How to create a sticky sidebar with Bootstrap
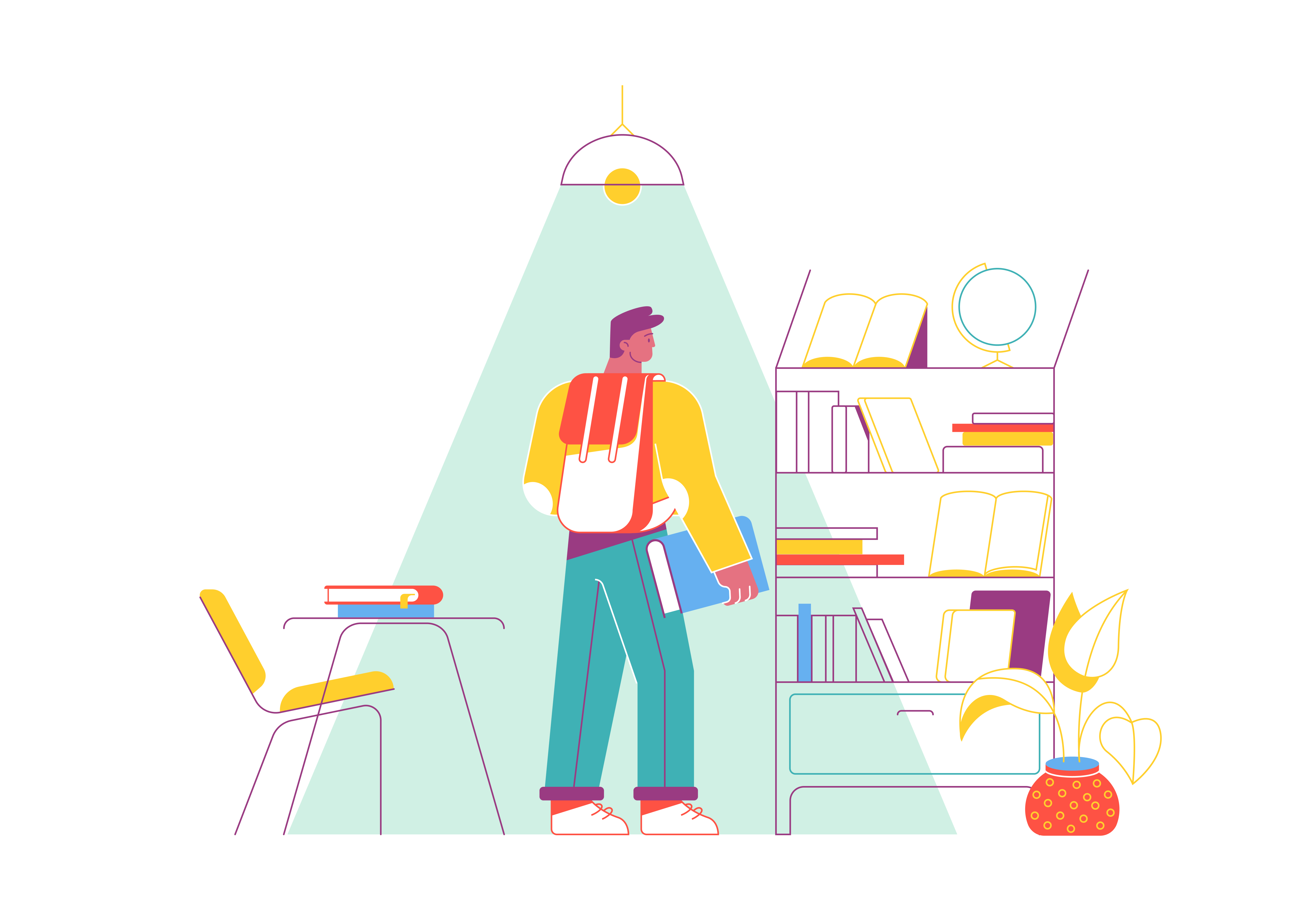
Sticky sidebars are a popular web design element that can significantly enhance user experience by keeping important content or navigation readily accessible as users scroll through a page. Bootstrap, a leading front-end framework, provides the tools and components to create a sticky sidebar with ease. In this comprehensive guide, we’ll walk you through the step-by-step process of creating a sticky sidebar using Bootstrap, helping you improve the functionality and usability of your website.
