What are hooks, and how are they used in Next.js
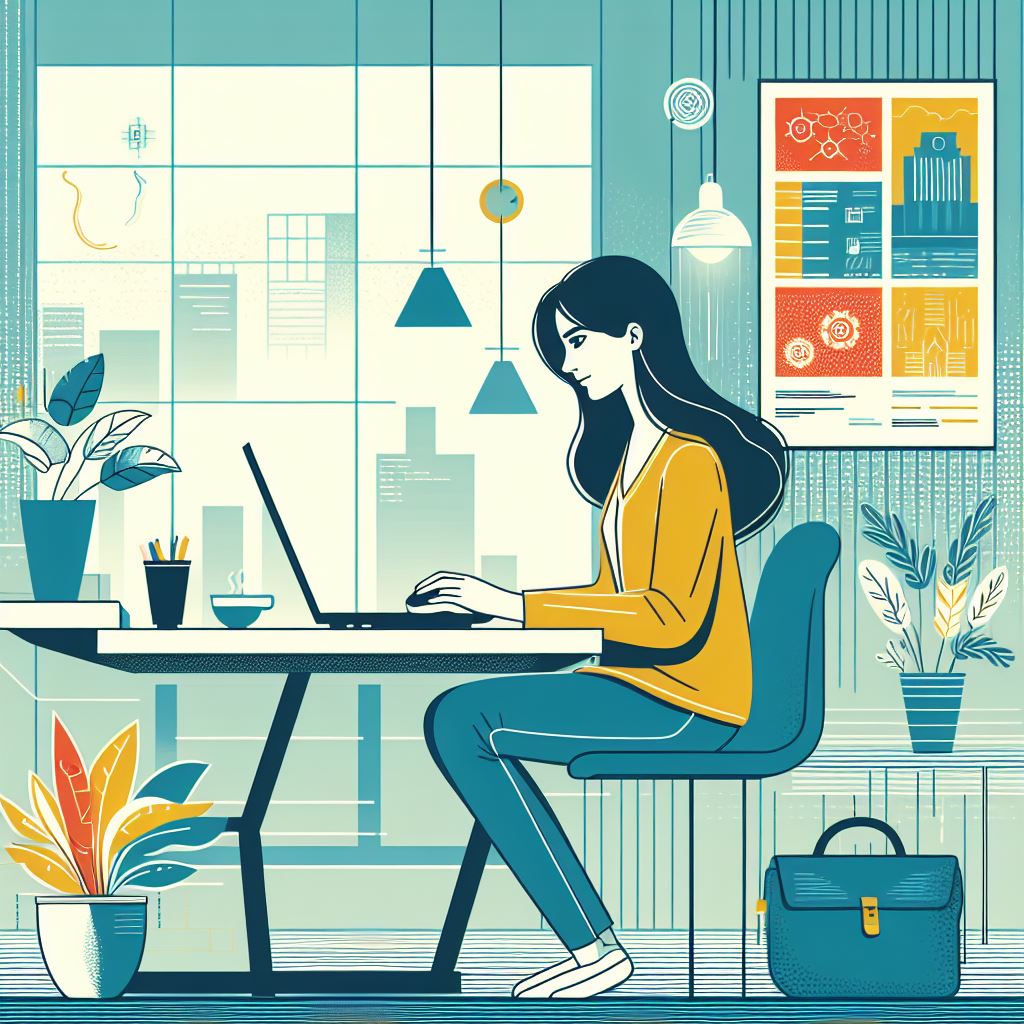
React Hooks have revolutionized the way developers manage stateful logic and side effects in React applications, providing a cleaner and more concise alternative to class components. In the context of Next.js, a powerful React framework, understanding how to leverage hooks is crucial for building efficient and maintainable web applications. In this article, we’ll delve into the world of React Hooks, exploring their fundamentals and demonstrating how they can be effectively used in Next.js.
