How can I optimize the performance of my website built with Tailwind CSS
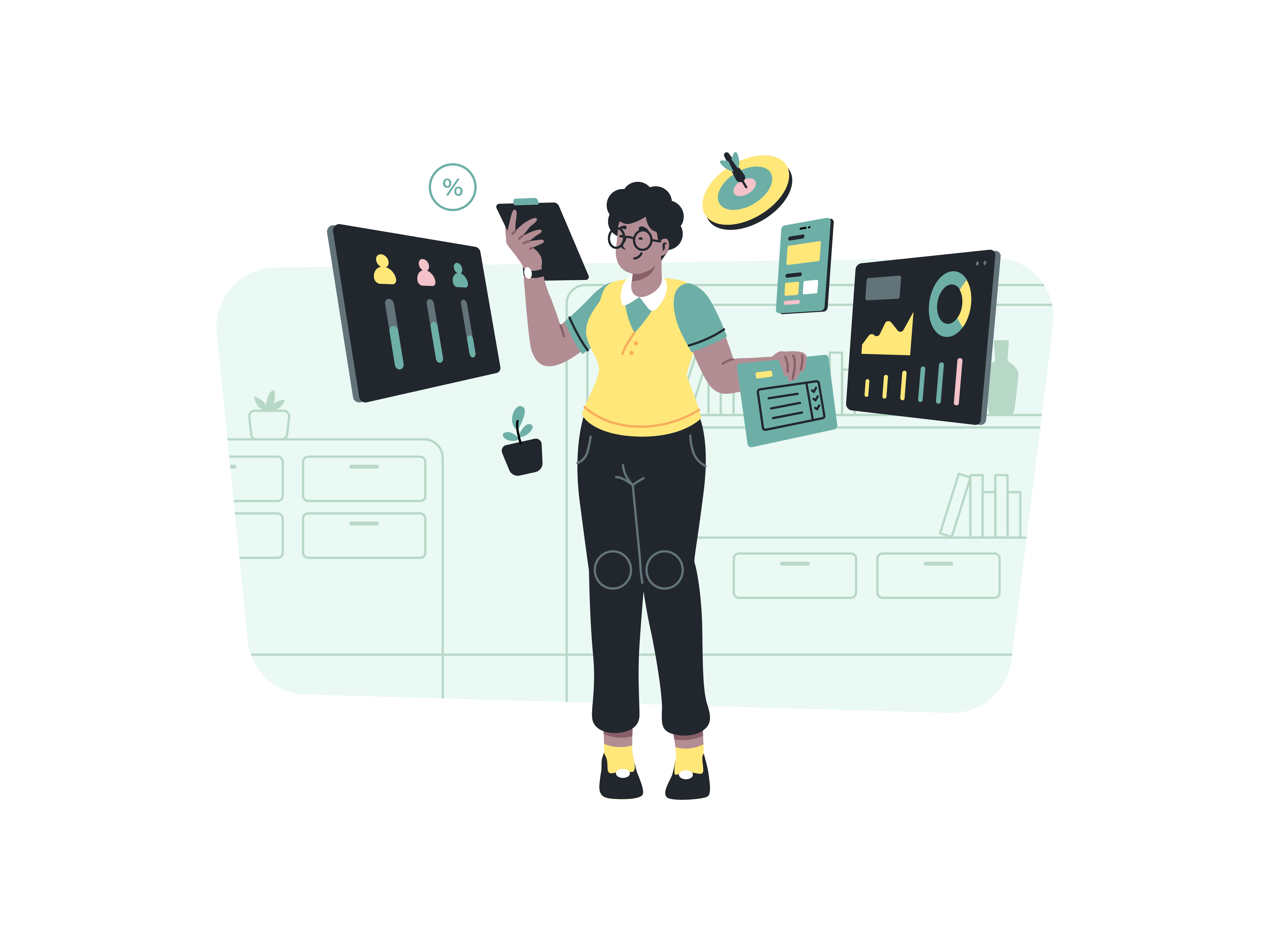
Tailwind CSS has revolutionized front-end development with its utility-first approach, offering developers unprecedented flexibility and rapid design capabilities. However, with great power comes great responsibility—particularly when it comes to website performance. This comprehensive guide will dive deep into five critical strategies for optimizing the performance of your Tailwind CSS-powered website.
1. Leveraging Content Delivery Networks (CDNs): Supercharging Your Website’s Global Performance
Content Delivery Networks (CDNs) are far more than just a technical nicety—they are a fundamental performance optimization strategy that can dramatically transform your website’s speed and user experience. At its core, a CDN works by distributing your website’s static content across multiple servers located in different geographical regions.
How CDNs Actually Work
When a user visits your website, the CDN serves content from the server closest to their physical location. This means a user in Tokyo will retrieve files from a nearby Asian server, while a user in New York connects to a North American server. The result? Dramatically reduced latency and faster load times.
