How does React handle dynamic imports
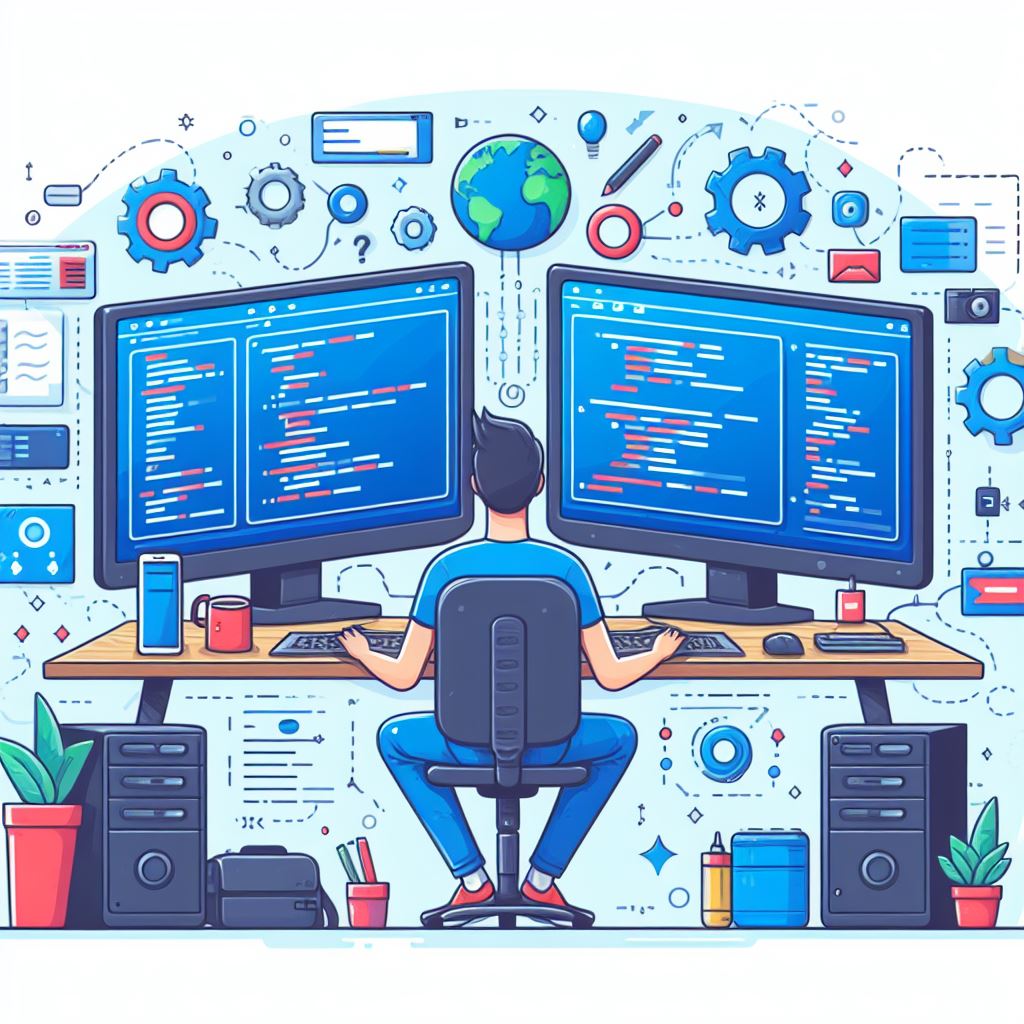
As web applications become more sophisticated, efficient code organization and loading strategies become imperative. React addresses this challenge through dynamic imports, a powerful feature that enables code-splitting. In this comprehensive guide, we’ll delve into how React handles dynamic imports, explore the benefits of code-splitting, and unveil best practices for optimizing performance in your applications.
Understanding Code-Splitting
- Code-Splitting Basics: Code-splitting is the technique of breaking down a large bundle of JavaScript into smaller, more manageable chunks. Instead of loading the entire application code upfront, code-splitting allows loading only the portions required for a particular user interaction or page.
- Benefits of Code-Splitting: Code-splitting enhances the user experience by reducing initial page load times and optimizing resource utilization. This is particularly crucial for large-scale applications where a significant amount of code might not be needed immediately.
Introduction to Dynamic Imports
-
Dynamic Imports in ECMAScript: Dynamic imports are a feature introduced in ECMAScript 6 that allows loading modules on-demand. In React, dynamic imports are often used for code-splitting, enabling the loading of components, libraries, or other resources only when they are required.
// Dynamic Import Syntax const MyComponent = React.lazy(() => import('./MyComponent'));
-
React.lazy and Suspense: React provides the
React.lazy
function for creating dynamically imported components. When combined with theSuspense
component, React allows rendering fallback content (such as loading spinners) while waiting for the dynamically imported component to load.
