Explain the purpose of the next.config.js option target
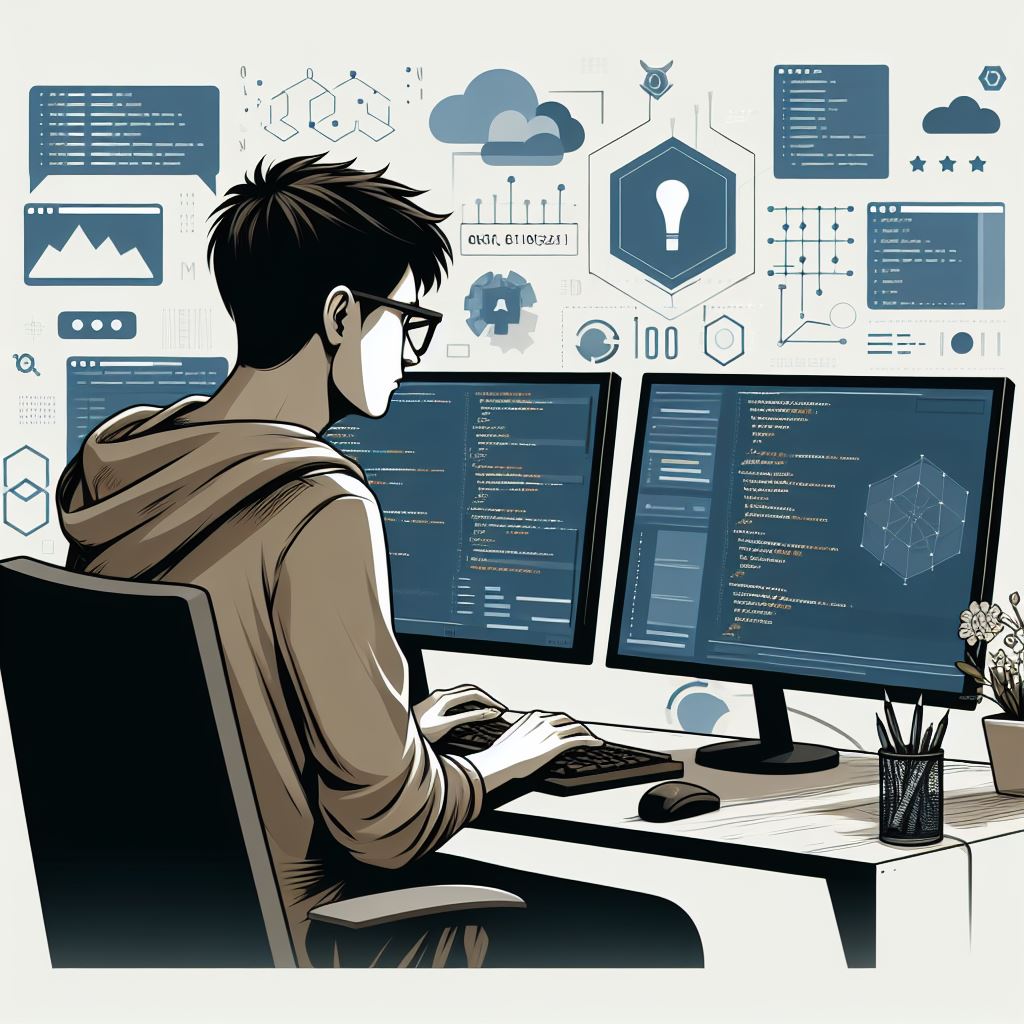
In the realm of Next.js configuration, the next.config.js
file stands as a gateway to tailor the behavior of your application. Among its myriad options, the target
configuration holds a pivotal role, influencing how your Next.js application is compiled and executed. In this blog post, we’ll embark on a journey to unravel the purpose of the target
option, understanding its significance and exploring scenarios where its manipulation can be a game-changer.
Understanding the next.config.js
File
Before delving into the intricacies of the target
option, let’s establish a foundational understanding of the next.config.js
file. This configuration file serves as a central hub for customizing various aspects of your Next.js application, offering a way to override the default settings and tailor the build process to your specific needs.