Implementing CDN with Tailwind CSS
There are multiple approaches to integrating a CDN with your Tailwind CSS project:
- External CDN Hosting
<!-- Loading Tailwind CSS from a CDN -->
<link href="https://cdn.jsdelivr.net/npm/tailwindcss@2.2.19/dist/tailwind.min.css" rel="stylesheet">
- Self-Hosted CDN Solutions Many cloud providers offer CDN services that can be configured to serve your Tailwind CSS files:
- Cloudflare
- Amazon CloudFront
- Google Cloud CDN
- Azure CDN
Performance Metrics to Consider
- Time to First Byte (TTFB): Measure how quickly the first byte of content is received
- First Contentful Paint (FCP): Track when the first content appears on screen
- Total Load Time: Comprehensive measure of complete page load
Advanced CDN Optimization Techniques
- Implement cache-control headers
- Use versioned CDN links for consistent caching
- Utilize stale-while-revalidate caching strategies
- Configure edge caching for dynamic content
2. Minification and Compression: Reducing Payload for Lightning-Fast Experiences
Code minification is not just about reducing file size—it’s about creating lean, efficient web experiences that load almost instantaneously. Tailwind CSS generates extensive utility classes, making minification crucial.
Minification Strategies
Tailwind CSS CLI Minification
# Minify Tailwind CSS output
npx tailwindcss -i ./src/input.css -o ./dist/output.css --minify
Webpack Configuration
module.exports = {
optimization: {
minimize: true,
minimizer: [
new CssMinimizerPlugin(),
new TerserPlugin()
]
}
}
Compression Techniques
Gzip Compression
- Reduces file size by up to 70%
- Supported by most web servers
- Minimal performance overhead
Brotli Compression
- Advanced compression algorithm
- 15-20% better compression than Gzip
- Requires modern browser support
Nginx Configuration Example
# Enable Brotli compression
brotli on;
brotli_comp_level 6;
brotli_types text/plain text/css application/javascript;
Tools for Effective Minification
- PostCSS
- Terser
- webpack-bundle-analyzer
- cssnano
3. Strategic Caching: Minimizing Redundant Resource Requests
Caching is the silent performance hero of web development. With Tailwind CSS, intelligent caching can significantly reduce load times and server strain.
Browser Caching Fundamentals
# Effective Cache-Control Header
Cache-Control: public, max-age=31536000, immutable
Tailwind-Specific Caching Strategies
- Implement component-level caching
- Use framework-specific memoization techniques
- Leverage service workers for advanced caching
React Memoization Example
const MemoizedComponent = React.memo(({ props }) => {
// Tailwind-styled component that only re-renders when props change
});
Caching Best Practices
- Version static assets
- Implement progressive loading
- Use appropriate cache-control headers
- Monitor cache hit rates
4. PurgeCSS: Eliminating Unused CSS Ruthlessly
PurgeCSS is a powerful tool that removes unused CSS, dramatically reducing stylesheet size—critical for Tailwind CSS projects.
Configuring PurgeCSS in Tailwind
// tailwind.config.js
module.exports = {
purge: {
content: [
'./src/**/*.html',
'./src/**/*.js',
'./src/**/*.vue'
],
options: {
safelist: [/^bg-/, /^text-/]
}
}
}
Advanced PurgeCSS Techniques
- Configure precise content paths
- Use safelisting for dynamic classes
- Regularly audit unused CSS
- Test thoroughly to prevent style removal
Potential Pitfalls
- Accidentally removing critical styles
- Incomplete content path configuration
- Performance overhead during build process
5. Avoiding Over-Engineering: The Art of Minimalist Design
Over-engineering is the silent performance killer. With Tailwind’s extensive utility classes, restraint becomes a critical optimization strategy.
Design Simplification Principles
- Limit color palette
- Create consistent spacing systems
- Define minimal typography rules
- Prioritize core functionality
Example of Constrained Design System
// tailwind.config.js
module.exports = {
theme: {
colors: {
primary: '#3B82F6',
secondary: '#10B981',
neutral: {
100: '#F3F4F6',
900: '#111827'
}
},
spacing: {
xs: '0.5rem',
sm: '1rem',
md: '1.5rem',
lg: '2rem'
}
}
}
Performance Budgeting
- Set strict CSS file size limits
- Use Lighthouse for performance auditing
- Conduct regular performance reviews
- Implement continuous monitoring
Conclusion: Performance as a Continuous Journey
Optimizing a Tailwind CSS website is not a one-time task but an ongoing commitment to delivering exceptional user experiences. By strategically implementing CDN technologies, aggressive minification, intelligent caching, precise CSS purging, and maintaining design simplicity, you can create websites that are not just visually stunning but blazingly fast.
How can you deploy a Next.js application
How can you implement global CSS styles in a Next.js project
How can you implement pagination in a Next.js application
What is the purpose of the useContext hook in React
How can you handle authentication in a Next.js application
How can you set up and use environment variables in a Next.js project