How do I vertically center an element in Tailwind CSS
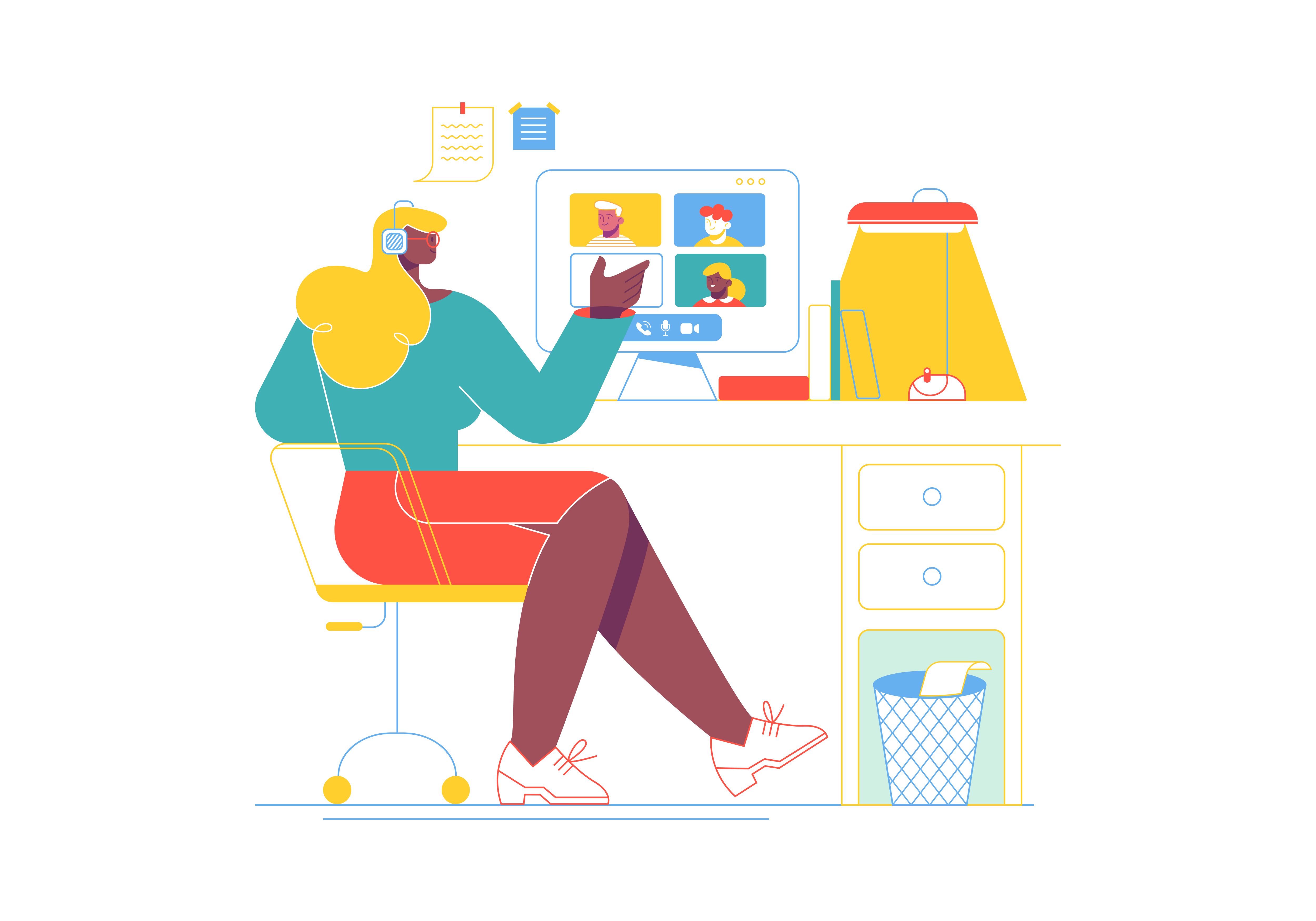
Vertical centering is a fundamental aspect of web design that can often prove challenging for developers. Tailwind CSS, with its utility-first approach, offers multiple elegant solutions for vertically centering elements. This comprehensive guide will walk you through various techniques, their use cases, and practical examples to help you master vertical alignment in your web projects.
