How to use Bootstrap's navigation components
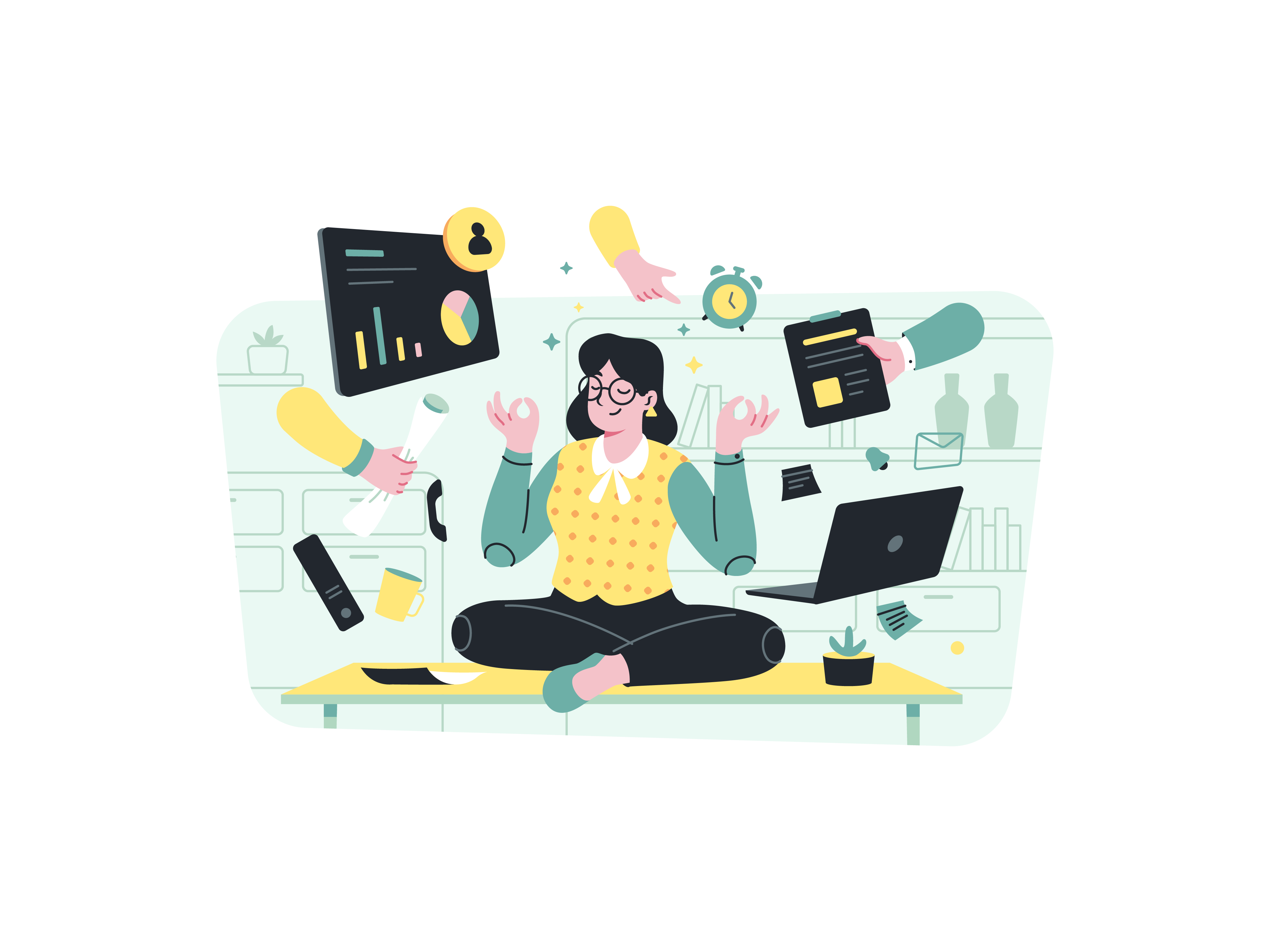
Effective navigation is a cornerstone of user-friendly web design. Bootstrap, the widely-used front-end framework, provides an array of navigation components that can elevate your website’s user experience. In this comprehensive guide, we’ll explore how to leverage Bootstrap’s navigation elements, including tabs and pills, to create sleek, interactive, and intuitive navigation systems.
