How can you handle authentication in a Next.js application
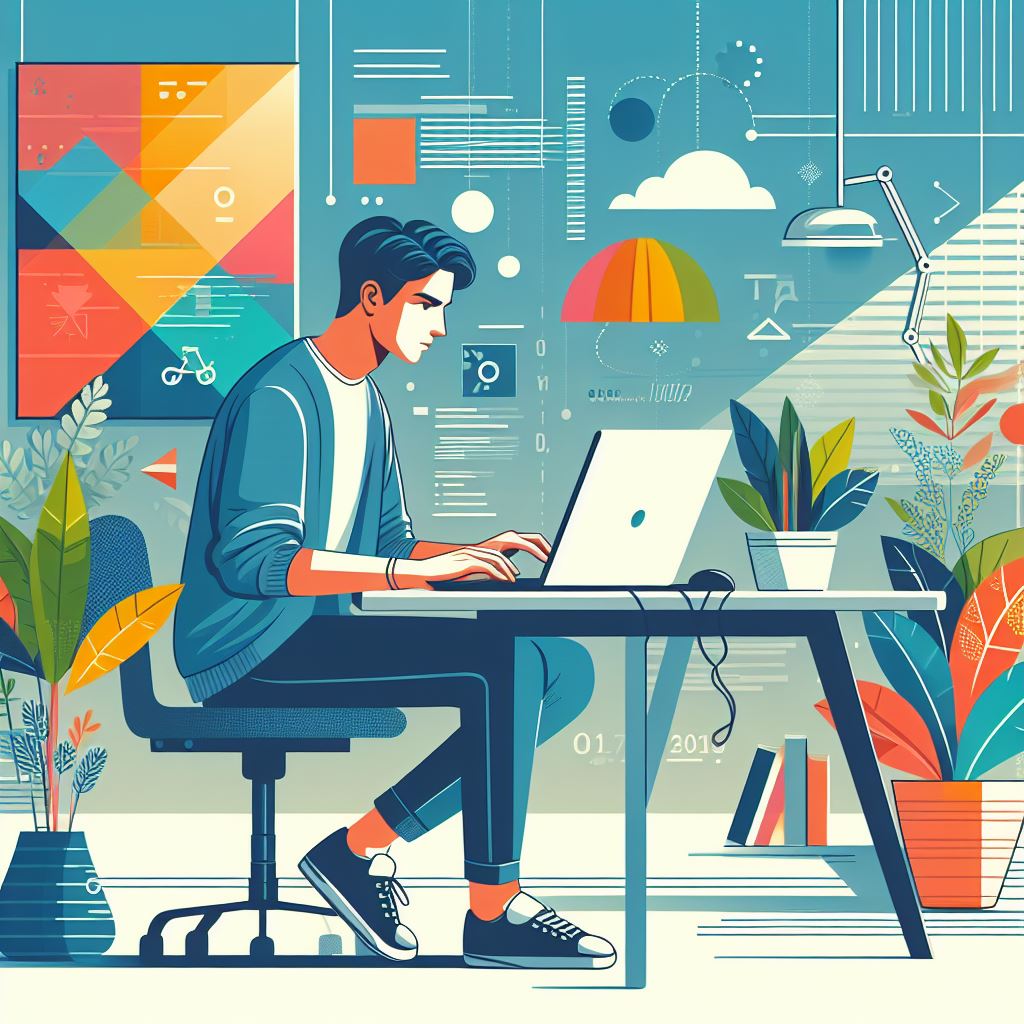
Authentication is a crucial aspect of web development, ensuring secure access to resources and personalized user experiences. In a Next.js application, handling authentication requires a thoughtful approach to balance security, usability, and seamless integration. In this comprehensive guide, we’ll explore various authentication methods, best practices, and implementation strategies tailored for Next.js.
1. Introduction to Authentication in Next.js
1.1 Why Authentication Matters
Authentication is the process of verifying the identity of a user or system. In web development, it ensures that users accessing certain resources are who they claim to be. This is crucial for protecting sensitive data, maintaining user privacy, and offering personalized experiences.
1.2 Challenges in Authentication
While authentication is essential, implementing it effectively comes with challenges. Next.js developers must navigate issues like securely storing user credentials, managing session states, and handling various authentication methods.
2. Authentication Methods in Next.js
Next.js supports multiple authentication methods, each with its strengths and use cases.
2.1 JWT Authentication
JSON Web Tokens (JWT) are widely used for stateless authentication. In Next.js, implementing JWT authentication involves generating and verifying tokens, ensuring secure storage, and managing token expiration.
2.2 OAuth Authentication
OAuth allows users to log in using external services like Google, Facebook, or GitHub. Next.js applications can integrate OAuth by configuring providers, redirecting users for authentication, and handling callback URLs.