Understanding Vertical Centering in Web Design
Before diving into specific Tailwind CSS techniques, it’s essential to understand why vertical centering matters. In responsive web design, creating visually balanced layouts is crucial for:
- Improving user experience
- Enhancing visual hierarchy
- Creating more professional-looking interfaces
- Ensuring content is easily readable across different device sizes
Tailwind CSS provides several utility classes that make vertical centering both simple and flexible.
Method 1: Flexbox Vertical Centering
Using align-items-center
Flexbox is the most straightforward and widely-used method for vertical centering in Tailwind CSS. The align-items-center
class is your go-to solution for simple vertical alignment.
<div class="flex align-items-center h-screen">
<div class="p-4 bg-gray-100">
Vertically Centered Content
</div>
</div>
Key points about this method:
- Works with both single and multiple items
- Centers items vertically within the container
- Can be combined with
justify-center
for both vertical and horizontal centering - Responsive and works across different screen sizes
Advanced Flexbox Centering
For more complex layouts, you can combine multiple Tailwind flex utilities:
<div class="flex flex-col justify-center items-center h-screen">
<div class="text-center">
<h1 class="text-2xl font-bold">Centered Heading</h1>
<p class="mt-4">Centered paragraph content</p>
</div>
</div>
This example demonstrates:
flex-col
: Stacks items verticallyjustify-center
: Centers content verticallyitems-center
: Centers content horizontallyh-screen
: Full viewport height
Method 2: Grid-Based Vertical Centering
CSS Grid provides another powerful method for vertical centering in Tailwind CSS.
<div class="grid place-items-center h-screen">
<div class="bg-blue-100 p-6 rounded">
Grid-Centered Content
</div>
</div>
Advantages of grid centering:
place-items-center
simultaneously centers content vertically and horizontally- Works well for single or multiple items
- Provides a more modern alternative to flexbox
Method 3: Absolute Positioning Technique
For scenarios requiring precise positioning, Tailwind’s positioning utilities offer a solution:
<div class="relative h-screen">
<div class="absolute top-1/2 left-1/2 transform -translate-x-1/2 -translate-y-1/2">
Absolutely Centered Content
</div>
</div>
Breaking down this approach:
relative
on the parent containerabsolute
on the child elementtop-1/2
andleft-1/2
move the element halfwaytransform
and negative translate classes center precisely- Useful for modal dialogs, overlays, and complex layouts
Responsive Vertical Centering
Tailwind CSS makes responsive vertical centering effortless:
<div class="flex sm:block md:flex lg:grid items-center justify-center h-screen">
<div class="p-4">
Responsively Centered Content
</div>
</div>
This example demonstrates:
- Different centering strategies at various breakpoints
- Flexibility across device sizes
- Tailwind’s mobile-first responsive design approach
Performance Considerations
While Tailwind CSS provides multiple centering methods, consider these performance tips:
- Flexbox Performance: Generally the most performant method
- Grid Performance: Slightly more complex but still efficient
- Absolute Positioning: Can be less performant, use sparingly
Common Pitfalls and Best Practices
Avoid Overcomplicating Layouts
- Choose the simplest method that meets your design requirements
- Don’t nest too many centered containers
Browser Compatibility
- Flexbox and Grid are well-supported in modern browsers
- Always test across different devices and browsers
Accessibility Considerations
- Ensure centered content remains readable
- Maintain proper color contrast
- Consider screen reader experience
Tailwind Configuration Tips
You can customize vertical centering in your tailwind.config.js
:
module.exports = {
theme: {
extend: {
display: ['flex', 'grid'],
alignItems: ['center'],
justifyContent: ['center']
}
}
}
Conclusion
Vertical centering in Tailwind CSS is more than just a technical implementation—it’s an art of creating balanced, responsive designs. By understanding and leveraging flexbox, grid, and positioning utilities, you can create visually appealing layouts with minimal effort.
Recommended Workflow
- Start with flexbox for most use cases
- Use grid for more complex layouts
- Resort to absolute positioning sparingly
- Always test responsiveness
Mastering these techniques will significantly enhance your web development skills and allow you to create more professional, visually appealing interfaces.
Further Resources
Remember, the key to great design is not just knowing the techniques, but knowing when and how to apply them.
What is the purpose of the “purge” option in the Tailwind CSS configuration
What are the utility classes for responsive design in Tailwind CSS
How do I create a fixed navbar using Tailwind CSS
How do I create a custom plugin in Tailwind CSS
How can I integrate Tailwind CSS with a CSS-in-JS solution like Emotion or Styled Components
What is the difference between the minified and unminified versions of Tailwind CSS