Implementing Dynamic Imports in React
-
Using React.lazy: The
React.lazy
function takes a function that returns a dynamic import. This function should resolve to a module with a default export containing the React component.// Dynamic Import with React.lazy const MyComponent = React.lazy(() => import('./MyComponent'));
-
Rendering with Suspense: To use the dynamically imported component, wrap it in a
Suspense
component. This enables rendering fallback content until the dynamically imported component is ready.// Using Dynamically Imported Component with Suspense const MyComponentWrapper = () => ( <React.Suspense fallback={<LoadingSpinner />}> <MyComponent /> </React.Suspense> );
Dynamic Imports with Named Exports
-
Named Exports in Dynamic Imports: Dynamic imports can also handle named exports by specifying the desired export name in the import statement. This is useful when dynamically importing functions, utilities, or constants.
// Dynamic Import with Named Exports const { myFunction } = await import('./myModule');
-
React.lazy with Named Exports: When using
React.lazy
with named exports, the syntax remains similar. The dynamically imported module should have a default export containing the React component and named exports for other elements.// Dynamic Import with Named Exports in React.lazy const { MyComponent, myFunction } = React.lazy(() => import('./myModule'));
Optimizing Performance with Webpack
-
Webpack Code-Splitting Configuration: Webpack, a popular JavaScript bundler, plays a crucial role in optimizing code-splitting. Webpack’s configuration allows specifying how chunks should be generated and loaded, optimizing performance based on the application’s needs.
// Webpack Dynamic Import Configuration module.exports = { // ... other configurations optimization: { splitChunks: { chunks: 'all', }, }, };
-
Bundle Analysis Tools: Utilize bundle analysis tools like Webpack Bundle Analyzer to visualize the size and composition of your bundles. This helps identify opportunities for further code-splitting and optimization.
Combining Dynamic Imports with React Router
-
React Router and Code-Splitting: React Router, a popular routing library for React, seamlessly integrates with code-splitting. By associating each route with a dynamically imported component, you can ensure that only the necessary code is loaded for each route.
// React Router Dynamic Import const Home = React.lazy(() => import('./Home')); const About = React.lazy(() => import('./About')); // React Router Configuration const App = () => ( <Router> <Switch> <Route path="/" exact component={Home} /> <Route path="/about" component={About} /> </Switch> </Router> );
-
Enhancing User Experience: By combining React Router with dynamic imports, you enhance the user experience by loading specific components only when users navigate to the corresponding routes, reducing initial page load times.
Handling Errors with Error Boundaries
-
Error Boundaries in React: When working with dynamic imports, it’s essential to handle errors that may occur during the loading process. React provides Error Boundaries, components that catch JavaScript errors anywhere in their child component tree.
// Error Boundary Component class ErrorBoundary extends React.Component { constructor(props) { super(props); this.state = { hasError: false }; } static getDerivedStateFromError(error) { return { hasError: true }; } componentDidCatch(error, errorInfo) { logErrorToService(error, errorInfo); } render() { if (this.state.hasError) { return <FallbackUI />; } return this.props.children; } }
-
Wrapping Dynamically Imported Components: Wrap dynamically imported components with the Error Boundary component to gracefully handle any errors that may occur during the loading or rendering process.
Browser Support and Polyfills
-
Browser Support for Dynamic Imports: Dynamic imports are supported in modern browsers, but for compatibility with older browsers, consider using polyfills or transpilers like Babel. Babel allows transforming dynamic imports into a format supported by a wider range of browsers.
// Babel Plugin for Dynamic Imports const MyComponent = await import(/* webpackChunkName: "myComponent" */ './MyComponent');
-
Polyfilling Dynamic Imports: Include a polyfill for dynamic imports in your project to ensure compatibility with browsers that don’t support this feature natively.
// Dynamic Imports Polyfill import('dynamic-import-polyfill').then(() => { // Now dynamic imports are supported });
Testing and Debugging Dynamic Imports
- Testing Dynamically Imported Components: When writing tests
for components that are dynamically imported, tools like Jest and testing libraries like React Testing Library provide methods for handling asynchronous rendering and code-splitting.
```jsx
// Jest Test for Dynamically Imported Component
test('renders MyComponent', async () => {
const { findByText } = render(
<React.Suspense fallback="Loading...">
<MyComponent />
</React.Suspense>
);
expect(await findByText('Hello World!')).toBeInTheDocument();
});
```
- Debugging Dynamic Imports: Debugging dynamically imported components can be challenging. Use browser developer tools to inspect network requests and loaded chunks. Additionally, leverage tools like React DevTools for React-specific debugging.
Conclusion
Dynamic imports in React, fueled by the combination of React.lazy, Suspense, and tools like Webpack, empower developers to optimize their applications for performance and efficiency. By strategically splitting code and loading components on-demand, you can significantly improve the user experience, reduce initial page load times, and create more responsive and scalable React applications. As the landscape of web development continues to evolve, mastering the art of dynamic imports becomes a key skill for developers seeking to build cutting-edge and performant React applications.
- What is JSX in React
- How many states are there in React
- Is Redux still relevant 2023
- Where is state stored in React
- Why Babel is used in React
- Why React is better than JSX
- Why use React without JSX
- What is frontend state
- What is difference between variable and state in React
- What is useEffect in React
- Why we should never update React state directly