How do you handle authentication in a React-Redux application
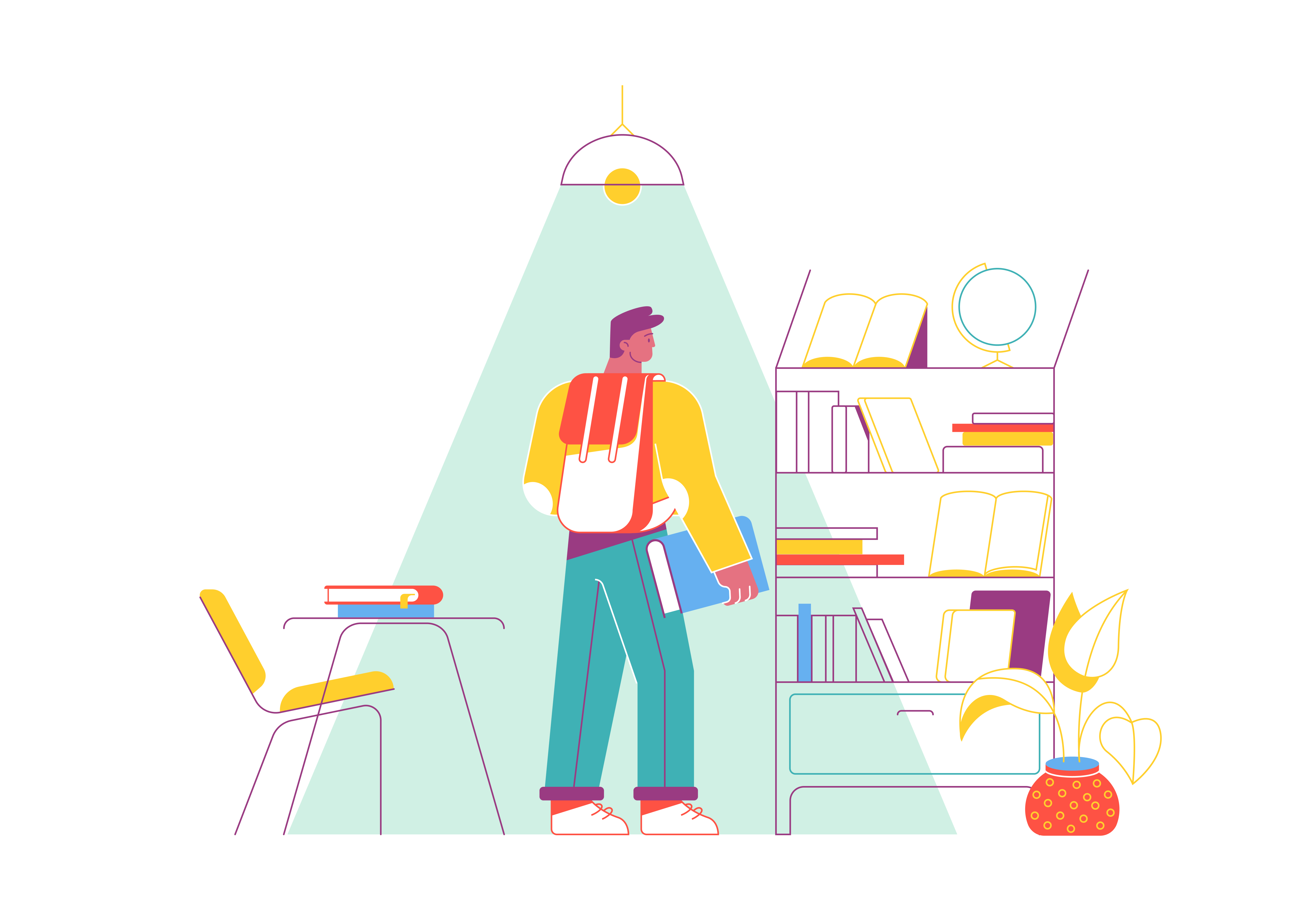
Authentication is a crucial aspect of web development, ensuring that only authorized users access certain features or data. In React-Redux applications, handling authentication involves integrating authentication workflows seamlessly with state management. In this comprehensive guide, we’ll explore the best practices, tools, and strategies for implementing secure and efficient authentication in React-Redux applications.
-
Understanding Authentication in Web Development
- Authentication Basics: Authentication involves verifying the identity of users. In web development, it typically revolves around validating usernames and passwords, often with additional security measures like two-factor authentication.
- Token-Based Authentication: Token-based authentication, using technologies like JSON Web Tokens (JWT), has become a prevalent method. It involves issuing a token upon successful login, which is then included in subsequent requests for authorization.
-
Setting Up a React-Redux Application
- Creating a React-Redux Project: Begin by setting up a React-Redux project using tools like Create React App. This provides a foundation for integrating authentication seamlessly into your application.
- Installing Necessary Packages:
Install required packages, such as
redux
andreact-redux
, to manage the application’s state globally. Additionally, you may need libraries likeaxios
for making HTTP requests andjwt-decode
for decoding JWT tokens.