// next.config.js
module.exports = {
// Other configurations go here
};
Now, let’s focus on the star of the show - the target
option.
The Role of target
in Next.js
The target
option in next.config.js
defines the environment in which your Next.js application will run. It plays a crucial role in determining how your code is compiled and executed, aligning with the deployment platform or server environment.
Default Value
By default, the target
is set to 'server'
, indicating that the application will be built as a server-rendered app. This is suitable for scenarios where server-side rendering (SSR) or hybrid rendering is desired.
// Default next.config.js
module.exports = {
target: 'server',
// Other configurations go here
};
Other Possible Values
The target
option can take other values, each catering to specific deployment scenarios:
'server'
(Default): As mentioned, this is the default value, indicating a server-rendered application.'serverless'
: Optimized for serverless deployment environments, this configuration generates a serverless function for each page.
// next.config.js
module.exports = {
target: 'serverless',
// Other configurations go here
};
'experimental-serverless-trace'
: An experimental option that aims to provide improved serverless deployment performance by reducing the size of the serverless function.
// next.config.js
module.exports = {
target: 'experimental-serverless-trace',
// Other configurations go here
};
'static'
: Generates a fully static website. This is suitable for scenarios where you want to pre-render all pages at build time.
// next.config.js
module.exports = {
target: 'static',
// Other configurations go here
};
Use Cases and Considerations
1. Serverless Deployments
If you’re deploying your Next.js application to a serverless environment, such as AWS Lambda or Vercel, setting the target
to 'serverless'
is often the preferred choice. This configuration optimizes the build for serverless deployments, generating individual serverless functions for each page.
// next.config.js
module.exports = {
target: 'serverless',
// Other configurations go here
};
2. Static Site Generation (SSG)
For scenarios where you want to generate a fully static website with all pages pre-rendered at build time, the target
can be set to 'static'
. This is particularly useful for content-heavy websites with infrequent updates.
// next.config.js
module.exports = {
target: 'static',
// Other configurations go here
};
3. Hybrid Rendering
When server-side rendering is required for certain pages while others can be pre-rendered statically, Next.js supports a hybrid rendering approach. This involves using the default 'server'
target and selectively enabling static site generation for specific routes.
// next.config.js
module.exports = {
target: 'server',
// Other configurations go here
// Enable static site generation for specific routes
// Example: { '/dynamic-route': { target: 'serverless' } }
};
Advanced Configurations
In certain scenarios, advanced configurations might be required based on the hosting platform or deployment environment. For example, in serverless deployments, the 'experimental-serverless-trace'
target may be considered for improved performance.
// next.config.js
module.exports = {
target: 'experimental-serverless-trace',
// Other configurations go here
};
Troubleshooting and Tips
- Compatibility: Ensure that the target you choose aligns with the capabilities and requirements of your hosting platform. Different platforms may have specific expectations regarding the type of deployment they support.
- Experimental Features: Keep in mind that experimental features, such as
'experimental-serverless-trace'
, may be subject to changes and improvements in future Next.js releases. Use them with caution, especially in production environments. - Documentation: Regularly refer to the Next.js documentation for updates and best practices regarding the
target
option and associated features.
Conclusion
In the intricate dance of web development, the next.config.js
file takes center stage, orchestrating the behavior of your Next.js application. The target
option, in particular, serves as a powerful conductor, determining the environment in which your application will flourish. Whether you opt for server-side rendering, serverless deployment, static site generation, or experiment with hybrid approaches, understanding the purpose of the target
option empowers you to tailor your Next.js application for optimal performance and compatibility. Harness the potential of target
in next.config.js
, and let your Next.js project shine in the digital realm.
What are hooks, and how are they used in Next.js
How can you optimize images in a Next.js application
What is the purpose of the getInitialProps function in Next.js
How can you deploy a Next.js application
How can you implement global CSS styles in a Next.js project
How can you implement pagination in a Next.js application
What is the purpose of the useContext hook in React
How can you handle authentication in a Next.js application
How can you set up and use environment variables in a Next.js project