How does Next.js handle routing
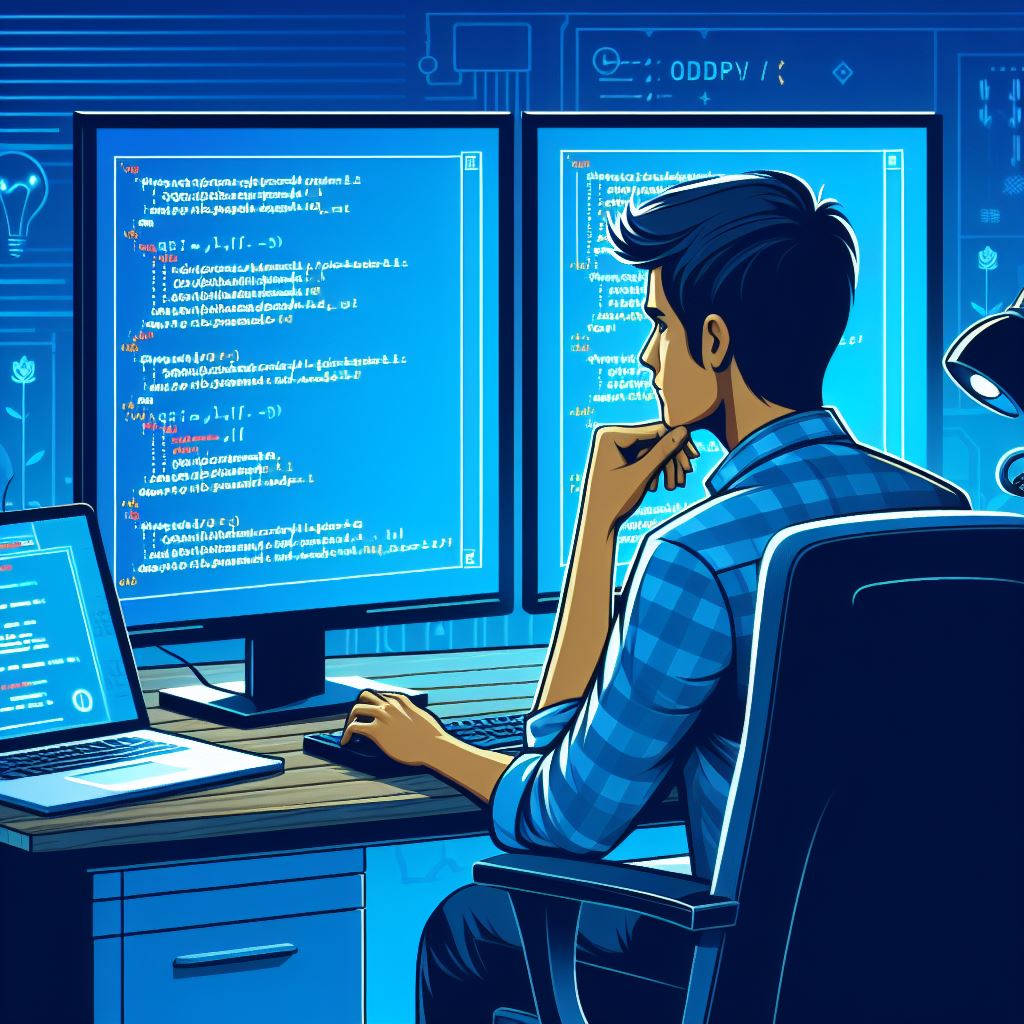
Routing is a fundamental aspect of web development, allowing users to navigate between different pages and content within an application. Next.js, a popular React framework, offers a powerful routing system that simplifies the development of client-side and server-side routes. In this extensive guide, we’ll delve into how Next.js handles routing, covering its features, mechanisms, best practices, and advanced techniques.
