How does data fetching work in Next.js
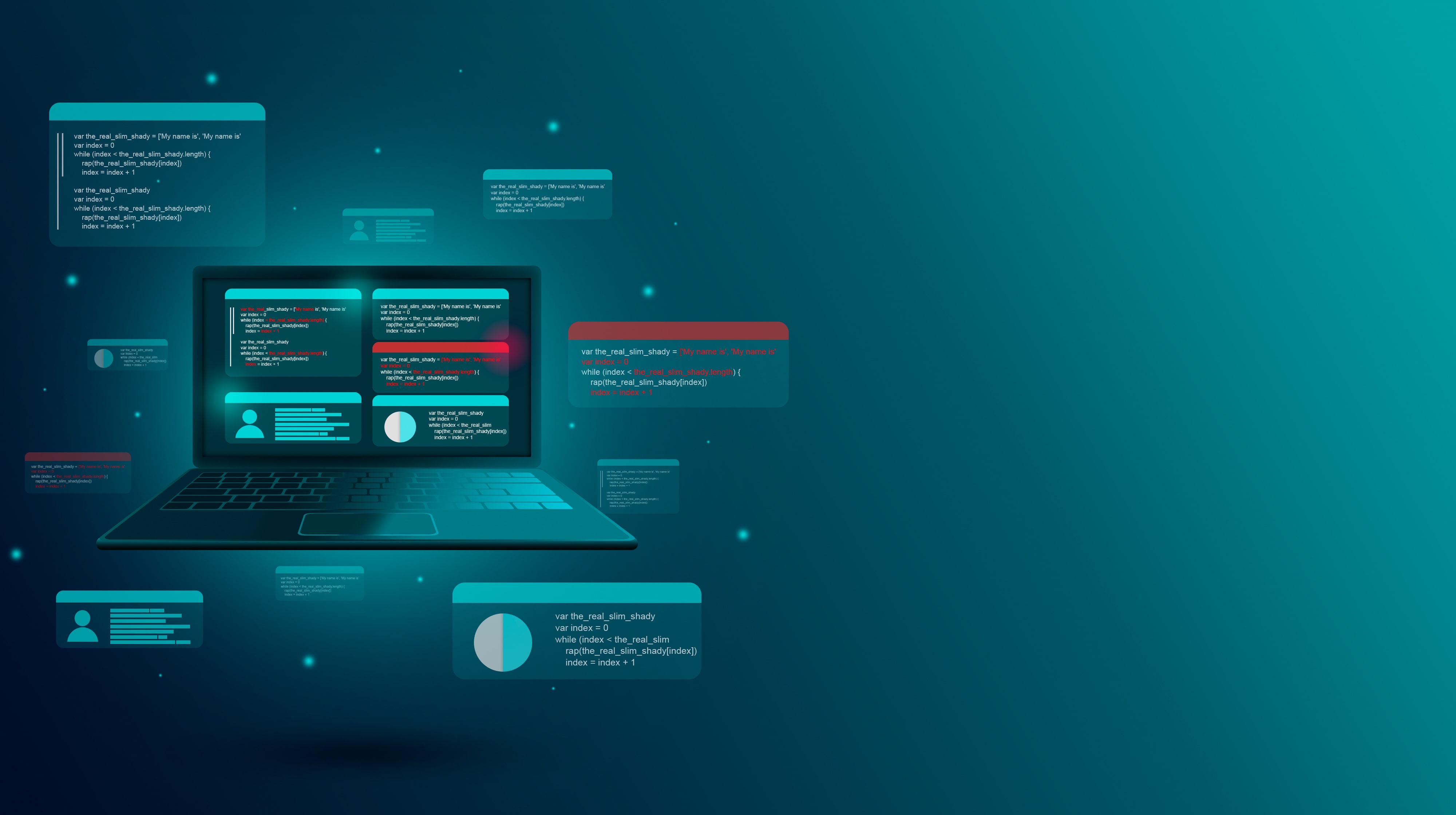
Next.js, a popular React framework, streamlines the process of building robust web applications. A fundamental aspect of creating dynamic and data-driven pages in Next.js is understanding how data fetching works. In this article, we will delve into the mechanisms and strategies behind data fetching in Next.js, exploring the various approaches and their implications.
The Basics of Data Fetching
Data fetching in Next.js refers to the process of retrieving external data to populate a page with dynamic content. This content could range from simple text to more complex data, such as images, user information, or any other data that enhances the user experience.