Understanding Bootstrap Navigation Components
Before we dive into the practical details, let’s familiarize ourselves with Bootstrap’s navigation components. Tabs and pills are visually appealing ways to present content and enable users to switch between different sections seamlessly. Tabs are typically used for horizontal navigation, while pills work well for vertical or stacked navigation. These components not only enhance aesthetics but also improve accessibility and user engagement.
Getting Started with Bootstrap Tabs and Pills
To begin working with Bootstrap’s navigation components, ensure you have Bootstrap integrated into your project. You can either download Bootstrap and host it locally or use a Content Delivery Network (CDN). Here’s an example of including Bootstrap via CDN:
<!DOCTYPE html>
<html>
<head>
<!-- Include Bootstrap CSS via CDN -->
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css">
<title>Bootstrap Navigation Components</title>
</head>
<body>
<!-- Your content goes here -->
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
With Bootstrap integrated, you’re ready to dive into creating engaging navigation systems.
Creating Tabs for Horizontal Navigation
Tabs are excellent for organizing content in horizontal navigation menus. They allow users to switch between sections without loading new pages.
Basic Tab Structure
To create a basic tab structure, use the .nav
and .nav-tabs
classes along with the .nav-item
and .nav-link
classes for individual tabs.
<ul class="nav nav-tabs" id="myTabs" role="tablist">
<li class="nav-item" role="presentation">
<a class="nav-link active" id="home-tab" data-bs-toggle="tab" href="#home" role="tab" aria-controls="home" aria-selected="true">Home</a>
</li>
<li class="nav-item" role="presentation">
<a class="nav-link" id="profile-tab" data-bs-toggle="tab" href="#profile" role="tab" aria-controls="profile" aria-selected="false">Profile</a>
</li>
<!-- Additional tabs -->
</ul>
<div class="tab-content" id="myTabContent">
<div class="tab-pane fade show active" id="home" role="tabpanel" aria-labelledby="home-tab">Home content</div>
<div class="tab-pane fade" id="profile" role="tabpanel" aria-labelledby="profile-tab">Profile content</div>
<!-- Additional tab content -->
</div>
In this example, each tab has a corresponding content section defined by the tab-pane
class. The data-bs-toggle
attribute specifies that clicking the link toggles the tab content.
Styling Tabs and Pills
Bootstrap offers various styling options for tabs and pills to match your website’s design aesthetic.
Horizontal and Vertical Pills
Horizontal pills are the default style, but you can create vertical pills by adding the .flex-column
class to the .nav
element.
<ul class="nav nav-pills flex-column" id="myPills" role="tablist">
<!-- Pill items -->
</ul>
Custom Styles and Colors
Bootstrap allows you to apply custom styles and colors to navigation components using contextual classes such as .nav-primary
, .nav-secondary
, and so on.
JavaScript Interaction
JavaScript can enhance the interaction of tabs and pills, allowing you to programmatically switch between tabs or perform actions when tabs are clicked.
<script>
$(document).ready(function() {
$('#myTabs a').on('click', function (e) {
e.preventDefault();
$(this).tab('show');
});
});
</script>
In this example, clicking a tab triggers the show
event and switches to the corresponding content.
Conclusion
Bootstrap’s navigation components provide an elegant solution for creating organized and user-friendly navigation systems on your website. By mastering the use of tabs and pills, you can present content in an interactive and visually appealing manner, enhancing user engagement and experience. From basic navigation structures to customized styles and JavaScript interactions, Bootstrap’s navigation components offer a wealth of options to cater to your design needs. Start implementing these techniques in your projects and guide your users seamlessly through your website’s content.