Understanding Next.js Routing
Next.js provides a versatile routing system that supports both client-side and server-side routing. It seamlessly integrates with React components and pages, allowing developers to define routes and handle navigation with ease. Key aspects of Next.js routing include:
- File-Based Routing: Next.js uses a file-based routing system, where each page is represented by a React component in the
pages
directory. The file structure directly corresponds to the URL structure, making it intuitive and easy to organize. - Dynamic Routes: Next.js supports dynamic routes, allowing for the creation of dynamic pages with URL parameters. Dynamic routes enable developers to build flexible and scalable applications that adapt to varying content and user interactions.
- Link Component: Next.js provides a
Link
component for client-side navigation between pages. TheLink
component pre-fetches linked pages in the background, ensuring fast and smooth transitions without full page reloads. - Programmatic Navigation: Next.js allows for programmatic navigation using the
Router
module from thenext/router
package. Developers can navigate to different pages programmatically based on user interactions or application logic.
File-Based Routing
Next.js follows a convention-over-configuration approach to routing, where the file structure directly corresponds to the URL structure. Each page in a Next.js application is represented by a React component placed in the pages
directory. The filename becomes the route path, and nested directories create nested routes.
pages/
|-- index.js // Route: /
|-- about.js // Route: /about
|-- products/
| |-- index.js // Route: /products
| |-- [id].js // Dynamic Route: /products/:id
With file-based routing, developers can easily organize and manage routes, resulting in a clean and intuitive project structure.
Dynamic Routes
Next.js supports dynamic routes, allowing for the creation of pages with dynamic content based on URL parameters. Dynamic routes are defined by placing square brackets []
around the parameter in the filename. Next.js automatically parses the URL and provides the parameter value to the corresponding page component.
// pages/products/[id].js
const ProductPage = ({ id }) => {
return (
<div>
<h1>Product ID: {id}</h1>
</div>
);
};
export async function getServerSideProps({ params }) {
const { id } = params;
return {
props: {
id,
},
};
}
export default ProductPage;
Dynamic routes enable developers to create pages that dynamically fetch data based on URL parameters, offering flexibility and scalability.
Link Component
Next.js provides a Link
component for client-side navigation between pages. The Link
component optimizes navigation by pre-fetching linked pages in the background, ensuring fast and smooth transitions without full page reloads.
import Link from 'next/link';
const HomePage = () => {
return (
<div>
<h1>Home Page</h1>
<Link href="/about">
<a>About</a>
</Link>
</div>
);
};
export default HomePage;
The Link
component simplifies client-side navigation and enhances the user experience by reducing loading times and providing seamless page transitions.
Programmatic Navigation
Next.js allows for programmatic navigation using the Router
module from the next/router
package. Developers can navigate to different pages programmatically based on user interactions, form submissions, or application logic.
import { useRouter } from 'next/router';
const LoginPage = () => {
const router = useRouter();
const handleLogin = () => {
// Perform login logic
// Redirect to dashboard after successful login
router.push('/dashboard');
};
return (
<div>
<h1>Login Page</h1>
<button onClick={handleLogin}>Login</button>
</div>
);
};
export default LoginPage;
Programmatic navigation empowers developers to create dynamic and interactive applications that respond to user actions in real-time.
Conclusion
Routing is a critical aspect of web development, enabling users to navigate between different pages and content within an application. Next.js provides a powerful routing system that simplifies the development of client-side and server-side routes. By leveraging features such as file-based routing, dynamic routes, the Link
component, and programmatic navigation, developers can create scalable, intuitive, and performant web applications with Next.js. Whether building simple static sites or complex dynamic applications, Next.js offers the flexibility and tools necessary to handle routing efficiently.
Explain the role of the babel.config.js file in a Next.js project
How can you implement server-side rendering for only certain parts of a page
How can you implement authentication with third-party providers in Next.js
What is the purpose of the noSsg option in getStaticProps
Explain the purpose of the Image component in Next.js
How to implement a custom loading state for data fetching in a Next.js app
How can you configure custom routes in Next.js
How can you implement a loading spinner for data fetching in Next.js
How can you handle state management in a Next.js application