Understanding the Concept
Before we dive into the implementation, let’s first understand what a sticky sidebar is and why it’s valuable.
Sticky Sidebar
A sticky sidebar is a sidebar element on a web page that remains visible on the screen even as users scroll down the page. This feature is particularly useful for displaying navigation menus, call-to-action buttons, or any other content that you want users to access conveniently without having to scroll back to the top of the page.
Why Use a Sticky Sidebar
Using a sticky sidebar offers several benefits:
- Improved Navigation: It provides easy access to important links, reducing the need for users to scroll back up to the top of the page.
- Enhanced User Engagement: Sticky sidebars can display calls to action, advertisements, or related content, increasing user interaction.
- Better User Experience: It simplifies the browsing experience, especially on long pages, making it more user-friendly and efficient.
Now that we understand the concept and benefits, let’s proceed with creating a sticky sidebar using Bootstrap.
Getting Started
Before you start creating a sticky sidebar, ensure that you have Bootstrap integrated into your project. You can either download Bootstrap and include the necessary CSS and JavaScript files or use the Bootstrap CDN for faster access.
Creating the HTML Structure
To create a sticky sidebar, you’ll need to structure your HTML in a specific way. Here’s a basic example:
<div class="container">
<div class="row">
<div class="col-lg-3">
<!-- Your sidebar content goes here -->
<div class="sticky-top">
<!-- Sidebar content -->
</div>
</div>
<div class="col-lg-9">
<!-- Main content goes here -->
</div>
</div>
</div>
In this structure:
.container
and.row
are Bootstrap’s grid system classes for creating a responsive layout..col-lg-3
and.col-lg-9
define the columns for the sidebar and main content, respectively.- The
sticky-top
class is the key to making the sidebar sticky. It ensures that the sidebar sticks to the top of the viewport as users scroll down the page.
Styling the Sidebar
You can style the sidebar to match your website’s design and branding. Here’s an example of CSS to style the sidebar:
/* Style for the sidebar */
.sticky-top {
position: -webkit-sticky;
position: sticky;
top: 20px; /* Adjust this value to set the distance from the top of the viewport */
padding: 15px;
background-color: #f8f9fa; /* Sidebar background color */
border: 1px solid #e0e0e0; /* Sidebar border */
border-radius: 5px; /* Rounded corners for the sidebar */
}
/* Style for sidebar content */
.sticky-top h3 {
font-size: 18px;
margin-bottom: 10px;
}
/* Add more styles as needed */
In this CSS code:
- The
position: sticky;
property ensures that the sidebar sticks to the top of the viewport when users scroll. top
determines how far from the top of the viewport the sidebar should start sticking. Adjust this value to your preference.- Various styles for background color, border, and typography are applied to the sidebar content.
JavaScript for Smooth Scrolling (Optional)
To enhance the user experience, you can add smooth scrolling behavior when users click on links in the sticky sidebar. Here’s a simple JavaScript example:
$(document).ready(function () {
// Smooth scrolling for anchor links in the sidebar
$('.sticky-top a[href^="#"]').on('click', function (event) {
event.preventDefault();
$('html, body').animate(
{
scrollTop: $($.attr(this, 'href')).offset().top
},
800
);
});
});
This JavaScript code uses jQuery to create a smooth scrolling effect for anchor links in the sticky sidebar. It’s optional but can enhance the user experience.
Testing and Refining
After implementing your sticky sidebar, thoroughly test it on various devices and screen sizes to ensure it functions as expected. Make adjustments as needed to refine the appearance and behavior.
Conclusion
Creating a sticky sidebar with Bootstrap can significantly improve the user experience on your website by providing easy access to important content or navigation. By following the steps outlined in this guide and customizing the design to match your branding, you can create a more efficient and user-friendly browsing experience for your audience.