Next.js provides multiple ways to fetch data, catering to different scenarios and requirements. The primary methods include:
- Static Generation (getStaticProps): Pre-render pages at build time by fetching data during the build process.
- Server-Side Rendering (getServerSideProps): Fetch data on every request, rendering pages on the server.
- Client-Side Rendering (useEffect, SWR): Fetch data on the client side, after the initial page load.
Let’s explore each of these methods in detail.
Static Generation with getStaticProps
Static Generation is a key feature of Next.js that allows you to pre-render pages at build time. The getStaticProps
function is used to fetch data during the build process, generating static HTML files for each page.
// Example of using getStaticProps for data fetching
export async function getStaticProps() {
// Fetch data from an API or database
const data = await fetchData();
return {
props: { data },
};
}
const StaticPage = ({ data }) => (
<div>
<h1>{data.title}</h1>
<p>{data.content}</p>
</div>
);
export default StaticPage;
In this example, the getStaticProps
function fetches data at build time, and the resulting HTML is served to users on each request. This approach is suitable for content that does not change frequently and benefits from faster initial page loads.
Server-Side Rendering with getServerSideProps
Server-Side Rendering (SSR) involves fetching data on each request, enabling dynamic content that can change on the server side. The getServerSideProps
function is responsible for fetching data at runtime, allowing for up-to-date content.
// Example of using getServerSideProps for data fetching
export async function getServerSideProps() {
// Fetch data from an API or database
const data = await fetchData();
return {
props: { data },
};
}
const SSRPage = ({ data }) => (
<div>
<h1>{data.title}</h1>
<p>{data.content}</p>
</div>
);
export default SSRPage;
In this example, the getServerSideProps
function fetches data on every request, ensuring the most recent data is displayed. This approach is suitable for pages with frequently changing content or data that is not known at build time.
Client-Side Rendering with useEffect
and SWR
Client-Side Rendering (CSR) is employed when you want to fetch data on the client side, after the initial page load. React’s useEffect
hook is often used for client-side data fetching, along with libraries like SWR (Stale-While-Revalidate) for efficient caching and state management.
// Example of using useEffect and SWR for client-side data fetching
import { useEffect, useState } from 'react';
import useSWR from 'swr';
const ClientSidePage = () => {
const { data, error } = useSWR('/api/data', fetcher);
if (error) return <div>Error loading data</div>;
if (!data) return <div>Loading...</div>;
return (
<div>
<h1>{data.title}</h1>
<p>{data.content}</p>
</div>
);
};
export default ClientSidePage;
In this example, the useSWR
hook is used to fetch data from an API endpoint on the client side. The fetcher
function specifies how data should be retrieved. This approach is suitable for scenarios where you want to fetch data based on user interactions or when the data is not needed during the initial page load.
Choosing the Right Approach
The choice between Static Generation, Server-Side Rendering, and Client-Side Rendering depends on your specific use case and requirements. Consider the following factors:
- Data Freshness: If your data changes frequently and needs to be up-to-date on every request, Server-Side Rendering might be more suitable.
- Performance: For content that rarely changes and requires optimal performance, Static Generation is often the best choice.
- User Interactivity: If you need to fetch data based on user interactions or client-side events, Client-Side Rendering is a viable option.
- SEO: If search engine optimization is a priority, Static Generation or Server-Side Rendering is preferable, as search engines can crawl and index the content during the build process.
- Build Time vs. Runtime: Static Generation fetches data at build time, making it suitable for scenarios where data is known in advance. Server-Side Rendering and Client-Side Rendering fetch data at runtime, providing flexibility for dynamic content.
Conclusion
Data fetching is a critical aspect of building dynamic and engaging web applications, and Next.js provides a versatile set of tools to cater to different scenarios. Whether you choose Static Generation, Server-Side Rendering, or Client-Side Rendering depends on the nature of your data and the requirements of your application. By understanding the strengths and use cases of each approach, you can harness the full potential of Next.js to create performant, dynamic, and user-friendly web experiences.
How does client-side rendering (CSR) work in Next.js
How does Next.js handle bundling and code splitting
What is incremental static regeneration in Next.js
Can a Next.js application use both server-side rendering and client-side rendering
How does Next.js handle SEO for dynamic pages
How can you implement custom 404 error handling for API routes
What is the purpose of the styled-jsx library in Next.js
What is the purpose of the getStaticPaths function in dynamic routes
Explain the concept of SSR (Server-Side Rendering) vs. CSR (Client-Side Rendering) in React