-
Implementing User Authentication
-
User Registration and Login: Create components and actions for user registration and login. These components will interact with your backend server, typically sending requests to an authentication endpoint.
// Example of User Login Action const loginUser = (credentials) => { return async (dispatch) => { try { const response = await axios.post('/api/login', credentials); const { token } = response.data; dispatch({ type: 'LOGIN_SUCCESS', payload: token }); } catch (error) { dispatch({ type: 'LOGIN_FAILURE', payload: error.message }); } }; };
-
Token Storage: Upon successful login, store the authentication token securely, often in browser storage such as
localStorage
orsessionStorage
. This allows the application to maintain the user’s authentication state across sessions.
-
-
Integrating Authentication with Redux
-
Redux Reducers and Actions: Create Redux reducers and actions dedicated to handling authentication state. Actions should cover login success and failure, user logout, and any other relevant authentication events.
// Example of Authentication Reducer const authReducer = (state = initialState, action) => { switch (action.type) { case 'LOGIN_SUCCESS': return { ...state, isAuthenticated: true, token: action.payload }; case 'LOGIN_FAILURE': return { ...state, isAuthenticated: false, error: action.payload }; case 'LOGOUT': return { ...state, isAuthenticated: false, token: null }; default: return state; } };
-
Protected Routes: Implement logic to protect routes that require authentication. This involves checking the authentication state before rendering certain components or redirecting users to a login page if they are not authenticated.
-
-
Securing API Requests
-
Sending Authenticated Requests: Ensure that authenticated users send their tokens with each API request. This may involve creating an Axios interceptor to automatically attach the authentication token to outgoing requests.
// Axios Interceptor for Authentication axios.interceptors.request.use( (config) => { const token = localStorage.getItem('token'); if (token) { config.headers.Authorization = `Bearer ${token}`; } return config; }, (error) => { return Promise.reject(error); } );
-
Handling Token Expiry: Implement a mechanism to handle token expiry. When a token expires, users should be prompted to reauthenticate. This can be achieved through refreshing tokens or requesting a new token upon expiry.
-
-
Handling Authentication Errors
-
Global Error Handling: Implement global error handling to capture authentication-related errors. For instance, handle cases where the server responds with a 401 Unauthorized status code.
// Axios Interceptor for Error Handling axios.interceptors.response.use( (response) => response, (error) => { if (error.response.status === 401) { // Handle unauthorized access } return Promise.reject(error); } );
-
User Feedback: Provide meaningful feedback to users when authentication fails or when they attempt to access protected resources without proper authorization. This enhances the user experience and helps them understand the reason for authentication issues.
-
-
Implementing Refresh Tokens
-
Refresh Token Flow: For long-lived sessions, consider implementing a refresh token flow. This involves issuing short-lived access tokens and long-lived refresh tokens. When an access token expires, use the refresh token to obtain a new one without requiring the user to log in again.
// Example of Refresh Token Request const refreshAccessToken = async (refreshToken) => { try { const response = await axios.post('/api/refresh-token', { refreshToken }); const newAccessToken = response.data.accessToken; // Update the stored token with the new access token localStorage.setItem('token', newAccessToken); return newAccessToken; } catch (error) { // Handle refresh token failure throw new Error('Unable to refresh access token'); } };
-
-
Logging Out Users
-
User Logout Action: Implement a user logout action that clears the authentication token from storage and updates the authentication state in the Redux store.
// Example of Logout Action const logoutUser = () => { return (dispatch) => { localStorage.removeItem('token'); dispatch({ type: 'LOGOUT' }); }; };
-
Redirecting on Logout: Redirect users to the login page upon logout to ensure they can’t access protected routes without proper authentication.
-
-
Best Practices and Security Considerations
- Use HTTPS: Always use HTTPS to encrypt data transmitted between the client and server, preventing unauthorized interception.
- Secure Token Storage: Store authentication tokens securely, and consider using features like HttpOnly cookies for additional security.
- Token Expiry: Set appropriate expiration times for tokens to limit their validity, reducing the risk associated with compromised tokens.
- Protect Against Cross-Site Request Forgery (CSRF): Implement measures to protect against CSRF attacks, such as including anti-CSRF tokens in your application.
-
Conclusion
Navigating the seas of authentication in React-Redux applications involves careful planning, implementation of best practices, and consideration of security measures. By integrating user authentication seamlessly with the state management capabilities of Redux, developers can ensure a secure and efficient authentication process. As the landscape of web development evolves, staying informed about the latest authentication strategies is paramount for building robust and user-friendly React-Redux applications.
How to use Bootstrap’s utility classes for flexbox alignment
How does React differ from other JavaScript frameworks
How do I install Tailwind CSS in my project
How do I integrate Tailwind CSS with a JavaScript framework like React or Vue.js
How do I create a responsive layout with Tailwind CSS
How do I vertically center an element in Tailwind CSS
How do I customize the spacing like padding and margin between elements in Tailwind CSS