How can you optimize images in a Next.js application
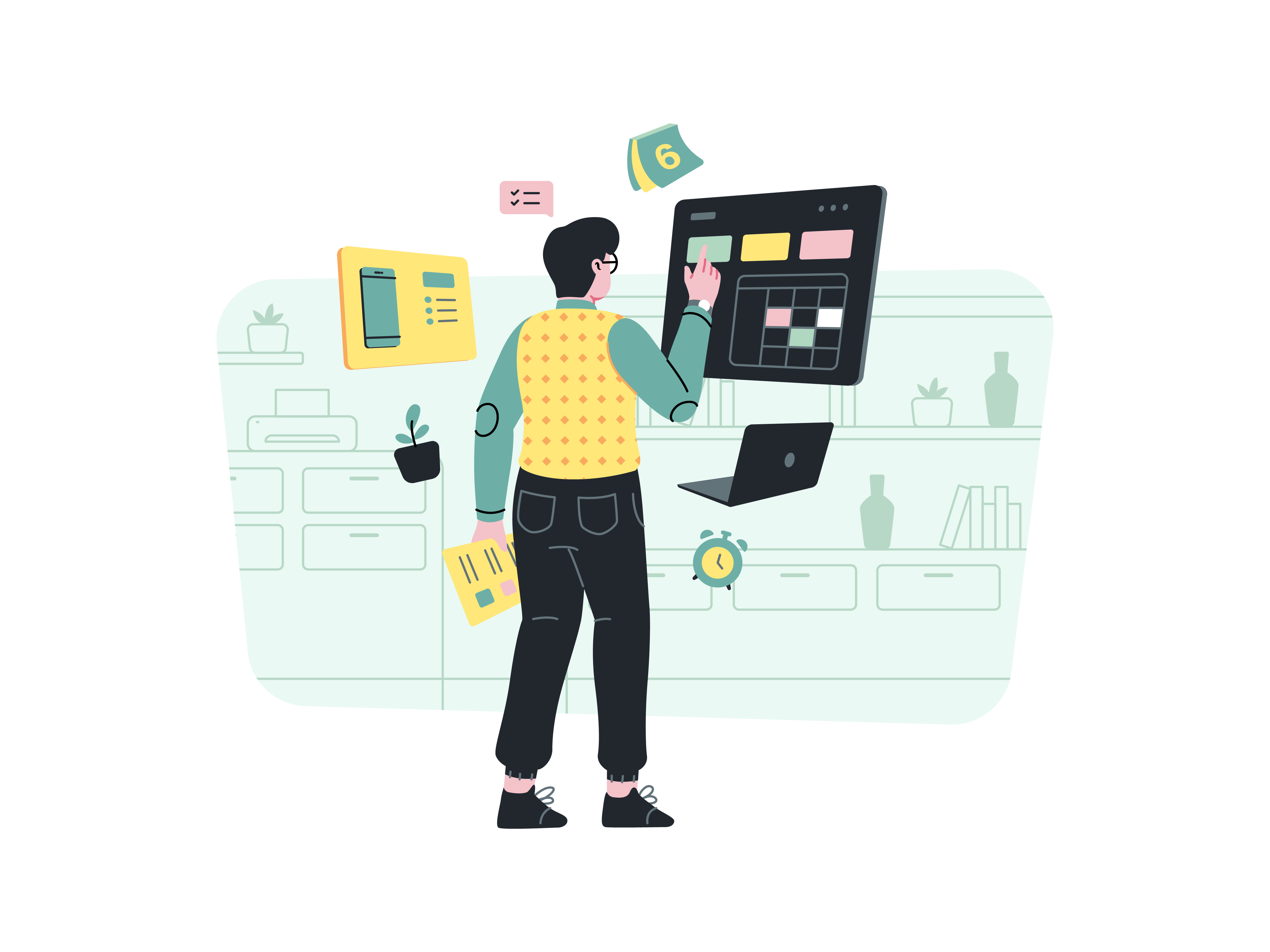
In the fast-paced realm of web development, optimizing images is a crucial aspect of creating high-performance applications. In a Next.js application, where speed and efficiency are paramount, understanding how to optimize images can significantly impact user experience and overall website performance. In this article, we will explore various strategies and tools for image optimization in a Next.js application, ensuring that your images are not only visually appealing but also load swiftly across different devices and network conditions.
The Importance of Image Optimization
Images play a pivotal role in web design, enhancing the visual appeal and conveying information effectively. However, high-quality images often come with large file sizes, which can lead to slower page loads, especially on slower internet connections or less powerful devices. Image optimization is the process of reducing the file size of images without compromising their visual quality. This optimization is essential for improving website performance, reducing bandwidth usage, and providing a better user experience.
