How to implement a scrollspy with Bootstrap
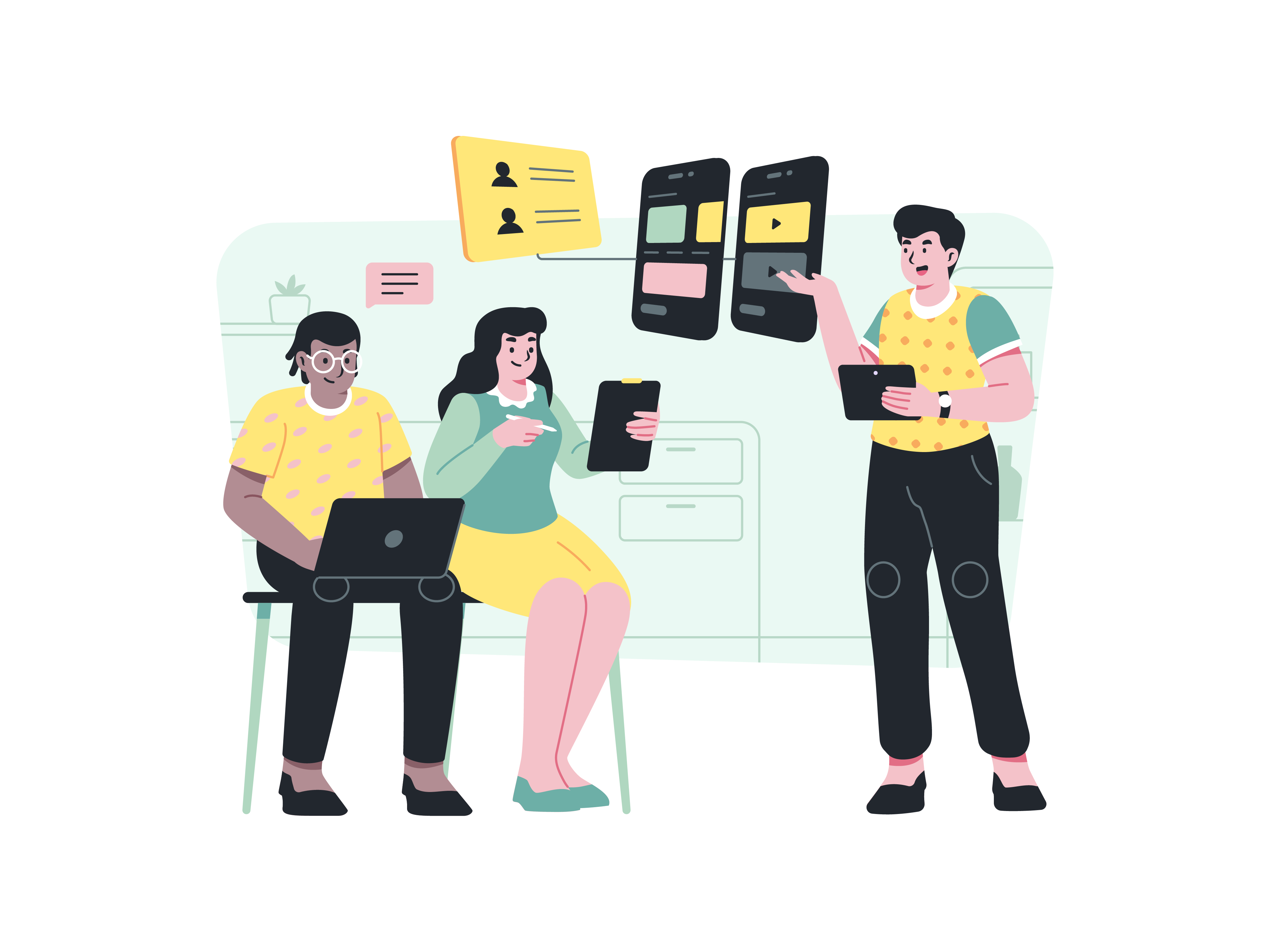
Scrollspy is a powerful navigation tool that can greatly improve the user experience on websites with long or scrollable content. It allows users to easily navigate to different sections of a page by highlighting the corresponding navigation links as they scroll through the content. Bootstrap, a leading front-end framework, provides a built-in Scrollspy component that simplifies the implementation of this feature. In this comprehensive guide, we’ll walk you through the step-by-step process of implementing a Scrollspy with Bootstrap, helping you enhance navigation and improve user engagement on your website.