What are React Hooks
React Hooks are functions that allow developers to use state and other React features in functional components. Introduced in React 16.8, hooks provide a more direct and expressive way to handle stateful logic without relying on class components. The most commonly used hooks include useState
, useEffect
, useContext
, and useReducer
.
useState
The useState
hook enables functional components to manage local state. It returns an array containing the current state value and a function to update that value. Let’s see an example in a Next.js component:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
In this example, the useState
hook is used to create a count
state variable with an initial value of 0
. The setCount
function is used to update the state when the button is clicked.
useEffect
The useEffect
hook is employed for side effects in functional components, such as data fetching, subscriptions, or manually changing the DOM. It takes a function that contains the code for the side effect and runs it after the component renders. Here’s an example in a Next.js component:
import React, { useState, useEffect } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data from an API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(result => setData(result))
.catch(error => console.error('Error fetching data:', error));
}, []); // Empty dependency array means this effect runs once after the initial render
return (
<div>
{data ? (
<p>Data: {data}</p>
) : (
<p>Loading...</p>
)}
</div>
);
};
export default DataFetcher;
In this example, the useEffect
hook is used to fetch data from an API after the component mounts. The empty dependency array ensures that the effect runs only once after the initial render.
useContext
The useContext
hook allows components to subscribe to React context without introducing a context consumer wrapper. It accepts a context object created by React.createContext
and returns the current context value. Here’s a simple example:
import React, { useContext } from 'react';
const ThemeContext = React.createContext('light');
const ThemedComponent = () => {
const theme = useContext(ThemeContext);
return (
<div>
<p>Current theme: {theme}</p>
</div>
);
};
export default ThemedComponent;
In this example, the useContext
hook is used to access the current theme from the ThemeContext
.
useReducer
The useReducer
hook is an alternative to useState
for managing more complex state logic. It takes a reducer function and an initial state, returning the current state and a dispatch function. Here’s a simple example:
import React, { useReducer } from 'react';
const initialState = { count: 0 };
const reducer = (state, action) => {
switch (action.type) {
case 'increment':
return { count: state.count + 1 };
case 'decrement':
return { count: state.count - 1 };
default:
return state;
}
};
const CounterWithReducer = () => {
const [state, dispatch] = useReducer(reducer, initialState);
return (
<div>
<p>Count: {state.count}</p>
<button onClick={() => dispatch({ type: 'increment' })}>Increment</button>
<button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button>
</div>
);
};
export default CounterWithReducer;
In this example, the useReducer
hook is used to manage the state of a counter with increment and decrement actions.
Using React Hooks in Next.js
Next.js seamlessly integrates with React hooks, allowing you to use them in functional components just like in any other React application. Whether you’re building static pages with getStaticProps
or dynamic pages with getServerSideProps
, hooks can be applied to manage state, fetch data, and handle side effects.
Example: Using useState
in a Next.js Component
Let’s create a simple Next.js component that uses the useState
hook:
import React, { useState } from 'react';
const Counter = () => {
const [count, setCount] = useState(0);
return (
<div>
<p>Count: {count}</p>
<button onClick={() => setCount(count + 1)}>Increment</button>
</div>
);
};
export default Counter;
In this example, the useState
hook is used to manage the state of a counter in a Next.js component. The setCount
function updates the state, triggering a re-render of the component.
Example: Using useEffect
in a Next.js Component
Let’s create a Next.js component that uses the useEffect
hook to fetch data:
import React, { useState, useEffect } from 'react';
const DataFetcher = () => {
const [data, setData] = useState(null);
useEffect(() => {
// Fetch data from an API
fetch('https://api.example.com/data')
.then(response => response.json())
.then(result => setData(result))
.catch(error => console.error('Error fetching data:', error));
}, []); // Empty dependency array means this effect runs once after the initial render
return (
<div>
{data ? (
<p>Data: {data}</p>
) : (
<p>Loading...</p>
)}
</div>
);
};
export default DataFetcher;
In this example, the useEffect
hook is used to fetch data from an API after the component mounts. The empty dependency array ensures that the effect runs only once after the initial render.
Conclusion
React Hooks have become an integral part of React development, enabling functional components to manage stateful logic and side effects efficiently. In Next.js, a framework that builds on React, harnessing the power of hooks is essential for creating dynamic and performant web applications. By understanding the fundamentals of hooks and their application in Next.js components, developers can unlock a more streamlined and expressive approach to building modern web experiences. Whether you’re managing state with useState
, handling side effects with useEffect
, or employing other hooks for specific use cases, incorporating React Hooks into your Next.js projects can elevate your development workflow and deliver enhanced user experiences.
How many states are there in React
Where is state stored in React