What is the purpose of the pages directory in a Next.js project
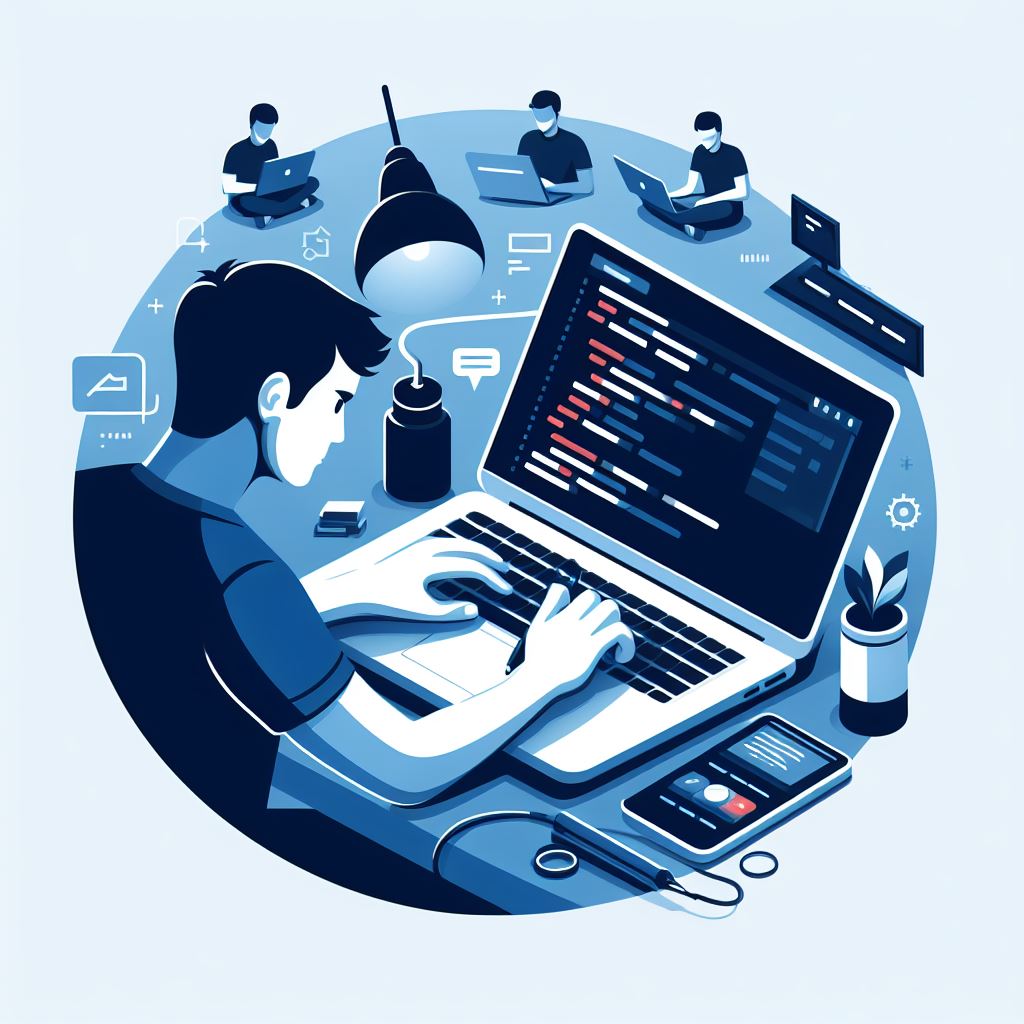
In the world of Next.js development, the “pages” directory holds a central role, serving as the backbone of routing and page structure. Understanding the purpose and functionality of the “pages” directory is crucial for building efficient and maintainable Next.js applications. In this comprehensive guide, we’ll delve into the significance of the “pages” directory in a Next.js project, exploring its purpose, structure, conventions, and best practices.
Understanding the “pages” Directory
The “pages” directory is a core feature of Next.js, responsible for defining the routes and page components of a Next.js application. It follows a convention-over-configuration approach, where the file structure directly corresponds to the URL structure of the application. Each file within the “pages” directory represents a different route or page of the application.
Purpose of the “pages” Directory
The primary purpose of the “pages” directory is to organize and define the routes and page components of a Next.js application. By following a simple and intuitive file-based routing system, Next.js eliminates the need for manual route configuration and simplifies the development process.
Structure of the “pages” Directory
The structure of the “pages” directory reflects the URL structure of the application, with each file representing a specific route or page. Nested directories within the “pages” directory create nested routes, allowing for hierarchical organization of pages.
pages/
|-- index.js // Route: /
|-- about.js // Route: /about
|-- products/
| |-- index.js // Route: /products
| |-- [id].js // Dynamic Route: /products/:id
File-Based Routing
Next.js utilizes file-based routing, where the filename determines the route path of the corresponding page. For example, a file named “about.js” in the “pages” directory represents the “/about” route of the application. This convention simplifies route configuration and ensures a consistent and predictable URL structure.
Dynamic Routes
In addition to static routes, the “pages” directory supports dynamic routes, allowing for the creation of pages with dynamic content based on URL parameters. Dynamic routes are defined by placing square brackets []
around the parameter in the filename. Next.js automatically parses the URL and provides the parameter value to the corresponding page component.
// pages/products/[id].js
const ProductPage = ({ id }) => {
return (
<div>
<h1>Product ID: {id}</h1>
</div>
);
};
export async function getServerSideProps({ params }) {
const { id } = params;
return {
props: {
id,
},
};
}
export default ProductPage;
Conventions and Best Practices
To ensure consistency and maintainability, it’s important to follow established conventions and best practices when organizing the “pages” directory:
- Use Descriptive Filenames: Choose descriptive filenames that accurately represent the content and purpose of each page.
- Organize Nested Routes: Organize nested routes using nested directories within the “pages” directory to maintain a clear and hierarchical structure.
- Leverage Dynamic Routes: Take advantage of dynamic routes to create pages with dynamic content and parameters.
- Avoid Over-Nesting: Avoid over-nesting of directories within the “pages” directory, as it can lead to complexity and confusion.
Conclusion
The “pages” directory is a fundamental aspect of Next.js development, serving as the foundation for routing and page structure. By following a simple and intuitive file-based routing system, Next.js eliminates the need for manual route configuration and streamlines the development process. Understanding the purpose, structure, conventions, and best practices of the “pages” directory is essential for building efficient, maintainable, and scalable Next.js applications. Whether organizing static routes, implementing dynamic routes, or nesting directories for hierarchical organization, the “pages” directory provides developers with a powerful and flexible mechanism for defining the routes and page components of their Next.js projects.
How many states are there in React
Where is state stored in React