What is the purpose of the useContext hook in React
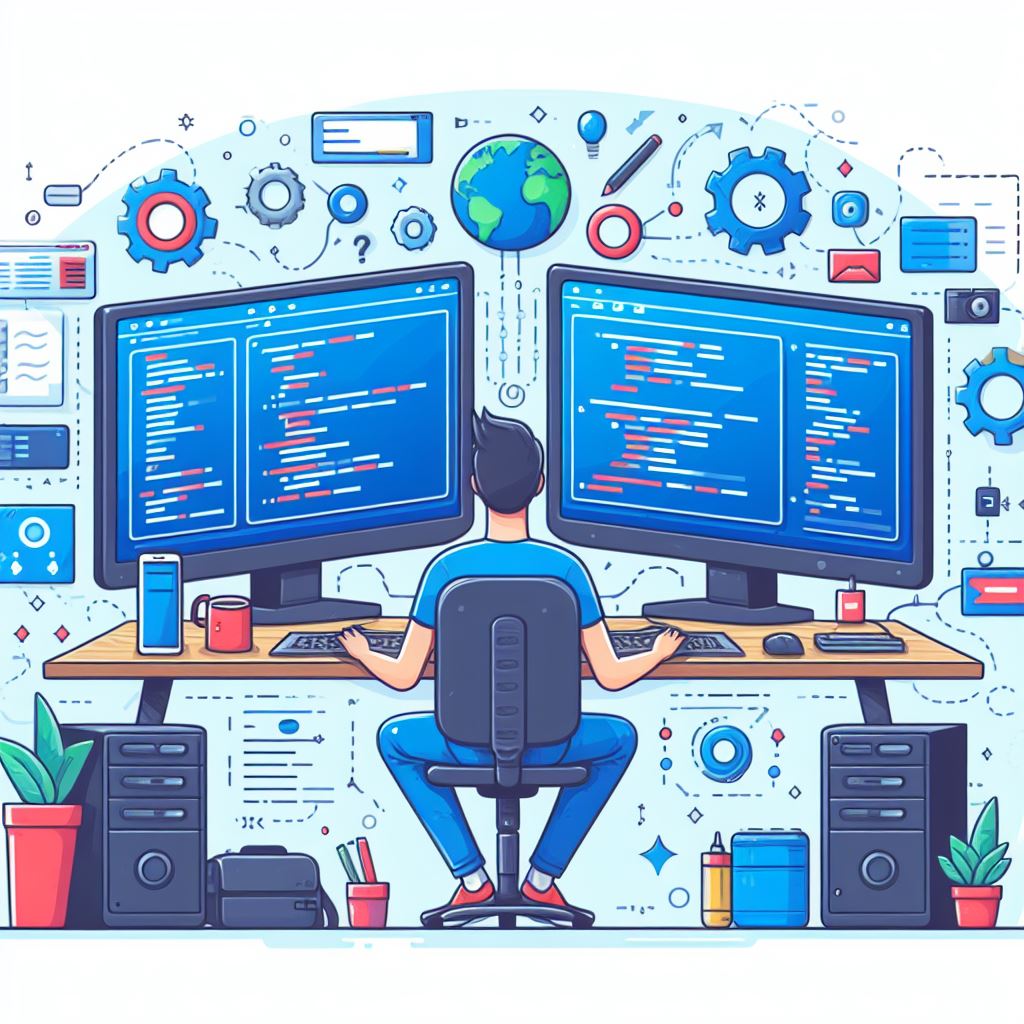
React’s useContext
hook is a powerful tool for state management, providing a streamlined way to share and access data across components. Understanding its purpose and capabilities is essential for React developers seeking efficient and clean state management solutions. In this comprehensive guide, we will explore the intricacies of the useContext
hook, its core purpose, and practical examples to harness its full potential.
1.1 What is State Management
In React, state management is the process of handling and manipulating the data within a component and ensuring its synchronization across the application. As applications grow in complexity, managing state becomes a critical aspect of development.
1.2 The Role of Context API
The Context API, introduced in React 16.3, provides a way to share values like themes, user authentication, or language preferences between components without having to pass the data explicitly through each level of the component tree. While Context API alone is powerful, useContext
enhances its usability and readability.
1.3 Enter useContext
Hook
The useContext
hook is part of the React Hooks API, introduced in React 16.8, allowing functional components to consume context values. It simplifies the process of subscribing to a context within a functional component, making the code more concise and readable compared to the traditional Context.Consumer
pattern.
2. Getting Started with useContext
2.1 Setting Up Context
Before diving into useContext
, let’s create a basic example. First, define a context using createContext
.
// contexts/ThemeContext.js
import { createContext } from 'react';
const ThemeContext = createContext();
export default ThemeContext;
2.2 Consuming Context with useContext
In a component where you want to access the context, import the context and use useContext
.