Understanding the Concept
Before we dive into the practical implementation, let’s first understand what Scrollspy is and why it’s valuable.
Scrollspy
Scrollspy is a user interface pattern that automatically highlights or updates navigation links or elements as users scroll down a web page. It provides users with a clear indication of their current position on the page and allows them to easily jump to different sections.
Why Use Scrollspy
Scrollspy offers several benefits:
- Improved Navigation: It enhances navigation on long pages, making it easier for users to find and access specific sections or content.
- User Engagement: By providing a visual indication of the content structure, Scrollspy encourages users to explore more of your content.
- Smoother Scrolling: It creates a smoother and more intuitive scrolling experience by reducing the need for manual scrolling and searching.
Now that we understand the concept and benefits, let’s proceed with implementing Scrollspy using Bootstrap.
Getting Started
To begin implementing Scrollspy with Bootstrap, you need to have Bootstrap integrated into your project. You can either download Bootstrap and include the necessary CSS and JavaScript files or use the Bootstrap CDN for faster access.
Creating the HTML Structure
To use Scrollspy, you’ll need to structure your HTML in a specific way. Here’s a basic example:
<nav id="navbar-example" class="navbar">
<ul class="nav">
<li class="nav-item">
<a class="nav-link" href="#section1">Section 1</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#section2">Section 2</a>
</li>
<li class="nav-item">
<a class="nav-link" href="#section3">Section 3</a>
</li>
<!-- Add more navigation links as needed -->
</ul>
</nav>
<div data-bs-spy="scroll" data-bs-target="#navbar-example" data-bs-offset="0">
<div id="section1">
<!-- Content for Section 1 -->
</div>
<div id="section2">
<!-- Content for Section 2 -->
</div>
<div id="section3">
<!-- Content for Section 3 -->
</div>
<!-- Add more content sections as needed -->
</div>
In this structure:
- The
<nav>
element contains navigation links withhref
attributes pointing to the corresponding content sections. - The
<div>
withdata-bs-spy
,data-bs-target
, anddata-bs-offset
attributes enables the Scrollspy functionality.
Activating Scrollspy with JavaScript
To activate Scrollspy, add the following JavaScript code to your page:
<script>
var scrollSpy = new bootstrap.ScrollSpy(document.body, {
target: '#navbar-example'
});
</script>
This code initializes the Scrollspy on the document.body
element and specifies the target as #navbar-example
, which matches the id
of your navigation component.
Styling the Navigation
You can style the navigation links to make them visually appealing. Here’s a basic example:
.navbar {
background-color: #333;
}
.nav-link {
color: white;
padding: 10px;
font-weight: bold;
text-decoration: none;
}
.nav-link:hover {
background-color: #555;
border-radius: 5px;
transition: background-color 0.3s ease;
}
In this CSS code:
- The
.navbar
class styles the navigation bar’s background color. .nav-link
styles the navigation links, including color, padding, font-weight, and text-decoration..nav-link:hover
styles the links on hover to provide feedback to users.
Testing and Refining
After implementing Scrollspy, thoroughly test it on various devices and screen sizes to ensure it functions as expected. Make adjustments as needed to refine the appearance and behavior.
Conclusion
Implementing Scrollspy with Bootstrap is a powerful way to enhance navigation and improve the user experience on your website, especially for long or content-heavy pages. By following the steps outlined in this guide and customizing the design to match your branding, you can create a more user-friendly and engaging browsing experience for your audience.