Strategies for Image Optimization in Next.js
Next.js provides several strategies and tools for image optimization, catering to different scenarios and use cases. Let’s explore some of the key approaches:
1. Next.js Image Component
Next.js introduces a powerful next/image
component that simplifies the process of optimizing and serving images. This component leverages the image
tag and automatically optimizes images based on the device screen and resolution.
// Example of using next/image component in Next.js
import Image from 'next/image';
const OptimizedImage = () => (
<div>
<Image
src="/images/example.jpg"
alt="Example Image"
width={500}
height={300}
/>
</div>
);
export default OptimizedImage;
In this example, the Image
component is used to display an image with a specified width and height. Next.js takes care of optimizing the image based on the provided dimensions, ensuring efficient loading.
2. Automatic Image Optimization
Next.js provides automatic image optimization out of the box. During the build process, images in the public
directory are optimized and served in multiple sizes to cater to various devices and screen resolutions.
To enable automatic image optimization, simply place your images in the public
directory and reference them in your components or pages.
// Example of referencing images in Next.js
const ImageExample = () => (
<div>
<img src="/images/example.jpg" alt="Example Image" />
</div>
);
export default ImageExample;
Next.js will automatically optimize and serve the image in different sizes, improving performance and reducing the need for manual optimization.
3. Responsive Images with srcset
The srcset
attribute allows you to provide multiple image sources with different resolutions and sizes. This ensures that browsers can choose the most appropriate image based on the user’s device and screen characteristics.
// Example of using responsive images with srcset in Next.js
const ResponsiveImage = () => (
<div>
<img
src="/images/example.jpg"
srcSet="/images/example-400w.jpg 400w,
/images/example-800w.jpg 800w,
/images/example-1200w.jpg 1200w"
sizes="(max-width: 400px) 100vw,
(max-width: 800px) 80vw,
1200px"
alt="Example Image"
/>
</div>
);
export default ResponsiveImage;
In this example, different image sources are provided based on the expected viewport widths. The browser then selects the most appropriate image, reducing unnecessary data transfer and improving page load times.
Third-Party Tools for Image Optimization
In addition to the built-in features of Next.js, there are several third-party tools and services that can further enhance image optimization in your Next.js application.
1. Image Compression Tools
Tools like TinyPNG or ImageOptim can be used to manually compress images before adding them to your Next.js project. These tools employ advanced compression algorithms to reduce file sizes while preserving image quality.
2. Cloudinary
Cloudinary is a cloud-based image and video management platform that offers powerful image optimization capabilities. It provides features such as automatic format selection, responsive image delivery, and dynamic resizing, helping to deliver optimized images tailored to each user’s device.
3. Imgix
Imgix is another cloud-based image processing service that offers real-time image optimization, resizing, and manipulation. It allows you to specify image parameters in the URL, enabling dynamic adjustments without the need for pre-processing.
Best Practices for Image Optimization in Next.js
To ensure effective image optimization in your Next.js application, consider the following best practices:
1. Choose the Right Format
Select the appropriate image format for each use case. JPEG is suitable for photographs, PNG for images with transparency, and SVG for vector graphics. WebP is a modern format that provides excellent compression and quality.
2. Leverage Responsive Images
Use responsive images with the srcset
attribute to provide multiple image sources for different screen sizes and resolutions. This allows browsers to choose the most suitable image, reducing unnecessary data transfer.
3. Utilize next/image
Component
Leverage the built-in next/image
component for easy and automatic image optimization in Next.js. It handles various optimization techniques, such as lazy loading and responsive image generation.
4. Implement Lazy Loading
Enable lazy loading for images to defer their loading until they are about to enter the user’s viewport. This can significantly improve initial page load times.
// Example of lazy loading images in Next.js
<Image
src="/images/example.jpg"
alt="Example Image"
width={500}
height={300}
loading="lazy"
/>
5. Optimize and Compress
Prior to deployment, use image optimization tools to compress images and reduce file sizes. This ensures a balance between visual quality and performance.
6. Consider Image CDN
Explore content delivery networks (CDNs) that specialize in serving images
efficiently. CDNs can distribute images globally, reducing latency and improving load times for users around the world.
Conclusion
Image optimization is a vital component of creating high-performance web applications, and Next.js provides a range of tools and strategies to streamline this process. By leveraging the built-in next/image
component, automatic image optimization, and responsive image techniques, you can ensure that your images are delivered efficiently to users across various devices and network conditions. Additionally, exploring third-party tools and services such as Cloudinary or Imgix can provide advanced features and optimizations tailored to your specific needs. Implementing best practices and staying mindful of image formats and sizes will contribute to a faster and more engaging user experience in your Next.js application.
How to create a responsive pricing table with Bootstrap
How to implement a split-screen layout with Bootstrap
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap
How to use Bootstrap’s media objects
How to integrate Bootstrap with JavaScript libraries like jQuery