step-by-step guide and best practices for implementing the integration. Why Integrate Tailwind with a CSS-in-JS Solution?
Integrating Tailwind CSS with a CSS-in-JS solution like Emotion or Styled Components offers several benefits:
1. Consistency Across Components
CSS-in-JS solutions provide a centralized location for styling components, ensuring consistency across the application. Integrating Tailwind with these solutions allows developers to leverage the power of pre-defined classes while maintaining a consistent stylesheet.
2. Easier Styling Management
Tailwind CSS provides a vast array of pre-defined classes for common design patterns. By integrating Tailwind with Emotion or Styled Components, developers can easily apply these classes to components without manually writing CSS. This approach simplifies the styling process and reduces the likelihood of styling conflicts.
3. Better Performance
Emotion and Styled Components offer better performance compared to traditional CSS solutions. By integrating Tailwind with these solutions, developers can take advantage of the improved performance while maintaining a consistent stylesheet. Steps for Integrating Tailwind with Emotion or Styled Components
To integrate Tailwind CSS with Emotion or Styled Components, follow these steps:
Step 1: Install Tailwind CSS
First, install Tailwind CSS using the following command:
npm install tailwindcss
Step 2: Create a Tailwind Config File
Next, create a tailwind.config.js file in your project’s root directory and add the following code:
module.exports = {
mode: 'jit',
purge: [
'./src/**/*.{js,ts,tsx,jsx}',
'./public/index.html',
],
theme: {
extend: {}, // Add your custom theme here
},
variants: {},
plugins: [],
};
This configuration file sets up Tailwind to use the “jit” mode, which compiles classes to CSS on demand. It also specifies the files to purge from the build and adds a custom theme.
Step 3: Create an Emotion or Styled Components Config File
Create a config file for Emotion or Styled Components, depending on your choice of solution. Here’s an example for Emotion:
import { styled } from '@emotion/styled';
export const theme = {
boxShadows: {
default: '0px 0px 10px rgba(0, 0, 0, 0.2)',
},
typography: {
fontSize: '16px',
fontFamily: `'Open Sans', sans-serif`,
},
colors: {
primary: '#3498DB',
secondary: '#f7b6d2',
danger: '#ff5252',
},
};
This configuration file sets up the default theme for Emotion, including box shadows, typography, and colors.
Step 4: Integrate Tailwind with Emotion or Styled Components
Now, you’re ready to integrate Tailwind with Emotion or Styled Components. Here’s an example for Emotion:
import { styled } from '@emotion/styled';
import { theme } from './tailwind.config.js';
const Container = styled.div`
${theme.boxShadows.default};
padding: 2rem;
`;
export default function App() {
return (
<Container>
<h1>Hello World</h1>
</Container>
);
}
This example imports the theme object from the tailwind.config.js file and passes it to the styled component. The Container component now inherits the box shadow style defined in the theme.
Best Practices for Integrating Tailwind with CSS-in-JS Solutions
When integrating Tailwind with Emotion or Styled Components, follow these best practices:
1. Use a Centralized Theme Configuration File
Store your theme configuration in a centralized file (tailwind.config.js) to ensure consistency across your application. This approach also makes it easier to update the theme or add custom styles.
2. Avoid Overwriting Tailwind Classes
When using Emotion or Styled Components, avoid directly applying Tailwind classes to components. Instead, use the styled
function to create components that inherit the Tailwind styles. This approach ensures consistency and maintainability across your application.
3. Use a Consistent Naming Convention
Use a consistent naming convention for your components and styles. This approach makes it easier to identify and maintain your codebase.
Conclusion
Integrating Tailwind CSS with Emotion or Styled Components offers several benefits, including consistency across components, easier styling management, and better performance. By following the steps outlined in this article and adhering to best practices, you can successfully integrate Tailwind with these CSS-in-JS solutions.