2.3 Session-Based Authentication
Session-based authentication involves creating and managing user sessions. Next.js applications can implement session-based authentication using server-side storage, securely handling sessions, and implementing logout functionality.
3. Setting Up Authentication in Next.js
3.1 Project Initialization
Begin by initializing a Next.js project using the appropriate template and folder structure.
npx create-next-app my-auth-app
3.2 Installing Dependencies
Install necessary packages for authentication, such as next-auth
for OAuth and session-based authentication, or jsonwebtoken
for JWT authentication.
npm install next-auth jsonwebtoken
3.3 Creating Authentication Components
Create components for login forms, registration forms, and authentication context providers to encapsulate authentication logic.
4. JWT Authentication in Next.js
4.1 Generating JWT Tokens
Implement server-side logic to generate JWT tokens upon successful authentication, including user information and expiration details.
// utils/auth.js
const jwt = require('jsonwebtoken');
const generateToken = (user) => {
const token = jwt.sign({ userId: user.id }, process.env.JWT_SECRET, { expiresIn: '1h' });
return token;
};
module.exports = { generateToken };
4.2 Verifying JWT Tokens
On subsequent requests, verify JWT tokens to ensure their validity and extract user information.
// middleware/auth.js
const jwt = require('jsonwebtoken');
const verifyToken = (token) => {
try {
const decoded = jwt.verify(token, process.env.JWT_SECRET);
return decoded.userId;
} catch (error) {
return null;
}
};
module.exports = { verifyToken
};
4.3 Storing Tokens Securely
Securely store JWT tokens on the client-side using HTTP-only cookies or other secure storage mechanisms.
5. OAuth Authentication in Next.js
5.1 Configuring OAuth Providers
Configure OAuth providers like Google, Facebook, or GitHub in the Next.js application.
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
// Add more providers as needed
],
});
5.2 Redirecting Users for Authentication
Redirect users to the appropriate OAuth provider for authentication.
// pages/auth/login.js
import { signIn } from 'next-auth/react';
const LoginPage = () => {
const handleLogin = () => {
signIn('google');
};
return (
<div>
<button onClick={handleLogin}>Login with Google</button>
</div>
);
};
export default LoginPage;
5.3 Handling OAuth Callbacks
Handle OAuth callbacks to complete the authentication process.
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
// Add more providers as needed
],
callbacks: {
async jwt(token, user) {
if (user) {
token.id = user.id;
}
return token;
},
},
});
6. Session-Based Authentication in Next.js
6.1 Setting Up Sessions
Implement server-side logic to manage user sessions, including session creation, storage, and cleanup.
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Credentials({
credentials: {
username: { label: 'Username', type: 'text' },
password: { label: 'Password', type: 'password' },
},
async authorize(credentials) {
// Check credentials against a database
const user = { id: 1, name: 'John Doe' };
if (user) {
return Promise.resolve(user);
} else {
return Promise.resolve(null);
}
},
}),
],
session: {
jwt: true,
},
});
6.2 Managing Session State
Manage session state on the client-side by storing session information in secure storage.
// pages/auth/login.js
import { signIn } from 'next-auth/react';
const LoginPage = () => {
const handleLogin = () => {
signIn('credentials', { username: 'john', password: 'pass' });
};
return (
<div>
<button onClick={handleLogin}>Login with Credentials</button>
</div>
);
};
export default LoginPage;
6.3 Implementing Logout Functionality
Implement logout functionality by clearing session information.
// pages/auth/logout.js
import { signOut } from 'next-auth/react';
const LogoutPage = () => {
const handleLogout = () => {
signOut();
};
return (
<div>
<button onClick={handleLogout}>Logout</button>
</div>
);
};
export default LogoutPage;
7. Securing Routes in Next.js
7.1 Public and Private Routes
Implement public and private routes to control access to certain parts of the application.
// pages/dashboard.js
import { useSession } from 'next-auth/react';
import { useRouter } from 'next/router';
const DashboardPage = () => {
const { data: session, status } = useSession();
const router = useRouter();
if (status === 'loading') {
return <p>Loading...</p>;
}
if (!session) {
// Redirect to login page if not authenticated
router.push('/auth/login');
return null;
}
return (
<div>
<h1>Welcome, {session.user.name}!</h1>
{/* Dashboard content */}
</div>
);
};
export default DashboardPage;
7.2 Role-Based Access Control
Implement role-based access control to restrict certain actions or views based on user roles.
// pages/admin/dashboard.js
import { useSession } from 'next-auth/react';
import { useRouter } from 'next/router';
const AdminDashboardPage = () => {
const { data: session, status } = useSession();
const router = useRouter();
if (status === 'loading') {
return <p>Loading...</p>;
}
if (!session || session.user.role !== 'admin') {
// Redirect to unauthorized page if not authenticated or not an admin
router.push('/auth/unauthorized');
return null;
}
return (
<div>
<h1>Welcome, Admin {session.user.name}!</h1>
{/* Admin dashboard content */}
</div>
);
};
export default AdminDashboardPage;
7.3 Protecting API Routes
Protect API routes by requiring authentication and handling access control within the route.