// components/ThemedComponent.js
import React, { useContext } from 'react';
import ThemeContext from '../contexts/ThemeContext';
const ThemedComponent = () => {
const theme = useContext(ThemeContext);
return (
<div style={{ background: theme.background, color: theme.text }}>
This component is themed!
</div>
);
};
export default ThemedComponent;
2.3 Sharing Data Between Components
useContext
allows you to access the context values directly, eliminating the need for a wrapper component. This leads to cleaner code and avoids prop drilling.
// components/App.js
import React from 'react';
import ThemedComponent from './ThemedComponent';
import ThemeContext from '../contexts/ThemeContext';
const App = () => {
return (
<ThemeContext.Provider value={{ background: 'lightblue', text: 'black' }}>
<ThemedComponent />
</ThemeContext.Provider>
);
};
export default App;
3. Real-World Example: Theming in React
3.1 Creating a Theme Context
Let’s create a more practical example by implementing theming in a React app. Start by defining a theme context.
// contexts/ThemeContext.js
import { createContext } from 'react';
const ThemeContext = createContext();
export default ThemeContext;
3.2 Implementing ThemeProvider
Create a ThemeProvider
component to manage the theme state and provide it through the context.
// components/ThemeProvider.js
import React, { useState } from 'react';
import ThemeContext from '../contexts/ThemeContext';
const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState({ background: 'lightblue', text: 'black' });
const toggleTheme = () => {
setTheme((prevTheme) => ({
background: prevTheme.background === 'lightblue' ? 'darkblue' : 'lightblue',
text: prevTheme.text === 'black' ? 'white' : 'black',
}));
};
return (
<ThemeContext.Provider value={{ theme, toggleTheme }}>
{children}
</ThemeContext.Provider>
);
};
export default ThemeProvider;
3.3 Consuming Theme in Components
Now, any component within the ThemeProvider
can consume and modify the theme using useContext
.
// components/ThemedComponent.js
import React, { useContext } from 'react';
import ThemeContext from '../contexts/ThemeContext';
const ThemedComponent = () => {
const { theme, toggleTheme } = useContext(ThemeContext);
return (
<div style={{ background: theme.background, color: theme.text }}>
This component is themed!
<button onClick={toggleTheme}>Toggle Theme</button>
</div>
);
};
export default ThemedComponent;
4. Dynamic Context with useContext
4.1 Dynamically Changing Context Values
One of the strengths of useContext
is its ability to dynamically change context values. In the theme example, modifying the theme dynamically triggers a re-render of all components consuming that context.
4.2 Use Case: User Authentication
Consider a use case where user authentication state changes dynamically. useContext
allows components to react to these changes without explicit prop passing.
// contexts/AuthContext.js
import { createContext, useState } from 'react';
const AuthContext = createContext();
const AuthProvider = ({ children }) => {
const [authenticated, setAuthenticated] = useState(false);
const login = () => setAuthenticated(true);
const logout = () => setAuthenticated(false);
return (
<AuthContext.Provider value={{ authenticated, login, logout }}>
{children}
</AuthContext.Provider>
);
};
export { AuthProvider, AuthContext };
5. Optimizing Performance with useContext
5.1 Memoization in React
Memoization is crucial for optimizing React performance. Memoization prevents unnecessary re-renders by caching the results of expensive operations.
5.2 useMemo
and `use
Callback` Hooks
In the context of useContext
, useMemo
can be employed to memoize values, and useCallback
can memoize functions.
6. Best Practices and Caveats
6.1 When to Use useContext
useContext
is best suited for global or widely shared state. Avoid using it for deeply nested components where prop drilling might be a more appropriate solution.
6.2 Avoiding Overuse
While useContext
is powerful, it’s essential to use it judiciously. Overusing it can lead to a complex web of dependencies and make the codebase harder to maintain.
6.3 Alternatives to useContext
In some scenarios, alternatives like Redux or Recoil might be more suitable for state management. Evaluate your project’s requirements and choose the solution that fits best.
7. Conclusion
Understanding the purpose and capabilities of the useContext
hook is fundamental for React developers aiming to create scalable and maintainable applications. By delving into real-world examples, dynamic context changes, and performance optimization techniques, this guide has provided a comprehensive exploration of the useContext
hook. Integrating it wisely into your React applications empowers you to manage state efficiently, resulting in more readable and maintainable code. Whether you’re building simple theming functionality or handling complex user authentication, useContext
stands as a valuable tool in your React state management toolkit.
How to use Bootstrap’s utilities for hiding and showing elements
How to implement a sticky footer with a content area that scrolls
How to use Bootstrap’s form control sizing classes
How to create a responsive image carousel with captions
How to use Bootstrap’s responsive utilities for text alignment
How to implement a full-screen background image with Bootstrap