What is the purpose of the next.config.js option env
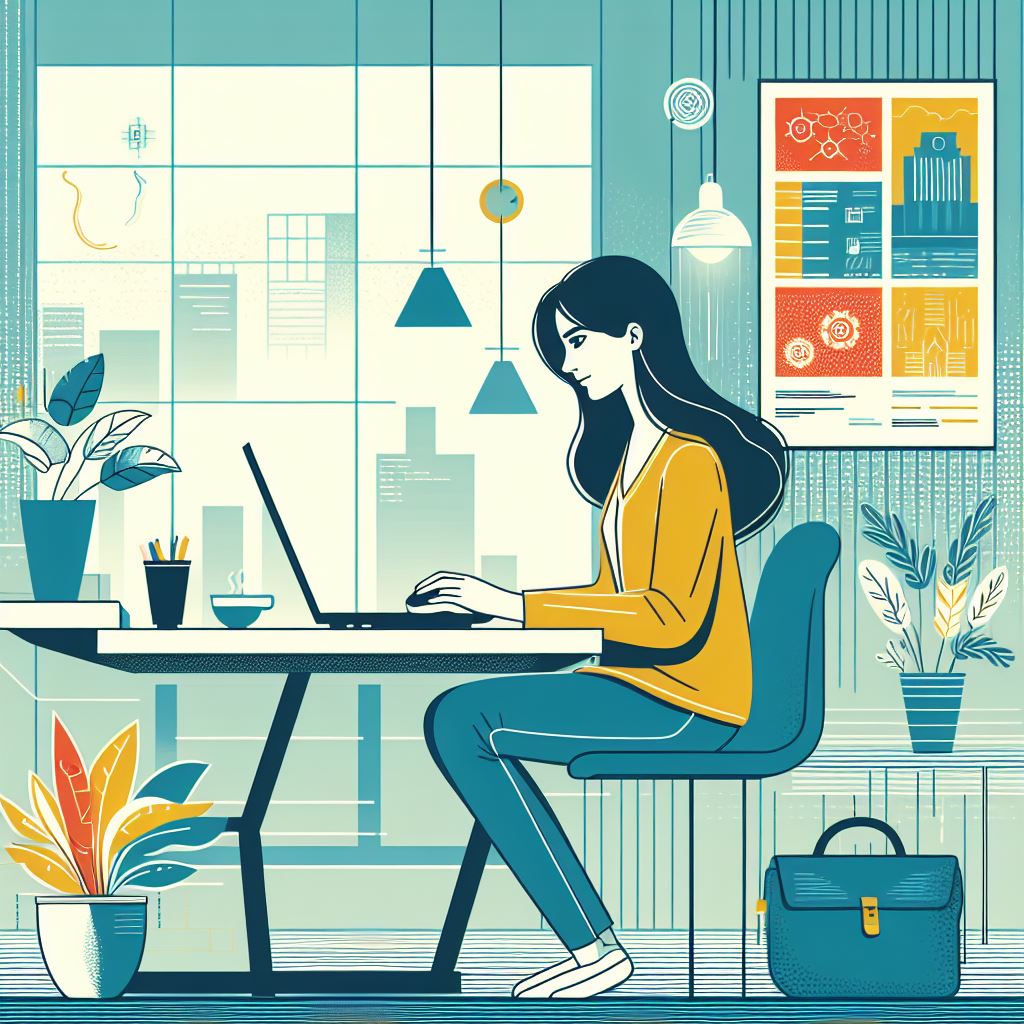
The next.config.js
file serves as a central configuration hub for Next.js applications, providing developers with a way to customize various aspects of their projects. Among the options available in next.config.js
, the env
option stands out as a powerful tool for managing environment variables. In this comprehensive guide, we’ll explore the purpose of the env
option in next.config.js
, its significance, use cases, and best practices.
Understanding Environment Variables
Before diving into the env
option in next.config.js
, let’s briefly discuss environment variables and their role in web development. Environment variables are dynamic values that can affect the behavior of an application depending on the environment it’s running in. They are commonly used to store sensitive information, configuration settings, or runtime data.
In a Next.js application, environment variables can be accessed both on the server-side and the client-side, making them versatile tools for configuring and customizing applications across different environments.
The env
Option in next.config.js
The env
option in next.config.js
allows developers to define environment variables that are accessible within the Next.js application. These environment variables can be used to configure various aspects of the application, such as API endpoints, authentication tokens, feature toggles, and more.
The env
option accepts an object containing key-value pairs of environment variable names and their corresponding values:
// next.config.js
module.exports = {
env: {
API_URL: 'https://api.example.com',
API_KEY: 'your_api_key',
},
};
Purpose and Significance
The primary purpose of the env
option in next.config.js
is to provide a centralized and structured way to manage environment variables within a Next.js application. By defining environment variables in next.config.js
, developers can ensure consistency, maintainability, and security across different environments, such as development, staging, and production.
Additionally, the env
option enables developers to abstract sensitive or configuration-specific information away from the codebase, reducing the risk of exposing sensitive data and simplifying deployment workflows.
Use Cases and Best Practices
The env
option in next.config.js
can be utilized in various scenarios and use cases, including:
1. API Configuration
// next.config.js
module.exports = {
env: {
API_URL: process.env.NODE_ENV === 'production' ? 'https://api.example.com' : 'http://localhost:3000',
API_KEY: 'your_api_key',
},
};
2. Feature Toggles
// next.config.js
module.exports = {
env: {
FEATURE_FLAG_ENABLED: process.env.NODE_ENV === 'production' ? 'true' : 'false',
},
};
3. Authentication Tokens
// next.config.js
module.exports = {
env: {
AUTH_TOKEN: 'your_auth_token',
},
};
4. Configuration Overrides
// next.config.js
module.exports = {
env: {
CUSTOM_CONFIG: process.env.CUSTOM_CONFIG || 'default_value',
},
};
When using environment variables in next.config.js
, it’s important to follow best practices to ensure security and maintainability:
- Avoid hardcoding sensitive information directly into
next.config.js
. Instead, use environment variables or secret management tools. - Keep environment-specific configurations separate by utilizing different environment files (
development.env
,production.env
, etc.). - Ensure that environment variables are properly documented and communicated to other team members to maintain clarity and consistency.
Conclusion
The env
option in next.config.js
provides a convenient and centralized mechanism for managing environment variables in Next.js applications. By defining environment variables in next.config.js
, developers can configure various aspects of their applications, such as API endpoints, feature toggles, and authentication tokens, while maintaining security and consistency across different environments. Understanding the purpose, significance, and best practices of the env
option empowers developers to build robust, customizable, and maintainable Next.js applications that adapt to different deployment environments seamlessly.
How to implement a split-screen layout with Bootstrap
How to implement a card flip animation with Bootstrap
How to use Bootstrap’s table-responsive class
How to create a responsive login form with Bootstrap
How to use Bootstrap’s media objects
How to integrate Bootstrap with JavaScript libraries like jQuery
How to implement a modal/popup with Bootstrap