// pages/api/admin/dashboard.js
import { getSession } from 'next-auth/react';
const handler = async (req, res) => {
const session = await getSession({ req });
if (!session || session.user.role !== 'admin') {
// Return unauthorized status if not authenticated or not an admin
return res.status(401).json({ error: 'Unauthorized' });
}
// Return admin dashboard data
res.status(200).json({ message: 'Admin dashboard data' });
};
export default handler;
8. Best Practices for Next.js Authentication
8.1 Securely Handling User Credentials
Ensure secure storage and transmission of user credentials, use HTTPS, and avoid storing sensitive information in client-side code.
8.2 Implementing Multi-Factor Authentication
Enhance security by implementing multi-factor authentication (MFA) for an additional layer of user verification.
8.3 Keeping Sessions Secure
Regularly rotate session tokens, use secure cookies, and implement session timeout to enhance overall session security.
9. Social Authentication in Next.js
9.1 Integrating Social Logins
Allow users to log in using their social media accounts by integrating social login providers.
// pages/api/auth/[...nextauth].js
import NextAuth from 'next-auth';
import Providers from 'next-auth/providers';
export default NextAuth({
providers: [
Providers.Google({
clientId: process.env.GOOGLE_CLIENT_ID,
clientSecret: process.env.GOOGLE_CLIENT_SECRET,
}),
Providers.Facebook({
clientId: process.env.FACEBOOK_CLIENT_ID,
clientSecret: process.env.FACEBOOK_CLIENT_SECRET,
}),
// Add more providers as needed
],
});
9.2 Customizing User Profiles
Customize user profiles by fetching additional information from social providers and storing it in the user database.
9.3 Managing Linked Accounts
Allow users to link multiple authentication methods to their account for flexibility and convenience.
10. Testing Authentication in Next.js
10.1 Unit Testing Authentication Components
Write unit tests to ensure that authentication components handle user interactions and state changes correctly.
10.2 Integration Testing Authentication Flows
Perform integration tests to validate the entire authentication flow, including form submissions, redirects, and session management.
10.3 End-to-End Testing User Scenarios
Conduct end-to-end tests to simulate real user scenarios, covering various authentication methods and edge cases.
11. Troubleshooting Authentication Issues
11.1 Common Authentication Pitfalls
Identify and address common authentication issues, such as token expiration, session conflicts, and misconfigured OAuth providers.
11.2 Debugging Authentication Errors
Use debugging tools and logs to diagnose authentication errors, check server-side logs, and inspect network requests.
11.3 Monitoring Authentication Logs
Implement logging for authentication-related events to monitor user activity, detect anomalies, and troubleshoot issues efficiently.
12. Scaling Authentication in Next.js
12.1 Handling Increased User Traffic
Implement strategies to handle increased user traffic, optimize authentication server performance, and scale infrastructure as needed.
12.2 Scaling Authentication Servers
Consider distributed systems, load balancing, and caching mechanisms to scale authentication servers horizontally.
12.3 Load Balancing for Authentication
Use load balancing techniques to distribute authentication requests evenly and prevent server overload during peak times.
13. Future Trends in Next.js Authentication
13.1 Passwordless Authentication
Explore passwordless authentication methods, such as email or SMS-based verification, for a more user-friendly experience.
13.2 Biometric Authentication
Keep an eye on emerging biometric authentication technologies, providing users with secure and convenient login options.
13.3 Zero-Trust Security Models
Adopt zero-trust security models, where authentication is required for every access attempt, enhancing overall system security.
14. Conclusion
Mastering authentication in Next.js requires a holistic approach, considering various methods, security measures, and user experience aspects. By delving into JWT, OAuth, session-based authentication, and securing routes, this guide equips developers with the knowledge needed to implement robust authentication systems. Best practices, troubleshooting tips, and scalability considerations ensure that your Next.js applications not only authenticate users securely but also provide a seamless and reliable experience. Stay ahead of the curve by exploring social authentication, testing strategies, and keeping an eye on future trends, ensuring your authentication mechanisms evolve with the ever-changing landscape of web development. Elevate your Next.js applications to new heights by mastering the art of authentication.
How to implement a collapsible sidebar menu with Bootstrap
How to use Bootstrap’s text truncation classes for long content
How to create a responsive contact form with validation in Bootstrap
How to use Bootstrap’s container and container-fluid classes
How to implement a sticky header with Bootstrap
How to use Bootstrap’s utility classes for flexbox alignment
How does React differ from other JavaScript frameworks
What is JSX, and how does it differ from regular JavaScript syntax